React native upload image to php
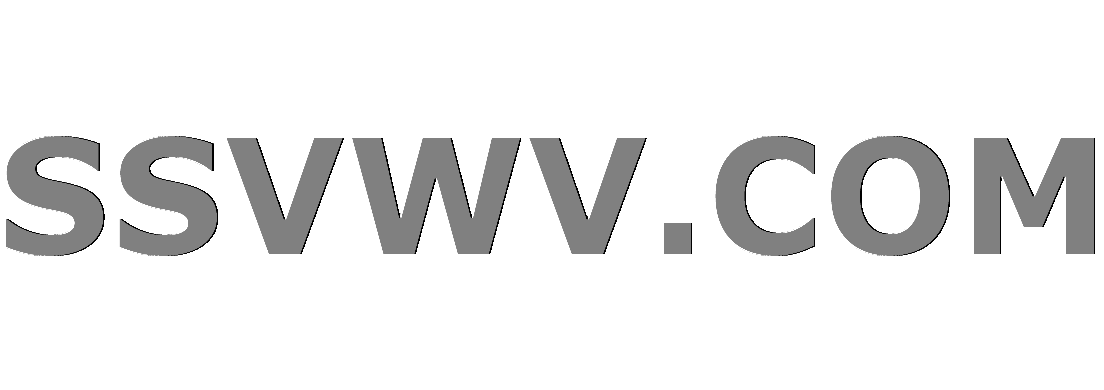
Multi tool use
I'm trying to save an image returned from react-native-view-shot which looks something like this (file:///data/user/ReactNative-snapshot-image123.png) but I don't know why the image is not saved on my server.
This is what I've done.
savePicture = () =>{
this.refs.viewShot.capture().then(uri => {
this.setState(
{
imageURI:uri
});
var data = new FormData();
data.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
fetch('http://www.url.com/upload.php', {
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data'
},
method: 'POST',
body: JSON.stringify({
image: data
})
})
.then((response) => response.json())
.then((responseData) => {console.warn(responseData);})
.catch((error) => {
console.warn(error);
})
.done();
});
}
and here is my php to save the image on my server.
<?
header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Headers: *");
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
$json = json_decode(file_get_contents('php://input'), true);
$img = $json['image'];
list($width,$height,$type) = getimagesize($img);
switch(strtolower(image_type_to_mime_type($type)))
{
case 'image/gif':
$pic = imagecreatefromgif($img);
break;
case 'image/png':
$pic = imagecreatefrompng($img);
break;
case 'image/jpeg':
$pic = imagecreatefromjpeg($img);
break;
default:
return false;
break;
}
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$img,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($img);
echo "done";
}
?>
My PHP just returns this error:
getimagesize() expects parameter 1 to be string, array given
I tried with var_dump($_POST) to see what is returned but I got something like this
{line:21352",column:16,sourceUrl:http.../index.delta?platform=android$dev=true...
Do you have any idea what I'm not doing right?
I've also did
body: data
on my react fetch without json.stringify but still nothing.
Thank you!
Solved! If someone needs this
const formData = new FormData();
formData.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
formData.append('Content-Type', 'image/png');
fetch('http://www.url.com/upload.php',{
method: 'POST',
headers: {
'Content-Type': 'multipart/form-data',
},
body: formData
})
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson);
})
.catch((error) => {
console.log(error);
});
});
PHP
$img = $_FILES['image']['tmp_name'];
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
list($width,$height,$type) = getimagesize($img);
$pic = imagecreatefrompng($img);
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$pic,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($pic);
echo json_encode("done");
php json react-native server upload
|
show 3 more comments
I'm trying to save an image returned from react-native-view-shot which looks something like this (file:///data/user/ReactNative-snapshot-image123.png) but I don't know why the image is not saved on my server.
This is what I've done.
savePicture = () =>{
this.refs.viewShot.capture().then(uri => {
this.setState(
{
imageURI:uri
});
var data = new FormData();
data.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
fetch('http://www.url.com/upload.php', {
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data'
},
method: 'POST',
body: JSON.stringify({
image: data
})
})
.then((response) => response.json())
.then((responseData) => {console.warn(responseData);})
.catch((error) => {
console.warn(error);
})
.done();
});
}
and here is my php to save the image on my server.
<?
header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Headers: *");
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
$json = json_decode(file_get_contents('php://input'), true);
$img = $json['image'];
list($width,$height,$type) = getimagesize($img);
switch(strtolower(image_type_to_mime_type($type)))
{
case 'image/gif':
$pic = imagecreatefromgif($img);
break;
case 'image/png':
$pic = imagecreatefrompng($img);
break;
case 'image/jpeg':
$pic = imagecreatefromjpeg($img);
break;
default:
return false;
break;
}
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$img,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($img);
echo "done";
}
?>
My PHP just returns this error:
getimagesize() expects parameter 1 to be string, array given
I tried with var_dump($_POST) to see what is returned but I got something like this
{line:21352",column:16,sourceUrl:http.../index.delta?platform=android$dev=true...
Do you have any idea what I'm not doing right?
I've also did
body: data
on my react fetch without json.stringify but still nothing.
Thank you!
Solved! If someone needs this
const formData = new FormData();
formData.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
formData.append('Content-Type', 'image/png');
fetch('http://www.url.com/upload.php',{
method: 'POST',
headers: {
'Content-Type': 'multipart/form-data',
},
body: formData
})
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson);
})
.catch((error) => {
console.log(error);
});
});
PHP
$img = $_FILES['image']['tmp_name'];
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
list($width,$height,$type) = getimagesize($img);
$pic = imagecreatefrompng($img);
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$pic,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($pic);
echo json_encode("done");
php json react-native server upload
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09
|
show 3 more comments
I'm trying to save an image returned from react-native-view-shot which looks something like this (file:///data/user/ReactNative-snapshot-image123.png) but I don't know why the image is not saved on my server.
This is what I've done.
savePicture = () =>{
this.refs.viewShot.capture().then(uri => {
this.setState(
{
imageURI:uri
});
var data = new FormData();
data.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
fetch('http://www.url.com/upload.php', {
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data'
},
method: 'POST',
body: JSON.stringify({
image: data
})
})
.then((response) => response.json())
.then((responseData) => {console.warn(responseData);})
.catch((error) => {
console.warn(error);
})
.done();
});
}
and here is my php to save the image on my server.
<?
header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Headers: *");
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
$json = json_decode(file_get_contents('php://input'), true);
$img = $json['image'];
list($width,$height,$type) = getimagesize($img);
switch(strtolower(image_type_to_mime_type($type)))
{
case 'image/gif':
$pic = imagecreatefromgif($img);
break;
case 'image/png':
$pic = imagecreatefrompng($img);
break;
case 'image/jpeg':
$pic = imagecreatefromjpeg($img);
break;
default:
return false;
break;
}
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$img,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($img);
echo "done";
}
?>
My PHP just returns this error:
getimagesize() expects parameter 1 to be string, array given
I tried with var_dump($_POST) to see what is returned but I got something like this
{line:21352",column:16,sourceUrl:http.../index.delta?platform=android$dev=true...
Do you have any idea what I'm not doing right?
I've also did
body: data
on my react fetch without json.stringify but still nothing.
Thank you!
Solved! If someone needs this
const formData = new FormData();
formData.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
formData.append('Content-Type', 'image/png');
fetch('http://www.url.com/upload.php',{
method: 'POST',
headers: {
'Content-Type': 'multipart/form-data',
},
body: formData
})
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson);
})
.catch((error) => {
console.log(error);
});
});
PHP
$img = $_FILES['image']['tmp_name'];
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
list($width,$height,$type) = getimagesize($img);
$pic = imagecreatefrompng($img);
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$pic,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($pic);
echo json_encode("done");
php json react-native server upload
I'm trying to save an image returned from react-native-view-shot which looks something like this (file:///data/user/ReactNative-snapshot-image123.png) but I don't know why the image is not saved on my server.
This is what I've done.
savePicture = () =>{
this.refs.viewShot.capture().then(uri => {
this.setState(
{
imageURI:uri
});
var data = new FormData();
data.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
fetch('http://www.url.com/upload.php', {
headers: {
'Accept': 'application/json',
'Content-Type': 'multipart/form-data'
},
method: 'POST',
body: JSON.stringify({
image: data
})
})
.then((response) => response.json())
.then((responseData) => {console.warn(responseData);})
.catch((error) => {
console.warn(error);
})
.done();
});
}
and here is my php to save the image on my server.
<?
header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Headers: *");
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
$json = json_decode(file_get_contents('php://input'), true);
$img = $json['image'];
list($width,$height,$type) = getimagesize($img);
switch(strtolower(image_type_to_mime_type($type)))
{
case 'image/gif':
$pic = imagecreatefromgif($img);
break;
case 'image/png':
$pic = imagecreatefrompng($img);
break;
case 'image/jpeg':
$pic = imagecreatefromjpeg($img);
break;
default:
return false;
break;
}
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$img,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($img);
echo "done";
}
?>
My PHP just returns this error:
getimagesize() expects parameter 1 to be string, array given
I tried with var_dump($_POST) to see what is returned but I got something like this
{line:21352",column:16,sourceUrl:http.../index.delta?platform=android$dev=true...
Do you have any idea what I'm not doing right?
I've also did
body: data
on my react fetch without json.stringify but still nothing.
Thank you!
Solved! If someone needs this
const formData = new FormData();
formData.append('image', {
uri: this.state.imageURI,
name: 'my_photo.png',
type: 'image/png'
});
formData.append('Content-Type', 'image/png');
fetch('http://www.url.com/upload.php',{
method: 'POST',
headers: {
'Content-Type': 'multipart/form-data',
},
body: formData
})
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson);
})
.catch((error) => {
console.log(error);
});
});
PHP
$img = $_FILES['image']['tmp_name'];
$shuffledid = str_shuffle(124243543534543534);
$url = $shuffledid;
$path = $_SERVER['DOCUMENT_ROOT'].'/android';
list($width,$height,$type) = getimagesize($img);
$pic = imagecreatefrompng($img);
$bg = imagecreatetruecolor(600,700);
imagecopyresampled($bg,$pic,0,0,0,0,600,700,$width,$height);
imagepng($bg, $path.'/'.$url.'.png');
imagedestroy($pic);
echo json_encode("done");
php json react-native server upload
php json react-native server upload
edited Nov 14 '18 at 12:31
paulv
asked Nov 13 '18 at 20:00
paulvpaulv
188
188
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09
|
show 3 more comments
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09
|
show 3 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288617%2freact-native-upload-image-to-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288617%2freact-native-upload-image-to-php%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XRlF8JUZm nEuyz2QUp
Possible duplicate of Reference - What does this error mean in PHP?
– miken32
Nov 13 '18 at 21:12
Look at the JSON you're building on the client side, and then look at what you're trying to extract from it on the server side. They do not match. I don't know react, but it doesn't look like you're sending an image at all.
– miken32
Nov 13 '18 at 21:15
this.state.imageURI is the image saved on the device cache.
– paulv
Nov 13 '18 at 23:07
But is it an image, or just a URI to an image?
– miken32
Nov 13 '18 at 23:08
Regardless, you have to get to it first, by making sure your PHP knows the correct format of your JSON, which it does not at the moment.
– miken32
Nov 13 '18 at 23:09