Can not set fixed number of threads for Recursive function with two branches using OpenMP
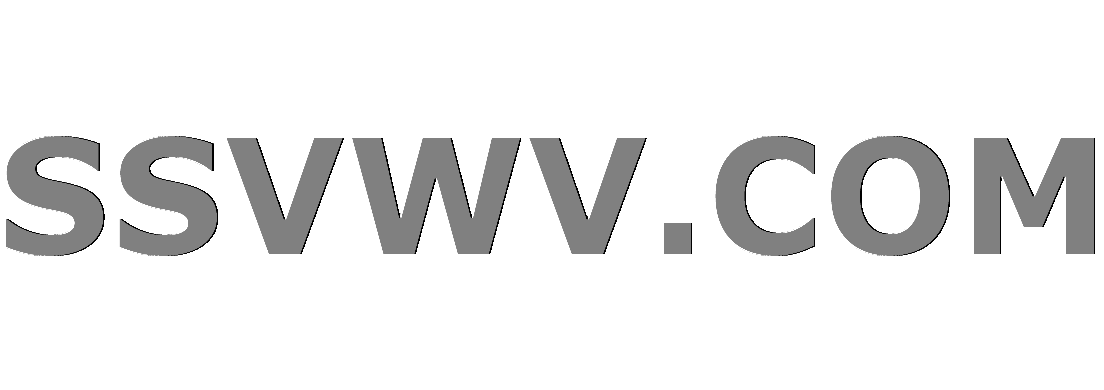
Multi tool use
up vote
0
down vote
favorite
I am trying to parallelize with OpenMP a recursive function with this form:
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
RecursiveFunction(array, index);
RecursiveFunction(array, n - index-1);
}
}
int main()
{
//Serial code
RecursiveFunction(array, size);
//Serial code
}
The compiler (gcc) throws an error when more than a certain number of threads are created (between 800 and 900), stating that "libgomp: Thread creation failed: Resource temporarily unavailable". So I am trying to set a fixed number of threads, using omp_set_num_threads(fixedThreadNumber), so that when, while recursing, more than the maximum number of threads are created, the system must wait for a thread to be freed in order to make another recursion.
Apart from setting the max thread number with omp_set_num_threads, I also tried setting omp_set_dynamic to 0, omp_set_nested to 1 and modifying the recursive function to this form:
int main()
{
//Serial code
omp_set_num_threads(numberOfThreads);
omp_set_dynamic(0);
omp_set_nested(1);
RecursiveFunction(array, size);
//Serial code
}
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
#pragma omp parallel sections
{
#pragma omp section
{
RecursiveFunction(array, index);
}
#pragma omp section
{
RecursiveFunction(array, n-index-1);
}
}
}
}
But no matter what I tried, threads will either be created dynamically and will lead to the aforementioned error, or only two threads will be created and every recursion will use these two threads.
I would be grateful if someone could point me in the right direction.
c multithreading recursion parallel-processing openmp
add a comment |
up vote
0
down vote
favorite
I am trying to parallelize with OpenMP a recursive function with this form:
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
RecursiveFunction(array, index);
RecursiveFunction(array, n - index-1);
}
}
int main()
{
//Serial code
RecursiveFunction(array, size);
//Serial code
}
The compiler (gcc) throws an error when more than a certain number of threads are created (between 800 and 900), stating that "libgomp: Thread creation failed: Resource temporarily unavailable". So I am trying to set a fixed number of threads, using omp_set_num_threads(fixedThreadNumber), so that when, while recursing, more than the maximum number of threads are created, the system must wait for a thread to be freed in order to make another recursion.
Apart from setting the max thread number with omp_set_num_threads, I also tried setting omp_set_dynamic to 0, omp_set_nested to 1 and modifying the recursive function to this form:
int main()
{
//Serial code
omp_set_num_threads(numberOfThreads);
omp_set_dynamic(0);
omp_set_nested(1);
RecursiveFunction(array, size);
//Serial code
}
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
#pragma omp parallel sections
{
#pragma omp section
{
RecursiveFunction(array, index);
}
#pragma omp section
{
RecursiveFunction(array, n-index-1);
}
}
}
}
But no matter what I tried, threads will either be created dynamically and will lead to the aforementioned error, or only two threads will be created and every recursion will use these two threads.
I would be grateful if someone could point me in the right direction.
c multithreading recursion parallel-processing openmp
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
3
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
1
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to parallelize with OpenMP a recursive function with this form:
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
RecursiveFunction(array, index);
RecursiveFunction(array, n - index-1);
}
}
int main()
{
//Serial code
RecursiveFunction(array, size);
//Serial code
}
The compiler (gcc) throws an error when more than a certain number of threads are created (between 800 and 900), stating that "libgomp: Thread creation failed: Resource temporarily unavailable". So I am trying to set a fixed number of threads, using omp_set_num_threads(fixedThreadNumber), so that when, while recursing, more than the maximum number of threads are created, the system must wait for a thread to be freed in order to make another recursion.
Apart from setting the max thread number with omp_set_num_threads, I also tried setting omp_set_dynamic to 0, omp_set_nested to 1 and modifying the recursive function to this form:
int main()
{
//Serial code
omp_set_num_threads(numberOfThreads);
omp_set_dynamic(0);
omp_set_nested(1);
RecursiveFunction(array, size);
//Serial code
}
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
#pragma omp parallel sections
{
#pragma omp section
{
RecursiveFunction(array, index);
}
#pragma omp section
{
RecursiveFunction(array, n-index-1);
}
}
}
}
But no matter what I tried, threads will either be created dynamically and will lead to the aforementioned error, or only two threads will be created and every recursion will use these two threads.
I would be grateful if someone could point me in the right direction.
c multithreading recursion parallel-processing openmp
I am trying to parallelize with OpenMP a recursive function with this form:
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
RecursiveFunction(array, index);
RecursiveFunction(array, n - index-1);
}
}
int main()
{
//Serial code
RecursiveFunction(array, size);
//Serial code
}
The compiler (gcc) throws an error when more than a certain number of threads are created (between 800 and 900), stating that "libgomp: Thread creation failed: Resource temporarily unavailable". So I am trying to set a fixed number of threads, using omp_set_num_threads(fixedThreadNumber), so that when, while recursing, more than the maximum number of threads are created, the system must wait for a thread to be freed in order to make another recursion.
Apart from setting the max thread number with omp_set_num_threads, I also tried setting omp_set_dynamic to 0, omp_set_nested to 1 and modifying the recursive function to this form:
int main()
{
//Serial code
omp_set_num_threads(numberOfThreads);
omp_set_dynamic(0);
omp_set_nested(1);
RecursiveFunction(array, size);
//Serial code
}
void RecursiveFunction(double *array, int size)
{
if(n > 1)
{
//Serial code
//0 < index < n
#pragma omp parallel sections
{
#pragma omp section
{
RecursiveFunction(array, index);
}
#pragma omp section
{
RecursiveFunction(array, n-index-1);
}
}
}
}
But no matter what I tried, threads will either be created dynamically and will lead to the aforementioned error, or only two threads will be created and every recursion will use these two threads.
I would be grateful if someone could point me in the right direction.
c multithreading recursion parallel-processing openmp
c multithreading recursion parallel-processing openmp
asked Nov 11 at 17:10
Vector Sigma
1084
1084
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
3
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
1
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10
add a comment |
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
3
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
1
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
3
3
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
1
1
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251166%2fcan-not-set-fixed-number-of-threads-for-recursive-function-with-two-branches-usi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53251166%2fcan-not-set-fixed-number-of-threads-for-recursive-function-with-two-branches-usi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L3 7SeAk,L,m,EL
I don't understand what you expect will happen here. Once a thread reaches the call but not other threads can be made, what do you think should happen? Also, creating more active threads than there are resources on your CPU will lead to a decrease in performance (which is not generally what you want to achieve by parallelizing code).
– Qubit
Nov 11 at 19:11
3
Take a look at the OpenMP tasks. This is a perfect match for them.
– Gilles
Nov 11 at 19:24
@Gilles Thank you very much for your contribution, I took a look and tasks clearly are suited for the job. Out of curiosity though, I was wondering if there is any other way to do this, since tasks were introduced in the latest version of OpenMP and, thus, I assume there must have been some other way to accomplish something similar using other methods. Trying to make it work without tasks would help me to better understand the whole concept behind OpenMP and parallel programming in general.
– Vector Sigma
Nov 11 at 22:20
1
Tasks were introduced in OpenMP 3.0 more than ten years ago. The current version is 5.0. The reason they were introduced is precisely because there is no other way to (cleanly) accomplish that with the previous methods. There are workarounds for stupid compilers (I'm looking at you MSVC), but they all have severe disadvantages.
– Zulan
Nov 12 at 8:10