Add a limit to users creating a item
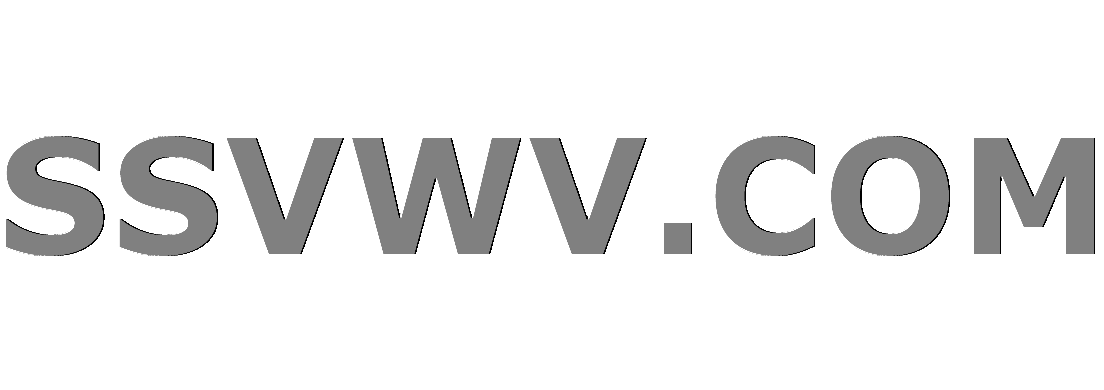
Multi tool use
I am using laravel for a project i am making.
So i want that users can only create 30 products, and if they have more then 30 products, they cant create more products until they have removed some. What do i have to add in my code so that they cant add more products.
My Product controller
public function store(Request $request)
{
//check if user has more then 30 products
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos) <= 5) {
foreach (range(1, $photos) as $i) {
foreach ($photos as $imageData) {
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
} else {
return response()->json("You cant add any photo's to your product", 400);
}
return response()->json([$product, $productPhotos], 201);
}
if i need to send some more code, let me know.
Thx in advance.
FINAL CODE:
$totalProduct = Product::where('supplier_id', $request->user()->Supplier->id)->count();
if ($totalProduct < 30){
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos)) {
foreach ($photos as $i => $imageData) {
if ($i >= 5) {
continue;
}
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
return response()->json([$product, $productPhotos], 201);
} else{
return response()->json("you have to much products", 201);
}
}
php laravel
add a comment |
I am using laravel for a project i am making.
So i want that users can only create 30 products, and if they have more then 30 products, they cant create more products until they have removed some. What do i have to add in my code so that they cant add more products.
My Product controller
public function store(Request $request)
{
//check if user has more then 30 products
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos) <= 5) {
foreach (range(1, $photos) as $i) {
foreach ($photos as $imageData) {
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
} else {
return response()->json("You cant add any photo's to your product", 400);
}
return response()->json([$product, $productPhotos], 201);
}
if i need to send some more code, let me know.
Thx in advance.
FINAL CODE:
$totalProduct = Product::where('supplier_id', $request->user()->Supplier->id)->count();
if ($totalProduct < 30){
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos)) {
foreach ($photos as $i => $imageData) {
if ($i >= 5) {
continue;
}
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
return response()->json([$product, $productPhotos], 201);
} else{
return response()->json("you have to much products", 201);
}
}
php laravel
3
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)
– hktang
Nov 14 '18 at 14:10
add a comment |
I am using laravel for a project i am making.
So i want that users can only create 30 products, and if they have more then 30 products, they cant create more products until they have removed some. What do i have to add in my code so that they cant add more products.
My Product controller
public function store(Request $request)
{
//check if user has more then 30 products
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos) <= 5) {
foreach (range(1, $photos) as $i) {
foreach ($photos as $imageData) {
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
} else {
return response()->json("You cant add any photo's to your product", 400);
}
return response()->json([$product, $productPhotos], 201);
}
if i need to send some more code, let me know.
Thx in advance.
FINAL CODE:
$totalProduct = Product::where('supplier_id', $request->user()->Supplier->id)->count();
if ($totalProduct < 30){
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos)) {
foreach ($photos as $i => $imageData) {
if ($i >= 5) {
continue;
}
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
return response()->json([$product, $productPhotos], 201);
} else{
return response()->json("you have to much products", 201);
}
}
php laravel
I am using laravel for a project i am making.
So i want that users can only create 30 products, and if they have more then 30 products, they cant create more products until they have removed some. What do i have to add in my code so that they cant add more products.
My Product controller
public function store(Request $request)
{
//check if user has more then 30 products
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos) <= 5) {
foreach (range(1, $photos) as $i) {
foreach ($photos as $imageData) {
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
} else {
return response()->json("You cant add any photo's to your product", 400);
}
return response()->json([$product, $productPhotos], 201);
}
if i need to send some more code, let me know.
Thx in advance.
FINAL CODE:
$totalProduct = Product::where('supplier_id', $request->user()->Supplier->id)->count();
if ($totalProduct < 30){
$product = Product::create($request->all());
$productPhotos = ;
$photos = $request->post('photo');
if (count($photos)) {
foreach ($photos as $i => $imageData) {
if ($i >= 5) {
continue;
}
$bcheck = explode(';', $imageData);
if (count($bcheck) > 1) {
list($type, $imageData) = explode(';', $imageData);
list(, $extension) = explode('/', $type);
list(, $imageData) = explode(',', $imageData);
$fileName = uniqid() . '.' . $extension;
$imageData = base64_decode($imageData);
Storage::put("public/products/$fileName", $imageData);
$imagePath = ('storage/products/' . $fileName);
$productPhotos = ProductPhoto::create([
'product_id' => $product->id,
'path' => $imagePath
]);
}
}
}
return response()->json([$product, $productPhotos], 201);
} else{
return response()->json("you have to much products", 201);
}
}
php laravel
php laravel
edited Nov 14 '18 at 14:50
JabirM
asked Nov 14 '18 at 14:00
JabirMJabirM
144
144
3
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)
– hktang
Nov 14 '18 at 14:10
add a comment |
3
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)
– hktang
Nov 14 '18 at 14:10
3
3
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)– hktang
Nov 14 '18 at 14:10
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)– hktang
Nov 14 '18 at 14:10
add a comment |
4 Answers
4
active
oldest
votes
User Model
public function projects()
{
return $this->hasMany('AppProject', 'id', 'user_id');
}
Project Model
public function users()
{
return $this->belongsTo('AppUser', 'user_id', 'id');
}
Controller to Add projects
public function store(Request $request)
{
$totalCreatedProjects = $request->user()->products->count();
if ($totalCreatedProjects < 30) {
`Your code to add projects here`
}
`Your code to alert maximum projects achieved here`
}
This example assumed:
1. Your users are authenticated;
2. You created a database relationship 1 to many between Projects and Users;
Notes: When you compare to the amount of projects that exist and the number you wish to hold the creation instead of proceeding, this should be a service.
The number should be a constant with a proper semantic so other developers understand what you are trying to achieve
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
add a comment |
You can use this:
public function store(Request $request)
{
if($request->user()->products->count() < 30) {
//Add product
...
}else{
return redirect()->back();
}
}
add a comment |
public function store(Request $request)
{
//check if user has more then 29 products
if ($request->user()->products->count() >= 30) {
return response()->json("You cant add more then 30 products", 400);
}
// Your code...
add a comment |
In User Model Add
public function canAddNewProduct()
{
return $this->products->count() < 30;
}
In Controller
Auth::guard('web')->user()->canAddNewProduct()
Better way of doing is make a new middleware and use
return Auth::guard('web')->user()->canAddNewProduct() ? true : false
to protect the routes
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301994%2fadd-a-limit-to-users-creating-a-item%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
User Model
public function projects()
{
return $this->hasMany('AppProject', 'id', 'user_id');
}
Project Model
public function users()
{
return $this->belongsTo('AppUser', 'user_id', 'id');
}
Controller to Add projects
public function store(Request $request)
{
$totalCreatedProjects = $request->user()->products->count();
if ($totalCreatedProjects < 30) {
`Your code to add projects here`
}
`Your code to alert maximum projects achieved here`
}
This example assumed:
1. Your users are authenticated;
2. You created a database relationship 1 to many between Projects and Users;
Notes: When you compare to the amount of projects that exist and the number you wish to hold the creation instead of proceeding, this should be a service.
The number should be a constant with a proper semantic so other developers understand what you are trying to achieve
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
add a comment |
User Model
public function projects()
{
return $this->hasMany('AppProject', 'id', 'user_id');
}
Project Model
public function users()
{
return $this->belongsTo('AppUser', 'user_id', 'id');
}
Controller to Add projects
public function store(Request $request)
{
$totalCreatedProjects = $request->user()->products->count();
if ($totalCreatedProjects < 30) {
`Your code to add projects here`
}
`Your code to alert maximum projects achieved here`
}
This example assumed:
1. Your users are authenticated;
2. You created a database relationship 1 to many between Projects and Users;
Notes: When you compare to the amount of projects that exist and the number you wish to hold the creation instead of proceeding, this should be a service.
The number should be a constant with a proper semantic so other developers understand what you are trying to achieve
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
add a comment |
User Model
public function projects()
{
return $this->hasMany('AppProject', 'id', 'user_id');
}
Project Model
public function users()
{
return $this->belongsTo('AppUser', 'user_id', 'id');
}
Controller to Add projects
public function store(Request $request)
{
$totalCreatedProjects = $request->user()->products->count();
if ($totalCreatedProjects < 30) {
`Your code to add projects here`
}
`Your code to alert maximum projects achieved here`
}
This example assumed:
1. Your users are authenticated;
2. You created a database relationship 1 to many between Projects and Users;
Notes: When you compare to the amount of projects that exist and the number you wish to hold the creation instead of proceeding, this should be a service.
The number should be a constant with a proper semantic so other developers understand what you are trying to achieve
User Model
public function projects()
{
return $this->hasMany('AppProject', 'id', 'user_id');
}
Project Model
public function users()
{
return $this->belongsTo('AppUser', 'user_id', 'id');
}
Controller to Add projects
public function store(Request $request)
{
$totalCreatedProjects = $request->user()->products->count();
if ($totalCreatedProjects < 30) {
`Your code to add projects here`
}
`Your code to alert maximum projects achieved here`
}
This example assumed:
1. Your users are authenticated;
2. You created a database relationship 1 to many between Projects and Users;
Notes: When you compare to the amount of projects that exist and the number you wish to hold the creation instead of proceeding, this should be a service.
The number should be a constant with a proper semantic so other developers understand what you are trying to achieve
edited Nov 14 '18 at 14:25
answered Nov 14 '18 at 14:14


Diogo SantoDiogo Santo
48818
48818
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
add a comment |
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
This somewhat worked for me, i will update a final code.
– JabirM
Nov 14 '18 at 14:40
add a comment |
You can use this:
public function store(Request $request)
{
if($request->user()->products->count() < 30) {
//Add product
...
}else{
return redirect()->back();
}
}
add a comment |
You can use this:
public function store(Request $request)
{
if($request->user()->products->count() < 30) {
//Add product
...
}else{
return redirect()->back();
}
}
add a comment |
You can use this:
public function store(Request $request)
{
if($request->user()->products->count() < 30) {
//Add product
...
}else{
return redirect()->back();
}
}
You can use this:
public function store(Request $request)
{
if($request->user()->products->count() < 30) {
//Add product
...
}else{
return redirect()->back();
}
}
answered Nov 14 '18 at 14:17
hktanghktang
9491429
9491429
add a comment |
add a comment |
public function store(Request $request)
{
//check if user has more then 29 products
if ($request->user()->products->count() >= 30) {
return response()->json("You cant add more then 30 products", 400);
}
// Your code...
add a comment |
public function store(Request $request)
{
//check if user has more then 29 products
if ($request->user()->products->count() >= 30) {
return response()->json("You cant add more then 30 products", 400);
}
// Your code...
add a comment |
public function store(Request $request)
{
//check if user has more then 29 products
if ($request->user()->products->count() >= 30) {
return response()->json("You cant add more then 30 products", 400);
}
// Your code...
public function store(Request $request)
{
//check if user has more then 29 products
if ($request->user()->products->count() >= 30) {
return response()->json("You cant add more then 30 products", 400);
}
// Your code...
answered Nov 14 '18 at 14:13


aleksejjjaleksejjj
1,170416
1,170416
add a comment |
add a comment |
In User Model Add
public function canAddNewProduct()
{
return $this->products->count() < 30;
}
In Controller
Auth::guard('web')->user()->canAddNewProduct()
Better way of doing is make a new middleware and use
return Auth::guard('web')->user()->canAddNewProduct() ? true : false
to protect the routes
add a comment |
In User Model Add
public function canAddNewProduct()
{
return $this->products->count() < 30;
}
In Controller
Auth::guard('web')->user()->canAddNewProduct()
Better way of doing is make a new middleware and use
return Auth::guard('web')->user()->canAddNewProduct() ? true : false
to protect the routes
add a comment |
In User Model Add
public function canAddNewProduct()
{
return $this->products->count() < 30;
}
In Controller
Auth::guard('web')->user()->canAddNewProduct()
Better way of doing is make a new middleware and use
return Auth::guard('web')->user()->canAddNewProduct() ? true : false
to protect the routes
In User Model Add
public function canAddNewProduct()
{
return $this->products->count() < 30;
}
In Controller
Auth::guard('web')->user()->canAddNewProduct()
Better way of doing is make a new middleware and use
return Auth::guard('web')->user()->canAddNewProduct() ? true : false
to protect the routes
answered Nov 14 '18 at 17:56


Dawood AkramDawood Akram
912
912
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301994%2fadd-a-limit-to-users-creating-a-item%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3l,18Ivspo2k,7iJBuwXunfgPYS Po2E,PR6i51M2HwbIAberWwTe LQJI KjzP2BMBzZg,piC kRXU9o
3
$request->user()->products->count()
gets you a count of total products created by the user (assuming the User and Product models are correctly set up)– hktang
Nov 14 '18 at 14:10