OpenStream throws IOException in AsyncTask
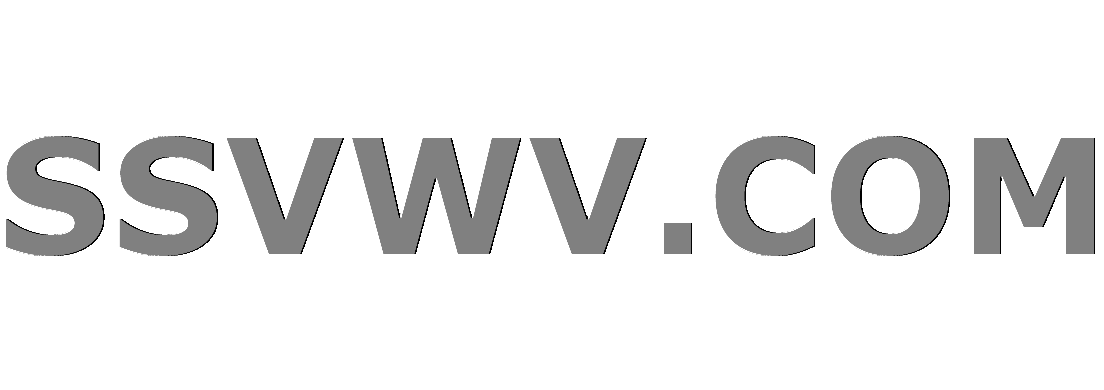
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Context:
I'm developing an Android weather application in Java for a course. This application uses asynctask in order to get from OpenWeather's API the temperature of a city. The API returns a JSON with weather information through an URL like this:
http://api.openweathermap.org/data/2.5/weather?q={city name}&appid={key}
APPID is a key associated to an account in OpenWeather. Here is a JSON example for Berlin city:
{"coord":{"lon":13.39,"lat":52.52},"weather":[{"id":800,"main":"Clear","description":"clear sky","icon":"01d"}],"base":"stations","main":{"temp":8.49,"pressure":1035,"humidity":61,"temp_min":8,"temp_max":9},"visibility":10000,"wind":{"speed":6.7,"deg":120},"clouds":{"all":0},"dt":1542369000,"sys":{"type":1,"id":4892,"message":0.0027,"country":"DE","sunrise":1542349837,"sunset":1542381079},"id":2950159,"name":"Berlin","cod":200}
If the city doesn't exists, the API returns a JSON with error 404. The next one is a JSON example for the nonexistent city Berlina.
{"cod":"404","message":"city not found"}
I get the JSON with Asynctask. In doInBackground() method, I call the following line in order to get an inputStream:
InputStream inputStream = new URL(url).openStream();
Then, I parse the JSON in postOnExecute() and show the temperature information with a Toast.
My question:
The code works fine with Berlin, but openStream throws IOException for Berlina. The URL exists and have JSON data, as I explained. So, what could be the reasons for this Exception? Could it be a connection problem?
Edit with Logcat
This is the exception detail:
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp D/IOException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp W/System.err: java.io.FileNotFoundException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.android.okhttp.internal.http.HttpURLConnectionImpl.getInputStream(HttpURLConnectionImpl.java:206)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.net.URL.openStream(URL.java:470)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:219)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:204)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$2.call(AsyncTask.java:292)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.FutureTask.run(FutureTask.java:237)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$SerialExecutor$1.run(AsyncTask.java:231)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.lang.Thread.run(Thread.java:818)
11-27 00:01:34.192 3152-3180/com.androidapps.user.weatherapp D/EGL_emulation: eglMakeCurrent: 0xb42b9b20: ver 2 0
11-27 00:01:34.227 3152-3152/com.androidapps.user.weatherapp D/JSONObjetc: End of input at character 0 of

|
show 3 more comments
Context:
I'm developing an Android weather application in Java for a course. This application uses asynctask in order to get from OpenWeather's API the temperature of a city. The API returns a JSON with weather information through an URL like this:
http://api.openweathermap.org/data/2.5/weather?q={city name}&appid={key}
APPID is a key associated to an account in OpenWeather. Here is a JSON example for Berlin city:
{"coord":{"lon":13.39,"lat":52.52},"weather":[{"id":800,"main":"Clear","description":"clear sky","icon":"01d"}],"base":"stations","main":{"temp":8.49,"pressure":1035,"humidity":61,"temp_min":8,"temp_max":9},"visibility":10000,"wind":{"speed":6.7,"deg":120},"clouds":{"all":0},"dt":1542369000,"sys":{"type":1,"id":4892,"message":0.0027,"country":"DE","sunrise":1542349837,"sunset":1542381079},"id":2950159,"name":"Berlin","cod":200}
If the city doesn't exists, the API returns a JSON with error 404. The next one is a JSON example for the nonexistent city Berlina.
{"cod":"404","message":"city not found"}
I get the JSON with Asynctask. In doInBackground() method, I call the following line in order to get an inputStream:
InputStream inputStream = new URL(url).openStream();
Then, I parse the JSON in postOnExecute() and show the temperature information with a Toast.
My question:
The code works fine with Berlin, but openStream throws IOException for Berlina. The URL exists and have JSON data, as I explained. So, what could be the reasons for this Exception? Could it be a connection problem?
Edit with Logcat
This is the exception detail:
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp D/IOException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp W/System.err: java.io.FileNotFoundException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.android.okhttp.internal.http.HttpURLConnectionImpl.getInputStream(HttpURLConnectionImpl.java:206)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.net.URL.openStream(URL.java:470)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:219)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:204)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$2.call(AsyncTask.java:292)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.FutureTask.run(FutureTask.java:237)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$SerialExecutor$1.run(AsyncTask.java:231)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.lang.Thread.run(Thread.java:818)
11-27 00:01:34.192 3152-3180/com.androidapps.user.weatherapp D/EGL_emulation: eglMakeCurrent: 0xb42b9b20: ver 2 0
11-27 00:01:34.227 3152-3152/com.androidapps.user.weatherapp D/JSONObjetc: End of input at character 0 of

What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
1
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21
|
show 3 more comments
Context:
I'm developing an Android weather application in Java for a course. This application uses asynctask in order to get from OpenWeather's API the temperature of a city. The API returns a JSON with weather information through an URL like this:
http://api.openweathermap.org/data/2.5/weather?q={city name}&appid={key}
APPID is a key associated to an account in OpenWeather. Here is a JSON example for Berlin city:
{"coord":{"lon":13.39,"lat":52.52},"weather":[{"id":800,"main":"Clear","description":"clear sky","icon":"01d"}],"base":"stations","main":{"temp":8.49,"pressure":1035,"humidity":61,"temp_min":8,"temp_max":9},"visibility":10000,"wind":{"speed":6.7,"deg":120},"clouds":{"all":0},"dt":1542369000,"sys":{"type":1,"id":4892,"message":0.0027,"country":"DE","sunrise":1542349837,"sunset":1542381079},"id":2950159,"name":"Berlin","cod":200}
If the city doesn't exists, the API returns a JSON with error 404. The next one is a JSON example for the nonexistent city Berlina.
{"cod":"404","message":"city not found"}
I get the JSON with Asynctask. In doInBackground() method, I call the following line in order to get an inputStream:
InputStream inputStream = new URL(url).openStream();
Then, I parse the JSON in postOnExecute() and show the temperature information with a Toast.
My question:
The code works fine with Berlin, but openStream throws IOException for Berlina. The URL exists and have JSON data, as I explained. So, what could be the reasons for this Exception? Could it be a connection problem?
Edit with Logcat
This is the exception detail:
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp D/IOException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp W/System.err: java.io.FileNotFoundException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.android.okhttp.internal.http.HttpURLConnectionImpl.getInputStream(HttpURLConnectionImpl.java:206)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.net.URL.openStream(URL.java:470)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:219)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:204)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$2.call(AsyncTask.java:292)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.FutureTask.run(FutureTask.java:237)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$SerialExecutor$1.run(AsyncTask.java:231)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.lang.Thread.run(Thread.java:818)
11-27 00:01:34.192 3152-3180/com.androidapps.user.weatherapp D/EGL_emulation: eglMakeCurrent: 0xb42b9b20: ver 2 0
11-27 00:01:34.227 3152-3152/com.androidapps.user.weatherapp D/JSONObjetc: End of input at character 0 of

Context:
I'm developing an Android weather application in Java for a course. This application uses asynctask in order to get from OpenWeather's API the temperature of a city. The API returns a JSON with weather information through an URL like this:
http://api.openweathermap.org/data/2.5/weather?q={city name}&appid={key}
APPID is a key associated to an account in OpenWeather. Here is a JSON example for Berlin city:
{"coord":{"lon":13.39,"lat":52.52},"weather":[{"id":800,"main":"Clear","description":"clear sky","icon":"01d"}],"base":"stations","main":{"temp":8.49,"pressure":1035,"humidity":61,"temp_min":8,"temp_max":9},"visibility":10000,"wind":{"speed":6.7,"deg":120},"clouds":{"all":0},"dt":1542369000,"sys":{"type":1,"id":4892,"message":0.0027,"country":"DE","sunrise":1542349837,"sunset":1542381079},"id":2950159,"name":"Berlin","cod":200}
If the city doesn't exists, the API returns a JSON with error 404. The next one is a JSON example for the nonexistent city Berlina.
{"cod":"404","message":"city not found"}
I get the JSON with Asynctask. In doInBackground() method, I call the following line in order to get an inputStream:
InputStream inputStream = new URL(url).openStream();
Then, I parse the JSON in postOnExecute() and show the temperature information with a Toast.
My question:
The code works fine with Berlin, but openStream throws IOException for Berlina. The URL exists and have JSON data, as I explained. So, what could be the reasons for this Exception? Could it be a connection problem?
Edit with Logcat
This is the exception detail:
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp D/IOException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.151 3152-3346/com.androidapps.user.weatherapp W/System.err: java.io.FileNotFoundException: http://api.openweathermap.org/data/2.5/weather?q=Berlina&units=metric&appid=d32068a850405b593a87d410fcc50a4f
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.android.okhttp.internal.http.HttpURLConnectionImpl.getInputStream(HttpURLConnectionImpl.java:206)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.net.URL.openStream(URL.java:470)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:219)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at com.androidapps.user.weatherapp.MainActivity$GetJSONOpenWeather.doInBackground(MainActivity.java:204)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$2.call(AsyncTask.java:292)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.FutureTask.run(FutureTask.java:237)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at android.os.AsyncTask$SerialExecutor$1.run(AsyncTask.java:231)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1112)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:587)
11-27 00:01:34.152 3152-3346/com.androidapps.user.weatherapp W/System.err: at java.lang.Thread.run(Thread.java:818)
11-27 00:01:34.192 3152-3180/com.androidapps.user.weatherapp D/EGL_emulation: eglMakeCurrent: 0xb42b9b20: ver 2 0
11-27 00:01:34.227 3152-3152/com.androidapps.user.weatherapp D/JSONObjetc: End of input at character 0 of


edited Nov 27 '18 at 0:11
JCMiguel
asked Nov 16 '18 at 15:15
JCMiguelJCMiguel
638
638
What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
1
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21
|
show 3 more comments
What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
1
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21
What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
1
1
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21
|
show 3 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53340599%2fopenstream-throws-ioexception-in-asynctask%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53340599%2fopenstream-throws-ioexception-in-asynctask%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
d11MdNwcyk9xHj4C,S7OX9L8Far bxePYs4G26OsEvYv32
What is the exception? Your log should show it unless you're swallowing it.
– nasch
Nov 16 '18 at 15:21
How can I know what is the exception reason? The getMessage() method of IOException shows only the URL.
– JCMiguel
Nov 16 '18 at 15:32
1
Exception.printStackTrace()
– nasch
Nov 16 '18 at 15:34
It throws java.io.FileNotFoundException in the URL, but I understand it. I see in the browser that the JSON with the error exists.
– JCMiguel
Nov 21 '18 at 23:16
I read the next paragraph about the exception in the documentation: "It will also be thrown by these constructors if the file does exist but for some reason is inaccessible, for example when an attempt is made to open a read-only file for writing". So, the JSON is inaccesible for the app. Why?
– JCMiguel
Nov 21 '18 at 23:21