getting data from server and passing it around pages Angular
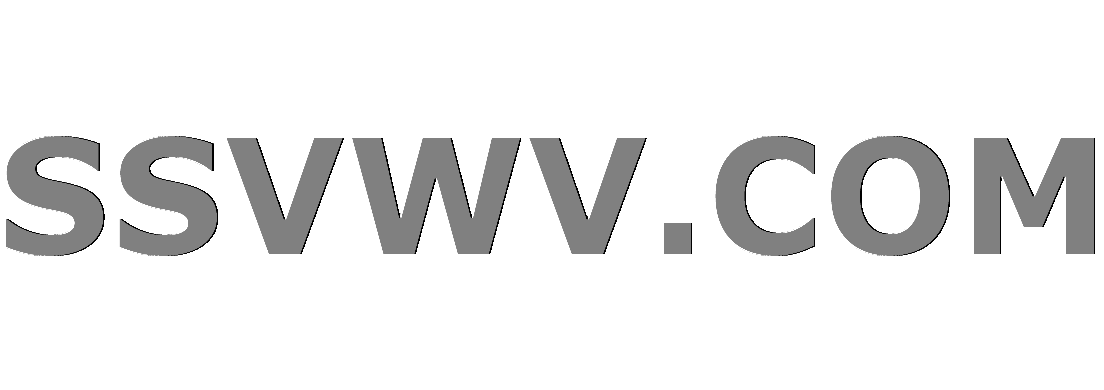
Multi tool use
I have a service that fetches data from an api endpoint in my ionic app. Here is the function that gets data from the server.
getDriverInfo(username)
{
return this.http.get(this.base_url + 'user/' + username, {
headers: new HttpHeaders().set('Authorization', 'Bearer ' + this.access_token)
});
}
i have a page that gets the data is responds with.
handleResponse(data) {
this.db.getDriverInfo(data.username).subscribe(
resp => {
this.driverData = resp;
this.driverId = this.driverData.driver.id;
this.driverUserid = this.driverData.id;
console.log(this.driverData);
}
);
this.token.handle(data.access_token);
this.navCtrl.setRoot(HomePage, {
driverData: this.driverData,
driverId: this.driverId,
driverUserId: this.driverUserId
});
}
toDash()
{
let loading = this.loadCtrl.create({
content:'Logging In...'
});
loading.present();
this.auth.login(this.form).subscribe(
data => {
this.handleResponse(data);
loading.dismiss();
},
error => {
this.handleError(error);
loading.dismiss();
}
);
}
console.log() this.driverData gives me the data.
in my homePage i try to save the data in variables like so
constructor(public navCtrl: NavController, public platform: Platform,
public geo: Geolocation, public loadingCtrl: LoadingController,
public driverProvider: DriverProvider, public alertCtrl: AlertController,
public navParams: NavParams, public modalCtrl: ModalController, public db: DbProvider
) {
this.driverDetails = this.navParams.get('driver_data');
this.driverUserId = this.navParams.get('driver_user_id');
this.driverDBId = this.navParams.get('driver_id');
this.isOnline = this.navParams.get('isOnline');
console.log(this.driverDetails);
}
but console.log gives me undefined. How can i pass the data around efficiently?
angular firebase firebase-realtime-database ionic3 geofire
add a comment |
I have a service that fetches data from an api endpoint in my ionic app. Here is the function that gets data from the server.
getDriverInfo(username)
{
return this.http.get(this.base_url + 'user/' + username, {
headers: new HttpHeaders().set('Authorization', 'Bearer ' + this.access_token)
});
}
i have a page that gets the data is responds with.
handleResponse(data) {
this.db.getDriverInfo(data.username).subscribe(
resp => {
this.driverData = resp;
this.driverId = this.driverData.driver.id;
this.driverUserid = this.driverData.id;
console.log(this.driverData);
}
);
this.token.handle(data.access_token);
this.navCtrl.setRoot(HomePage, {
driverData: this.driverData,
driverId: this.driverId,
driverUserId: this.driverUserId
});
}
toDash()
{
let loading = this.loadCtrl.create({
content:'Logging In...'
});
loading.present();
this.auth.login(this.form).subscribe(
data => {
this.handleResponse(data);
loading.dismiss();
},
error => {
this.handleError(error);
loading.dismiss();
}
);
}
console.log() this.driverData gives me the data.
in my homePage i try to save the data in variables like so
constructor(public navCtrl: NavController, public platform: Platform,
public geo: Geolocation, public loadingCtrl: LoadingController,
public driverProvider: DriverProvider, public alertCtrl: AlertController,
public navParams: NavParams, public modalCtrl: ModalController, public db: DbProvider
) {
this.driverDetails = this.navParams.get('driver_data');
this.driverUserId = this.navParams.get('driver_user_id');
this.driverDBId = this.navParams.get('driver_id');
this.isOnline = this.navParams.get('isOnline');
console.log(this.driverDetails);
}
but console.log gives me undefined. How can i pass the data around efficiently?
angular firebase firebase-realtime-database ionic3 geofire
add a comment |
I have a service that fetches data from an api endpoint in my ionic app. Here is the function that gets data from the server.
getDriverInfo(username)
{
return this.http.get(this.base_url + 'user/' + username, {
headers: new HttpHeaders().set('Authorization', 'Bearer ' + this.access_token)
});
}
i have a page that gets the data is responds with.
handleResponse(data) {
this.db.getDriverInfo(data.username).subscribe(
resp => {
this.driverData = resp;
this.driverId = this.driverData.driver.id;
this.driverUserid = this.driverData.id;
console.log(this.driverData);
}
);
this.token.handle(data.access_token);
this.navCtrl.setRoot(HomePage, {
driverData: this.driverData,
driverId: this.driverId,
driverUserId: this.driverUserId
});
}
toDash()
{
let loading = this.loadCtrl.create({
content:'Logging In...'
});
loading.present();
this.auth.login(this.form).subscribe(
data => {
this.handleResponse(data);
loading.dismiss();
},
error => {
this.handleError(error);
loading.dismiss();
}
);
}
console.log() this.driverData gives me the data.
in my homePage i try to save the data in variables like so
constructor(public navCtrl: NavController, public platform: Platform,
public geo: Geolocation, public loadingCtrl: LoadingController,
public driverProvider: DriverProvider, public alertCtrl: AlertController,
public navParams: NavParams, public modalCtrl: ModalController, public db: DbProvider
) {
this.driverDetails = this.navParams.get('driver_data');
this.driverUserId = this.navParams.get('driver_user_id');
this.driverDBId = this.navParams.get('driver_id');
this.isOnline = this.navParams.get('isOnline');
console.log(this.driverDetails);
}
but console.log gives me undefined. How can i pass the data around efficiently?
angular firebase firebase-realtime-database ionic3 geofire
I have a service that fetches data from an api endpoint in my ionic app. Here is the function that gets data from the server.
getDriverInfo(username)
{
return this.http.get(this.base_url + 'user/' + username, {
headers: new HttpHeaders().set('Authorization', 'Bearer ' + this.access_token)
});
}
i have a page that gets the data is responds with.
handleResponse(data) {
this.db.getDriverInfo(data.username).subscribe(
resp => {
this.driverData = resp;
this.driverId = this.driverData.driver.id;
this.driverUserid = this.driverData.id;
console.log(this.driverData);
}
);
this.token.handle(data.access_token);
this.navCtrl.setRoot(HomePage, {
driverData: this.driverData,
driverId: this.driverId,
driverUserId: this.driverUserId
});
}
toDash()
{
let loading = this.loadCtrl.create({
content:'Logging In...'
});
loading.present();
this.auth.login(this.form).subscribe(
data => {
this.handleResponse(data);
loading.dismiss();
},
error => {
this.handleError(error);
loading.dismiss();
}
);
}
console.log() this.driverData gives me the data.
in my homePage i try to save the data in variables like so
constructor(public navCtrl: NavController, public platform: Platform,
public geo: Geolocation, public loadingCtrl: LoadingController,
public driverProvider: DriverProvider, public alertCtrl: AlertController,
public navParams: NavParams, public modalCtrl: ModalController, public db: DbProvider
) {
this.driverDetails = this.navParams.get('driver_data');
this.driverUserId = this.navParams.get('driver_user_id');
this.driverDBId = this.navParams.get('driver_id');
this.isOnline = this.navParams.get('isOnline');
console.log(this.driverDetails);
}
but console.log gives me undefined. How can i pass the data around efficiently?
angular firebase firebase-realtime-database ionic3 geofire
angular firebase firebase-realtime-database ionic3 geofire
asked Nov 13 '18 at 19:43
Patrick ObafemiPatrick Obafemi
112113
112113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In the HomePage
change the following:
this.driverDetails = this.navParams.get('driver_data');
into this:
this.driverDetails = this.navParams.get('driverData');
When you are sending the data to the second page, you are using the attribute driverData
, thus to recieve this attribute you need to add it exactly the same in the get()
To get all the data, you can do the following inside the constructor:
this.allData = this.navParams.data;
console.log(this.allData);
Check the docs here for more information.
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288395%2fgetting-data-from-server-and-passing-it-around-pages-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In the HomePage
change the following:
this.driverDetails = this.navParams.get('driver_data');
into this:
this.driverDetails = this.navParams.get('driverData');
When you are sending the data to the second page, you are using the attribute driverData
, thus to recieve this attribute you need to add it exactly the same in the get()
To get all the data, you can do the following inside the constructor:
this.allData = this.navParams.data;
console.log(this.allData);
Check the docs here for more information.
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
add a comment |
In the HomePage
change the following:
this.driverDetails = this.navParams.get('driver_data');
into this:
this.driverDetails = this.navParams.get('driverData');
When you are sending the data to the second page, you are using the attribute driverData
, thus to recieve this attribute you need to add it exactly the same in the get()
To get all the data, you can do the following inside the constructor:
this.allData = this.navParams.data;
console.log(this.allData);
Check the docs here for more information.
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
add a comment |
In the HomePage
change the following:
this.driverDetails = this.navParams.get('driver_data');
into this:
this.driverDetails = this.navParams.get('driverData');
When you are sending the data to the second page, you are using the attribute driverData
, thus to recieve this attribute you need to add it exactly the same in the get()
To get all the data, you can do the following inside the constructor:
this.allData = this.navParams.data;
console.log(this.allData);
Check the docs here for more information.
In the HomePage
change the following:
this.driverDetails = this.navParams.get('driver_data');
into this:
this.driverDetails = this.navParams.get('driverData');
When you are sending the data to the second page, you are using the attribute driverData
, thus to recieve this attribute you need to add it exactly the same in the get()
To get all the data, you can do the following inside the constructor:
this.allData = this.navParams.data;
console.log(this.allData);
Check the docs here for more information.
answered Nov 13 '18 at 20:12


Peter HaddadPeter Haddad
20.8k94257
20.8k94257
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
add a comment |
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
1
1
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
Thanks worked for me
– Patrick Obafemi
Nov 13 '18 at 20:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53288395%2fgetting-data-from-server-and-passing-it-around-pages-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eK7xDOkp4t8TGjqQhM2dZf5wl5EE PMtkMyXXPzv5h