Leaflet : icon based on data source
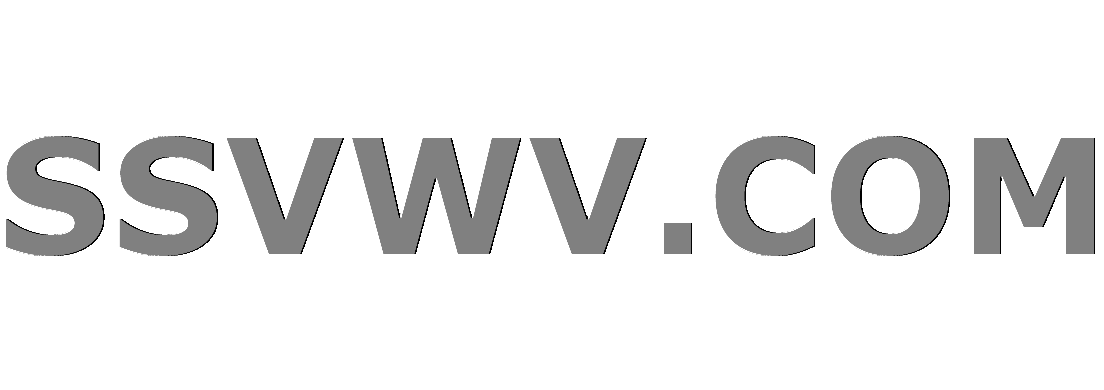
Multi tool use
I am building a website with Leaflet map that uses a data source to populate the map and the popups. Here is how my JSON data looks like:
{
"time_exo": null,
"time_stm": 1541787833,
"results": [
{
"agency": "STM",
"icon": "busSTMIcon",
"vehicle_id": "22210",
"route_id": "55",
"trip_id": "188767688",
"start_time": "12:59:00",
"start_date": "20181109",
"current_stop_sequence": 20,
"current_status": 2,
"lat": 45.52397,
"lon": -73.59475
},
...
}
Here is my JavaScript code:
$.get(dataUrl, function(data, pos_status) {
console.log("pos call result: " + pos_status);
// show data on map
data.results.forEach(function(element) {
L.marker(L.latLng(element.lat, element.lon), {
icon: busSTMIcon
}).addTo(posLayer)
.bindPopup("<h3>" + element.agency + " " + element.vehicle_id + "</h3><b>Trip: </b> " + element.trip_id + "<br><b>Route: </b>" + element.route_id + "<br><b>Start: </b>" + element.start_date + element.start_time + "<br><b>Current stop sequence: </b>" + element.current_stop_sequence + "<br><b>Status: </b>" + element.current_status);
});
});
Here is how I declare my busSTMIcon :
var busSTMIcon = L.icon({
iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg',
iconSize: [20, 20]
});
Currently I use the static way which causes all markers to have the same icon. I would like to have the icon based on my data source. What would be the best way to achieve this?
javascript json leaflet
add a comment |
I am building a website with Leaflet map that uses a data source to populate the map and the popups. Here is how my JSON data looks like:
{
"time_exo": null,
"time_stm": 1541787833,
"results": [
{
"agency": "STM",
"icon": "busSTMIcon",
"vehicle_id": "22210",
"route_id": "55",
"trip_id": "188767688",
"start_time": "12:59:00",
"start_date": "20181109",
"current_stop_sequence": 20,
"current_status": 2,
"lat": 45.52397,
"lon": -73.59475
},
...
}
Here is my JavaScript code:
$.get(dataUrl, function(data, pos_status) {
console.log("pos call result: " + pos_status);
// show data on map
data.results.forEach(function(element) {
L.marker(L.latLng(element.lat, element.lon), {
icon: busSTMIcon
}).addTo(posLayer)
.bindPopup("<h3>" + element.agency + " " + element.vehicle_id + "</h3><b>Trip: </b> " + element.trip_id + "<br><b>Route: </b>" + element.route_id + "<br><b>Start: </b>" + element.start_date + element.start_time + "<br><b>Current stop sequence: </b>" + element.current_stop_sequence + "<br><b>Status: </b>" + element.current_status);
});
});
Here is how I declare my busSTMIcon :
var busSTMIcon = L.icon({
iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg',
iconSize: [20, 20]
});
Currently I use the static way which causes all markers to have the same icon. I would like to have the icon based on my data source. What would be the best way to achieve this?
javascript json leaflet
add a comment |
I am building a website with Leaflet map that uses a data source to populate the map and the popups. Here is how my JSON data looks like:
{
"time_exo": null,
"time_stm": 1541787833,
"results": [
{
"agency": "STM",
"icon": "busSTMIcon",
"vehicle_id": "22210",
"route_id": "55",
"trip_id": "188767688",
"start_time": "12:59:00",
"start_date": "20181109",
"current_stop_sequence": 20,
"current_status": 2,
"lat": 45.52397,
"lon": -73.59475
},
...
}
Here is my JavaScript code:
$.get(dataUrl, function(data, pos_status) {
console.log("pos call result: " + pos_status);
// show data on map
data.results.forEach(function(element) {
L.marker(L.latLng(element.lat, element.lon), {
icon: busSTMIcon
}).addTo(posLayer)
.bindPopup("<h3>" + element.agency + " " + element.vehicle_id + "</h3><b>Trip: </b> " + element.trip_id + "<br><b>Route: </b>" + element.route_id + "<br><b>Start: </b>" + element.start_date + element.start_time + "<br><b>Current stop sequence: </b>" + element.current_stop_sequence + "<br><b>Status: </b>" + element.current_status);
});
});
Here is how I declare my busSTMIcon :
var busSTMIcon = L.icon({
iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg',
iconSize: [20, 20]
});
Currently I use the static way which causes all markers to have the same icon. I would like to have the icon based on my data source. What would be the best way to achieve this?
javascript json leaflet
I am building a website with Leaflet map that uses a data source to populate the map and the popups. Here is how my JSON data looks like:
{
"time_exo": null,
"time_stm": 1541787833,
"results": [
{
"agency": "STM",
"icon": "busSTMIcon",
"vehicle_id": "22210",
"route_id": "55",
"trip_id": "188767688",
"start_time": "12:59:00",
"start_date": "20181109",
"current_stop_sequence": 20,
"current_status": 2,
"lat": 45.52397,
"lon": -73.59475
},
...
}
Here is my JavaScript code:
$.get(dataUrl, function(data, pos_status) {
console.log("pos call result: " + pos_status);
// show data on map
data.results.forEach(function(element) {
L.marker(L.latLng(element.lat, element.lon), {
icon: busSTMIcon
}).addTo(posLayer)
.bindPopup("<h3>" + element.agency + " " + element.vehicle_id + "</h3><b>Trip: </b> " + element.trip_id + "<br><b>Route: </b>" + element.route_id + "<br><b>Start: </b>" + element.start_date + element.start_time + "<br><b>Current stop sequence: </b>" + element.current_stop_sequence + "<br><b>Status: </b>" + element.current_status);
});
});
Here is how I declare my busSTMIcon :
var busSTMIcon = L.icon({
iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg',
iconSize: [20, 20]
});
Currently I use the static way which causes all markers to have the same icon. I would like to have the icon based on my data source. What would be the best way to achieve this?
javascript json leaflet
javascript json leaflet
edited Nov 12 '18 at 19:34
Félix Desjardins
asked Nov 12 '18 at 19:10


Félix DesjardinsFélix Desjardins
1,66631429
1,66631429
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If you create the icon variable as part of an object (to which you could add other icons):
var icons = {
"busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] })
};
and then you would write
icon: icons[element.icon]
(instead of icon: busSTMIcon
)
then it would dynamically pick the right item from the icons object based on the string in element.icon
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of yourbusSTMIcon
variable in your original code?
– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
Either that or create the icon as part of an object (to which you could add other icons):var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would writeicon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string inelement.icon
.
– ADyson
Nov 12 '18 at 19:41
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268606%2fleaflet-icon-based-on-data-source%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you create the icon variable as part of an object (to which you could add other icons):
var icons = {
"busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] })
};
and then you would write
icon: icons[element.icon]
(instead of icon: busSTMIcon
)
then it would dynamically pick the right item from the icons object based on the string in element.icon
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of yourbusSTMIcon
variable in your original code?
– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
Either that or create the icon as part of an object (to which you could add other icons):var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would writeicon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string inelement.icon
.
– ADyson
Nov 12 '18 at 19:41
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
|
show 2 more comments
If you create the icon variable as part of an object (to which you could add other icons):
var icons = {
"busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] })
};
and then you would write
icon: icons[element.icon]
(instead of icon: busSTMIcon
)
then it would dynamically pick the right item from the icons object based on the string in element.icon
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of yourbusSTMIcon
variable in your original code?
– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
Either that or create the icon as part of an object (to which you could add other icons):var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would writeicon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string inelement.icon
.
– ADyson
Nov 12 '18 at 19:41
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
|
show 2 more comments
If you create the icon variable as part of an object (to which you could add other icons):
var icons = {
"busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] })
};
and then you would write
icon: icons[element.icon]
(instead of icon: busSTMIcon
)
then it would dynamically pick the right item from the icons object based on the string in element.icon
If you create the icon variable as part of an object (to which you could add other icons):
var icons = {
"busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] })
};
and then you would write
icon: icons[element.icon]
(instead of icon: busSTMIcon
)
then it would dynamically pick the right item from the icons object based on the string in element.icon
edited Nov 14 '18 at 8:50
answered Nov 12 '18 at 19:21


ADysonADyson
23.1k112444
23.1k112444
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of yourbusSTMIcon
variable in your original code?
– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
Either that or create the icon as part of an object (to which you could add other icons):var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would writeicon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string inelement.icon
.
– ADyson
Nov 12 '18 at 19:41
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
|
show 2 more comments
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of yourbusSTMIcon
variable in your original code?
– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
Either that or create the icon as part of an object (to which you could add other icons):var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would writeicon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string inelement.icon
.
– ADyson
Nov 12 '18 at 19:41
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :
var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
That's what I try but it dosen't work. The way that Leaflet work, you have to first declare the icon :
var busSTMIcon = L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] });
– Félix Desjardins
Nov 12 '18 at 19:29
what is the content of your
busSTMIcon
variable in your original code?– ADyson
Nov 12 '18 at 19:30
what is the content of your
busSTMIcon
variable in your original code?– ADyson
Nov 12 '18 at 19:30
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
check my edited post
– Félix Desjardins
Nov 12 '18 at 19:34
2
2
Either that or create the icon as part of an object (to which you could add other icons):
var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would write icon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string in element.icon
.– ADyson
Nov 12 '18 at 19:41
Either that or create the icon as part of an object (to which you could add other icons):
var icons = { "busSTMIcon" : L.icon({ iconUrl: 'https://felixinx.github.io/mtl-gtfs-rt/assets/map-bus-stm.svg', iconSize: [20, 20] }) };
and then you would write icon: icons[element.icon]
so it would dynamically pick the right item from the icons object based on the string in element.icon
.– ADyson
Nov 12 '18 at 19:41
1
1
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
@FélixDesjardins done, thankyou :-)
– ADyson
Nov 14 '18 at 8:51
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53268606%2fleaflet-icon-based-on-data-source%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OF5OqMZRb5cqII339t6lgBGlaDCLg5NP,Z,M6bGrZDo,NDVWMh732,CxoJhQJtyj,J,idz584ta G,2tj5jckD7lcWGZX