StringUtils.isBlank() vs String.isEmpty()
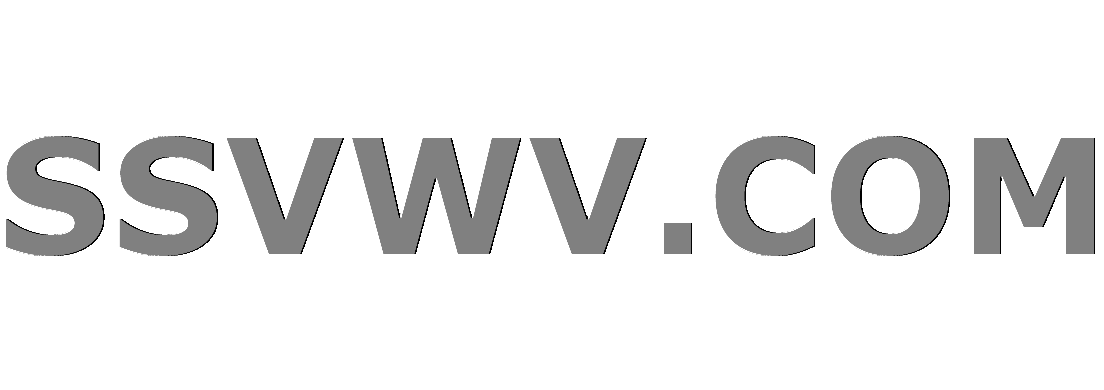
Multi tool use
up vote
127
down vote
favorite
I ran into some code that has the following:
String foo = getvalue("foo");
if (StringUtils.isBlank(foo))
doStuff();
else
doOtherStuff();
This appears to be functionally equivalent to the following:
String foo = getvalue("foo");
if (foo.isEmpty())
doStuff();
else
doOtherStuff();
Is a difference between the two (org.apache.commons.lang3.StringUtils.isBlank
and java.lang.String.isEmpty
)?
java string string-utils
add a comment |
up vote
127
down vote
favorite
I ran into some code that has the following:
String foo = getvalue("foo");
if (StringUtils.isBlank(foo))
doStuff();
else
doOtherStuff();
This appears to be functionally equivalent to the following:
String foo = getvalue("foo");
if (foo.isEmpty())
doStuff();
else
doOtherStuff();
Is a difference between the two (org.apache.commons.lang3.StringUtils.isBlank
and java.lang.String.isEmpty
)?
java string string-utils
6
Might be worth mentioning that there's also aStringUtils.isEmpty(foo)
which helps you avoid null pointers, just likeisBlank
, but doesn't check for whitespace characters.
– Xavi
Jun 24 '14 at 19:32
add a comment |
up vote
127
down vote
favorite
up vote
127
down vote
favorite
I ran into some code that has the following:
String foo = getvalue("foo");
if (StringUtils.isBlank(foo))
doStuff();
else
doOtherStuff();
This appears to be functionally equivalent to the following:
String foo = getvalue("foo");
if (foo.isEmpty())
doStuff();
else
doOtherStuff();
Is a difference between the two (org.apache.commons.lang3.StringUtils.isBlank
and java.lang.String.isEmpty
)?
java string string-utils
I ran into some code that has the following:
String foo = getvalue("foo");
if (StringUtils.isBlank(foo))
doStuff();
else
doOtherStuff();
This appears to be functionally equivalent to the following:
String foo = getvalue("foo");
if (foo.isEmpty())
doStuff();
else
doOtherStuff();
Is a difference between the two (org.apache.commons.lang3.StringUtils.isBlank
and java.lang.String.isEmpty
)?
java string string-utils
java string string-utils
edited Oct 22 '15 at 9:29


rmoestl
1,87321733
1,87321733
asked May 2 '14 at 0:51
NSA
1,64052334
1,64052334
6
Might be worth mentioning that there's also aStringUtils.isEmpty(foo)
which helps you avoid null pointers, just likeisBlank
, but doesn't check for whitespace characters.
– Xavi
Jun 24 '14 at 19:32
add a comment |
6
Might be worth mentioning that there's also aStringUtils.isEmpty(foo)
which helps you avoid null pointers, just likeisBlank
, but doesn't check for whitespace characters.
– Xavi
Jun 24 '14 at 19:32
6
6
Might be worth mentioning that there's also a
StringUtils.isEmpty(foo)
which helps you avoid null pointers, just like isBlank
, but doesn't check for whitespace characters.– Xavi
Jun 24 '14 at 19:32
Might be worth mentioning that there's also a
StringUtils.isEmpty(foo)
which helps you avoid null pointers, just like isBlank
, but doesn't check for whitespace characters.– Xavi
Jun 24 '14 at 19:32
add a comment |
8 Answers
8
active
oldest
votes
up vote
217
down vote
accepted
StringUtils.isBlank()
checks that each character of the string is a whitespace character (or that the string is empty or that it's null). This is totally different than just checking if the string is empty.
From the linked documentation:
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
add a comment |
up vote
103
down vote
The accepted answer from @arshajii is totally correct. However just being more explicit by saying below,
StringUtils.isBlank()
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isEmpty
StringUtils.isEmpty(null) = true
StringUtils.isEmpty("") = true
StringUtils.isEmpty(" ") = false
StringUtils.isEmpty("bob") = false
StringUtils.isEmpty(" bob ") = false
add a comment |
up vote
32
down vote
StringUtils isEmpty = String isEmpty checks + checks for null.
StringUtils isBlank = StringUtils isEmpty checks + checks if the text contains only whitespace character(s).
Useful links for further investigation:
- StringUtils isBlank documentation
- StringUtils isEmpty documentation
- String isEmpty documentation
add a comment |
up vote
13
down vote
StringUtils.isBlank()
will also check for null, whereas this:
String foo = getvalue("foo");
if (foo.isEmpty())
will throw a NullPointerException
if foo
is null.
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
add a comment |
up vote
6
down vote
StringUtils.isBlank
also returns true
for just whitespace:
isBlank(String str)
Checks if a String is whitespace, empty ("") or null.
add a comment |
up vote
4
down vote
StringUtils.isBlank(foo)
will perform a null check for you. If you perform foo.isEmpty()
and foo
is null, you will raise a NullPointerException.
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
add a comment |
up vote
2
down vote
StringUtils.isBlank() returns true for blanks(just whitespaces)and for null String as well. Actually it trims the Char sequences and then performs check.
StringUtils.isEmpty() returns true when there is no charsequence in the String parameter or when String parameter is null. Difference is that isEmpty() returns false if String parameter contains just whiltespaces. It considers whitespaces as a state of being non empty.
add a comment |
up vote
1
down vote
public static boolean isEmpty(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
public static boolean isBlank(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
Both have the same code how will isBlank handle white spaces probably you meant isBlankString this has the code for handling whitespaces.
public static boolean isBlankString( String pString ) {
int strLength;
if( pString == null || (strLength = pString.length()) == 0)
return true;
for(int i=0; i < strLength; i++)
if(!Character.isWhitespace(pString.charAt(i)))
return false;
return false;
}
add a comment |
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
217
down vote
accepted
StringUtils.isBlank()
checks that each character of the string is a whitespace character (or that the string is empty or that it's null). This is totally different than just checking if the string is empty.
From the linked documentation:
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
add a comment |
up vote
217
down vote
accepted
StringUtils.isBlank()
checks that each character of the string is a whitespace character (or that the string is empty or that it's null). This is totally different than just checking if the string is empty.
From the linked documentation:
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
add a comment |
up vote
217
down vote
accepted
up vote
217
down vote
accepted
StringUtils.isBlank()
checks that each character of the string is a whitespace character (or that the string is empty or that it's null). This is totally different than just checking if the string is empty.
From the linked documentation:
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isBlank()
checks that each character of the string is a whitespace character (or that the string is empty or that it's null). This is totally different than just checking if the string is empty.
From the linked documentation:
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
edited May 2 '14 at 1:01
answered May 2 '14 at 0:54


arshajii
99.3k18178249
99.3k18178249
add a comment |
add a comment |
up vote
103
down vote
The accepted answer from @arshajii is totally correct. However just being more explicit by saying below,
StringUtils.isBlank()
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isEmpty
StringUtils.isEmpty(null) = true
StringUtils.isEmpty("") = true
StringUtils.isEmpty(" ") = false
StringUtils.isEmpty("bob") = false
StringUtils.isEmpty(" bob ") = false
add a comment |
up vote
103
down vote
The accepted answer from @arshajii is totally correct. However just being more explicit by saying below,
StringUtils.isBlank()
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isEmpty
StringUtils.isEmpty(null) = true
StringUtils.isEmpty("") = true
StringUtils.isEmpty(" ") = false
StringUtils.isEmpty("bob") = false
StringUtils.isEmpty(" bob ") = false
add a comment |
up vote
103
down vote
up vote
103
down vote
The accepted answer from @arshajii is totally correct. However just being more explicit by saying below,
StringUtils.isBlank()
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isEmpty
StringUtils.isEmpty(null) = true
StringUtils.isEmpty("") = true
StringUtils.isEmpty(" ") = false
StringUtils.isEmpty("bob") = false
StringUtils.isEmpty(" bob ") = false
The accepted answer from @arshajii is totally correct. However just being more explicit by saying below,
StringUtils.isBlank()
StringUtils.isBlank(null) = true
StringUtils.isBlank("") = true
StringUtils.isBlank(" ") = true
StringUtils.isBlank("bob") = false
StringUtils.isBlank(" bob ") = false
StringUtils.isEmpty
StringUtils.isEmpty(null) = true
StringUtils.isEmpty("") = true
StringUtils.isEmpty(" ") = false
StringUtils.isEmpty("bob") = false
StringUtils.isEmpty(" bob ") = false
edited Oct 10 '17 at 16:50
answered Mar 31 '17 at 21:42


nilesh
10.7k44564
10.7k44564
add a comment |
add a comment |
up vote
32
down vote
StringUtils isEmpty = String isEmpty checks + checks for null.
StringUtils isBlank = StringUtils isEmpty checks + checks if the text contains only whitespace character(s).
Useful links for further investigation:
- StringUtils isBlank documentation
- StringUtils isEmpty documentation
- String isEmpty documentation
add a comment |
up vote
32
down vote
StringUtils isEmpty = String isEmpty checks + checks for null.
StringUtils isBlank = StringUtils isEmpty checks + checks if the text contains only whitespace character(s).
Useful links for further investigation:
- StringUtils isBlank documentation
- StringUtils isEmpty documentation
- String isEmpty documentation
add a comment |
up vote
32
down vote
up vote
32
down vote
StringUtils isEmpty = String isEmpty checks + checks for null.
StringUtils isBlank = StringUtils isEmpty checks + checks if the text contains only whitespace character(s).
Useful links for further investigation:
- StringUtils isBlank documentation
- StringUtils isEmpty documentation
- String isEmpty documentation
StringUtils isEmpty = String isEmpty checks + checks for null.
StringUtils isBlank = StringUtils isEmpty checks + checks if the text contains only whitespace character(s).
Useful links for further investigation:
- StringUtils isBlank documentation
- StringUtils isEmpty documentation
- String isEmpty documentation
edited Nov 10 at 18:09
ChrisOdney
1,69282338
1,69282338
answered Sep 26 '14 at 13:13
yallam
6161712
6161712
add a comment |
add a comment |
up vote
13
down vote
StringUtils.isBlank()
will also check for null, whereas this:
String foo = getvalue("foo");
if (foo.isEmpty())
will throw a NullPointerException
if foo
is null.
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
add a comment |
up vote
13
down vote
StringUtils.isBlank()
will also check for null, whereas this:
String foo = getvalue("foo");
if (foo.isEmpty())
will throw a NullPointerException
if foo
is null.
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
add a comment |
up vote
13
down vote
up vote
13
down vote
StringUtils.isBlank()
will also check for null, whereas this:
String foo = getvalue("foo");
if (foo.isEmpty())
will throw a NullPointerException
if foo
is null.
StringUtils.isBlank()
will also check for null, whereas this:
String foo = getvalue("foo");
if (foo.isEmpty())
will throw a NullPointerException
if foo
is null.
edited May 27 '15 at 17:15
answered May 2 '14 at 0:53
chut
54725
54725
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
add a comment |
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
4
4
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:56
1
1
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
This is incorrect. String.isEmpty() will return true if it is null. At least if you are talking about the apache.commons.lang version. I'm not sure about the Spring version.
– ryoung
May 26 '15 at 13:52
1
1
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
The meaning of my comment was edited away (to be fair it could have been more clear from the get-go); I was not comparing StringUtils.isBlank() to StringUtils.isEmpty() - rather StringUtils.isBlank() to the OP's value.isEmpty()
– chut
May 27 '15 at 17:15
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
chut's answer is correct. If java String foo is null, then foo.isEmpty() throws NullPointerException. The apache StringUtils.isBlank(foo) returns true even if foo is null.
– user2590805
Oct 5 at 8:53
add a comment |
up vote
6
down vote
StringUtils.isBlank
also returns true
for just whitespace:
isBlank(String str)
Checks if a String is whitespace, empty ("") or null.
add a comment |
up vote
6
down vote
StringUtils.isBlank
also returns true
for just whitespace:
isBlank(String str)
Checks if a String is whitespace, empty ("") or null.
add a comment |
up vote
6
down vote
up vote
6
down vote
StringUtils.isBlank
also returns true
for just whitespace:
isBlank(String str)
Checks if a String is whitespace, empty ("") or null.
StringUtils.isBlank
also returns true
for just whitespace:
isBlank(String str)
Checks if a String is whitespace, empty ("") or null.
answered May 2 '14 at 0:54
Octavia Togami
1,85331932
1,85331932
add a comment |
add a comment |
up vote
4
down vote
StringUtils.isBlank(foo)
will perform a null check for you. If you perform foo.isEmpty()
and foo
is null, you will raise a NullPointerException.
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
add a comment |
up vote
4
down vote
StringUtils.isBlank(foo)
will perform a null check for you. If you perform foo.isEmpty()
and foo
is null, you will raise a NullPointerException.
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
add a comment |
up vote
4
down vote
up vote
4
down vote
StringUtils.isBlank(foo)
will perform a null check for you. If you perform foo.isEmpty()
and foo
is null, you will raise a NullPointerException.
StringUtils.isBlank(foo)
will perform a null check for you. If you perform foo.isEmpty()
and foo
is null, you will raise a NullPointerException.
answered May 2 '14 at 0:53
Default
8,85111936
8,85111936
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
add a comment |
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
There is a bigger difference than that; see my answer.
– arshajii
May 2 '14 at 0:55
add a comment |
up vote
2
down vote
StringUtils.isBlank() returns true for blanks(just whitespaces)and for null String as well. Actually it trims the Char sequences and then performs check.
StringUtils.isEmpty() returns true when there is no charsequence in the String parameter or when String parameter is null. Difference is that isEmpty() returns false if String parameter contains just whiltespaces. It considers whitespaces as a state of being non empty.
add a comment |
up vote
2
down vote
StringUtils.isBlank() returns true for blanks(just whitespaces)and for null String as well. Actually it trims the Char sequences and then performs check.
StringUtils.isEmpty() returns true when there is no charsequence in the String parameter or when String parameter is null. Difference is that isEmpty() returns false if String parameter contains just whiltespaces. It considers whitespaces as a state of being non empty.
add a comment |
up vote
2
down vote
up vote
2
down vote
StringUtils.isBlank() returns true for blanks(just whitespaces)and for null String as well. Actually it trims the Char sequences and then performs check.
StringUtils.isEmpty() returns true when there is no charsequence in the String parameter or when String parameter is null. Difference is that isEmpty() returns false if String parameter contains just whiltespaces. It considers whitespaces as a state of being non empty.
StringUtils.isBlank() returns true for blanks(just whitespaces)and for null String as well. Actually it trims the Char sequences and then performs check.
StringUtils.isEmpty() returns true when there is no charsequence in the String parameter or when String parameter is null. Difference is that isEmpty() returns false if String parameter contains just whiltespaces. It considers whitespaces as a state of being non empty.
answered Feb 11 '15 at 15:15
user4555388
211
211
add a comment |
add a comment |
up vote
1
down vote
public static boolean isEmpty(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
public static boolean isBlank(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
Both have the same code how will isBlank handle white spaces probably you meant isBlankString this has the code for handling whitespaces.
public static boolean isBlankString( String pString ) {
int strLength;
if( pString == null || (strLength = pString.length()) == 0)
return true;
for(int i=0; i < strLength; i++)
if(!Character.isWhitespace(pString.charAt(i)))
return false;
return false;
}
add a comment |
up vote
1
down vote
public static boolean isEmpty(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
public static boolean isBlank(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
Both have the same code how will isBlank handle white spaces probably you meant isBlankString this has the code for handling whitespaces.
public static boolean isBlankString( String pString ) {
int strLength;
if( pString == null || (strLength = pString.length()) == 0)
return true;
for(int i=0; i < strLength; i++)
if(!Character.isWhitespace(pString.charAt(i)))
return false;
return false;
}
add a comment |
up vote
1
down vote
up vote
1
down vote
public static boolean isEmpty(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
public static boolean isBlank(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
Both have the same code how will isBlank handle white spaces probably you meant isBlankString this has the code for handling whitespaces.
public static boolean isBlankString( String pString ) {
int strLength;
if( pString == null || (strLength = pString.length()) == 0)
return true;
for(int i=0; i < strLength; i++)
if(!Character.isWhitespace(pString.charAt(i)))
return false;
return false;
}
public static boolean isEmpty(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
public static boolean isBlank(String ptext) {
return ptext == null || ptext.trim().length() == 0;
}
Both have the same code how will isBlank handle white spaces probably you meant isBlankString this has the code for handling whitespaces.
public static boolean isBlankString( String pString ) {
int strLength;
if( pString == null || (strLength = pString.length()) == 0)
return true;
for(int i=0; i < strLength; i++)
if(!Character.isWhitespace(pString.charAt(i)))
return false;
return false;
}
answered Apr 13 '16 at 7:47


Apoorva Dhanvanthri
191
191
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f23419087%2fstringutils-isblank-vs-string-isempty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oimeH8Dz7bXUhWO47I 6TXz5SBizIw0NLQXdM3ya,vp,zH5kDYW9HrxQOQmGcldyi18EbRWUHjM
6
Might be worth mentioning that there's also a
StringUtils.isEmpty(foo)
which helps you avoid null pointers, just likeisBlank
, but doesn't check for whitespace characters.– Xavi
Jun 24 '14 at 19:32