Django multifile upload doesn't send files to directory
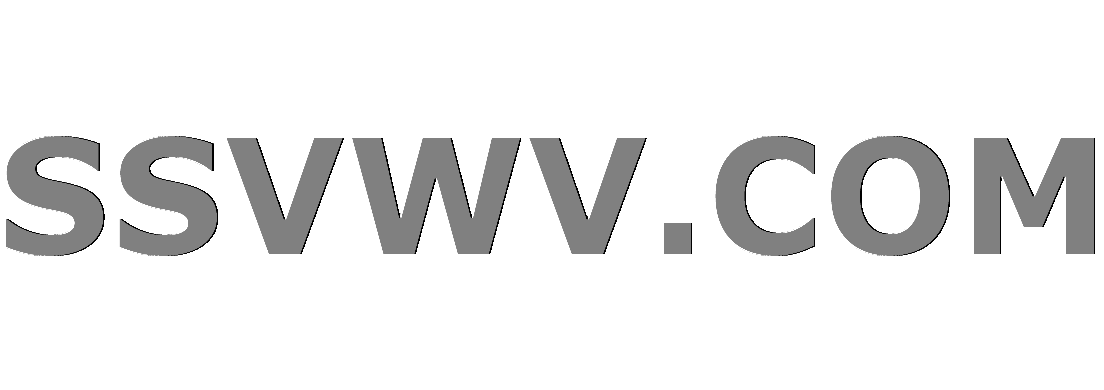
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'd like to implement a multifile upload feature into a site. There is already an upload feature on the site, but for single files. I'm following loosely the Simple Is Better Than Complex: Django Multiple Files Upload Using Ajax.
My code:
views->mulit_upload.py
class BasicUploadView(View):
def get(self, request):
files_list = Multi_File.objects.all()
#files_list = ['testing_123']
print ("files_list: {0}".format(files_list))
return render(self.request, 'myproject/multi_upload.html', {'filesList': files_list})
def post(self, request):
form = FileForm(self.request.POST, self.request.FILES)
if form.is_valid():
multiFile = form.save()
data = {'is_valid': True, 'name': multiFile.file.name, 'url': multiFile.file.url}
else:
data = {'is_valid': False}
return JsonResponse(data)
models.py
class Multi_File(models.Model):
title = models.CharField(max_length=255, blank=True)
file = models.FileField(upload_to='files/')
uploaded_at = models.DateTimeField(auto_now_add=True)
forms.py
class FileForm(forms.ModelForm):
class Meta:
model = Multi_File
fields = ('file', )
multi_upload.html
{% load static %}
{% block javascript %}
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/vendor/jquery.ui.widget.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.iframe-transport.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.fileupload.js' %}"></script>
<script src="{% static 'myproject/upload-files.js' %}"></script>
{% endblock %}
{# 1. BUTTON TO TRIGGER THE ACTION #}
<div class="col-md-4">
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="myfile" multiple>
<button type="submit">Upload<span class="glyphicon glyphicon-cloud-upload"></span> </button>
</form>
</div>
{# 2. FILE INPUT TO BE USED BY THE PLUG-IN #}
<input id="fileupload" type="file" name="myfile" multiple
style="display: none;"
data-url="{% url 'myproject:multi_import' %}"
data-form-data='{"csrfmiddlewaretoken": "{{ csrf_token }}"}'>
{# 3. TABLE TO DISPLAY THE UPLOADED PHOTOS #}
<table id="gallery" class="table table-bordered">
<thead>
<tr>
<th>Uploaded Files:</th>
</tr>
</thead>
<tbody>
{% for aFile in filesList %}
<tr>
<td><a href="{{ aFile.file.url }}">{{ aFile.file.name }}</a></td>
</tr>
{% endfor %}
</tbody>
</table>
upload_files.js
$(function () {
/* 1. OPEN THE FILE EXPLORER WINDOW */
$(".js-upload-files").click(function () {
$("#fileupload").click();
});
/* 2. INITIALIZE THE FILE UPLOAD COMPONENT */
$("#fileupload").fileupload({
dataType: 'json',
done: function (e, data) { /* 3. PROCESS THE RESPONSE FROM THE SERVER */
if (data.result.is_valid) {
$("#gallery tbody").prepend(
"<tr><td><a href='" + data.result.url + "'>" + data.result.name + "</a></td></tr>"
)
}
}
});
});
Problems so far:
1. Upload doesn't work when clicking on the button. The box appears, I can select numerous files and "upload" to the page i.e. it says 2 files selected. But then clicking "Upload" gives the error:
{"is_valid": false}
This comes from the view (mulit_uploade.py
) but I'm a bit confused why
I'd like to use the
base.html
that was also used for the single upload feature. So when I add{% extends "myproject/base.html" %}
to themulti_upload.html
, a new problem appears that doesn't appear when I just use the single upload feature:
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name:
Learn more »
NoReverseMatch at /myproject/import/
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name
django python-3.x django-uploads
add a comment |
I'd like to implement a multifile upload feature into a site. There is already an upload feature on the site, but for single files. I'm following loosely the Simple Is Better Than Complex: Django Multiple Files Upload Using Ajax.
My code:
views->mulit_upload.py
class BasicUploadView(View):
def get(self, request):
files_list = Multi_File.objects.all()
#files_list = ['testing_123']
print ("files_list: {0}".format(files_list))
return render(self.request, 'myproject/multi_upload.html', {'filesList': files_list})
def post(self, request):
form = FileForm(self.request.POST, self.request.FILES)
if form.is_valid():
multiFile = form.save()
data = {'is_valid': True, 'name': multiFile.file.name, 'url': multiFile.file.url}
else:
data = {'is_valid': False}
return JsonResponse(data)
models.py
class Multi_File(models.Model):
title = models.CharField(max_length=255, blank=True)
file = models.FileField(upload_to='files/')
uploaded_at = models.DateTimeField(auto_now_add=True)
forms.py
class FileForm(forms.ModelForm):
class Meta:
model = Multi_File
fields = ('file', )
multi_upload.html
{% load static %}
{% block javascript %}
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/vendor/jquery.ui.widget.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.iframe-transport.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.fileupload.js' %}"></script>
<script src="{% static 'myproject/upload-files.js' %}"></script>
{% endblock %}
{# 1. BUTTON TO TRIGGER THE ACTION #}
<div class="col-md-4">
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="myfile" multiple>
<button type="submit">Upload<span class="glyphicon glyphicon-cloud-upload"></span> </button>
</form>
</div>
{# 2. FILE INPUT TO BE USED BY THE PLUG-IN #}
<input id="fileupload" type="file" name="myfile" multiple
style="display: none;"
data-url="{% url 'myproject:multi_import' %}"
data-form-data='{"csrfmiddlewaretoken": "{{ csrf_token }}"}'>
{# 3. TABLE TO DISPLAY THE UPLOADED PHOTOS #}
<table id="gallery" class="table table-bordered">
<thead>
<tr>
<th>Uploaded Files:</th>
</tr>
</thead>
<tbody>
{% for aFile in filesList %}
<tr>
<td><a href="{{ aFile.file.url }}">{{ aFile.file.name }}</a></td>
</tr>
{% endfor %}
</tbody>
</table>
upload_files.js
$(function () {
/* 1. OPEN THE FILE EXPLORER WINDOW */
$(".js-upload-files").click(function () {
$("#fileupload").click();
});
/* 2. INITIALIZE THE FILE UPLOAD COMPONENT */
$("#fileupload").fileupload({
dataType: 'json',
done: function (e, data) { /* 3. PROCESS THE RESPONSE FROM THE SERVER */
if (data.result.is_valid) {
$("#gallery tbody").prepend(
"<tr><td><a href='" + data.result.url + "'>" + data.result.name + "</a></td></tr>"
)
}
}
});
});
Problems so far:
1. Upload doesn't work when clicking on the button. The box appears, I can select numerous files and "upload" to the page i.e. it says 2 files selected. But then clicking "Upload" gives the error:
{"is_valid": false}
This comes from the view (mulit_uploade.py
) but I'm a bit confused why
I'd like to use the
base.html
that was also used for the single upload feature. So when I add{% extends "myproject/base.html" %}
to themulti_upload.html
, a new problem appears that doesn't appear when I just use the single upload feature:
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name:
Learn more »
NoReverseMatch at /myproject/import/
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name
django python-3.x django-uploads
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19
add a comment |
I'd like to implement a multifile upload feature into a site. There is already an upload feature on the site, but for single files. I'm following loosely the Simple Is Better Than Complex: Django Multiple Files Upload Using Ajax.
My code:
views->mulit_upload.py
class BasicUploadView(View):
def get(self, request):
files_list = Multi_File.objects.all()
#files_list = ['testing_123']
print ("files_list: {0}".format(files_list))
return render(self.request, 'myproject/multi_upload.html', {'filesList': files_list})
def post(self, request):
form = FileForm(self.request.POST, self.request.FILES)
if form.is_valid():
multiFile = form.save()
data = {'is_valid': True, 'name': multiFile.file.name, 'url': multiFile.file.url}
else:
data = {'is_valid': False}
return JsonResponse(data)
models.py
class Multi_File(models.Model):
title = models.CharField(max_length=255, blank=True)
file = models.FileField(upload_to='files/')
uploaded_at = models.DateTimeField(auto_now_add=True)
forms.py
class FileForm(forms.ModelForm):
class Meta:
model = Multi_File
fields = ('file', )
multi_upload.html
{% load static %}
{% block javascript %}
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/vendor/jquery.ui.widget.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.iframe-transport.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.fileupload.js' %}"></script>
<script src="{% static 'myproject/upload-files.js' %}"></script>
{% endblock %}
{# 1. BUTTON TO TRIGGER THE ACTION #}
<div class="col-md-4">
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="myfile" multiple>
<button type="submit">Upload<span class="glyphicon glyphicon-cloud-upload"></span> </button>
</form>
</div>
{# 2. FILE INPUT TO BE USED BY THE PLUG-IN #}
<input id="fileupload" type="file" name="myfile" multiple
style="display: none;"
data-url="{% url 'myproject:multi_import' %}"
data-form-data='{"csrfmiddlewaretoken": "{{ csrf_token }}"}'>
{# 3. TABLE TO DISPLAY THE UPLOADED PHOTOS #}
<table id="gallery" class="table table-bordered">
<thead>
<tr>
<th>Uploaded Files:</th>
</tr>
</thead>
<tbody>
{% for aFile in filesList %}
<tr>
<td><a href="{{ aFile.file.url }}">{{ aFile.file.name }}</a></td>
</tr>
{% endfor %}
</tbody>
</table>
upload_files.js
$(function () {
/* 1. OPEN THE FILE EXPLORER WINDOW */
$(".js-upload-files").click(function () {
$("#fileupload").click();
});
/* 2. INITIALIZE THE FILE UPLOAD COMPONENT */
$("#fileupload").fileupload({
dataType: 'json',
done: function (e, data) { /* 3. PROCESS THE RESPONSE FROM THE SERVER */
if (data.result.is_valid) {
$("#gallery tbody").prepend(
"<tr><td><a href='" + data.result.url + "'>" + data.result.name + "</a></td></tr>"
)
}
}
});
});
Problems so far:
1. Upload doesn't work when clicking on the button. The box appears, I can select numerous files and "upload" to the page i.e. it says 2 files selected. But then clicking "Upload" gives the error:
{"is_valid": false}
This comes from the view (mulit_uploade.py
) but I'm a bit confused why
I'd like to use the
base.html
that was also used for the single upload feature. So when I add{% extends "myproject/base.html" %}
to themulti_upload.html
, a new problem appears that doesn't appear when I just use the single upload feature:
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name:
Learn more »
NoReverseMatch at /myproject/import/
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name
django python-3.x django-uploads
I'd like to implement a multifile upload feature into a site. There is already an upload feature on the site, but for single files. I'm following loosely the Simple Is Better Than Complex: Django Multiple Files Upload Using Ajax.
My code:
views->mulit_upload.py
class BasicUploadView(View):
def get(self, request):
files_list = Multi_File.objects.all()
#files_list = ['testing_123']
print ("files_list: {0}".format(files_list))
return render(self.request, 'myproject/multi_upload.html', {'filesList': files_list})
def post(self, request):
form = FileForm(self.request.POST, self.request.FILES)
if form.is_valid():
multiFile = form.save()
data = {'is_valid': True, 'name': multiFile.file.name, 'url': multiFile.file.url}
else:
data = {'is_valid': False}
return JsonResponse(data)
models.py
class Multi_File(models.Model):
title = models.CharField(max_length=255, blank=True)
file = models.FileField(upload_to='files/')
uploaded_at = models.DateTimeField(auto_now_add=True)
forms.py
class FileForm(forms.ModelForm):
class Meta:
model = Multi_File
fields = ('file', )
multi_upload.html
{% load static %}
{% block javascript %}
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/vendor/jquery.ui.widget.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.iframe-transport.js' %}"></script>
<script src="{% static 'myproject/js/jQuery-File-Upload-9.14.1/js/jquery.fileupload.js' %}"></script>
<script src="{% static 'myproject/upload-files.js' %}"></script>
{% endblock %}
{# 1. BUTTON TO TRIGGER THE ACTION #}
<div class="col-md-4">
<form method="post" enctype="multipart/form-data">
{% csrf_token %}
<input type="file" name="myfile" multiple>
<button type="submit">Upload<span class="glyphicon glyphicon-cloud-upload"></span> </button>
</form>
</div>
{# 2. FILE INPUT TO BE USED BY THE PLUG-IN #}
<input id="fileupload" type="file" name="myfile" multiple
style="display: none;"
data-url="{% url 'myproject:multi_import' %}"
data-form-data='{"csrfmiddlewaretoken": "{{ csrf_token }}"}'>
{# 3. TABLE TO DISPLAY THE UPLOADED PHOTOS #}
<table id="gallery" class="table table-bordered">
<thead>
<tr>
<th>Uploaded Files:</th>
</tr>
</thead>
<tbody>
{% for aFile in filesList %}
<tr>
<td><a href="{{ aFile.file.url }}">{{ aFile.file.name }}</a></td>
</tr>
{% endfor %}
</tbody>
</table>
upload_files.js
$(function () {
/* 1. OPEN THE FILE EXPLORER WINDOW */
$(".js-upload-files").click(function () {
$("#fileupload").click();
});
/* 2. INITIALIZE THE FILE UPLOAD COMPONENT */
$("#fileupload").fileupload({
dataType: 'json',
done: function (e, data) { /* 3. PROCESS THE RESPONSE FROM THE SERVER */
if (data.result.is_valid) {
$("#gallery tbody").prepend(
"<tr><td><a href='" + data.result.url + "'>" + data.result.name + "</a></td></tr>"
)
}
}
});
});
Problems so far:
1. Upload doesn't work when clicking on the button. The box appears, I can select numerous files and "upload" to the page i.e. it says 2 files selected. But then clicking "Upload" gives the error:
{"is_valid": false}
This comes from the view (mulit_uploade.py
) but I'm a bit confused why
I'd like to use the
base.html
that was also used for the single upload feature. So when I add{% extends "myproject/base.html" %}
to themulti_upload.html
, a new problem appears that doesn't appear when I just use the single upload feature:
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name:
Learn more »
NoReverseMatch at /myproject/import/
Reverse for 'myproject_about' not found. 'myproject_about' is not a valid view function or pattern name
django python-3.x django-uploads
django python-3.x django-uploads
asked Nov 16 '18 at 15:16
pymatpymat
192722
192722
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19
add a comment |
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19
add a comment |
1 Answer
1
active
oldest
votes
To start off you will need a formset(https://docs.djangoproject.com/en/2.1/topics/forms/formsets/), to be able to upload multiple files at once. So the code will roughly look like this
{% for form in formset %}
<input type="file" name="{{form.name}}" id="{{form.id_for_label}}" />
{{form.file.errors}}
{% endfor %}
Now each file will have some unique id, as you will see when you use formset.
to populate data in the table, you need to listen for file change event and change the table.
<td data-name="fileUniqueId1"></td>
<td data-name="fileUniqueId2"></td>
<td data-name="fileUniqueId3"></td>
$("form input[type='file']").change(function(e){
var fileId = e.target.id;
$("table td[data-name='"+ fileId +"']").text(e.target.files[0].name);
});
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53340620%2fdjango-multifile-upload-doesnt-send-files-to-directory%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To start off you will need a formset(https://docs.djangoproject.com/en/2.1/topics/forms/formsets/), to be able to upload multiple files at once. So the code will roughly look like this
{% for form in formset %}
<input type="file" name="{{form.name}}" id="{{form.id_for_label}}" />
{{form.file.errors}}
{% endfor %}
Now each file will have some unique id, as you will see when you use formset.
to populate data in the table, you need to listen for file change event and change the table.
<td data-name="fileUniqueId1"></td>
<td data-name="fileUniqueId2"></td>
<td data-name="fileUniqueId3"></td>
$("form input[type='file']").change(function(e){
var fileId = e.target.id;
$("table td[data-name='"+ fileId +"']").text(e.target.files[0].name);
});
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
add a comment |
To start off you will need a formset(https://docs.djangoproject.com/en/2.1/topics/forms/formsets/), to be able to upload multiple files at once. So the code will roughly look like this
{% for form in formset %}
<input type="file" name="{{form.name}}" id="{{form.id_for_label}}" />
{{form.file.errors}}
{% endfor %}
Now each file will have some unique id, as you will see when you use formset.
to populate data in the table, you need to listen for file change event and change the table.
<td data-name="fileUniqueId1"></td>
<td data-name="fileUniqueId2"></td>
<td data-name="fileUniqueId3"></td>
$("form input[type='file']").change(function(e){
var fileId = e.target.id;
$("table td[data-name='"+ fileId +"']").text(e.target.files[0].name);
});
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
add a comment |
To start off you will need a formset(https://docs.djangoproject.com/en/2.1/topics/forms/formsets/), to be able to upload multiple files at once. So the code will roughly look like this
{% for form in formset %}
<input type="file" name="{{form.name}}" id="{{form.id_for_label}}" />
{{form.file.errors}}
{% endfor %}
Now each file will have some unique id, as you will see when you use formset.
to populate data in the table, you need to listen for file change event and change the table.
<td data-name="fileUniqueId1"></td>
<td data-name="fileUniqueId2"></td>
<td data-name="fileUniqueId3"></td>
$("form input[type='file']").change(function(e){
var fileId = e.target.id;
$("table td[data-name='"+ fileId +"']").text(e.target.files[0].name);
});
To start off you will need a formset(https://docs.djangoproject.com/en/2.1/topics/forms/formsets/), to be able to upload multiple files at once. So the code will roughly look like this
{% for form in formset %}
<input type="file" name="{{form.name}}" id="{{form.id_for_label}}" />
{{form.file.errors}}
{% endfor %}
Now each file will have some unique id, as you will see when you use formset.
to populate data in the table, you need to listen for file change event and change the table.
<td data-name="fileUniqueId1"></td>
<td data-name="fileUniqueId2"></td>
<td data-name="fileUniqueId3"></td>
$("form input[type='file']").change(function(e){
var fileId = e.target.id;
$("table td[data-name='"+ fileId +"']").text(e.target.files[0].name);
});
edited Nov 21 '18 at 3:42
answered Nov 19 '18 at 10:55
Kireeti KKireeti K
384213
384213
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
add a comment |
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
thank you, I'm not very familiar with javascript.
– pymat
Nov 19 '18 at 18:24
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Glad it helped.
– Kireeti K
Nov 20 '18 at 1:57
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
Not quite, the javascript loop is something that needs to be determined
– pymat
Nov 20 '18 at 12:18
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
I've changed the code to work for multiple files
– Kireeti K
Nov 21 '18 at 3:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53340620%2fdjango-multifile-upload-doesnt-send-files-to-directory%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wQsC pkd dTy8gYZ9BTUGcnpR Ji4nxLRrMAHmXr yFkTh2KqU KCRJOOgJWaNwlXI,S03BQSIAYL5L
Does it have to use ajax? I was thinking of providing a concise example how this would work without ajax (just to you can actually see how its done with formsets; the django way)
– robotHamster
Nov 21 '18 at 8:19