Converting C# fluent interface to code in VB.NET
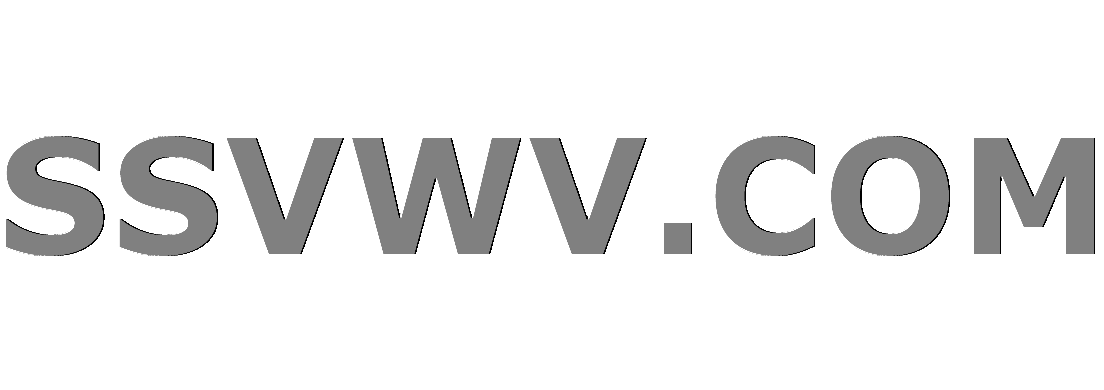
Multi tool use
I have the following C# code which I'm trying to convert to VB.NET.
I also want to replace the "Console.WriteLine"s with callbacks.
I think and hope I've managed to convert it to some extent:
nInteractorsAgent
.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, currentWindowHandle.ToString(), "MyFirstActivatable")
.WithActivatable()
.HasActivationFocus(id => Console.WriteLine("Id: {0} got activation focus.", id))
.SetTentativeFocusEnabled(true)
.HasTentativeFocus(id => Console.WriteLine("Id: {0} got tentative activation focus.", id))
.WhenFocusChanged((id, hasTentativeFocus, hasActivationFocus) => { })
.LostFocus(id => Console.WriteLine("Id: {0} lost focus.", id))
.WhenActivated(id => Console.WriteLine("Id: {0} activated", id));
My approach in VB.NET is this:
Dim v1 As VirtualInteractor = nInteractorsAgent.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, "0", "MyFirstActivatable").WithActivatable().HasActivationFocus(Sub() HasActivationFocus(v1.Id)).SetTentativeFocusEnabled(True).HasTentativeFocus(Sub() HasTentativeFocus(v1.Id)).WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False)).LostFocus(Sub() LostFocus(v1.Id)).WhenActivated(Sub() WhenActivated(v1.Id))
Public Shared Sub HasActivationFocus(ByVal id As String)
End Sub
Public Shared Sub HasTentativeFocus(ByVal id As String)
End Sub
Public Shared Sub WhenFocusChanged(ByVal id As String, ByVal hasTentativeFocus As Boolean, ByVal hasActivationFocus As Boolean)
End Sub
Public Shared Sub WhenFocusChanged()
End Sub
Public Shared Sub LostFocus(ByVal id As String)
End Sub
Public Shared Sub WhenActivated(ByVal id As String)
End Sub
However, the compiler tells me that something is wrong about
.WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False))
and
.WhenActivated(Sub() WhenActivated(v1.Id))
The compiler tells me that there's no version of WhenFocusChanged.
The declarations are these:
<Extension>
Public Shared Function WhenFocusChanged(behaviors As IEnumerable(Of ActivatableBehavior), action As ActivationFocusChangedCallback) As IEnumerable(Of ActivatableBehavior)
<Extension>
Public Shared Function WhenFocusChanged(behavior As ActivatableBehavior, action As ActivationFocusChangedCallback) As ActivatableBehavior
Can anybody show me what I'm doing wrong here and how to do it correctly?
I don't know which information apart from the given is important for readers in this case.
Thank you.
c# vb.net fluent
add a comment |
I have the following C# code which I'm trying to convert to VB.NET.
I also want to replace the "Console.WriteLine"s with callbacks.
I think and hope I've managed to convert it to some extent:
nInteractorsAgent
.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, currentWindowHandle.ToString(), "MyFirstActivatable")
.WithActivatable()
.HasActivationFocus(id => Console.WriteLine("Id: {0} got activation focus.", id))
.SetTentativeFocusEnabled(true)
.HasTentativeFocus(id => Console.WriteLine("Id: {0} got tentative activation focus.", id))
.WhenFocusChanged((id, hasTentativeFocus, hasActivationFocus) => { })
.LostFocus(id => Console.WriteLine("Id: {0} lost focus.", id))
.WhenActivated(id => Console.WriteLine("Id: {0} activated", id));
My approach in VB.NET is this:
Dim v1 As VirtualInteractor = nInteractorsAgent.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, "0", "MyFirstActivatable").WithActivatable().HasActivationFocus(Sub() HasActivationFocus(v1.Id)).SetTentativeFocusEnabled(True).HasTentativeFocus(Sub() HasTentativeFocus(v1.Id)).WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False)).LostFocus(Sub() LostFocus(v1.Id)).WhenActivated(Sub() WhenActivated(v1.Id))
Public Shared Sub HasActivationFocus(ByVal id As String)
End Sub
Public Shared Sub HasTentativeFocus(ByVal id As String)
End Sub
Public Shared Sub WhenFocusChanged(ByVal id As String, ByVal hasTentativeFocus As Boolean, ByVal hasActivationFocus As Boolean)
End Sub
Public Shared Sub WhenFocusChanged()
End Sub
Public Shared Sub LostFocus(ByVal id As String)
End Sub
Public Shared Sub WhenActivated(ByVal id As String)
End Sub
However, the compiler tells me that something is wrong about
.WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False))
and
.WhenActivated(Sub() WhenActivated(v1.Id))
The compiler tells me that there's no version of WhenFocusChanged.
The declarations are these:
<Extension>
Public Shared Function WhenFocusChanged(behaviors As IEnumerable(Of ActivatableBehavior), action As ActivationFocusChangedCallback) As IEnumerable(Of ActivatableBehavior)
<Extension>
Public Shared Function WhenFocusChanged(behavior As ActivatableBehavior, action As ActivationFocusChangedCallback) As ActivatableBehavior
Can anybody show me what I'm doing wrong here and how to do it correctly?
I don't know which information apart from the given is important for readers in this case.
Thank you.
c# vb.net fluent
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
1
You can't useSub
for fluent interface methods, they need to beFunctions
that return a reference to an object that can be used for the next method (typically, they returnMe
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has theWith
keyword that allows similar behavior, but with a mix ofSub
,Function
& properties.
– Flydog57
Nov 14 '18 at 17:40
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43
add a comment |
I have the following C# code which I'm trying to convert to VB.NET.
I also want to replace the "Console.WriteLine"s with callbacks.
I think and hope I've managed to convert it to some extent:
nInteractorsAgent
.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, currentWindowHandle.ToString(), "MyFirstActivatable")
.WithActivatable()
.HasActivationFocus(id => Console.WriteLine("Id: {0} got activation focus.", id))
.SetTentativeFocusEnabled(true)
.HasTentativeFocus(id => Console.WriteLine("Id: {0} got tentative activation focus.", id))
.WhenFocusChanged((id, hasTentativeFocus, hasActivationFocus) => { })
.LostFocus(id => Console.WriteLine("Id: {0} lost focus.", id))
.WhenActivated(id => Console.WriteLine("Id: {0} activated", id));
My approach in VB.NET is this:
Dim v1 As VirtualInteractor = nInteractorsAgent.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, "0", "MyFirstActivatable").WithActivatable().HasActivationFocus(Sub() HasActivationFocus(v1.Id)).SetTentativeFocusEnabled(True).HasTentativeFocus(Sub() HasTentativeFocus(v1.Id)).WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False)).LostFocus(Sub() LostFocus(v1.Id)).WhenActivated(Sub() WhenActivated(v1.Id))
Public Shared Sub HasActivationFocus(ByVal id As String)
End Sub
Public Shared Sub HasTentativeFocus(ByVal id As String)
End Sub
Public Shared Sub WhenFocusChanged(ByVal id As String, ByVal hasTentativeFocus As Boolean, ByVal hasActivationFocus As Boolean)
End Sub
Public Shared Sub WhenFocusChanged()
End Sub
Public Shared Sub LostFocus(ByVal id As String)
End Sub
Public Shared Sub WhenActivated(ByVal id As String)
End Sub
However, the compiler tells me that something is wrong about
.WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False))
and
.WhenActivated(Sub() WhenActivated(v1.Id))
The compiler tells me that there's no version of WhenFocusChanged.
The declarations are these:
<Extension>
Public Shared Function WhenFocusChanged(behaviors As IEnumerable(Of ActivatableBehavior), action As ActivationFocusChangedCallback) As IEnumerable(Of ActivatableBehavior)
<Extension>
Public Shared Function WhenFocusChanged(behavior As ActivatableBehavior, action As ActivationFocusChangedCallback) As ActivatableBehavior
Can anybody show me what I'm doing wrong here and how to do it correctly?
I don't know which information apart from the given is important for readers in this case.
Thank you.
c# vb.net fluent
I have the following C# code which I'm trying to convert to VB.NET.
I also want to replace the "Console.WriteLine"s with callbacks.
I think and hope I've managed to convert it to some extent:
nInteractorsAgent
.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, currentWindowHandle.ToString(), "MyFirstActivatable")
.WithActivatable()
.HasActivationFocus(id => Console.WriteLine("Id: {0} got activation focus.", id))
.SetTentativeFocusEnabled(true)
.HasTentativeFocus(id => Console.WriteLine("Id: {0} got tentative activation focus.", id))
.WhenFocusChanged((id, hasTentativeFocus, hasActivationFocus) => { })
.LostFocus(id => Console.WriteLine("Id: {0} lost focus.", id))
.WhenActivated(id => Console.WriteLine("Id: {0} activated", id));
My approach in VB.NET is this:
Dim v1 As VirtualInteractor = nInteractorsAgent.AddInteractorFor(currentWindowBounds, Literals.RootId, 0, "0", "MyFirstActivatable").WithActivatable().HasActivationFocus(Sub() HasActivationFocus(v1.Id)).SetTentativeFocusEnabled(True).HasTentativeFocus(Sub() HasTentativeFocus(v1.Id)).WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False)).LostFocus(Sub() LostFocus(v1.Id)).WhenActivated(Sub() WhenActivated(v1.Id))
Public Shared Sub HasActivationFocus(ByVal id As String)
End Sub
Public Shared Sub HasTentativeFocus(ByVal id As String)
End Sub
Public Shared Sub WhenFocusChanged(ByVal id As String, ByVal hasTentativeFocus As Boolean, ByVal hasActivationFocus As Boolean)
End Sub
Public Shared Sub WhenFocusChanged()
End Sub
Public Shared Sub LostFocus(ByVal id As String)
End Sub
Public Shared Sub WhenActivated(ByVal id As String)
End Sub
However, the compiler tells me that something is wrong about
.WhenFocusChanged(Sub() WhenFocusChanged(v1.Id, False, False))
and
.WhenActivated(Sub() WhenActivated(v1.Id))
The compiler tells me that there's no version of WhenFocusChanged.
The declarations are these:
<Extension>
Public Shared Function WhenFocusChanged(behaviors As IEnumerable(Of ActivatableBehavior), action As ActivationFocusChangedCallback) As IEnumerable(Of ActivatableBehavior)
<Extension>
Public Shared Function WhenFocusChanged(behavior As ActivatableBehavior, action As ActivationFocusChangedCallback) As ActivatableBehavior
Can anybody show me what I'm doing wrong here and how to do it correctly?
I don't know which information apart from the given is important for readers in this case.
Thank you.
c# vb.net fluent
c# vb.net fluent
asked Nov 14 '18 at 17:14
tmightytmighty
2,1531057126
2,1531057126
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
1
You can't useSub
for fluent interface methods, they need to beFunctions
that return a reference to an object that can be used for the next method (typically, they returnMe
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has theWith
keyword that allows similar behavior, but with a mix ofSub
,Function
& properties.
– Flydog57
Nov 14 '18 at 17:40
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43
add a comment |
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
1
You can't useSub
for fluent interface methods, they need to beFunctions
that return a reference to an object that can be used for the next method (typically, they returnMe
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has theWith
keyword that allows similar behavior, but with a mix ofSub
,Function
& properties.
– Flydog57
Nov 14 '18 at 17:40
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
1
1
You can't use
Sub
for fluent interface methods, they need to be Functions
that return a reference to an object that can be used for the next method (typically, they return Me
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has the With
keyword that allows similar behavior, but with a mix of Sub
, Function
& properties.– Flydog57
Nov 14 '18 at 17:40
You can't use
Sub
for fluent interface methods, they need to be Functions
that return a reference to an object that can be used for the next method (typically, they return Me
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has the With
keyword that allows similar behavior, but with a mix of Sub
, Function
& properties.– Flydog57
Nov 14 '18 at 17:40
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43
add a comment |
1 Answer
1
active
oldest
votes
That won't work like the original code. You need a mix of lambda and delegate. Here's a quick example of something similar in vb.net.
Module Module1
Sub Main()
Dim o As New Test
o.DoSomething(Sub(paramName) Console.WriteLine("The id is {0}", paramName))
End Sub
End Module
Class Test
Public Property ID As Integer = 10
Delegate Sub SomeDelegate(ByVal id As Integer)
Public Function DoSomething(ByVal f As SomeDelegate) As Test
f(Me.ID)
Return Me
End Function
End Class
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305529%2fconverting-c-sharp-fluent-interface-to-code-in-vb-net%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
That won't work like the original code. You need a mix of lambda and delegate. Here's a quick example of something similar in vb.net.
Module Module1
Sub Main()
Dim o As New Test
o.DoSomething(Sub(paramName) Console.WriteLine("The id is {0}", paramName))
End Sub
End Module
Class Test
Public Property ID As Integer = 10
Delegate Sub SomeDelegate(ByVal id As Integer)
Public Function DoSomething(ByVal f As SomeDelegate) As Test
f(Me.ID)
Return Me
End Function
End Class
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
add a comment |
That won't work like the original code. You need a mix of lambda and delegate. Here's a quick example of something similar in vb.net.
Module Module1
Sub Main()
Dim o As New Test
o.DoSomething(Sub(paramName) Console.WriteLine("The id is {0}", paramName))
End Sub
End Module
Class Test
Public Property ID As Integer = 10
Delegate Sub SomeDelegate(ByVal id As Integer)
Public Function DoSomething(ByVal f As SomeDelegate) As Test
f(Me.ID)
Return Me
End Function
End Class
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
add a comment |
That won't work like the original code. You need a mix of lambda and delegate. Here's a quick example of something similar in vb.net.
Module Module1
Sub Main()
Dim o As New Test
o.DoSomething(Sub(paramName) Console.WriteLine("The id is {0}", paramName))
End Sub
End Module
Class Test
Public Property ID As Integer = 10
Delegate Sub SomeDelegate(ByVal id As Integer)
Public Function DoSomething(ByVal f As SomeDelegate) As Test
f(Me.ID)
Return Me
End Function
End Class
That won't work like the original code. You need a mix of lambda and delegate. Here's a quick example of something similar in vb.net.
Module Module1
Sub Main()
Dim o As New Test
o.DoSomething(Sub(paramName) Console.WriteLine("The id is {0}", paramName))
End Sub
End Module
Class Test
Public Property ID As Integer = 10
Delegate Sub SomeDelegate(ByVal id As Integer)
Public Function DoSomething(ByVal f As SomeDelegate) As Test
f(Me.ID)
Return Me
End Function
End Class
answered Nov 14 '18 at 17:34
the_lotusthe_lotus
10.1k12246
10.1k12246
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
add a comment |
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
Thank you very much for the explanation. Is there any chance to get the entire conversion?
– tmighty
Nov 14 '18 at 21:48
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305529%2fconverting-c-sharp-fluent-interface-to-code-in-vb-net%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LoG5nGki,I9oxhXW Pi7,2qe5qowwkL1dzd62XwxXF3kqYwK,X vDytEVN fRWw 5cQOw
make sure the "using" statements in c# have the same "import" statement in VB.net. The Solution Explore References should be the same in vb.net and c#.
– jdweng
Nov 14 '18 at 17:22
1
You can't use
Sub
for fluent interface methods, they need to beFunctions
that return a reference to an object that can be used for the next method (typically, they returnMe
(/this
)).. The idea is you call a function which returns a reference to the object you are working on. Then you can just call the next function on that same reference, chaining your way through the calls, always referring to the same object. Now, VB has theWith
keyword that allows similar behavior, but with a mix ofSub
,Function
& properties.– Flydog57
Nov 14 '18 at 17:40
Are you recreating the extension methods or trying to use the existing C# code / extensions in VB.NET?
– Michael Puckett II
Nov 14 '18 at 17:43