non-persisting state between function calls in React.js
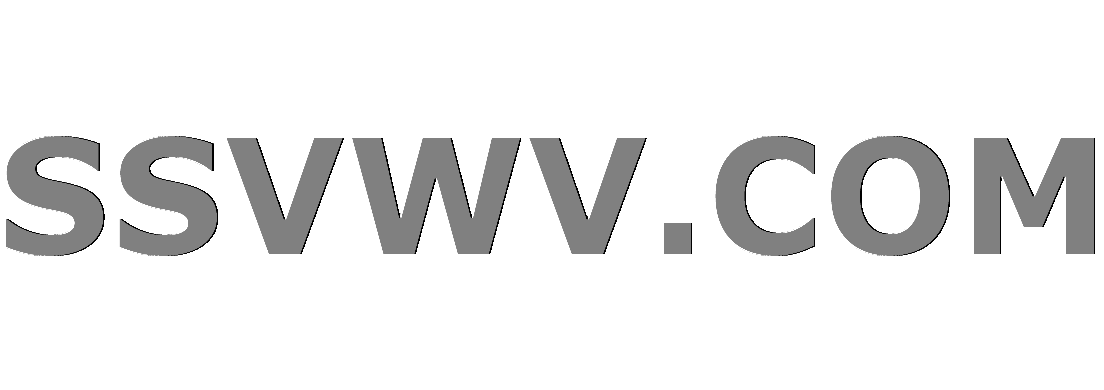
Multi tool use
up vote
0
down vote
favorite
Generally, I am trying to load some data when component loads (in initialiseSellerData() ) but I only want to do this once, i.e. if page is refreshed it doesn't do it again. Please see attempt below and description of specific problem beneath
class Index extends React.Component {
constructor(props) {
super(props);
this.state = {
initialised: false
};
}
componentDidMount = () =>
{
this.initialised()
if(this.state.initialised == false)
{
this.initialiseSellerData();
}
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
this.setState({initialised: true})
}
}
The problem I am having is that in componentDidMount, initialised() function is called correctly and initialises state gets set to true however then when do I the check for initialised it returns false (should return true). Why isn't my state persisting / being passed between function calls.
reactjs ethereum
add a comment |
up vote
0
down vote
favorite
Generally, I am trying to load some data when component loads (in initialiseSellerData() ) but I only want to do this once, i.e. if page is refreshed it doesn't do it again. Please see attempt below and description of specific problem beneath
class Index extends React.Component {
constructor(props) {
super(props);
this.state = {
initialised: false
};
}
componentDidMount = () =>
{
this.initialised()
if(this.state.initialised == false)
{
this.initialiseSellerData();
}
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
this.setState({initialised: true})
}
}
The problem I am having is that in componentDidMount, initialised() function is called correctly and initialises state gets set to true however then when do I the check for initialised it returns false (should return true). Why isn't my state persisting / being passed between function calls.
reactjs ethereum
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Generally, I am trying to load some data when component loads (in initialiseSellerData() ) but I only want to do this once, i.e. if page is refreshed it doesn't do it again. Please see attempt below and description of specific problem beneath
class Index extends React.Component {
constructor(props) {
super(props);
this.state = {
initialised: false
};
}
componentDidMount = () =>
{
this.initialised()
if(this.state.initialised == false)
{
this.initialiseSellerData();
}
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
this.setState({initialised: true})
}
}
The problem I am having is that in componentDidMount, initialised() function is called correctly and initialises state gets set to true however then when do I the check for initialised it returns false (should return true). Why isn't my state persisting / being passed between function calls.
reactjs ethereum
Generally, I am trying to load some data when component loads (in initialiseSellerData() ) but I only want to do this once, i.e. if page is refreshed it doesn't do it again. Please see attempt below and description of specific problem beneath
class Index extends React.Component {
constructor(props) {
super(props);
this.state = {
initialised: false
};
}
componentDidMount = () =>
{
this.initialised()
if(this.state.initialised == false)
{
this.initialiseSellerData();
}
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
this.setState({initialised: true})
}
}
The problem I am having is that in componentDidMount, initialised() function is called correctly and initialises state gets set to true however then when do I the check for initialised it returns false (should return true). Why isn't my state persisting / being passed between function calls.
reactjs ethereum
reactjs ethereum
edited Nov 11 at 13:58
asked Nov 11 at 13:52
Chris Johnston
124
124
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
I think you forgot to use await
. initialised
is an async function. so the calling function should use await
or then
to handle the promise.
componentDidMount = async () =>
{
await this.initialised()
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
await initialiseSellerData();
this.setState({initialised: true});
}
}
and even then it may not working sincesetState
call inside is not guaranteed to be sync
– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I think you forgot to use await
. initialised
is an async function. so the calling function should use await
or then
to handle the promise.
componentDidMount = async () =>
{
await this.initialised()
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
await initialiseSellerData();
this.setState({initialised: true});
}
}
and even then it may not working sincesetState
call inside is not guaranteed to be sync
– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
add a comment |
up vote
0
down vote
accepted
I think you forgot to use await
. initialised
is an async function. so the calling function should use await
or then
to handle the promise.
componentDidMount = async () =>
{
await this.initialised()
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
await initialiseSellerData();
this.setState({initialised: true});
}
}
and even then it may not working sincesetState
call inside is not guaranteed to be sync
– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I think you forgot to use await
. initialised
is an async function. so the calling function should use await
or then
to handle the promise.
componentDidMount = async () =>
{
await this.initialised()
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
await initialiseSellerData();
this.setState({initialised: true});
}
}
I think you forgot to use await
. initialised
is an async function. so the calling function should use await
or then
to handle the promise.
componentDidMount = async () =>
{
await this.initialised()
}
initialiseSellerData = async () =>
{
// this function makes a call to contract to initialise data
}
initialised = async () =>
{
const {sellContract } = this.props
const response = await sellContract.methods.initialised().call()
if(response == true)
{
await initialiseSellerData();
this.setState({initialised: true});
}
}
edited Nov 11 at 14:15
answered Nov 11 at 14:03
KitKarson
83821535
83821535
and even then it may not working sincesetState
call inside is not guaranteed to be sync
– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
add a comment |
and even then it may not working sincesetState
call inside is not guaranteed to be sync
– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
and even then it may not working since
setState
call inside is not guaranteed to be sync– skyboyer
Nov 11 at 14:08
and even then it may not working since
setState
call inside is not guaranteed to be sync– skyboyer
Nov 11 at 14:08
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
worked for me, thanks @KitKarson
– Chris Johnston
Nov 11 at 14:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53249423%2fnon-persisting-state-between-function-calls-in-react-js%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gDC,En L sm40wIcPfEy1UUzhvM,ILnWvb7fSRAxDPwrrD0YhjTTF6UHFoJ TvZ 9Cfb Uc,uy1km2U6,0vuPZ4HLFYZsSy Adt