How to remove a key from a Python dictionary?
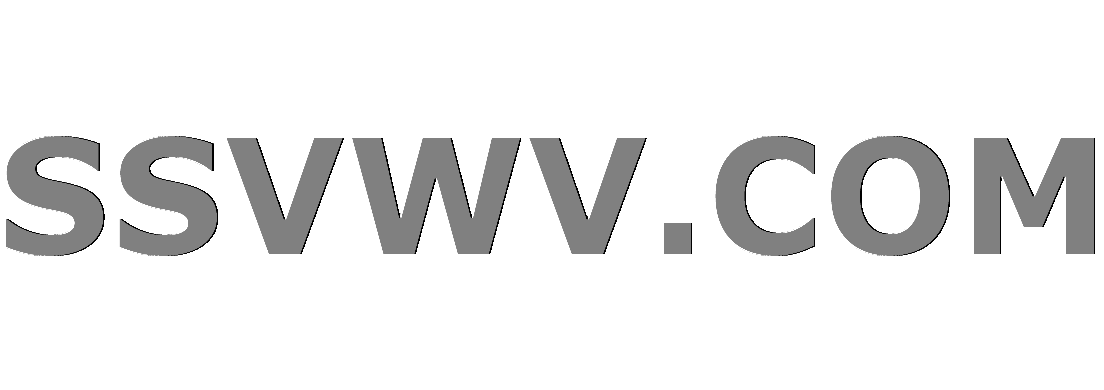
Multi tool use
up vote
1195
down vote
favorite
When trying to delete a key from a dictionary, I write:
if 'key' in myDict:
del myDict['key']
Is there a one line way of doing this?
python dictionary unset
add a comment |
up vote
1195
down vote
favorite
When trying to delete a key from a dictionary, I write:
if 'key' in myDict:
del myDict['key']
Is there a one line way of doing this?
python dictionary unset
14
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19
add a comment |
up vote
1195
down vote
favorite
up vote
1195
down vote
favorite
When trying to delete a key from a dictionary, I write:
if 'key' in myDict:
del myDict['key']
Is there a one line way of doing this?
python dictionary unset
When trying to delete a key from a dictionary, I write:
if 'key' in myDict:
del myDict['key']
Is there a one line way of doing this?
python dictionary unset
python dictionary unset
edited May 29 at 13:43


Peter Mortensen
13.4k1983111
13.4k1983111
asked Jun 30 '12 at 20:27
Tony
10.4k93668
10.4k93668
14
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19
add a comment |
14
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19
14
14
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19
add a comment |
8 Answers
8
active
oldest
votes
up vote
1991
down vote
accepted
Use dict.pop()
:
my_dict.pop('key', None)
This will return my_dict[key]
if key
exists in the dictionary, and None
otherwise. If the second parameter is not specified (ie. my_dict.pop('key')
) and key
does not exist, a KeyError
is raised.
85
Sometimes an advantage of usingpop()
overdel
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.
– kratenko
Aug 18 '13 at 12:21
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than thetry
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.
– Sven Marnach
Jun 15 '17 at 18:44
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returnsself
, so it would be rather surprising if this one did.
– Sven Marnach
Aug 2 at 10:09
|
show 5 more comments
up vote
286
down vote
Specifically to answer "is there a one line way of doing this?"
if 'key' in myDict: del myDict['key']
...well, you asked ;-)
You should consider, though, that this way of deleting an object from a dict
is not atomic—it is possible that 'key'
may be in myDict
during the if
statement, but may be deleted before del
is executed, in which case del
will fail with a KeyError
. Given this, it would be safest to either use dict.pop
or something along the lines of
try:
del myDict['key']
except KeyError:
pass
which, of course, is definitely not a one-liner.
15
Yeah,pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.
– zigg
Jul 1 '12 at 16:30
2
Thetry/except
statement is more expensive. Raising an exception is slow.
– Chris Barker
Aug 20 '13 at 5:01
7
@ChrisBarker I've found if the key exists,try
is marginally faster, though if it doesn't,try
is indeed a good deal slower.pop
is fairly consistent but slower than all buttry
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)
– zigg
Aug 20 '13 at 12:18
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
regarding expense of try/except, you can also goif 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.
– Marc
Jul 9 '15 at 18:00
|
show 4 more comments
up vote
120
down vote
It took me some time to figure out what exactly my_dict.pop("key", None)
is doing. So I'll add this as an answer to save others googling time:
pop(key[, default])
If key is in the dictionary, remove it and return its value, else
return default. If default is not given and key is not in the
dictionary, a KeyError is raised
Documentation
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
ordict.pop?
in IPython.
– Erik Allik
Apr 13 '14 at 1:09
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
add a comment |
up vote
27
down vote
Timing of the three solutions described above.
Small dictionary:
>>> import timeit
>>> timeit.timeit("d={'a':1}; d.pop('a')")
0.23399464370632472
>>> timeit.timeit("d={'a':1}; del d['a']")
0.15225347193388927
>>> timeit.timeit("d={'a':1}; d2 = {key: val for key, val in d.items() if key != 'a'}")
0.5365207354998063
Larger dictionary:
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d.pop(3)")
5.478138627299643
>>> timeit.timeit("d={nr: nr for nr in range(100)}; del d[3]")
5.362219126590048
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d2 = {key: val for key, val in d.items() if key != 3}")
13.93129749387532
13
Normally people do write aconclusion
rather than just dumping some benchmarks.
– user1767754
Dec 20 '17 at 6:46
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
add a comment |
up vote
24
down vote
If you need to remove a lot of keys from a dictionary in one line of code, I think using map() is quite succinct and Pythonic readable:
myDict = {'a':1,'b':2,'c':3,'d':4}
map(myDict.pop, ['a','c']) # The list of keys to remove
>>> myDict
{'b': 2, 'd': 4}
And if you need to catch errors where you pop a value that isn't in the dictionary, use lambda inside map() like this:
map(lambda x: myDict.pop(x,None), ['a','c','e'])
[1, 3, None] # pop returns
>>> myDict
{'b': 2, 'd': 4}
It works. And 'e' did not cause an error, even though myDict did not have an 'e' key.
30
This will not work in Python 3 becausemap
and friends are now lazy and return iterators. Usingmap
for side-effects is generally considered poor practice; a standardfor ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.
– Greg Krimer
Nov 7 '15 at 16:31
2
Regardless of taste and practice style, list comprehensions should still work in Py3[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative tomap
(andfilter
).
– Michael Ekoka
Oct 22 '17 at 8:48
add a comment |
up vote
16
down vote
Use:
>>> if myDict.get(key): myDict.pop(key)
Another way:
>>> {k:v for k, v in myDict.items() if k != 'key'}
You can delete by conditions. No error if key
doesn't exist.
2
dict.has_key
was removed in Python 3, and instead, you should usein
(key in myDict
). You should do this in Python 2 as well.
– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
add a comment |
up vote
8
down vote
Using the "del" keyword:
del dict[key]
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
add a comment |
up vote
5
down vote
We can delete a key from a Python dictionary by the some following approaches.
Using the del
keyword; it's almost the same approach like you did though -
myDict = {'one': 100, 'two': 200, 'three': 300 }
print(myDict) # {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : del myDict['one']
print(myDict) # {'two': 200, 'three': 300}
Or
We can do like following:
But one should keep in mind that, in this process actually it won't delete any key from the dictionary rather than making specific key excluded from that dictionary. In addition, I observed that it returned a dictionary which was not ordered the same as myDict
.
myDict = {'one': 100, 'two': 200, 'three': 300, 'four': 400, 'five': 500}
{key:value for key, value in myDict.items() if key != 'one'}
If we run it in the shell, it'll execute something like {'five': 500, 'four': 400, 'three': 300, 'two': 200}
- notice that it's not the same ordered as myDict
. Again if we try to print myDict
, then we can see all keys including which we excluded from the dictionary by this approach. However, we can make a new dictionary by assigning the following statement into a variable:
var = {key:value for key, value in myDict.items() if key != 'one'}
Now if we try to print it, then it'll follow the parent order:
print(var) # {'two': 200, 'three': 300, 'four': 400, 'five': 500}
Or
Using the pop()
method.
myDict = {'one': 100, 'two': 200, 'three': 300}
print(myDict)
if myDict.get('one') : myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
The difference between del
and pop
is that, using pop()
method, we can actually store the key's value if needed, like the following:
myDict = {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : var = myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
print(var) # 100
Fork this gist for future reference, if you find this useful.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f11277432%2fhow-to-remove-a-key-from-a-python-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1991
down vote
accepted
Use dict.pop()
:
my_dict.pop('key', None)
This will return my_dict[key]
if key
exists in the dictionary, and None
otherwise. If the second parameter is not specified (ie. my_dict.pop('key')
) and key
does not exist, a KeyError
is raised.
85
Sometimes an advantage of usingpop()
overdel
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.
– kratenko
Aug 18 '13 at 12:21
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than thetry
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.
– Sven Marnach
Jun 15 '17 at 18:44
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returnsself
, so it would be rather surprising if this one did.
– Sven Marnach
Aug 2 at 10:09
|
show 5 more comments
up vote
1991
down vote
accepted
Use dict.pop()
:
my_dict.pop('key', None)
This will return my_dict[key]
if key
exists in the dictionary, and None
otherwise. If the second parameter is not specified (ie. my_dict.pop('key')
) and key
does not exist, a KeyError
is raised.
85
Sometimes an advantage of usingpop()
overdel
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.
– kratenko
Aug 18 '13 at 12:21
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than thetry
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.
– Sven Marnach
Jun 15 '17 at 18:44
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returnsself
, so it would be rather surprising if this one did.
– Sven Marnach
Aug 2 at 10:09
|
show 5 more comments
up vote
1991
down vote
accepted
up vote
1991
down vote
accepted
Use dict.pop()
:
my_dict.pop('key', None)
This will return my_dict[key]
if key
exists in the dictionary, and None
otherwise. If the second parameter is not specified (ie. my_dict.pop('key')
) and key
does not exist, a KeyError
is raised.
Use dict.pop()
:
my_dict.pop('key', None)
This will return my_dict[key]
if key
exists in the dictionary, and None
otherwise. If the second parameter is not specified (ie. my_dict.pop('key')
) and key
does not exist, a KeyError
is raised.
edited Aug 5 '16 at 23:23


yiwei
1,58052743
1,58052743
answered Jun 30 '12 at 20:29
Sven Marnach
342k76741692
342k76741692
85
Sometimes an advantage of usingpop()
overdel
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.
– kratenko
Aug 18 '13 at 12:21
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than thetry
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.
– Sven Marnach
Jun 15 '17 at 18:44
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returnsself
, so it would be rather surprising if this one did.
– Sven Marnach
Aug 2 at 10:09
|
show 5 more comments
85
Sometimes an advantage of usingpop()
overdel
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.
– kratenko
Aug 18 '13 at 12:21
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than thetry
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.
– Sven Marnach
Jun 15 '17 at 18:44
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returnsself
, so it would be rather surprising if this one did.
– Sven Marnach
Aug 2 at 10:09
85
85
Sometimes an advantage of using
pop()
over del
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.– kratenko
Aug 18 '13 at 12:21
Sometimes an advantage of using
pop()
over del
: it returns the value for that key. This way you can get and delete an entry from a dict in one line of code.– kratenko
Aug 18 '13 at 12:21
3
3
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
In the question it is not required to keep the value. This would only add unneeded complexity. The answer from @zigg (below) is much better.
– Salvatore Cosentino
Jun 14 '17 at 2:05
5
5
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
@SalvatoreCosentino I can't follow your argument. How is the code in this answer more complex than the code in in the other answer?
– Sven Marnach
Jun 15 '17 at 13:52
13
13
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than the
try
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.– Sven Marnach
Jun 15 '17 at 18:44
@SalvatoreCosentino No, ignoring the return value of a function is not inefficient at all. Quite the opposite – this solution is much faster than the
try
/except
solution if the key does not exist. You might find one or the other easier to read, which is fine. Both are idiomatic Python, so choose whatever you prefer. But claiming that this answer is more complex or inefficient simply makes no sense.– Sven Marnach
Jun 15 '17 at 18:44
3
3
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returns
self
, so it would be rather surprising if this one did.– Sven Marnach
Aug 2 at 10:09
@user5359531 I don't understand. How is this a problem? None of the methods on Python's built-in types returns
self
, so it would be rather surprising if this one did.– Sven Marnach
Aug 2 at 10:09
|
show 5 more comments
up vote
286
down vote
Specifically to answer "is there a one line way of doing this?"
if 'key' in myDict: del myDict['key']
...well, you asked ;-)
You should consider, though, that this way of deleting an object from a dict
is not atomic—it is possible that 'key'
may be in myDict
during the if
statement, but may be deleted before del
is executed, in which case del
will fail with a KeyError
. Given this, it would be safest to either use dict.pop
or something along the lines of
try:
del myDict['key']
except KeyError:
pass
which, of course, is definitely not a one-liner.
15
Yeah,pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.
– zigg
Jul 1 '12 at 16:30
2
Thetry/except
statement is more expensive. Raising an exception is slow.
– Chris Barker
Aug 20 '13 at 5:01
7
@ChrisBarker I've found if the key exists,try
is marginally faster, though if it doesn't,try
is indeed a good deal slower.pop
is fairly consistent but slower than all buttry
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)
– zigg
Aug 20 '13 at 12:18
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
regarding expense of try/except, you can also goif 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.
– Marc
Jul 9 '15 at 18:00
|
show 4 more comments
up vote
286
down vote
Specifically to answer "is there a one line way of doing this?"
if 'key' in myDict: del myDict['key']
...well, you asked ;-)
You should consider, though, that this way of deleting an object from a dict
is not atomic—it is possible that 'key'
may be in myDict
during the if
statement, but may be deleted before del
is executed, in which case del
will fail with a KeyError
. Given this, it would be safest to either use dict.pop
or something along the lines of
try:
del myDict['key']
except KeyError:
pass
which, of course, is definitely not a one-liner.
15
Yeah,pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.
– zigg
Jul 1 '12 at 16:30
2
Thetry/except
statement is more expensive. Raising an exception is slow.
– Chris Barker
Aug 20 '13 at 5:01
7
@ChrisBarker I've found if the key exists,try
is marginally faster, though if it doesn't,try
is indeed a good deal slower.pop
is fairly consistent but slower than all buttry
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)
– zigg
Aug 20 '13 at 12:18
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
regarding expense of try/except, you can also goif 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.
– Marc
Jul 9 '15 at 18:00
|
show 4 more comments
up vote
286
down vote
up vote
286
down vote
Specifically to answer "is there a one line way of doing this?"
if 'key' in myDict: del myDict['key']
...well, you asked ;-)
You should consider, though, that this way of deleting an object from a dict
is not atomic—it is possible that 'key'
may be in myDict
during the if
statement, but may be deleted before del
is executed, in which case del
will fail with a KeyError
. Given this, it would be safest to either use dict.pop
or something along the lines of
try:
del myDict['key']
except KeyError:
pass
which, of course, is definitely not a one-liner.
Specifically to answer "is there a one line way of doing this?"
if 'key' in myDict: del myDict['key']
...well, you asked ;-)
You should consider, though, that this way of deleting an object from a dict
is not atomic—it is possible that 'key'
may be in myDict
during the if
statement, but may be deleted before del
is executed, in which case del
will fail with a KeyError
. Given this, it would be safest to either use dict.pop
or something along the lines of
try:
del myDict['key']
except KeyError:
pass
which, of course, is definitely not a one-liner.
edited May 23 '17 at 11:47
Community♦
11
11
answered Jun 30 '12 at 20:36
zigg
12.5k42749
12.5k42749
15
Yeah,pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.
– zigg
Jul 1 '12 at 16:30
2
Thetry/except
statement is more expensive. Raising an exception is slow.
– Chris Barker
Aug 20 '13 at 5:01
7
@ChrisBarker I've found if the key exists,try
is marginally faster, though if it doesn't,try
is indeed a good deal slower.pop
is fairly consistent but slower than all buttry
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)
– zigg
Aug 20 '13 at 12:18
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
regarding expense of try/except, you can also goif 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.
– Marc
Jul 9 '15 at 18:00
|
show 4 more comments
15
Yeah,pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.
– zigg
Jul 1 '12 at 16:30
2
Thetry/except
statement is more expensive. Raising an exception is slow.
– Chris Barker
Aug 20 '13 at 5:01
7
@ChrisBarker I've found if the key exists,try
is marginally faster, though if it doesn't,try
is indeed a good deal slower.pop
is fairly consistent but slower than all buttry
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)
– zigg
Aug 20 '13 at 12:18
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
regarding expense of try/except, you can also goif 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.
– Marc
Jul 9 '15 at 18:00
15
15
Yeah,
pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.– zigg
Jul 1 '12 at 16:30
Yeah,
pop
is a definitely more concise, though there is one key advantage of doing it this way: it's immediately clear what it's doing.– zigg
Jul 1 '12 at 16:30
2
2
The
try/except
statement is more expensive. Raising an exception is slow.– Chris Barker
Aug 20 '13 at 5:01
The
try/except
statement is more expensive. Raising an exception is slow.– Chris Barker
Aug 20 '13 at 5:01
7
7
@ChrisBarker I've found if the key exists,
try
is marginally faster, though if it doesn't, try
is indeed a good deal slower. pop
is fairly consistent but slower than all but try
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)– zigg
Aug 20 '13 at 12:18
@ChrisBarker I've found if the key exists,
try
is marginally faster, though if it doesn't, try
is indeed a good deal slower. pop
is fairly consistent but slower than all but try
with a non-present key. See gist.github.com/zigg/6280653. Ultimately, it depends on how often you expect the key to actually be in the dictionary, and whether or not you need atomicity—and, of course, whether or not you're engaging in premature optimization ;)– zigg
Aug 20 '13 at 12:18
4
4
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
I believe the value of clarity should not be overlooked. +1 for this.
– Juan Carlos Coto
Jun 30 '14 at 21:15
1
1
regarding expense of try/except, you can also go
if 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.– Marc
Jul 9 '15 at 18:00
regarding expense of try/except, you can also go
if 'key' in mydict: #then del...
. I needed to pull out a key/val from a dict to parse correctly, pop was not a perfect solution.– Marc
Jul 9 '15 at 18:00
|
show 4 more comments
up vote
120
down vote
It took me some time to figure out what exactly my_dict.pop("key", None)
is doing. So I'll add this as an answer to save others googling time:
pop(key[, default])
If key is in the dictionary, remove it and return its value, else
return default. If default is not given and key is not in the
dictionary, a KeyError is raised
Documentation
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
ordict.pop?
in IPython.
– Erik Allik
Apr 13 '14 at 1:09
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
add a comment |
up vote
120
down vote
It took me some time to figure out what exactly my_dict.pop("key", None)
is doing. So I'll add this as an answer to save others googling time:
pop(key[, default])
If key is in the dictionary, remove it and return its value, else
return default. If default is not given and key is not in the
dictionary, a KeyError is raised
Documentation
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
ordict.pop?
in IPython.
– Erik Allik
Apr 13 '14 at 1:09
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
add a comment |
up vote
120
down vote
up vote
120
down vote
It took me some time to figure out what exactly my_dict.pop("key", None)
is doing. So I'll add this as an answer to save others googling time:
pop(key[, default])
If key is in the dictionary, remove it and return its value, else
return default. If default is not given and key is not in the
dictionary, a KeyError is raised
Documentation
It took me some time to figure out what exactly my_dict.pop("key", None)
is doing. So I'll add this as an answer to save others googling time:
pop(key[, default])
If key is in the dictionary, remove it and return its value, else
return default. If default is not given and key is not in the
dictionary, a KeyError is raised
Documentation
edited Feb 27 '15 at 17:29
Community♦
11
11
answered Mar 4 '13 at 16:43


Akavall
39.3k30136176
39.3k30136176
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
ordict.pop?
in IPython.
– Erik Allik
Apr 13 '14 at 1:09
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
add a comment |
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
ordict.pop?
in IPython.
– Erik Allik
Apr 13 '14 at 1:09
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
10
10
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
Just type help(dict.pop) in the python interpreter.
– David Mulder
Aug 6 '13 at 18:07
10
10
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
help() and dir() can be your friends when you need to know what something does.
– David Mulder
Aug 6 '13 at 18:08
2
2
or
dict.pop?
in IPython.– Erik Allik
Apr 13 '14 at 1:09
or
dict.pop?
in IPython.– Erik Allik
Apr 13 '14 at 1:09
3
3
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
Also, the accepted answer had a link to the documentation (with an anchor to the function).
– Michael
Apr 30 '14 at 21:57
add a comment |
up vote
27
down vote
Timing of the three solutions described above.
Small dictionary:
>>> import timeit
>>> timeit.timeit("d={'a':1}; d.pop('a')")
0.23399464370632472
>>> timeit.timeit("d={'a':1}; del d['a']")
0.15225347193388927
>>> timeit.timeit("d={'a':1}; d2 = {key: val for key, val in d.items() if key != 'a'}")
0.5365207354998063
Larger dictionary:
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d.pop(3)")
5.478138627299643
>>> timeit.timeit("d={nr: nr for nr in range(100)}; del d[3]")
5.362219126590048
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d2 = {key: val for key, val in d.items() if key != 3}")
13.93129749387532
13
Normally people do write aconclusion
rather than just dumping some benchmarks.
– user1767754
Dec 20 '17 at 6:46
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
add a comment |
up vote
27
down vote
Timing of the three solutions described above.
Small dictionary:
>>> import timeit
>>> timeit.timeit("d={'a':1}; d.pop('a')")
0.23399464370632472
>>> timeit.timeit("d={'a':1}; del d['a']")
0.15225347193388927
>>> timeit.timeit("d={'a':1}; d2 = {key: val for key, val in d.items() if key != 'a'}")
0.5365207354998063
Larger dictionary:
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d.pop(3)")
5.478138627299643
>>> timeit.timeit("d={nr: nr for nr in range(100)}; del d[3]")
5.362219126590048
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d2 = {key: val for key, val in d.items() if key != 3}")
13.93129749387532
13
Normally people do write aconclusion
rather than just dumping some benchmarks.
– user1767754
Dec 20 '17 at 6:46
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
add a comment |
up vote
27
down vote
up vote
27
down vote
Timing of the three solutions described above.
Small dictionary:
>>> import timeit
>>> timeit.timeit("d={'a':1}; d.pop('a')")
0.23399464370632472
>>> timeit.timeit("d={'a':1}; del d['a']")
0.15225347193388927
>>> timeit.timeit("d={'a':1}; d2 = {key: val for key, val in d.items() if key != 'a'}")
0.5365207354998063
Larger dictionary:
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d.pop(3)")
5.478138627299643
>>> timeit.timeit("d={nr: nr for nr in range(100)}; del d[3]")
5.362219126590048
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d2 = {key: val for key, val in d.items() if key != 3}")
13.93129749387532
Timing of the three solutions described above.
Small dictionary:
>>> import timeit
>>> timeit.timeit("d={'a':1}; d.pop('a')")
0.23399464370632472
>>> timeit.timeit("d={'a':1}; del d['a']")
0.15225347193388927
>>> timeit.timeit("d={'a':1}; d2 = {key: val for key, val in d.items() if key != 'a'}")
0.5365207354998063
Larger dictionary:
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d.pop(3)")
5.478138627299643
>>> timeit.timeit("d={nr: nr for nr in range(100)}; del d[3]")
5.362219126590048
>>> timeit.timeit("d={nr: nr for nr in range(100)}; d2 = {key: val for key, val in d.items() if key != 3}")
13.93129749387532
answered Dec 7 '16 at 12:19
Peter Smit
695714
695714
13
Normally people do write aconclusion
rather than just dumping some benchmarks.
– user1767754
Dec 20 '17 at 6:46
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
add a comment |
13
Normally people do write aconclusion
rather than just dumping some benchmarks.
– user1767754
Dec 20 '17 at 6:46
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
13
13
Normally people do write a
conclusion
rather than just dumping some benchmarks.– user1767754
Dec 20 '17 at 6:46
Normally people do write a
conclusion
rather than just dumping some benchmarks.– user1767754
Dec 20 '17 at 6:46
1
1
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
I'll wrap it up for user1767754 : del ist the fastest method for removing a key from a Python dictionary
– Cpt_Jauchefuerst
Oct 17 at 13:57
add a comment |
up vote
24
down vote
If you need to remove a lot of keys from a dictionary in one line of code, I think using map() is quite succinct and Pythonic readable:
myDict = {'a':1,'b':2,'c':3,'d':4}
map(myDict.pop, ['a','c']) # The list of keys to remove
>>> myDict
{'b': 2, 'd': 4}
And if you need to catch errors where you pop a value that isn't in the dictionary, use lambda inside map() like this:
map(lambda x: myDict.pop(x,None), ['a','c','e'])
[1, 3, None] # pop returns
>>> myDict
{'b': 2, 'd': 4}
It works. And 'e' did not cause an error, even though myDict did not have an 'e' key.
30
This will not work in Python 3 becausemap
and friends are now lazy and return iterators. Usingmap
for side-effects is generally considered poor practice; a standardfor ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.
– Greg Krimer
Nov 7 '15 at 16:31
2
Regardless of taste and practice style, list comprehensions should still work in Py3[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative tomap
(andfilter
).
– Michael Ekoka
Oct 22 '17 at 8:48
add a comment |
up vote
24
down vote
If you need to remove a lot of keys from a dictionary in one line of code, I think using map() is quite succinct and Pythonic readable:
myDict = {'a':1,'b':2,'c':3,'d':4}
map(myDict.pop, ['a','c']) # The list of keys to remove
>>> myDict
{'b': 2, 'd': 4}
And if you need to catch errors where you pop a value that isn't in the dictionary, use lambda inside map() like this:
map(lambda x: myDict.pop(x,None), ['a','c','e'])
[1, 3, None] # pop returns
>>> myDict
{'b': 2, 'd': 4}
It works. And 'e' did not cause an error, even though myDict did not have an 'e' key.
30
This will not work in Python 3 becausemap
and friends are now lazy and return iterators. Usingmap
for side-effects is generally considered poor practice; a standardfor ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.
– Greg Krimer
Nov 7 '15 at 16:31
2
Regardless of taste and practice style, list comprehensions should still work in Py3[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative tomap
(andfilter
).
– Michael Ekoka
Oct 22 '17 at 8:48
add a comment |
up vote
24
down vote
up vote
24
down vote
If you need to remove a lot of keys from a dictionary in one line of code, I think using map() is quite succinct and Pythonic readable:
myDict = {'a':1,'b':2,'c':3,'d':4}
map(myDict.pop, ['a','c']) # The list of keys to remove
>>> myDict
{'b': 2, 'd': 4}
And if you need to catch errors where you pop a value that isn't in the dictionary, use lambda inside map() like this:
map(lambda x: myDict.pop(x,None), ['a','c','e'])
[1, 3, None] # pop returns
>>> myDict
{'b': 2, 'd': 4}
It works. And 'e' did not cause an error, even though myDict did not have an 'e' key.
If you need to remove a lot of keys from a dictionary in one line of code, I think using map() is quite succinct and Pythonic readable:
myDict = {'a':1,'b':2,'c':3,'d':4}
map(myDict.pop, ['a','c']) # The list of keys to remove
>>> myDict
{'b': 2, 'd': 4}
And if you need to catch errors where you pop a value that isn't in the dictionary, use lambda inside map() like this:
map(lambda x: myDict.pop(x,None), ['a','c','e'])
[1, 3, None] # pop returns
>>> myDict
{'b': 2, 'd': 4}
It works. And 'e' did not cause an error, even though myDict did not have an 'e' key.
edited May 29 at 13:53


Peter Mortensen
13.4k1983111
13.4k1983111
answered Sep 16 '15 at 19:17


Marc Maxson
81711326
81711326
30
This will not work in Python 3 becausemap
and friends are now lazy and return iterators. Usingmap
for side-effects is generally considered poor practice; a standardfor ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.
– Greg Krimer
Nov 7 '15 at 16:31
2
Regardless of taste and practice style, list comprehensions should still work in Py3[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative tomap
(andfilter
).
– Michael Ekoka
Oct 22 '17 at 8:48
add a comment |
30
This will not work in Python 3 becausemap
and friends are now lazy and return iterators. Usingmap
for side-effects is generally considered poor practice; a standardfor ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.
– Greg Krimer
Nov 7 '15 at 16:31
2
Regardless of taste and practice style, list comprehensions should still work in Py3[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative tomap
(andfilter
).
– Michael Ekoka
Oct 22 '17 at 8:48
30
30
This will not work in Python 3 because
map
and friends are now lazy and return iterators. Using map
for side-effects is generally considered poor practice; a standard for ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.– Greg Krimer
Nov 7 '15 at 16:31
This will not work in Python 3 because
map
and friends are now lazy and return iterators. Using map
for side-effects is generally considered poor practice; a standard for ... in
loop would be better. See Views And Iterators Instead Of Lists for more information.– Greg Krimer
Nov 7 '15 at 16:31
2
2
Regardless of taste and practice style, list comprehensions should still work in Py3
[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative to map
(and filter
).– Michael Ekoka
Oct 22 '17 at 8:48
Regardless of taste and practice style, list comprehensions should still work in Py3
[myDict.pop(i, None) for i in ['a', 'c']]
, as they offer a general alternative to map
(and filter
).– Michael Ekoka
Oct 22 '17 at 8:48
add a comment |
up vote
16
down vote
Use:
>>> if myDict.get(key): myDict.pop(key)
Another way:
>>> {k:v for k, v in myDict.items() if k != 'key'}
You can delete by conditions. No error if key
doesn't exist.
2
dict.has_key
was removed in Python 3, and instead, you should usein
(key in myDict
). You should do this in Python 2 as well.
– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
add a comment |
up vote
16
down vote
Use:
>>> if myDict.get(key): myDict.pop(key)
Another way:
>>> {k:v for k, v in myDict.items() if k != 'key'}
You can delete by conditions. No error if key
doesn't exist.
2
dict.has_key
was removed in Python 3, and instead, you should usein
(key in myDict
). You should do this in Python 2 as well.
– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
add a comment |
up vote
16
down vote
up vote
16
down vote
Use:
>>> if myDict.get(key): myDict.pop(key)
Another way:
>>> {k:v for k, v in myDict.items() if k != 'key'}
You can delete by conditions. No error if key
doesn't exist.
Use:
>>> if myDict.get(key): myDict.pop(key)
Another way:
>>> {k:v for k, v in myDict.items() if k != 'key'}
You can delete by conditions. No error if key
doesn't exist.
edited May 29 at 13:54


Peter Mortensen
13.4k1983111
13.4k1983111
answered Dec 7 '16 at 5:52


Shameem
929614
929614
2
dict.has_key
was removed in Python 3, and instead, you should usein
(key in myDict
). You should do this in Python 2 as well.
– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
add a comment |
2
dict.has_key
was removed in Python 3, and instead, you should usein
(key in myDict
). You should do this in Python 2 as well.
– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
2
2
dict.has_key
was removed in Python 3, and instead, you should use in
(key in myDict
). You should do this in Python 2 as well.– Artyer
Jun 29 '17 at 15:53
dict.has_key
was removed in Python 3, and instead, you should use in
(key in myDict
). You should do this in Python 2 as well.– Artyer
Jun 29 '17 at 15:53
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
I especially like the way using dictionary comprehension
– Matthias Herrmann
Sep 12 '17 at 17:21
add a comment |
up vote
8
down vote
Using the "del" keyword:
del dict[key]
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
add a comment |
up vote
8
down vote
Using the "del" keyword:
del dict[key]
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
add a comment |
up vote
8
down vote
up vote
8
down vote
Using the "del" keyword:
del dict[key]
Using the "del" keyword:
del dict[key]
edited May 29 at 13:55


Peter Mortensen
13.4k1983111
13.4k1983111
answered May 2 at 10:22


Sarthak Gupta
286314
286314
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
add a comment |
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
Neat solution for deleting key in dict
– Ajay Kumar
May 8 at 9:54
add a comment |
up vote
5
down vote
We can delete a key from a Python dictionary by the some following approaches.
Using the del
keyword; it's almost the same approach like you did though -
myDict = {'one': 100, 'two': 200, 'three': 300 }
print(myDict) # {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : del myDict['one']
print(myDict) # {'two': 200, 'three': 300}
Or
We can do like following:
But one should keep in mind that, in this process actually it won't delete any key from the dictionary rather than making specific key excluded from that dictionary. In addition, I observed that it returned a dictionary which was not ordered the same as myDict
.
myDict = {'one': 100, 'two': 200, 'three': 300, 'four': 400, 'five': 500}
{key:value for key, value in myDict.items() if key != 'one'}
If we run it in the shell, it'll execute something like {'five': 500, 'four': 400, 'three': 300, 'two': 200}
- notice that it's not the same ordered as myDict
. Again if we try to print myDict
, then we can see all keys including which we excluded from the dictionary by this approach. However, we can make a new dictionary by assigning the following statement into a variable:
var = {key:value for key, value in myDict.items() if key != 'one'}
Now if we try to print it, then it'll follow the parent order:
print(var) # {'two': 200, 'three': 300, 'four': 400, 'five': 500}
Or
Using the pop()
method.
myDict = {'one': 100, 'two': 200, 'three': 300}
print(myDict)
if myDict.get('one') : myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
The difference between del
and pop
is that, using pop()
method, we can actually store the key's value if needed, like the following:
myDict = {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : var = myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
print(var) # 100
Fork this gist for future reference, if you find this useful.
add a comment |
up vote
5
down vote
We can delete a key from a Python dictionary by the some following approaches.
Using the del
keyword; it's almost the same approach like you did though -
myDict = {'one': 100, 'two': 200, 'three': 300 }
print(myDict) # {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : del myDict['one']
print(myDict) # {'two': 200, 'three': 300}
Or
We can do like following:
But one should keep in mind that, in this process actually it won't delete any key from the dictionary rather than making specific key excluded from that dictionary. In addition, I observed that it returned a dictionary which was not ordered the same as myDict
.
myDict = {'one': 100, 'two': 200, 'three': 300, 'four': 400, 'five': 500}
{key:value for key, value in myDict.items() if key != 'one'}
If we run it in the shell, it'll execute something like {'five': 500, 'four': 400, 'three': 300, 'two': 200}
- notice that it's not the same ordered as myDict
. Again if we try to print myDict
, then we can see all keys including which we excluded from the dictionary by this approach. However, we can make a new dictionary by assigning the following statement into a variable:
var = {key:value for key, value in myDict.items() if key != 'one'}
Now if we try to print it, then it'll follow the parent order:
print(var) # {'two': 200, 'three': 300, 'four': 400, 'five': 500}
Or
Using the pop()
method.
myDict = {'one': 100, 'two': 200, 'three': 300}
print(myDict)
if myDict.get('one') : myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
The difference between del
and pop
is that, using pop()
method, we can actually store the key's value if needed, like the following:
myDict = {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : var = myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
print(var) # 100
Fork this gist for future reference, if you find this useful.
add a comment |
up vote
5
down vote
up vote
5
down vote
We can delete a key from a Python dictionary by the some following approaches.
Using the del
keyword; it's almost the same approach like you did though -
myDict = {'one': 100, 'two': 200, 'three': 300 }
print(myDict) # {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : del myDict['one']
print(myDict) # {'two': 200, 'three': 300}
Or
We can do like following:
But one should keep in mind that, in this process actually it won't delete any key from the dictionary rather than making specific key excluded from that dictionary. In addition, I observed that it returned a dictionary which was not ordered the same as myDict
.
myDict = {'one': 100, 'two': 200, 'three': 300, 'four': 400, 'five': 500}
{key:value for key, value in myDict.items() if key != 'one'}
If we run it in the shell, it'll execute something like {'five': 500, 'four': 400, 'three': 300, 'two': 200}
- notice that it's not the same ordered as myDict
. Again if we try to print myDict
, then we can see all keys including which we excluded from the dictionary by this approach. However, we can make a new dictionary by assigning the following statement into a variable:
var = {key:value for key, value in myDict.items() if key != 'one'}
Now if we try to print it, then it'll follow the parent order:
print(var) # {'two': 200, 'three': 300, 'four': 400, 'five': 500}
Or
Using the pop()
method.
myDict = {'one': 100, 'two': 200, 'three': 300}
print(myDict)
if myDict.get('one') : myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
The difference between del
and pop
is that, using pop()
method, we can actually store the key's value if needed, like the following:
myDict = {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : var = myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
print(var) # 100
Fork this gist for future reference, if you find this useful.
We can delete a key from a Python dictionary by the some following approaches.
Using the del
keyword; it's almost the same approach like you did though -
myDict = {'one': 100, 'two': 200, 'three': 300 }
print(myDict) # {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : del myDict['one']
print(myDict) # {'two': 200, 'three': 300}
Or
We can do like following:
But one should keep in mind that, in this process actually it won't delete any key from the dictionary rather than making specific key excluded from that dictionary. In addition, I observed that it returned a dictionary which was not ordered the same as myDict
.
myDict = {'one': 100, 'two': 200, 'three': 300, 'four': 400, 'five': 500}
{key:value for key, value in myDict.items() if key != 'one'}
If we run it in the shell, it'll execute something like {'five': 500, 'four': 400, 'three': 300, 'two': 200}
- notice that it's not the same ordered as myDict
. Again if we try to print myDict
, then we can see all keys including which we excluded from the dictionary by this approach. However, we can make a new dictionary by assigning the following statement into a variable:
var = {key:value for key, value in myDict.items() if key != 'one'}
Now if we try to print it, then it'll follow the parent order:
print(var) # {'two': 200, 'three': 300, 'four': 400, 'five': 500}
Or
Using the pop()
method.
myDict = {'one': 100, 'two': 200, 'three': 300}
print(myDict)
if myDict.get('one') : myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
The difference between del
and pop
is that, using pop()
method, we can actually store the key's value if needed, like the following:
myDict = {'one': 100, 'two': 200, 'three': 300}
if myDict.get('one') : var = myDict.pop('one')
print(myDict) # {'two': 200, 'three': 300}
print(var) # 100
Fork this gist for future reference, if you find this useful.
edited Nov 11 at 23:02
answered May 19 at 10:09


iPython
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f11277432%2fhow-to-remove-a-key-from-a-python-dictionary%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6zSwOwpAdbFOwoEYV2l7zR54sO EkW
14
Benchmark script for the various methods proposed in the answers to this question: gist.github.com/zigg/6280653
– zigg
Aug 20 '13 at 12:19