Logging Spring REST APIs
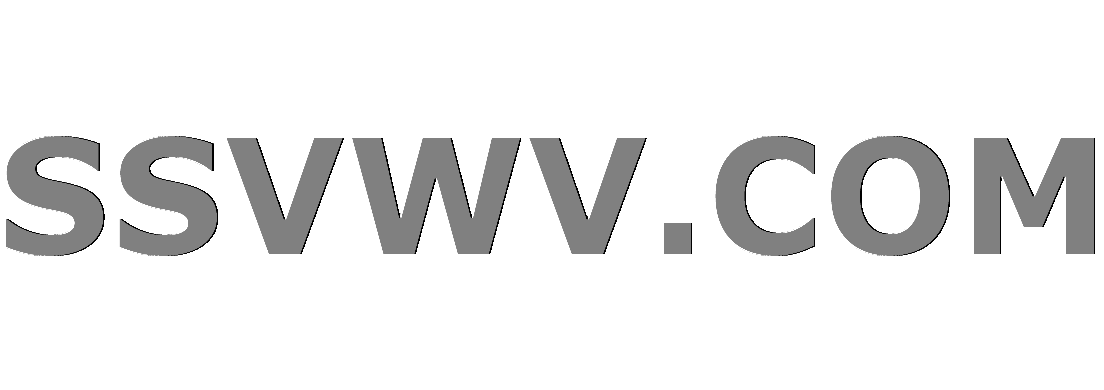
Multi tool use
up vote
0
down vote
favorite
I have custom Filter and I want to log body from request.
But when I use ContentCachingRequestWrapper
and try to call getContentAsByteArray()
I always get an empty array.
@Component
public class CustomFilter implements Filter {
private final Logger log = LoggerFactory.getLogger(CustomFilter.class);
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest requestToCache = new ContentCachingRequestWrapper(request);
chain.doFilter(req, res);
log.info(getRequestData(requestToCache));
}
@Override
public void init(FilterConfig filterConfig) {
}
@Override
public void destroy() {
}
public static String getRequestData(final HttpServletRequest request) throws UnsupportedEncodingException {
String payload = null;
ContentCachingRequestWrapper wrapper = WebUtils.getNativeRequest(request, ContentCachingRequestWrapper.class);
if (wrapper != null) {
byte buf = wrapper.getContentAsByteArray();
if (buf.length > 0) {
payload = new String(buf, 0, buf.length, wrapper.getCharacterEncoding());
}
}
return payload;
}
}
I also tried create Interceptor
, but had the same problem.
What am I doing wrong?
Thanks for help.
java spring logging filter interceptor
add a comment |
up vote
0
down vote
favorite
I have custom Filter and I want to log body from request.
But when I use ContentCachingRequestWrapper
and try to call getContentAsByteArray()
I always get an empty array.
@Component
public class CustomFilter implements Filter {
private final Logger log = LoggerFactory.getLogger(CustomFilter.class);
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest requestToCache = new ContentCachingRequestWrapper(request);
chain.doFilter(req, res);
log.info(getRequestData(requestToCache));
}
@Override
public void init(FilterConfig filterConfig) {
}
@Override
public void destroy() {
}
public static String getRequestData(final HttpServletRequest request) throws UnsupportedEncodingException {
String payload = null;
ContentCachingRequestWrapper wrapper = WebUtils.getNativeRequest(request, ContentCachingRequestWrapper.class);
if (wrapper != null) {
byte buf = wrapper.getContentAsByteArray();
if (buf.length > 0) {
payload = new String(buf, 0, buf.length, wrapper.getCharacterEncoding());
}
}
return payload;
}
}
I also tried create Interceptor
, but had the same problem.
What am I doing wrong?
Thanks for help.
java spring logging filter interceptor
Is this not caused by the fact you're creating aContentCachingRequestWrapper
before your call togetRequestData
, where it's creating anotherContentCachingRequestWrapper
? I suspect because it reads the body when you create the firstContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the firstContentCachingRequestWrapper
into your method rather than creating a second instance.
– jste89
Nov 10 at 17:35
I also used it once and had the same result.
– user_name
Nov 10 at 17:39
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have custom Filter and I want to log body from request.
But when I use ContentCachingRequestWrapper
and try to call getContentAsByteArray()
I always get an empty array.
@Component
public class CustomFilter implements Filter {
private final Logger log = LoggerFactory.getLogger(CustomFilter.class);
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest requestToCache = new ContentCachingRequestWrapper(request);
chain.doFilter(req, res);
log.info(getRequestData(requestToCache));
}
@Override
public void init(FilterConfig filterConfig) {
}
@Override
public void destroy() {
}
public static String getRequestData(final HttpServletRequest request) throws UnsupportedEncodingException {
String payload = null;
ContentCachingRequestWrapper wrapper = WebUtils.getNativeRequest(request, ContentCachingRequestWrapper.class);
if (wrapper != null) {
byte buf = wrapper.getContentAsByteArray();
if (buf.length > 0) {
payload = new String(buf, 0, buf.length, wrapper.getCharacterEncoding());
}
}
return payload;
}
}
I also tried create Interceptor
, but had the same problem.
What am I doing wrong?
Thanks for help.
java spring logging filter interceptor
I have custom Filter and I want to log body from request.
But when I use ContentCachingRequestWrapper
and try to call getContentAsByteArray()
I always get an empty array.
@Component
public class CustomFilter implements Filter {
private final Logger log = LoggerFactory.getLogger(CustomFilter.class);
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain) throws IOException, ServletException {
HttpServletRequest request = (HttpServletRequest) req;
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest requestToCache = new ContentCachingRequestWrapper(request);
chain.doFilter(req, res);
log.info(getRequestData(requestToCache));
}
@Override
public void init(FilterConfig filterConfig) {
}
@Override
public void destroy() {
}
public static String getRequestData(final HttpServletRequest request) throws UnsupportedEncodingException {
String payload = null;
ContentCachingRequestWrapper wrapper = WebUtils.getNativeRequest(request, ContentCachingRequestWrapper.class);
if (wrapper != null) {
byte buf = wrapper.getContentAsByteArray();
if (buf.length > 0) {
payload = new String(buf, 0, buf.length, wrapper.getCharacterEncoding());
}
}
return payload;
}
}
I also tried create Interceptor
, but had the same problem.
What am I doing wrong?
Thanks for help.
java spring logging filter interceptor
java spring logging filter interceptor
edited Nov 10 at 17:34


Hülya
42719
42719
asked Nov 10 at 17:26


user_name
32
32
Is this not caused by the fact you're creating aContentCachingRequestWrapper
before your call togetRequestData
, where it's creating anotherContentCachingRequestWrapper
? I suspect because it reads the body when you create the firstContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the firstContentCachingRequestWrapper
into your method rather than creating a second instance.
– jste89
Nov 10 at 17:35
I also used it once and had the same result.
– user_name
Nov 10 at 17:39
add a comment |
Is this not caused by the fact you're creating aContentCachingRequestWrapper
before your call togetRequestData
, where it's creating anotherContentCachingRequestWrapper
? I suspect because it reads the body when you create the firstContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the firstContentCachingRequestWrapper
into your method rather than creating a second instance.
– jste89
Nov 10 at 17:35
I also used it once and had the same result.
– user_name
Nov 10 at 17:39
Is this not caused by the fact you're creating a
ContentCachingRequestWrapper
before your call to getRequestData
, where it's creating another ContentCachingRequestWrapper
? I suspect because it reads the body when you create the first ContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the first ContentCachingRequestWrapper
into your method rather than creating a second instance.– jste89
Nov 10 at 17:35
Is this not caused by the fact you're creating a
ContentCachingRequestWrapper
before your call to getRequestData
, where it's creating another ContentCachingRequestWrapper
? I suspect because it reads the body when you create the first ContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the first ContentCachingRequestWrapper
into your method rather than creating a second instance.– jste89
Nov 10 at 17:35
I also used it once and had the same result.
– user_name
Nov 10 at 17:39
I also used it once and had the same result.
– user_name
Nov 10 at 17:39
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
You can use the existing spring implementation by just registering this bean in a @Configuration
annotated class:
@Bean
public static Filter requestLoggingFilter() {
final CommonsRequestLoggingFilter loggingFilter = new CommonsRequestLoggingFilter();
loggingFilter.setIncludePayload(true);
loggingFilter.setMaxPayloadLength(512);
return loggingFilter;
}
add a comment |
up vote
0
down vote
Whilst I'd recommend going with NiVer's answer, I've been looking into why this issue occurs and I can finally give you an answer.
When you create a new ContentCachingRequestWrapper
, the internal ByteArrayOutputStream
is initialized but no data is copied to it. The body is only written to the ByteArrayOutputStream
when you call getParameter
, getParameterMap()
, getParameterNames()
or getParameterValues(String name)
methods, and even then the data is only copied if the content type contains application/x-www-form-urlencoded
.
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You can use the existing spring implementation by just registering this bean in a @Configuration
annotated class:
@Bean
public static Filter requestLoggingFilter() {
final CommonsRequestLoggingFilter loggingFilter = new CommonsRequestLoggingFilter();
loggingFilter.setIncludePayload(true);
loggingFilter.setMaxPayloadLength(512);
return loggingFilter;
}
add a comment |
up vote
2
down vote
accepted
You can use the existing spring implementation by just registering this bean in a @Configuration
annotated class:
@Bean
public static Filter requestLoggingFilter() {
final CommonsRequestLoggingFilter loggingFilter = new CommonsRequestLoggingFilter();
loggingFilter.setIncludePayload(true);
loggingFilter.setMaxPayloadLength(512);
return loggingFilter;
}
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You can use the existing spring implementation by just registering this bean in a @Configuration
annotated class:
@Bean
public static Filter requestLoggingFilter() {
final CommonsRequestLoggingFilter loggingFilter = new CommonsRequestLoggingFilter();
loggingFilter.setIncludePayload(true);
loggingFilter.setMaxPayloadLength(512);
return loggingFilter;
}
You can use the existing spring implementation by just registering this bean in a @Configuration
annotated class:
@Bean
public static Filter requestLoggingFilter() {
final CommonsRequestLoggingFilter loggingFilter = new CommonsRequestLoggingFilter();
loggingFilter.setIncludePayload(true);
loggingFilter.setMaxPayloadLength(512);
return loggingFilter;
}
answered Nov 10 at 17:35
NiVeR
6,47141930
6,47141930
add a comment |
add a comment |
up vote
0
down vote
Whilst I'd recommend going with NiVer's answer, I've been looking into why this issue occurs and I can finally give you an answer.
When you create a new ContentCachingRequestWrapper
, the internal ByteArrayOutputStream
is initialized but no data is copied to it. The body is only written to the ByteArrayOutputStream
when you call getParameter
, getParameterMap()
, getParameterNames()
or getParameterValues(String name)
methods, and even then the data is only copied if the content type contains application/x-www-form-urlencoded
.
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
add a comment |
up vote
0
down vote
Whilst I'd recommend going with NiVer's answer, I've been looking into why this issue occurs and I can finally give you an answer.
When you create a new ContentCachingRequestWrapper
, the internal ByteArrayOutputStream
is initialized but no data is copied to it. The body is only written to the ByteArrayOutputStream
when you call getParameter
, getParameterMap()
, getParameterNames()
or getParameterValues(String name)
methods, and even then the data is only copied if the content type contains application/x-www-form-urlencoded
.
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
add a comment |
up vote
0
down vote
up vote
0
down vote
Whilst I'd recommend going with NiVer's answer, I've been looking into why this issue occurs and I can finally give you an answer.
When you create a new ContentCachingRequestWrapper
, the internal ByteArrayOutputStream
is initialized but no data is copied to it. The body is only written to the ByteArrayOutputStream
when you call getParameter
, getParameterMap()
, getParameterNames()
or getParameterValues(String name)
methods, and even then the data is only copied if the content type contains application/x-www-form-urlencoded
.
Whilst I'd recommend going with NiVer's answer, I've been looking into why this issue occurs and I can finally give you an answer.
When you create a new ContentCachingRequestWrapper
, the internal ByteArrayOutputStream
is initialized but no data is copied to it. The body is only written to the ByteArrayOutputStream
when you call getParameter
, getParameterMap()
, getParameterNames()
or getParameterValues(String name)
methods, and even then the data is only copied if the content type contains application/x-www-form-urlencoded
.
answered Nov 10 at 19:13
jste89
356314
356314
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
add a comment |
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
I saw empty array and could`t understand why. Thank you for help!
– user_name
Nov 11 at 8:33
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241554%2flogging-spring-rest-apis%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
K4CIgNb4JoCXJE,SWfu61aIrdJzooGxxP6ZUf lkavB f
Is this not caused by the fact you're creating a
ContentCachingRequestWrapper
before your call togetRequestData
, where it's creating anotherContentCachingRequestWrapper
? I suspect because it reads the body when you create the firstContentCachingRequestWrapper
that it can't re-read from the buffer when creating the second and therefore the body is null/empty. You could always pass the firstContentCachingRequestWrapper
into your method rather than creating a second instance.– jste89
Nov 10 at 17:35
I also used it once and had the same result.
– user_name
Nov 10 at 17:39