C, How to malloc the correct amount of space for an array of a struct inside another struct?
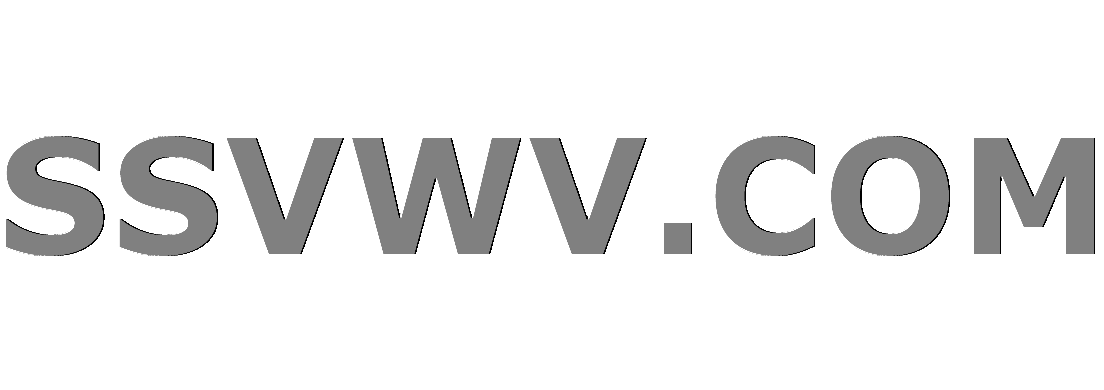
Multi tool use
up vote
0
down vote
favorite
I have two structs. I am trying the make an array of 'struct bird' inside another struct 'struct nest'.
I am having a hard time allocating the correct amount of space for the bird array when I am creating the nest struct.
Below is my code.
struct bird {
int value;
};
typedef struct bird bird;
struct nest {
int nb_birds;
bird * * birds; //bird * = points to the bird struct, * birds = Array with size unknown
};
typedef struct nest nest;
nest * create_nest(int nb_birds) {
nest * n = (nest *) malloc(sizeof(nest));
n->nb_birds = nb_birds;
//This is where I am stuck
***n->birds = (bird *) malloc(sizeof(bird) * nb_birds);***
int i;
for(i = 0; i < nb_birds; i++)
n->birds[i]=NULL;
return n;
}
c arrays struct malloc
add a comment |
up vote
0
down vote
favorite
I have two structs. I am trying the make an array of 'struct bird' inside another struct 'struct nest'.
I am having a hard time allocating the correct amount of space for the bird array when I am creating the nest struct.
Below is my code.
struct bird {
int value;
};
typedef struct bird bird;
struct nest {
int nb_birds;
bird * * birds; //bird * = points to the bird struct, * birds = Array with size unknown
};
typedef struct nest nest;
nest * create_nest(int nb_birds) {
nest * n = (nest *) malloc(sizeof(nest));
n->nb_birds = nb_birds;
//This is where I am stuck
***n->birds = (bird *) malloc(sizeof(bird) * nb_birds);***
int i;
for(i = 0; i < nb_birds; i++)
n->birds[i]=NULL;
return n;
}
c arrays struct malloc
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have two structs. I am trying the make an array of 'struct bird' inside another struct 'struct nest'.
I am having a hard time allocating the correct amount of space for the bird array when I am creating the nest struct.
Below is my code.
struct bird {
int value;
};
typedef struct bird bird;
struct nest {
int nb_birds;
bird * * birds; //bird * = points to the bird struct, * birds = Array with size unknown
};
typedef struct nest nest;
nest * create_nest(int nb_birds) {
nest * n = (nest *) malloc(sizeof(nest));
n->nb_birds = nb_birds;
//This is where I am stuck
***n->birds = (bird *) malloc(sizeof(bird) * nb_birds);***
int i;
for(i = 0; i < nb_birds; i++)
n->birds[i]=NULL;
return n;
}
c arrays struct malloc
I have two structs. I am trying the make an array of 'struct bird' inside another struct 'struct nest'.
I am having a hard time allocating the correct amount of space for the bird array when I am creating the nest struct.
Below is my code.
struct bird {
int value;
};
typedef struct bird bird;
struct nest {
int nb_birds;
bird * * birds; //bird * = points to the bird struct, * birds = Array with size unknown
};
typedef struct nest nest;
nest * create_nest(int nb_birds) {
nest * n = (nest *) malloc(sizeof(nest));
n->nb_birds = nb_birds;
//This is where I am stuck
***n->birds = (bird *) malloc(sizeof(bird) * nb_birds);***
int i;
for(i = 0; i < nb_birds; i++)
n->birds[i]=NULL;
return n;
}
c arrays struct malloc
c arrays struct malloc
edited Nov 11 at 12:33
asked Nov 11 at 12:24
seamus
6342921
6342921
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27
add a comment |
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
You want to allocate array of nb_birds
pointers to bird
structure, so size to allocation is nb_birds * sizeof(bird *)
.
Then you want to store pointer to this array, so cast should be to address of the first element — address of bird *
, i.e. bird **
.
Hence,
n->birds = (bird **) malloc(sizeof(bird *) * nb_birds);
p.s. If you want to allocate N
objects on which ptr
points to, you can write, or, at least, think about as
ptr = (typeof(ptr)) malloc(sizeof(*ptr) * N);
Update:
It should be noted that malloc
returns void *
pointer that compatible with any pointer type without explicit casting. So, quoted program line can be as short as
ptr = malloc(N * sizeof(*ptr));
Some programmers, despite them well informed about this void *
property, strongly prefer to use explicit cast in such cases. I'm not one of them, but I account such casts as stylistіc preference (like ()
for sizeof
operator). So I left the casting in code above because OP use it, and I thought it was his choice.
Neverthless it is needed (at least for answer completeness and for further readers) to note that such cast is unnecessary and excessive.
.
Thank you Paul Ogilvie and chux for patient notes in the comments.
1
..and don't cast the result ofmalloc
. It returnsvoid *
which is compatible with any pointer type.n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.
– Paul Ogilvie
Nov 11 at 12:51
Agree, casting ofvoid *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.
– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as inn->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You want to allocate array of nb_birds
pointers to bird
structure, so size to allocation is nb_birds * sizeof(bird *)
.
Then you want to store pointer to this array, so cast should be to address of the first element — address of bird *
, i.e. bird **
.
Hence,
n->birds = (bird **) malloc(sizeof(bird *) * nb_birds);
p.s. If you want to allocate N
objects on which ptr
points to, you can write, or, at least, think about as
ptr = (typeof(ptr)) malloc(sizeof(*ptr) * N);
Update:
It should be noted that malloc
returns void *
pointer that compatible with any pointer type without explicit casting. So, quoted program line can be as short as
ptr = malloc(N * sizeof(*ptr));
Some programmers, despite them well informed about this void *
property, strongly prefer to use explicit cast in such cases. I'm not one of them, but I account such casts as stylistіc preference (like ()
for sizeof
operator). So I left the casting in code above because OP use it, and I thought it was his choice.
Neverthless it is needed (at least for answer completeness and for further readers) to note that such cast is unnecessary and excessive.
.
Thank you Paul Ogilvie and chux for patient notes in the comments.
1
..and don't cast the result ofmalloc
. It returnsvoid *
which is compatible with any pointer type.n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.
– Paul Ogilvie
Nov 11 at 12:51
Agree, casting ofvoid *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.
– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as inn->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
add a comment |
up vote
1
down vote
accepted
You want to allocate array of nb_birds
pointers to bird
structure, so size to allocation is nb_birds * sizeof(bird *)
.
Then you want to store pointer to this array, so cast should be to address of the first element — address of bird *
, i.e. bird **
.
Hence,
n->birds = (bird **) malloc(sizeof(bird *) * nb_birds);
p.s. If you want to allocate N
objects on which ptr
points to, you can write, or, at least, think about as
ptr = (typeof(ptr)) malloc(sizeof(*ptr) * N);
Update:
It should be noted that malloc
returns void *
pointer that compatible with any pointer type without explicit casting. So, quoted program line can be as short as
ptr = malloc(N * sizeof(*ptr));
Some programmers, despite them well informed about this void *
property, strongly prefer to use explicit cast in such cases. I'm not one of them, but I account such casts as stylistіc preference (like ()
for sizeof
operator). So I left the casting in code above because OP use it, and I thought it was his choice.
Neverthless it is needed (at least for answer completeness and for further readers) to note that such cast is unnecessary and excessive.
.
Thank you Paul Ogilvie and chux for patient notes in the comments.
1
..and don't cast the result ofmalloc
. It returnsvoid *
which is compatible with any pointer type.n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.
– Paul Ogilvie
Nov 11 at 12:51
Agree, casting ofvoid *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.
– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as inn->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You want to allocate array of nb_birds
pointers to bird
structure, so size to allocation is nb_birds * sizeof(bird *)
.
Then you want to store pointer to this array, so cast should be to address of the first element — address of bird *
, i.e. bird **
.
Hence,
n->birds = (bird **) malloc(sizeof(bird *) * nb_birds);
p.s. If you want to allocate N
objects on which ptr
points to, you can write, or, at least, think about as
ptr = (typeof(ptr)) malloc(sizeof(*ptr) * N);
Update:
It should be noted that malloc
returns void *
pointer that compatible with any pointer type without explicit casting. So, quoted program line can be as short as
ptr = malloc(N * sizeof(*ptr));
Some programmers, despite them well informed about this void *
property, strongly prefer to use explicit cast in such cases. I'm not one of them, but I account such casts as stylistіc preference (like ()
for sizeof
operator). So I left the casting in code above because OP use it, and I thought it was his choice.
Neverthless it is needed (at least for answer completeness and for further readers) to note that such cast is unnecessary and excessive.
.
Thank you Paul Ogilvie and chux for patient notes in the comments.
You want to allocate array of nb_birds
pointers to bird
structure, so size to allocation is nb_birds * sizeof(bird *)
.
Then you want to store pointer to this array, so cast should be to address of the first element — address of bird *
, i.e. bird **
.
Hence,
n->birds = (bird **) malloc(sizeof(bird *) * nb_birds);
p.s. If you want to allocate N
objects on which ptr
points to, you can write, or, at least, think about as
ptr = (typeof(ptr)) malloc(sizeof(*ptr) * N);
Update:
It should be noted that malloc
returns void *
pointer that compatible with any pointer type without explicit casting. So, quoted program line can be as short as
ptr = malloc(N * sizeof(*ptr));
Some programmers, despite them well informed about this void *
property, strongly prefer to use explicit cast in such cases. I'm not one of them, but I account such casts as stylistіc preference (like ()
for sizeof
operator). So I left the casting in code above because OP use it, and I thought it was his choice.
Neverthless it is needed (at least for answer completeness and for further readers) to note that such cast is unnecessary and excessive.
.
Thank you Paul Ogilvie and chux for patient notes in the comments.
edited Nov 11 at 18:04
answered Nov 11 at 12:37
ReAl
581216
581216
1
..and don't cast the result ofmalloc
. It returnsvoid *
which is compatible with any pointer type.n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.
– Paul Ogilvie
Nov 11 at 12:51
Agree, casting ofvoid *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.
– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as inn->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
add a comment |
1
..and don't cast the result ofmalloc
. It returnsvoid *
which is compatible with any pointer type.n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.
– Paul Ogilvie
Nov 11 at 12:51
Agree, casting ofvoid *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.
– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as inn->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
1
1
..and don't cast the result of
malloc
. It returns void *
which is compatible with any pointer type. n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.– Paul Ogilvie
Nov 11 at 12:51
..and don't cast the result of
malloc
. It returns void *
which is compatible with any pointer type. n->birds = malloc(sizeof(bird *) * nb_birds);
is all the answer requires.– Paul Ogilvie
Nov 11 at 12:51
Agree, casting of
void *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.– ReAl
Nov 11 at 12:57
Agree, casting of
void *
returned by malloc is redundant (and I never use it). But some programmers prefer to explicit cast and I left this part of code as is. Anyway, your comment is very useful.– ReAl
Nov 11 at 12:57
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as in n->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
ptr = malloc(sizeof *ptr * N);
is even easier to code right, review and maintain. No "type" needed as in n->birds = malloc(sizeof *(n->birds) * nb_birds);
– chux
Nov 11 at 16:41
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
@chux Your comment is the same as one of Paul Ogilvie above. But I added note because it is hot topic :-). Thanks for help in war with my lazyness.
– ReAl
Nov 11 at 18:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53248732%2fc-how-to-malloc-the-correct-amount-of-space-for-an-array-of-a-struct-inside-ano%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
nvQJw,LwjbMiyqMK092q79wxpx94YKV,qmrp 93 AA7nUM4Ajo3S8CkZ2egAGSc5,R3l1F 1kN6rd
You forgot the prototype and/or function declaration of that snippet of code.
– usr2564301
Nov 11 at 12:27