File name “@” character is replaced with “%40” in uploading file to AWS S3 in Android
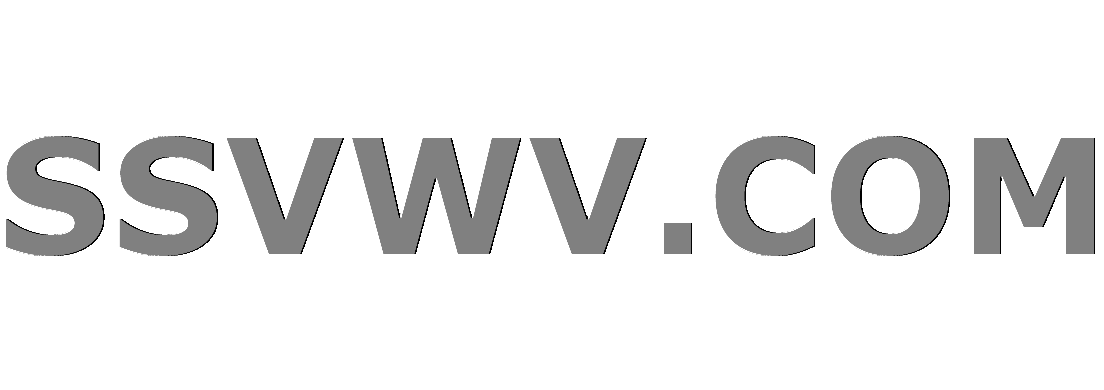
Multi tool use
up vote
0
down vote
favorite
I am developing an Android application. In my application, I am uploading file to AWS S3 bucket. I can upload the file successfully now to the s3 bucket using AWS Android SDK. But the problem is the "@" character in the filename is replaced with "%40" on the s3 Bucket.
This is my code for uploading file to S3
public void s3credentialsProvider()
{
CognitoCachingCredentialsProvider cognitoCachingCredentialsProvider = new CognitoCachingCredentialsProvider(
this.context,
AwsUtility.COGNITO_POOL_ID,
AwsUtility.BUCKET_REGION
);
createAmazonS3Client(cognitoCachingCredentialsProvider );
}
public void createAmazonS3Client(CognitoCachingCredentialsProvider
credentialsProvider){
if(s3Client==null)
{
// Create an S3 client
s3Client = new AmazonS3Client(credentialsProvider);
// Set the region of your S3 bucket
s3Client.setRegion(Region.getRegion(AwsUtility.BUCKET_REGION));
}
}
public void setTransferUtility(){
transferUtility = new TransferUtility(s3Client, context);
}
public CachedFile uploadFileTos3(CachedFile cachedFile)
{
File file = new File(Utilities.getRealPathFromUri(context, Uri.parse(String.valueOf(cachedFile.getFilePath()))));
String fileHash = Utilities.getFileMD5Hash(file);
cachedFile.setFileHash(fileHash);
String extension = file.getAbsolutePath().substring(file.getAbsolutePath().lastIndexOf("."));
String fileId = cachedFile.getEventCode() + "/" + cachedFile.getEmail().replace("%40","@") + "/" + cachedFile.getFileHash() + extension;
try{
//fileId = URLEncoder.encode(fileId, "utf-8");
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
transferObserverListener(transferObserver);
}
catch(Exception e)
{
}
cachedFile.setFileId(fileId);
return cachedFile;
}
public void transferObserverListener(TransferObserver transferObserver){
transferObserver.setTransferListener(new TransferListener(){
@Override
public void onStateChanged(int id, TransferState state) {
Log.i("SYNC_STATE", "State Change" + state);
}
@Override
public void onProgressChanged(int id, long bytesCurrent, long bytesTotal) {
int percentage = (int) (bytesCurrent/bytesTotal * 100);
Log.i("SYNC_PROGRESS", "Progress in %" + percentage);
}
@Override
public void onError(int id, Exception ex) {
Log.e("error","error");
}
});
}
I call the methods in this sequence:
s3credentialsProvide
setTransferUtility
uploadFileTos3
It is uploading to S3. But the problem is with the filename on S3:
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
If I passed this value, "myemail@gmail.com/img-1.jpg" for the fileId, the file on the bucket became "myemail%40gmail.com/img-1.jpg". I do not want the @ character to be replaced. How can I solve the issue?

|
show 4 more comments
up vote
0
down vote
favorite
I am developing an Android application. In my application, I am uploading file to AWS S3 bucket. I can upload the file successfully now to the s3 bucket using AWS Android SDK. But the problem is the "@" character in the filename is replaced with "%40" on the s3 Bucket.
This is my code for uploading file to S3
public void s3credentialsProvider()
{
CognitoCachingCredentialsProvider cognitoCachingCredentialsProvider = new CognitoCachingCredentialsProvider(
this.context,
AwsUtility.COGNITO_POOL_ID,
AwsUtility.BUCKET_REGION
);
createAmazonS3Client(cognitoCachingCredentialsProvider );
}
public void createAmazonS3Client(CognitoCachingCredentialsProvider
credentialsProvider){
if(s3Client==null)
{
// Create an S3 client
s3Client = new AmazonS3Client(credentialsProvider);
// Set the region of your S3 bucket
s3Client.setRegion(Region.getRegion(AwsUtility.BUCKET_REGION));
}
}
public void setTransferUtility(){
transferUtility = new TransferUtility(s3Client, context);
}
public CachedFile uploadFileTos3(CachedFile cachedFile)
{
File file = new File(Utilities.getRealPathFromUri(context, Uri.parse(String.valueOf(cachedFile.getFilePath()))));
String fileHash = Utilities.getFileMD5Hash(file);
cachedFile.setFileHash(fileHash);
String extension = file.getAbsolutePath().substring(file.getAbsolutePath().lastIndexOf("."));
String fileId = cachedFile.getEventCode() + "/" + cachedFile.getEmail().replace("%40","@") + "/" + cachedFile.getFileHash() + extension;
try{
//fileId = URLEncoder.encode(fileId, "utf-8");
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
transferObserverListener(transferObserver);
}
catch(Exception e)
{
}
cachedFile.setFileId(fileId);
return cachedFile;
}
public void transferObserverListener(TransferObserver transferObserver){
transferObserver.setTransferListener(new TransferListener(){
@Override
public void onStateChanged(int id, TransferState state) {
Log.i("SYNC_STATE", "State Change" + state);
}
@Override
public void onProgressChanged(int id, long bytesCurrent, long bytesTotal) {
int percentage = (int) (bytesCurrent/bytesTotal * 100);
Log.i("SYNC_PROGRESS", "Progress in %" + percentage);
}
@Override
public void onError(int id, Exception ex) {
Log.e("error","error");
}
});
}
I call the methods in this sequence:
s3credentialsProvide
setTransferUtility
uploadFileTos3
It is uploading to S3. But the problem is with the filename on S3:
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
If I passed this value, "myemail@gmail.com/img-1.jpg" for the fileId, the file on the bucket became "myemail%40gmail.com/img-1.jpg". I do not want the @ character to be replaced. How can I solve the issue?

docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and@
is a reserved character that needs to URL-encoded.
– CommonsWare
Feb 28 at 18:37
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
1
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46
|
show 4 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am developing an Android application. In my application, I am uploading file to AWS S3 bucket. I can upload the file successfully now to the s3 bucket using AWS Android SDK. But the problem is the "@" character in the filename is replaced with "%40" on the s3 Bucket.
This is my code for uploading file to S3
public void s3credentialsProvider()
{
CognitoCachingCredentialsProvider cognitoCachingCredentialsProvider = new CognitoCachingCredentialsProvider(
this.context,
AwsUtility.COGNITO_POOL_ID,
AwsUtility.BUCKET_REGION
);
createAmazonS3Client(cognitoCachingCredentialsProvider );
}
public void createAmazonS3Client(CognitoCachingCredentialsProvider
credentialsProvider){
if(s3Client==null)
{
// Create an S3 client
s3Client = new AmazonS3Client(credentialsProvider);
// Set the region of your S3 bucket
s3Client.setRegion(Region.getRegion(AwsUtility.BUCKET_REGION));
}
}
public void setTransferUtility(){
transferUtility = new TransferUtility(s3Client, context);
}
public CachedFile uploadFileTos3(CachedFile cachedFile)
{
File file = new File(Utilities.getRealPathFromUri(context, Uri.parse(String.valueOf(cachedFile.getFilePath()))));
String fileHash = Utilities.getFileMD5Hash(file);
cachedFile.setFileHash(fileHash);
String extension = file.getAbsolutePath().substring(file.getAbsolutePath().lastIndexOf("."));
String fileId = cachedFile.getEventCode() + "/" + cachedFile.getEmail().replace("%40","@") + "/" + cachedFile.getFileHash() + extension;
try{
//fileId = URLEncoder.encode(fileId, "utf-8");
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
transferObserverListener(transferObserver);
}
catch(Exception e)
{
}
cachedFile.setFileId(fileId);
return cachedFile;
}
public void transferObserverListener(TransferObserver transferObserver){
transferObserver.setTransferListener(new TransferListener(){
@Override
public void onStateChanged(int id, TransferState state) {
Log.i("SYNC_STATE", "State Change" + state);
}
@Override
public void onProgressChanged(int id, long bytesCurrent, long bytesTotal) {
int percentage = (int) (bytesCurrent/bytesTotal * 100);
Log.i("SYNC_PROGRESS", "Progress in %" + percentage);
}
@Override
public void onError(int id, Exception ex) {
Log.e("error","error");
}
});
}
I call the methods in this sequence:
s3credentialsProvide
setTransferUtility
uploadFileTos3
It is uploading to S3. But the problem is with the filename on S3:
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
If I passed this value, "myemail@gmail.com/img-1.jpg" for the fileId, the file on the bucket became "myemail%40gmail.com/img-1.jpg". I do not want the @ character to be replaced. How can I solve the issue?

I am developing an Android application. In my application, I am uploading file to AWS S3 bucket. I can upload the file successfully now to the s3 bucket using AWS Android SDK. But the problem is the "@" character in the filename is replaced with "%40" on the s3 Bucket.
This is my code for uploading file to S3
public void s3credentialsProvider()
{
CognitoCachingCredentialsProvider cognitoCachingCredentialsProvider = new CognitoCachingCredentialsProvider(
this.context,
AwsUtility.COGNITO_POOL_ID,
AwsUtility.BUCKET_REGION
);
createAmazonS3Client(cognitoCachingCredentialsProvider );
}
public void createAmazonS3Client(CognitoCachingCredentialsProvider
credentialsProvider){
if(s3Client==null)
{
// Create an S3 client
s3Client = new AmazonS3Client(credentialsProvider);
// Set the region of your S3 bucket
s3Client.setRegion(Region.getRegion(AwsUtility.BUCKET_REGION));
}
}
public void setTransferUtility(){
transferUtility = new TransferUtility(s3Client, context);
}
public CachedFile uploadFileTos3(CachedFile cachedFile)
{
File file = new File(Utilities.getRealPathFromUri(context, Uri.parse(String.valueOf(cachedFile.getFilePath()))));
String fileHash = Utilities.getFileMD5Hash(file);
cachedFile.setFileHash(fileHash);
String extension = file.getAbsolutePath().substring(file.getAbsolutePath().lastIndexOf("."));
String fileId = cachedFile.getEventCode() + "/" + cachedFile.getEmail().replace("%40","@") + "/" + cachedFile.getFileHash() + extension;
try{
//fileId = URLEncoder.encode(fileId, "utf-8");
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
transferObserverListener(transferObserver);
}
catch(Exception e)
{
}
cachedFile.setFileId(fileId);
return cachedFile;
}
public void transferObserverListener(TransferObserver transferObserver){
transferObserver.setTransferListener(new TransferListener(){
@Override
public void onStateChanged(int id, TransferState state) {
Log.i("SYNC_STATE", "State Change" + state);
}
@Override
public void onProgressChanged(int id, long bytesCurrent, long bytesTotal) {
int percentage = (int) (bytesCurrent/bytesTotal * 100);
Log.i("SYNC_PROGRESS", "Progress in %" + percentage);
}
@Override
public void onError(int id, Exception ex) {
Log.e("error","error");
}
});
}
I call the methods in this sequence:
s3credentialsProvide
setTransferUtility
uploadFileTos3
It is uploading to S3. But the problem is with the filename on S3:
TransferObserver transferObserver = transferUtility.upload(AwsUtility.BUCKET_NAME, fileId, file);
If I passed this value, "myemail@gmail.com/img-1.jpg" for the fileId, the file on the bucket became "myemail%40gmail.com/img-1.jpg". I do not want the @ character to be replaced. How can I solve the issue?


edited Nov 11 at 23:07


halfer
14.3k758108
14.3k758108
asked Feb 28 at 18:22


Wai Yan Hein
2,40744096
2,40744096
docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and@
is a reserved character that needs to URL-encoded.
– CommonsWare
Feb 28 at 18:37
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
1
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46
|
show 4 more comments
docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and@
is a reserved character that needs to URL-encoded.
– CommonsWare
Feb 28 at 18:37
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
1
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46
docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and
@
is a reserved character that needs to URL-encoded.– CommonsWare
Feb 28 at 18:37
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and
@
is a reserved character that needs to URL-encoded.– CommonsWare
Feb 28 at 18:37
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
1
1
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46
|
show 4 more comments
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49036159%2ffile-name-character-is-replaced-with-40-in-uploading-file-to-aws-s3-in-an%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49036159%2ffile-name-character-is-replaced-with-40-in-uploading-file-to-aws-s3-in-an%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N51tyzubbnevTPPoSsXnyDaHyLh jfvn8ZlwFXWsla1UK99rcknEsd4JIm18
docs.aws.amazon.com/AmazonS3/latest/dev/UsingMetadata.html stackoverflow.com/a/2637825/115145
– CommonsWare
Feb 28 at 18:25
I used UrlEncoder.encode("email") to my email address. It is still not working as expected.
– Wai Yan Hein
Feb 28 at 18:34
What does "the file on the bucket" mean? As the SO answer I pointed to mentions, download URLs have to be URL-encoded, and
@
is a reserved character that needs to URL-encoded.– CommonsWare
Feb 28 at 18:37
But when we upload using PHP and other languages, the @ is not replaced. It is working. Is there a way to do the same in android? Because there are some existing systems using that format too. That is why.
– Wai Yan Hein
Mar 1 at 1:44
1
I am saying that I would follow the S3 documentation and not use arbitrary characters in my keys.
– CommonsWare
Mar 1 at 11:46