Unable to resolve service for type 'GraphQL.Http.IDocumentWriter'
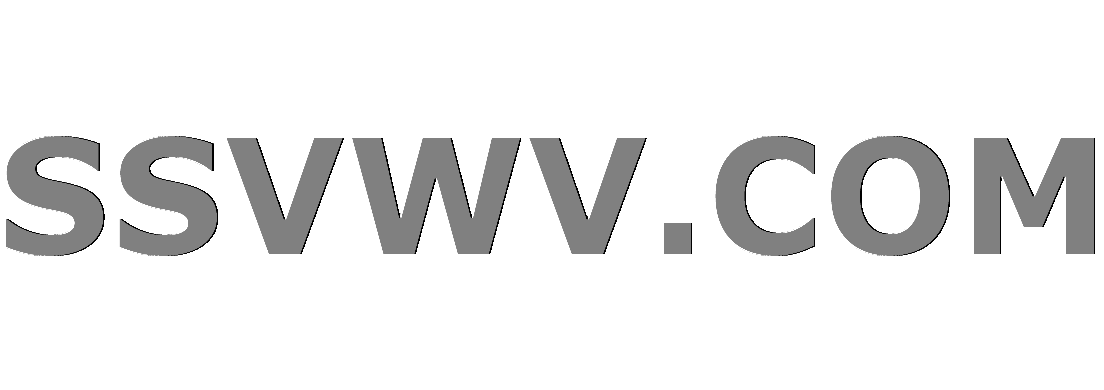
Multi tool use
up vote
0
down vote
favorite
I used following only controller in my project out of entire following project.
https://github.com/graphql-dotnet/examples/blob/master/src/AspNetWebApi/Example/Controllers/GraphQLController.cs
Here Controller file is as below.
using System.Collections.Generic;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
using GraphQL.Http;
using GraphQL.Instrumentation;
using GraphQL.Types;
using GraphQL.Validation.Complexity;
namespace GraphQL.GraphiQL.Controllers
{
public class GraphQLController : ApiController
{
private readonly ISchema _schema;
private readonly IDocumentExecuter _executer;
private readonly IDocumentWriter _writer;
private readonly IDictionary<string, string> _namedQueries;
public GraphQLController(
IDocumentExecuter executer,
IDocumentWriter writer,
ISchema schema)
{
_executer = executer;
_writer = writer;
_schema = schema;
_namedQueries = new Dictionary<string, string>
{
["a-query"] = @"query foo { hero { name } }"
};
}
// This will display an example error
[HttpGet]
public Task<HttpResponseMessage> GetAsync(HttpRequestMessage request)
{
return PostAsync(request, new GraphQLQuery { Query = "query foo { hero }", Variables = null });
}
[HttpPost]
public async Task<HttpResponseMessage> PostAsync(HttpRequestMessage request, GraphQLQuery query)
{
var inputs = query.Variables.ToInputs();
var queryToExecute = query.Query;
if (!string.IsNullOrWhiteSpace(query.NamedQuery))
{
queryToExecute = _namedQueries[query.NamedQuery];
}
var result = await _executer.ExecuteAsync(_ =>
{
_.Schema = _schema;
_.Query = queryToExecute;
_.OperationName = query.OperationName;
_.Inputs = inputs;
_.ComplexityConfiguration = new ComplexityConfiguration { MaxDepth = 15 };
_.FieldMiddleware.Use<InstrumentFieldsMiddleware>();
}).ConfigureAwait(false);
var httpResult = result.Errors?.Count > 0
? HttpStatusCode.BadRequest
: HttpStatusCode.OK;
var json = _writer.Write(result);
var response = request.CreateResponse(httpResult);
response.Content = new StringContent(json, Encoding.UTF8, "application/json");
return response;
}
}
public class GraphQLQuery
{
public string OperationName { get; set; }
public string NamedQuery { get; set; }
public string Query { get; set; }
public Newtonsoft.Json.Linq.JObject Variables { get; set; }
}
}
When I try to call this controller, it gives me following error.
InvalidOperationException: Unable to resolve service for type 'GraphQL.Http.IDocumentWriter' while attempting to activate 'MyWebAppNamespace.Controllers.GraphQLController'.
Do I need any startup configuration or I can use this standalone controller? What am I missing?
asp.net-core-mvc graphql asp.net-core-webapi
add a comment |
up vote
0
down vote
favorite
I used following only controller in my project out of entire following project.
https://github.com/graphql-dotnet/examples/blob/master/src/AspNetWebApi/Example/Controllers/GraphQLController.cs
Here Controller file is as below.
using System.Collections.Generic;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
using GraphQL.Http;
using GraphQL.Instrumentation;
using GraphQL.Types;
using GraphQL.Validation.Complexity;
namespace GraphQL.GraphiQL.Controllers
{
public class GraphQLController : ApiController
{
private readonly ISchema _schema;
private readonly IDocumentExecuter _executer;
private readonly IDocumentWriter _writer;
private readonly IDictionary<string, string> _namedQueries;
public GraphQLController(
IDocumentExecuter executer,
IDocumentWriter writer,
ISchema schema)
{
_executer = executer;
_writer = writer;
_schema = schema;
_namedQueries = new Dictionary<string, string>
{
["a-query"] = @"query foo { hero { name } }"
};
}
// This will display an example error
[HttpGet]
public Task<HttpResponseMessage> GetAsync(HttpRequestMessage request)
{
return PostAsync(request, new GraphQLQuery { Query = "query foo { hero }", Variables = null });
}
[HttpPost]
public async Task<HttpResponseMessage> PostAsync(HttpRequestMessage request, GraphQLQuery query)
{
var inputs = query.Variables.ToInputs();
var queryToExecute = query.Query;
if (!string.IsNullOrWhiteSpace(query.NamedQuery))
{
queryToExecute = _namedQueries[query.NamedQuery];
}
var result = await _executer.ExecuteAsync(_ =>
{
_.Schema = _schema;
_.Query = queryToExecute;
_.OperationName = query.OperationName;
_.Inputs = inputs;
_.ComplexityConfiguration = new ComplexityConfiguration { MaxDepth = 15 };
_.FieldMiddleware.Use<InstrumentFieldsMiddleware>();
}).ConfigureAwait(false);
var httpResult = result.Errors?.Count > 0
? HttpStatusCode.BadRequest
: HttpStatusCode.OK;
var json = _writer.Write(result);
var response = request.CreateResponse(httpResult);
response.Content = new StringContent(json, Encoding.UTF8, "application/json");
return response;
}
}
public class GraphQLQuery
{
public string OperationName { get; set; }
public string NamedQuery { get; set; }
public string Query { get; set; }
public Newtonsoft.Json.Linq.JObject Variables { get; set; }
}
}
When I try to call this controller, it gives me following error.
InvalidOperationException: Unable to resolve service for type 'GraphQL.Http.IDocumentWriter' while attempting to activate 'MyWebAppNamespace.Controllers.GraphQLController'.
Do I need any startup configuration or I can use this standalone controller? What am I missing?
asp.net-core-mvc graphql asp.net-core-webapi
1
Note thatApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core
– Nkosi
Nov 12 at 10:01
1
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I used following only controller in my project out of entire following project.
https://github.com/graphql-dotnet/examples/blob/master/src/AspNetWebApi/Example/Controllers/GraphQLController.cs
Here Controller file is as below.
using System.Collections.Generic;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
using GraphQL.Http;
using GraphQL.Instrumentation;
using GraphQL.Types;
using GraphQL.Validation.Complexity;
namespace GraphQL.GraphiQL.Controllers
{
public class GraphQLController : ApiController
{
private readonly ISchema _schema;
private readonly IDocumentExecuter _executer;
private readonly IDocumentWriter _writer;
private readonly IDictionary<string, string> _namedQueries;
public GraphQLController(
IDocumentExecuter executer,
IDocumentWriter writer,
ISchema schema)
{
_executer = executer;
_writer = writer;
_schema = schema;
_namedQueries = new Dictionary<string, string>
{
["a-query"] = @"query foo { hero { name } }"
};
}
// This will display an example error
[HttpGet]
public Task<HttpResponseMessage> GetAsync(HttpRequestMessage request)
{
return PostAsync(request, new GraphQLQuery { Query = "query foo { hero }", Variables = null });
}
[HttpPost]
public async Task<HttpResponseMessage> PostAsync(HttpRequestMessage request, GraphQLQuery query)
{
var inputs = query.Variables.ToInputs();
var queryToExecute = query.Query;
if (!string.IsNullOrWhiteSpace(query.NamedQuery))
{
queryToExecute = _namedQueries[query.NamedQuery];
}
var result = await _executer.ExecuteAsync(_ =>
{
_.Schema = _schema;
_.Query = queryToExecute;
_.OperationName = query.OperationName;
_.Inputs = inputs;
_.ComplexityConfiguration = new ComplexityConfiguration { MaxDepth = 15 };
_.FieldMiddleware.Use<InstrumentFieldsMiddleware>();
}).ConfigureAwait(false);
var httpResult = result.Errors?.Count > 0
? HttpStatusCode.BadRequest
: HttpStatusCode.OK;
var json = _writer.Write(result);
var response = request.CreateResponse(httpResult);
response.Content = new StringContent(json, Encoding.UTF8, "application/json");
return response;
}
}
public class GraphQLQuery
{
public string OperationName { get; set; }
public string NamedQuery { get; set; }
public string Query { get; set; }
public Newtonsoft.Json.Linq.JObject Variables { get; set; }
}
}
When I try to call this controller, it gives me following error.
InvalidOperationException: Unable to resolve service for type 'GraphQL.Http.IDocumentWriter' while attempting to activate 'MyWebAppNamespace.Controllers.GraphQLController'.
Do I need any startup configuration or I can use this standalone controller? What am I missing?
asp.net-core-mvc graphql asp.net-core-webapi
I used following only controller in my project out of entire following project.
https://github.com/graphql-dotnet/examples/blob/master/src/AspNetWebApi/Example/Controllers/GraphQLController.cs
Here Controller file is as below.
using System.Collections.Generic;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using System.Web.Http;
using GraphQL.Http;
using GraphQL.Instrumentation;
using GraphQL.Types;
using GraphQL.Validation.Complexity;
namespace GraphQL.GraphiQL.Controllers
{
public class GraphQLController : ApiController
{
private readonly ISchema _schema;
private readonly IDocumentExecuter _executer;
private readonly IDocumentWriter _writer;
private readonly IDictionary<string, string> _namedQueries;
public GraphQLController(
IDocumentExecuter executer,
IDocumentWriter writer,
ISchema schema)
{
_executer = executer;
_writer = writer;
_schema = schema;
_namedQueries = new Dictionary<string, string>
{
["a-query"] = @"query foo { hero { name } }"
};
}
// This will display an example error
[HttpGet]
public Task<HttpResponseMessage> GetAsync(HttpRequestMessage request)
{
return PostAsync(request, new GraphQLQuery { Query = "query foo { hero }", Variables = null });
}
[HttpPost]
public async Task<HttpResponseMessage> PostAsync(HttpRequestMessage request, GraphQLQuery query)
{
var inputs = query.Variables.ToInputs();
var queryToExecute = query.Query;
if (!string.IsNullOrWhiteSpace(query.NamedQuery))
{
queryToExecute = _namedQueries[query.NamedQuery];
}
var result = await _executer.ExecuteAsync(_ =>
{
_.Schema = _schema;
_.Query = queryToExecute;
_.OperationName = query.OperationName;
_.Inputs = inputs;
_.ComplexityConfiguration = new ComplexityConfiguration { MaxDepth = 15 };
_.FieldMiddleware.Use<InstrumentFieldsMiddleware>();
}).ConfigureAwait(false);
var httpResult = result.Errors?.Count > 0
? HttpStatusCode.BadRequest
: HttpStatusCode.OK;
var json = _writer.Write(result);
var response = request.CreateResponse(httpResult);
response.Content = new StringContent(json, Encoding.UTF8, "application/json");
return response;
}
}
public class GraphQLQuery
{
public string OperationName { get; set; }
public string NamedQuery { get; set; }
public string Query { get; set; }
public Newtonsoft.Json.Linq.JObject Variables { get; set; }
}
}
When I try to call this controller, it gives me following error.
InvalidOperationException: Unable to resolve service for type 'GraphQL.Http.IDocumentWriter' while attempting to activate 'MyWebAppNamespace.Controllers.GraphQLController'.
Do I need any startup configuration or I can use this standalone controller? What am I missing?
asp.net-core-mvc graphql asp.net-core-webapi
asp.net-core-mvc graphql asp.net-core-webapi
asked Nov 11 at 12:31


Anonymous Creator
11011
11011
1
Note thatApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core
– Nkosi
Nov 12 at 10:01
1
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05
add a comment |
1
Note thatApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core
– Nkosi
Nov 12 at 10:01
1
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05
1
1
Note that
ApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core– Nkosi
Nov 12 at 10:01
Note that
ApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core– Nkosi
Nov 12 at 10:01
1
1
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
Do I need any startup configuration or I can use this standalone controller? What am I missing?
As the error describes, it happens because the DI container cannot resolve service for IDocumentWriter
.
It seems that you're missing some service registration. Did you forget to add the IDocumentWrite
service ?
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDependencyResolver>(s => new FuncDependencyResolver(s.GetRequiredService));
services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
services.AddSingleton<IDocumentWriter, DocumentWriter>();
// ...
services.AddSingleton<ISchema, StarWarsSchema>();
// ...
}
For more details, see startup here
or I can use this standalone controller?
No. You're not supposed to do that. That's because your controller depends on the IDocumentExecuter
, IDocumentWriter
, and ISchema
service. They're all resolved by Dependency Injection. You have to register all the services before resolve the controller.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Do I need any startup configuration or I can use this standalone controller? What am I missing?
As the error describes, it happens because the DI container cannot resolve service for IDocumentWriter
.
It seems that you're missing some service registration. Did you forget to add the IDocumentWrite
service ?
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDependencyResolver>(s => new FuncDependencyResolver(s.GetRequiredService));
services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
services.AddSingleton<IDocumentWriter, DocumentWriter>();
// ...
services.AddSingleton<ISchema, StarWarsSchema>();
// ...
}
For more details, see startup here
or I can use this standalone controller?
No. You're not supposed to do that. That's because your controller depends on the IDocumentExecuter
, IDocumentWriter
, and ISchema
service. They're all resolved by Dependency Injection. You have to register all the services before resolve the controller.
add a comment |
up vote
1
down vote
accepted
Do I need any startup configuration or I can use this standalone controller? What am I missing?
As the error describes, it happens because the DI container cannot resolve service for IDocumentWriter
.
It seems that you're missing some service registration. Did you forget to add the IDocumentWrite
service ?
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDependencyResolver>(s => new FuncDependencyResolver(s.GetRequiredService));
services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
services.AddSingleton<IDocumentWriter, DocumentWriter>();
// ...
services.AddSingleton<ISchema, StarWarsSchema>();
// ...
}
For more details, see startup here
or I can use this standalone controller?
No. You're not supposed to do that. That's because your controller depends on the IDocumentExecuter
, IDocumentWriter
, and ISchema
service. They're all resolved by Dependency Injection. You have to register all the services before resolve the controller.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Do I need any startup configuration or I can use this standalone controller? What am I missing?
As the error describes, it happens because the DI container cannot resolve service for IDocumentWriter
.
It seems that you're missing some service registration. Did you forget to add the IDocumentWrite
service ?
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDependencyResolver>(s => new FuncDependencyResolver(s.GetRequiredService));
services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
services.AddSingleton<IDocumentWriter, DocumentWriter>();
// ...
services.AddSingleton<ISchema, StarWarsSchema>();
// ...
}
For more details, see startup here
or I can use this standalone controller?
No. You're not supposed to do that. That's because your controller depends on the IDocumentExecuter
, IDocumentWriter
, and ISchema
service. They're all resolved by Dependency Injection. You have to register all the services before resolve the controller.
Do I need any startup configuration or I can use this standalone controller? What am I missing?
As the error describes, it happens because the DI container cannot resolve service for IDocumentWriter
.
It seems that you're missing some service registration. Did you forget to add the IDocumentWrite
service ?
public void ConfigureServices(IServiceCollection services)
{
services.AddSingleton<IDependencyResolver>(s => new FuncDependencyResolver(s.GetRequiredService));
services.AddSingleton<IDocumentExecuter, DocumentExecuter>();
services.AddSingleton<IDocumentWriter, DocumentWriter>();
// ...
services.AddSingleton<ISchema, StarWarsSchema>();
// ...
}
For more details, see startup here
or I can use this standalone controller?
No. You're not supposed to do that. That's because your controller depends on the IDocumentExecuter
, IDocumentWriter
, and ISchema
service. They're all resolved by Dependency Injection. You have to register all the services before resolve the controller.
answered Nov 12 at 9:26
itminus
2,6511320
2,6511320
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248792%2funable-to-resolve-service-for-type-graphql-http-idocumentwriter%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fbG3cIpqOe7St0g90XCpcmHyNgoZfe FxdcW 2cW7z EPQY1gVzTNrehCue
1
Note that
ApiController
class (not attribute) is from previous version of the framework yet the question is tagged as asp.net-core– Nkosi
Nov 12 at 10:01
1
I would suggest you recheck the startup flow
– Nkosi
Nov 12 at 10:05