Google drive api folder permissions
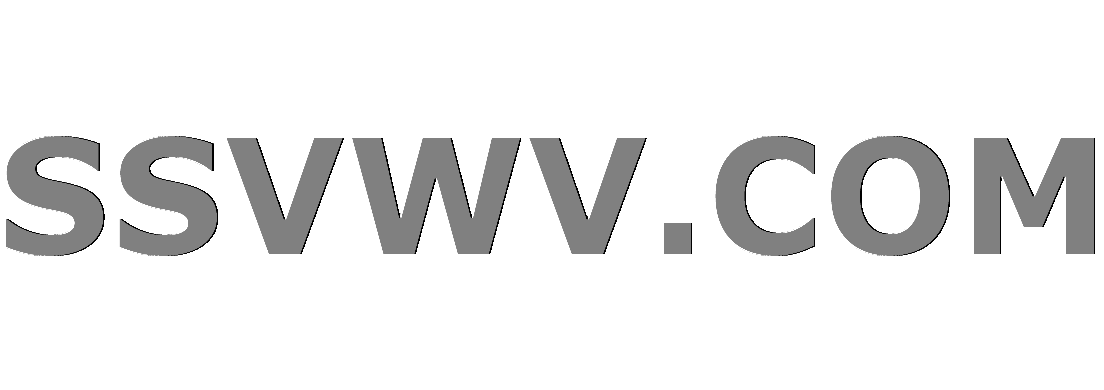
Multi tool use
up vote
0
down vote
favorite
I have problem with google drive api v3.
I took google example and I tried. I could read folder content but I did nothing with folder permission.
I received error 403, "Insufficient Permission".
I can't create or read all permissions for the folder.
<?php
require_once __DIR__.'/vendor/autoload.php';
$client = new Google_Client();
$client->setAuthConfig('client_secrets.json');
$client->addScope(Google_Service_Drive::DRIVE);
$client->setAccessType('offline');
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$drive = new Google_Service_Drive($client);
$folderId = '1_ip3-WUs4F6atNdElJ3KHccAV4lI0nLL';
$optParams = array(
'pageSize' => 100,
'fields' => "nextPageToken, files(id,name)",
'q' => "'".$folderId."' in parents"
);
$results = $drive->files->listFiles($optParams);
if (count($results->getFiles()) != 0) {
foreach ($results->getFiles() as $file) {
echo "Id: " . $file->getId() . " Name: " . $file->getName() . "<br>";
}
}
} else {
$redirect_uri = 'http://' . $_SERVER['HTTP_HOST'] . '/oauth2callback.php';
header('Location: ' . filter_var($redirect_uri, FILTER_SANITIZE_URL));
}
//+!+!+!+!+!+!+!+!+!+! Next code doesn't WORK !+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!//
$fileId = '1UXSg5W-XIX82izGK8uEXXWPUCLcDGRa1';
$userPermission = new Google_Service_Drive_Permission(array(
'type' => 'user',
'role' => 'reader',
'emailAddress' => 'email@gmail.com'
));
$request = $drive->permissions->create($fileId, $userPermission, array('fields' => 'id'));
echo $request;
?>
google-api

add a comment |
up vote
0
down vote
favorite
I have problem with google drive api v3.
I took google example and I tried. I could read folder content but I did nothing with folder permission.
I received error 403, "Insufficient Permission".
I can't create or read all permissions for the folder.
<?php
require_once __DIR__.'/vendor/autoload.php';
$client = new Google_Client();
$client->setAuthConfig('client_secrets.json');
$client->addScope(Google_Service_Drive::DRIVE);
$client->setAccessType('offline');
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$drive = new Google_Service_Drive($client);
$folderId = '1_ip3-WUs4F6atNdElJ3KHccAV4lI0nLL';
$optParams = array(
'pageSize' => 100,
'fields' => "nextPageToken, files(id,name)",
'q' => "'".$folderId."' in parents"
);
$results = $drive->files->listFiles($optParams);
if (count($results->getFiles()) != 0) {
foreach ($results->getFiles() as $file) {
echo "Id: " . $file->getId() . " Name: " . $file->getName() . "<br>";
}
}
} else {
$redirect_uri = 'http://' . $_SERVER['HTTP_HOST'] . '/oauth2callback.php';
header('Location: ' . filter_var($redirect_uri, FILTER_SANITIZE_URL));
}
//+!+!+!+!+!+!+!+!+!+! Next code doesn't WORK !+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!//
$fileId = '1UXSg5W-XIX82izGK8uEXXWPUCLcDGRa1';
$userPermission = new Google_Service_Drive_Permission(array(
'type' => 'user',
'role' => 'reader',
'emailAddress' => 'email@gmail.com'
));
$request = $drive->permissions->create($fileId, $userPermission, array('fields' => 'id'));
echo $request;
?>
google-api

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have problem with google drive api v3.
I took google example and I tried. I could read folder content but I did nothing with folder permission.
I received error 403, "Insufficient Permission".
I can't create or read all permissions for the folder.
<?php
require_once __DIR__.'/vendor/autoload.php';
$client = new Google_Client();
$client->setAuthConfig('client_secrets.json');
$client->addScope(Google_Service_Drive::DRIVE);
$client->setAccessType('offline');
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$drive = new Google_Service_Drive($client);
$folderId = '1_ip3-WUs4F6atNdElJ3KHccAV4lI0nLL';
$optParams = array(
'pageSize' => 100,
'fields' => "nextPageToken, files(id,name)",
'q' => "'".$folderId."' in parents"
);
$results = $drive->files->listFiles($optParams);
if (count($results->getFiles()) != 0) {
foreach ($results->getFiles() as $file) {
echo "Id: " . $file->getId() . " Name: " . $file->getName() . "<br>";
}
}
} else {
$redirect_uri = 'http://' . $_SERVER['HTTP_HOST'] . '/oauth2callback.php';
header('Location: ' . filter_var($redirect_uri, FILTER_SANITIZE_URL));
}
//+!+!+!+!+!+!+!+!+!+! Next code doesn't WORK !+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!//
$fileId = '1UXSg5W-XIX82izGK8uEXXWPUCLcDGRa1';
$userPermission = new Google_Service_Drive_Permission(array(
'type' => 'user',
'role' => 'reader',
'emailAddress' => 'email@gmail.com'
));
$request = $drive->permissions->create($fileId, $userPermission, array('fields' => 'id'));
echo $request;
?>
google-api

I have problem with google drive api v3.
I took google example and I tried. I could read folder content but I did nothing with folder permission.
I received error 403, "Insufficient Permission".
I can't create or read all permissions for the folder.
<?php
require_once __DIR__.'/vendor/autoload.php';
$client = new Google_Client();
$client->setAuthConfig('client_secrets.json');
$client->addScope(Google_Service_Drive::DRIVE);
$client->setAccessType('offline');
if (isset($_SESSION['access_token']) && $_SESSION['access_token']) {
$client->setAccessToken($_SESSION['access_token']);
$drive = new Google_Service_Drive($client);
$folderId = '1_ip3-WUs4F6atNdElJ3KHccAV4lI0nLL';
$optParams = array(
'pageSize' => 100,
'fields' => "nextPageToken, files(id,name)",
'q' => "'".$folderId."' in parents"
);
$results = $drive->files->listFiles($optParams);
if (count($results->getFiles()) != 0) {
foreach ($results->getFiles() as $file) {
echo "Id: " . $file->getId() . " Name: " . $file->getName() . "<br>";
}
}
} else {
$redirect_uri = 'http://' . $_SERVER['HTTP_HOST'] . '/oauth2callback.php';
header('Location: ' . filter_var($redirect_uri, FILTER_SANITIZE_URL));
}
//+!+!+!+!+!+!+!+!+!+! Next code doesn't WORK !+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!+!//
$fileId = '1UXSg5W-XIX82izGK8uEXXWPUCLcDGRa1';
$userPermission = new Google_Service_Drive_Permission(array(
'type' => 'user',
'role' => 'reader',
'emailAddress' => 'email@gmail.com'
));
$request = $drive->permissions->create($fileId, $userPermission, array('fields' => 'id'));
echo $request;
?>
google-api

google-api

asked Nov 11 at 10:21
Alex
62
62
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
It may be that wrong permissions have been set when creating the folder, even though you said you did nothing. I do not see the code for creating the folder here, just an ID, which indicates that the folder has already been created.
I suggest trying to list the permissions using Drive.Permissions.List
first. Particularly, look at the type
and role
or teamDrivePermissionDetails.role
properties. See the permissions guide on what operations each role or type can do. You can also visit this documentation on managing sharing in case you need to.
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
It may be that wrong permissions have been set when creating the folder, even though you said you did nothing. I do not see the code for creating the folder here, just an ID, which indicates that the folder has already been created.
I suggest trying to list the permissions using Drive.Permissions.List
first. Particularly, look at the type
and role
or teamDrivePermissionDetails.role
properties. See the permissions guide on what operations each role or type can do. You can also visit this documentation on managing sharing in case you need to.
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
add a comment |
up vote
2
down vote
It may be that wrong permissions have been set when creating the folder, even though you said you did nothing. I do not see the code for creating the folder here, just an ID, which indicates that the folder has already been created.
I suggest trying to list the permissions using Drive.Permissions.List
first. Particularly, look at the type
and role
or teamDrivePermissionDetails.role
properties. See the permissions guide on what operations each role or type can do. You can also visit this documentation on managing sharing in case you need to.
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
add a comment |
up vote
2
down vote
up vote
2
down vote
It may be that wrong permissions have been set when creating the folder, even though you said you did nothing. I do not see the code for creating the folder here, just an ID, which indicates that the folder has already been created.
I suggest trying to list the permissions using Drive.Permissions.List
first. Particularly, look at the type
and role
or teamDrivePermissionDetails.role
properties. See the permissions guide on what operations each role or type can do. You can also visit this documentation on managing sharing in case you need to.
It may be that wrong permissions have been set when creating the folder, even though you said you did nothing. I do not see the code for creating the folder here, just an ID, which indicates that the folder has already been created.
I suggest trying to list the permissions using Drive.Permissions.List
first. Particularly, look at the type
and role
or teamDrivePermissionDetails.role
properties. See the permissions guide on what operations each role or type can do. You can also visit this documentation on managing sharing in case you need to.
answered Nov 12 at 6:28
Jacque
2066
2066
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
add a comment |
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
perhaps also worth looking at the scopes of the project. have you obtained sufficient project scope permission for the API to access. Consider adding 'googleapis.com/auth/drive' to the OAUTH scope
– Peter Scott
Nov 12 at 16:05
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
Maybe it was a reason for problem. I have changed the scope of the project and now I have to wait couple of days for checking.
– Alex
Nov 12 at 17:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53247778%2fgoogle-drive-api-folder-permissions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ITr906hZWXx3mPV18p,6CiAir9xo8F0vFtI2dqP4LLi