javascript inheritance using setPrototypeOf
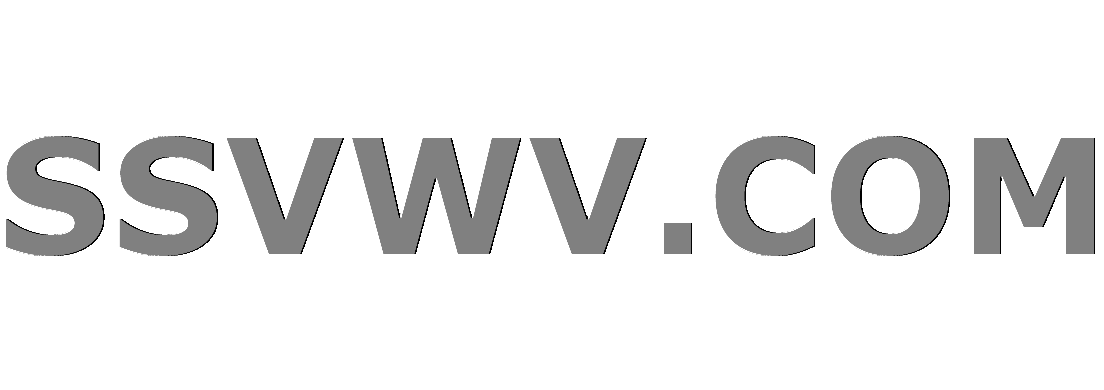
Multi tool use
up vote
1
down vote
favorite
I read here we can use Object.create
to achieve inheritance
Here is an example with a Rectangle
which inherits from Shape
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Rectangle.prototype = Object.create(Shape.prototype);
Rectangle.prototype.constructor = Rectangle;
var rect = new Rectangle();
I am wondering if using Object.setPrototypeOf
instead of Object.create
is correct ?
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
var rect = new Rectangle();
If it's correct, then I am wondering why so many examples show inheritance with Object.create
because you need to worry about the constructor
property when using this method.
With Object.setPrototypeOf
you don't need to redefine the consctrutor
prop, I find it safer and simpler.
javascript prototype prototypal-inheritance
add a comment |
up vote
1
down vote
favorite
I read here we can use Object.create
to achieve inheritance
Here is an example with a Rectangle
which inherits from Shape
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Rectangle.prototype = Object.create(Shape.prototype);
Rectangle.prototype.constructor = Rectangle;
var rect = new Rectangle();
I am wondering if using Object.setPrototypeOf
instead of Object.create
is correct ?
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
var rect = new Rectangle();
If it's correct, then I am wondering why so many examples show inheritance with Object.create
because you need to worry about the constructor
property when using this method.
With Object.setPrototypeOf
you don't need to redefine the consctrutor
prop, I find it safer and simpler.
javascript prototype prototypal-inheritance
Setting theconstructor
property is more like a nice-to-have. It is not required.
– trincot
Nov 10 at 18:29
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set theconstructor
property. It is only there to facilitate. It is not essential.
– trincot
Nov 10 at 18:45
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I read here we can use Object.create
to achieve inheritance
Here is an example with a Rectangle
which inherits from Shape
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Rectangle.prototype = Object.create(Shape.prototype);
Rectangle.prototype.constructor = Rectangle;
var rect = new Rectangle();
I am wondering if using Object.setPrototypeOf
instead of Object.create
is correct ?
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
var rect = new Rectangle();
If it's correct, then I am wondering why so many examples show inheritance with Object.create
because you need to worry about the constructor
property when using this method.
With Object.setPrototypeOf
you don't need to redefine the consctrutor
prop, I find it safer and simpler.
javascript prototype prototypal-inheritance
I read here we can use Object.create
to achieve inheritance
Here is an example with a Rectangle
which inherits from Shape
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Rectangle.prototype = Object.create(Shape.prototype);
Rectangle.prototype.constructor = Rectangle;
var rect = new Rectangle();
I am wondering if using Object.setPrototypeOf
instead of Object.create
is correct ?
function Shape() {}
function Rectangle() {
Shape.call(this);
}
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
var rect = new Rectangle();
If it's correct, then I am wondering why so many examples show inheritance with Object.create
because you need to worry about the constructor
property when using this method.
With Object.setPrototypeOf
you don't need to redefine the consctrutor
prop, I find it safer and simpler.
javascript prototype prototypal-inheritance
javascript prototype prototypal-inheritance
asked Nov 10 at 18:21


Olivier Boissé
3,1301025
3,1301025
Setting theconstructor
property is more like a nice-to-have. It is not required.
– trincot
Nov 10 at 18:29
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set theconstructor
property. It is only there to facilitate. It is not essential.
– trincot
Nov 10 at 18:45
add a comment |
Setting theconstructor
property is more like a nice-to-have. It is not required.
– trincot
Nov 10 at 18:29
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set theconstructor
property. It is only there to facilitate. It is not essential.
– trincot
Nov 10 at 18:45
Setting the
constructor
property is more like a nice-to-have. It is not required.– trincot
Nov 10 at 18:29
Setting the
constructor
property is more like a nice-to-have. It is not required.– trincot
Nov 10 at 18:29
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set the
constructor
property. It is only there to facilitate. It is not essential.– trincot
Nov 10 at 18:45
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set the
constructor
property. It is only there to facilitate. It is not essential.– trincot
Nov 10 at 18:45
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
Sometimes your code may rely on the constructor
property, which allows you to access the constructor without knowing its function name explicitly. But besides that, there is no real need to set it.
For example, the instanceof
operator does not depend on it.
If you don't need to bother about it in your own suggested solution, then you also would not need it in the Object.create
solution.
The reason to prefer the Object.create
method is that Object.setPrototypeOf
may impact the efficiency of your code, as is warned about in the mdn documentation. This does not happen with the Object.create
method.
with my solution (usingObject.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect theRectangle.prototype
object, soCat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)
– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing theprototype
property is a relatively light operation compared to callingsetPrototypeOf
.
– trincot
Nov 10 at 18:47
ok so it's preferable to useObject.create
because of performance
– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Sometimes your code may rely on the constructor
property, which allows you to access the constructor without knowing its function name explicitly. But besides that, there is no real need to set it.
For example, the instanceof
operator does not depend on it.
If you don't need to bother about it in your own suggested solution, then you also would not need it in the Object.create
solution.
The reason to prefer the Object.create
method is that Object.setPrototypeOf
may impact the efficiency of your code, as is warned about in the mdn documentation. This does not happen with the Object.create
method.
with my solution (usingObject.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect theRectangle.prototype
object, soCat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)
– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing theprototype
property is a relatively light operation compared to callingsetPrototypeOf
.
– trincot
Nov 10 at 18:47
ok so it's preferable to useObject.create
because of performance
– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
add a comment |
up vote
1
down vote
Sometimes your code may rely on the constructor
property, which allows you to access the constructor without knowing its function name explicitly. But besides that, there is no real need to set it.
For example, the instanceof
operator does not depend on it.
If you don't need to bother about it in your own suggested solution, then you also would not need it in the Object.create
solution.
The reason to prefer the Object.create
method is that Object.setPrototypeOf
may impact the efficiency of your code, as is warned about in the mdn documentation. This does not happen with the Object.create
method.
with my solution (usingObject.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect theRectangle.prototype
object, soCat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)
– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing theprototype
property is a relatively light operation compared to callingsetPrototypeOf
.
– trincot
Nov 10 at 18:47
ok so it's preferable to useObject.create
because of performance
– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
add a comment |
up vote
1
down vote
up vote
1
down vote
Sometimes your code may rely on the constructor
property, which allows you to access the constructor without knowing its function name explicitly. But besides that, there is no real need to set it.
For example, the instanceof
operator does not depend on it.
If you don't need to bother about it in your own suggested solution, then you also would not need it in the Object.create
solution.
The reason to prefer the Object.create
method is that Object.setPrototypeOf
may impact the efficiency of your code, as is warned about in the mdn documentation. This does not happen with the Object.create
method.
Sometimes your code may rely on the constructor
property, which allows you to access the constructor without knowing its function name explicitly. But besides that, there is no real need to set it.
For example, the instanceof
operator does not depend on it.
If you don't need to bother about it in your own suggested solution, then you also would not need it in the Object.create
solution.
The reason to prefer the Object.create
method is that Object.setPrototypeOf
may impact the efficiency of your code, as is warned about in the mdn documentation. This does not happen with the Object.create
method.
answered Nov 10 at 18:37


trincot
113k1476109
113k1476109
with my solution (usingObject.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect theRectangle.prototype
object, soCat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)
– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing theprototype
property is a relatively light operation compared to callingsetPrototypeOf
.
– trincot
Nov 10 at 18:47
ok so it's preferable to useObject.create
because of performance
– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
add a comment |
with my solution (usingObject.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect theRectangle.prototype
object, soCat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)
– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing theprototype
property is a relatively light operation compared to callingsetPrototypeOf
.
– trincot
Nov 10 at 18:47
ok so it's preferable to useObject.create
because of performance
– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
with my solution (using
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect the Rectangle.prototype
object, so Cat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)– Olivier Boissé
Nov 10 at 18:44
with my solution (using
Object.setPrototypeOf(Rectangle.prototype, Shape.prototype);
) it doesn't reaffect the Rectangle.prototype
object, so Cat.prototype.constructor === Cat
returns true (Cat.prototype.constructor is still valid)– Olivier Boissé
Nov 10 at 18:44
Yes, I see, but still: changing the
prototype
property is a relatively light operation compared to calling setPrototypeOf
.– trincot
Nov 10 at 18:47
Yes, I see, but still: changing the
prototype
property is a relatively light operation compared to calling setPrototypeOf
.– trincot
Nov 10 at 18:47
ok so it's preferable to use
Object.create
because of performance– Olivier Boissé
Nov 10 at 18:49
ok so it's preferable to use
Object.create
because of performance– Olivier Boissé
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
Yep, it boils down to that.
– trincot
Nov 10 at 18:49
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242053%2fjavascript-inheritance-using-setprototypeof%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9SKjPo8K X dl23DPUHVPJ3XE5WaIEuZqLHle
Setting the
constructor
property is more like a nice-to-have. It is not required.– trincot
Nov 10 at 18:29
it's required if we using in one of our method -> developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Olivier Boissé
Nov 10 at 18:41
That is the nice-to-have I talk about, but if one wanted to, they could keep this information in another way. It is not that JavaScript native methods or operators will break or behave differently if you don't set the
constructor
property. It is only there to facilitate. It is not essential.– trincot
Nov 10 at 18:45