How can I display KeyCode and KeyData for lowercase letters in c# winforms
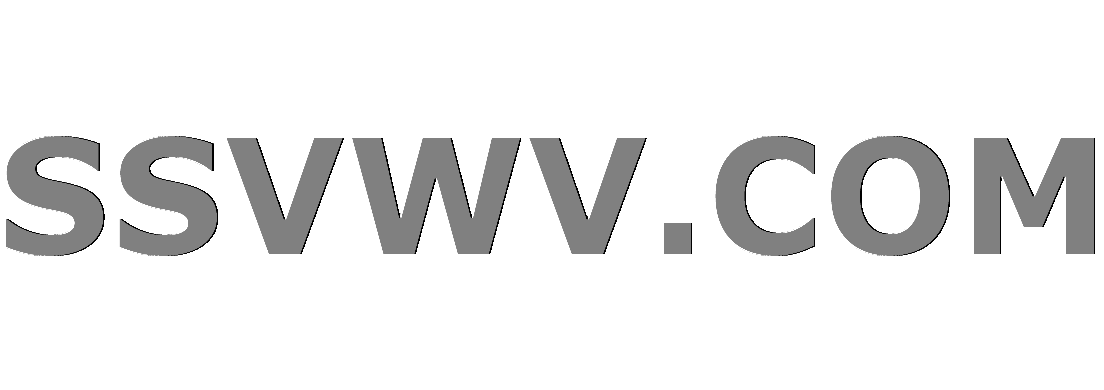
Multi tool use
up vote
0
down vote
favorite
I'm trying to show informations on the Keyboard Keys pressed.
This code displays the Uppercase letters correctly, but the Uppercase information is shown also when a lowercase letter is pressed.
How can I solve this problem?
Here is what I am trying to do:
public partial class keyDemo : Form
{
private void keyDemo_KeyPress(object sender, KeyPressEventArgs e)
{
charLabel.Text = $"Key pressed: {e.KeyChar}";
}
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}";
}
private void keyDemo_KeyUp(object sender, KeyEventArgs e)
{
charLabel.Text = "";
keyInfoLabel.Text = "";
}
}
c# winforms keypress keydown
add a comment |
up vote
0
down vote
favorite
I'm trying to show informations on the Keyboard Keys pressed.
This code displays the Uppercase letters correctly, but the Uppercase information is shown also when a lowercase letter is pressed.
How can I solve this problem?
Here is what I am trying to do:
public partial class keyDemo : Form
{
private void keyDemo_KeyPress(object sender, KeyPressEventArgs e)
{
charLabel.Text = $"Key pressed: {e.KeyChar}";
}
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}";
}
private void keyDemo_KeyUp(object sender, KeyEventArgs e)
{
charLabel.Text = "";
keyInfoLabel.Text = "";
}
}
c# winforms keypress keydown
As you can see,Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.
– Jimi
Nov 10 at 19:40
As you also noticed,KeyPressEventArgs
returns an already processed value for the lettersKeys
, including the lowercase representation.
– Jimi
Nov 10 at 20:35
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm trying to show informations on the Keyboard Keys pressed.
This code displays the Uppercase letters correctly, but the Uppercase information is shown also when a lowercase letter is pressed.
How can I solve this problem?
Here is what I am trying to do:
public partial class keyDemo : Form
{
private void keyDemo_KeyPress(object sender, KeyPressEventArgs e)
{
charLabel.Text = $"Key pressed: {e.KeyChar}";
}
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}";
}
private void keyDemo_KeyUp(object sender, KeyEventArgs e)
{
charLabel.Text = "";
keyInfoLabel.Text = "";
}
}
c# winforms keypress keydown
I'm trying to show informations on the Keyboard Keys pressed.
This code displays the Uppercase letters correctly, but the Uppercase information is shown also when a lowercase letter is pressed.
How can I solve this problem?
Here is what I am trying to do:
public partial class keyDemo : Form
{
private void keyDemo_KeyPress(object sender, KeyPressEventArgs e)
{
charLabel.Text = $"Key pressed: {e.KeyChar}";
}
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}";
}
private void keyDemo_KeyUp(object sender, KeyEventArgs e)
{
charLabel.Text = "";
keyInfoLabel.Text = "";
}
}
c# winforms keypress keydown
c# winforms keypress keydown
edited Nov 10 at 19:02


Jimi
5,78821032
5,78821032
asked Nov 10 at 17:26


Hashim Al-Mansouri
41
41
As you can see,Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.
– Jimi
Nov 10 at 19:40
As you also noticed,KeyPressEventArgs
returns an already processed value for the lettersKeys
, including the lowercase representation.
– Jimi
Nov 10 at 20:35
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02
add a comment |
As you can see,Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.
– Jimi
Nov 10 at 19:40
As you also noticed,KeyPressEventArgs
returns an already processed value for the lettersKeys
, including the lowercase representation.
– Jimi
Nov 10 at 20:35
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02
As you can see,
Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.– Jimi
Nov 10 at 19:40
As you can see,
Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.– Jimi
Nov 10 at 19:40
As you also noticed,
KeyPressEventArgs
returns an already processed value for the letters Keys
, including the lowercase representation.– Jimi
Nov 10 at 20:35
As you also noticed,
KeyPressEventArgs
returns an already processed value for the letters Keys
, including the lowercase representation.– Jimi
Nov 10 at 20:35
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
The reason for this is that KeyEventArgs
returns details of a key, not a character. (The capital and lowercase letters use the same key.)
Some keys don't produce characters (e.g. Shift, F-keys), some produce non-printable characters (e.g. Tab, Escape) and the character produced by a certain keystroke depends on on your keyboard layout.
If you want the character, you should probably be using the KeyPress
rather than KeyDown
event.
But if you want to do it:
As @PrinceOfRavens says, you'd have to check the state of the Caps Lock key.
You can do it like this, but this still doesn't handle keys other than letters, and doesn't handle Alt Gr (for accented letters). To fully convert a keystroke to a character, you'd have to convert both Keys.Add
(numeric keypad '+') and Shift '=' on most keyboards to "+", and Shift '1' usually to "!" (depending on your keyboard layout).
You could extend it by replacing the return null
in KeyToLetter
, but this is NOT the recommended way to get a character typed.
(I've added two items to the output.)
using System.Runtime.InteropServices; // for GetKeyState
...
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}n" +
$"CapsLock: {(CapsLockState ? "Yes" : "No")}n" +
$"Letter: {KeyToLetter(e.KeyData, CapsLockState)}";
}
// Import the DLL to use a Win32 API call.
// See https://docs.microsoft.com/en-us/windows/desktop/api/winuser/nf-winuser-getkeystate
// If using WPF, you could use Keyboard.IsKeyDown :
// https://docs.microsoft.com/en-us/dotnet/api/system.windows.input.keyboard.iskeydown?view=netframework-4.7.2
[DllImport("user32.dll")]
public static extern short GetKeyState(Keys nVirtKey);
/// <summary>
/// true if Caps Lock is on, otherwise false.
/// </summary>
public bool CapsLockState
=> (GetKeyState(Keys.CapsLock) & 1) == 1;
/// <summary/>
/// <param name="key">A base key (no modifiers).</param>
/// <returns>true if and only if the given key represents a letter.</returns>
public static bool IsLetterKey(Keys key)
=> key >= Keys.A && key <= Keys.Z;
/// <summary>
/// Given a keystroke that produces a letter, this returns the letter.
/// </summary>
/// <param name="key"></param>
/// <param name="capsLockState"></param>
/// <returns>the letter, or null if the given keystroke does not produce a letter.</returns>
public static char? KeyToLetter(Keys key, bool capsLockState)
{
Keys baseKey = key & ~Keys.Modifiers; // remove modifier keys
if (IsLetterKey(baseKey) && !key.HasFlag(Keys.Control)) // if a letter
{
bool shiftPressed = key.HasFlag(Keys.Shift); // check whether Shift was pressed
bool capital = capsLockState ^ shiftPressed; // if it should be capital
if (capital)
return (char)baseKey;
else
return Char.ToLower((char)baseKey);
}
else
{
return null; // not a letter
}
}
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-MansouriKeyPress
returns the character typed, not the key, so it doesn't provide key codes.
– John B. Lambe
Nov 11 at 15:45
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
The reason for this is that KeyEventArgs
returns details of a key, not a character. (The capital and lowercase letters use the same key.)
Some keys don't produce characters (e.g. Shift, F-keys), some produce non-printable characters (e.g. Tab, Escape) and the character produced by a certain keystroke depends on on your keyboard layout.
If you want the character, you should probably be using the KeyPress
rather than KeyDown
event.
But if you want to do it:
As @PrinceOfRavens says, you'd have to check the state of the Caps Lock key.
You can do it like this, but this still doesn't handle keys other than letters, and doesn't handle Alt Gr (for accented letters). To fully convert a keystroke to a character, you'd have to convert both Keys.Add
(numeric keypad '+') and Shift '=' on most keyboards to "+", and Shift '1' usually to "!" (depending on your keyboard layout).
You could extend it by replacing the return null
in KeyToLetter
, but this is NOT the recommended way to get a character typed.
(I've added two items to the output.)
using System.Runtime.InteropServices; // for GetKeyState
...
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}n" +
$"CapsLock: {(CapsLockState ? "Yes" : "No")}n" +
$"Letter: {KeyToLetter(e.KeyData, CapsLockState)}";
}
// Import the DLL to use a Win32 API call.
// See https://docs.microsoft.com/en-us/windows/desktop/api/winuser/nf-winuser-getkeystate
// If using WPF, you could use Keyboard.IsKeyDown :
// https://docs.microsoft.com/en-us/dotnet/api/system.windows.input.keyboard.iskeydown?view=netframework-4.7.2
[DllImport("user32.dll")]
public static extern short GetKeyState(Keys nVirtKey);
/// <summary>
/// true if Caps Lock is on, otherwise false.
/// </summary>
public bool CapsLockState
=> (GetKeyState(Keys.CapsLock) & 1) == 1;
/// <summary/>
/// <param name="key">A base key (no modifiers).</param>
/// <returns>true if and only if the given key represents a letter.</returns>
public static bool IsLetterKey(Keys key)
=> key >= Keys.A && key <= Keys.Z;
/// <summary>
/// Given a keystroke that produces a letter, this returns the letter.
/// </summary>
/// <param name="key"></param>
/// <param name="capsLockState"></param>
/// <returns>the letter, or null if the given keystroke does not produce a letter.</returns>
public static char? KeyToLetter(Keys key, bool capsLockState)
{
Keys baseKey = key & ~Keys.Modifiers; // remove modifier keys
if (IsLetterKey(baseKey) && !key.HasFlag(Keys.Control)) // if a letter
{
bool shiftPressed = key.HasFlag(Keys.Shift); // check whether Shift was pressed
bool capital = capsLockState ^ shiftPressed; // if it should be capital
if (capital)
return (char)baseKey;
else
return Char.ToLower((char)baseKey);
}
else
{
return null; // not a letter
}
}
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-MansouriKeyPress
returns the character typed, not the key, so it doesn't provide key codes.
– John B. Lambe
Nov 11 at 15:45
add a comment |
up vote
1
down vote
The reason for this is that KeyEventArgs
returns details of a key, not a character. (The capital and lowercase letters use the same key.)
Some keys don't produce characters (e.g. Shift, F-keys), some produce non-printable characters (e.g. Tab, Escape) and the character produced by a certain keystroke depends on on your keyboard layout.
If you want the character, you should probably be using the KeyPress
rather than KeyDown
event.
But if you want to do it:
As @PrinceOfRavens says, you'd have to check the state of the Caps Lock key.
You can do it like this, but this still doesn't handle keys other than letters, and doesn't handle Alt Gr (for accented letters). To fully convert a keystroke to a character, you'd have to convert both Keys.Add
(numeric keypad '+') and Shift '=' on most keyboards to "+", and Shift '1' usually to "!" (depending on your keyboard layout).
You could extend it by replacing the return null
in KeyToLetter
, but this is NOT the recommended way to get a character typed.
(I've added two items to the output.)
using System.Runtime.InteropServices; // for GetKeyState
...
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}n" +
$"CapsLock: {(CapsLockState ? "Yes" : "No")}n" +
$"Letter: {KeyToLetter(e.KeyData, CapsLockState)}";
}
// Import the DLL to use a Win32 API call.
// See https://docs.microsoft.com/en-us/windows/desktop/api/winuser/nf-winuser-getkeystate
// If using WPF, you could use Keyboard.IsKeyDown :
// https://docs.microsoft.com/en-us/dotnet/api/system.windows.input.keyboard.iskeydown?view=netframework-4.7.2
[DllImport("user32.dll")]
public static extern short GetKeyState(Keys nVirtKey);
/// <summary>
/// true if Caps Lock is on, otherwise false.
/// </summary>
public bool CapsLockState
=> (GetKeyState(Keys.CapsLock) & 1) == 1;
/// <summary/>
/// <param name="key">A base key (no modifiers).</param>
/// <returns>true if and only if the given key represents a letter.</returns>
public static bool IsLetterKey(Keys key)
=> key >= Keys.A && key <= Keys.Z;
/// <summary>
/// Given a keystroke that produces a letter, this returns the letter.
/// </summary>
/// <param name="key"></param>
/// <param name="capsLockState"></param>
/// <returns>the letter, or null if the given keystroke does not produce a letter.</returns>
public static char? KeyToLetter(Keys key, bool capsLockState)
{
Keys baseKey = key & ~Keys.Modifiers; // remove modifier keys
if (IsLetterKey(baseKey) && !key.HasFlag(Keys.Control)) // if a letter
{
bool shiftPressed = key.HasFlag(Keys.Shift); // check whether Shift was pressed
bool capital = capsLockState ^ shiftPressed; // if it should be capital
if (capital)
return (char)baseKey;
else
return Char.ToLower((char)baseKey);
}
else
{
return null; // not a letter
}
}
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-MansouriKeyPress
returns the character typed, not the key, so it doesn't provide key codes.
– John B. Lambe
Nov 11 at 15:45
add a comment |
up vote
1
down vote
up vote
1
down vote
The reason for this is that KeyEventArgs
returns details of a key, not a character. (The capital and lowercase letters use the same key.)
Some keys don't produce characters (e.g. Shift, F-keys), some produce non-printable characters (e.g. Tab, Escape) and the character produced by a certain keystroke depends on on your keyboard layout.
If you want the character, you should probably be using the KeyPress
rather than KeyDown
event.
But if you want to do it:
As @PrinceOfRavens says, you'd have to check the state of the Caps Lock key.
You can do it like this, but this still doesn't handle keys other than letters, and doesn't handle Alt Gr (for accented letters). To fully convert a keystroke to a character, you'd have to convert both Keys.Add
(numeric keypad '+') and Shift '=' on most keyboards to "+", and Shift '1' usually to "!" (depending on your keyboard layout).
You could extend it by replacing the return null
in KeyToLetter
, but this is NOT the recommended way to get a character typed.
(I've added two items to the output.)
using System.Runtime.InteropServices; // for GetKeyState
...
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}n" +
$"CapsLock: {(CapsLockState ? "Yes" : "No")}n" +
$"Letter: {KeyToLetter(e.KeyData, CapsLockState)}";
}
// Import the DLL to use a Win32 API call.
// See https://docs.microsoft.com/en-us/windows/desktop/api/winuser/nf-winuser-getkeystate
// If using WPF, you could use Keyboard.IsKeyDown :
// https://docs.microsoft.com/en-us/dotnet/api/system.windows.input.keyboard.iskeydown?view=netframework-4.7.2
[DllImport("user32.dll")]
public static extern short GetKeyState(Keys nVirtKey);
/// <summary>
/// true if Caps Lock is on, otherwise false.
/// </summary>
public bool CapsLockState
=> (GetKeyState(Keys.CapsLock) & 1) == 1;
/// <summary/>
/// <param name="key">A base key (no modifiers).</param>
/// <returns>true if and only if the given key represents a letter.</returns>
public static bool IsLetterKey(Keys key)
=> key >= Keys.A && key <= Keys.Z;
/// <summary>
/// Given a keystroke that produces a letter, this returns the letter.
/// </summary>
/// <param name="key"></param>
/// <param name="capsLockState"></param>
/// <returns>the letter, or null if the given keystroke does not produce a letter.</returns>
public static char? KeyToLetter(Keys key, bool capsLockState)
{
Keys baseKey = key & ~Keys.Modifiers; // remove modifier keys
if (IsLetterKey(baseKey) && !key.HasFlag(Keys.Control)) // if a letter
{
bool shiftPressed = key.HasFlag(Keys.Shift); // check whether Shift was pressed
bool capital = capsLockState ^ shiftPressed; // if it should be capital
if (capital)
return (char)baseKey;
else
return Char.ToLower((char)baseKey);
}
else
{
return null; // not a letter
}
}
The reason for this is that KeyEventArgs
returns details of a key, not a character. (The capital and lowercase letters use the same key.)
Some keys don't produce characters (e.g. Shift, F-keys), some produce non-printable characters (e.g. Tab, Escape) and the character produced by a certain keystroke depends on on your keyboard layout.
If you want the character, you should probably be using the KeyPress
rather than KeyDown
event.
But if you want to do it:
As @PrinceOfRavens says, you'd have to check the state of the Caps Lock key.
You can do it like this, but this still doesn't handle keys other than letters, and doesn't handle Alt Gr (for accented letters). To fully convert a keystroke to a character, you'd have to convert both Keys.Add
(numeric keypad '+') and Shift '=' on most keyboards to "+", and Shift '1' usually to "!" (depending on your keyboard layout).
You could extend it by replacing the return null
in KeyToLetter
, but this is NOT the recommended way to get a character typed.
(I've added two items to the output.)
using System.Runtime.InteropServices; // for GetKeyState
...
private void keyDemo_KeyDown(object sender, KeyEventArgs e)
{
keyInfoLabel.Text =
$"Alt: {(e.Alt ? "Yes" : "No")}n" +
$"Shift: {(e.Shift ? "Yes" : "No")}n" +
$"Ctrl: {(e.Control ? "Yes" : "No")}n" +
$"KeyCode: {e.KeyCode}n" +
$"KeyData: {e.KeyData}n" +
$"KeyValue: {e.KeyValue}n" +
$"CapsLock: {(CapsLockState ? "Yes" : "No")}n" +
$"Letter: {KeyToLetter(e.KeyData, CapsLockState)}";
}
// Import the DLL to use a Win32 API call.
// See https://docs.microsoft.com/en-us/windows/desktop/api/winuser/nf-winuser-getkeystate
// If using WPF, you could use Keyboard.IsKeyDown :
// https://docs.microsoft.com/en-us/dotnet/api/system.windows.input.keyboard.iskeydown?view=netframework-4.7.2
[DllImport("user32.dll")]
public static extern short GetKeyState(Keys nVirtKey);
/// <summary>
/// true if Caps Lock is on, otherwise false.
/// </summary>
public bool CapsLockState
=> (GetKeyState(Keys.CapsLock) & 1) == 1;
/// <summary/>
/// <param name="key">A base key (no modifiers).</param>
/// <returns>true if and only if the given key represents a letter.</returns>
public static bool IsLetterKey(Keys key)
=> key >= Keys.A && key <= Keys.Z;
/// <summary>
/// Given a keystroke that produces a letter, this returns the letter.
/// </summary>
/// <param name="key"></param>
/// <param name="capsLockState"></param>
/// <returns>the letter, or null if the given keystroke does not produce a letter.</returns>
public static char? KeyToLetter(Keys key, bool capsLockState)
{
Keys baseKey = key & ~Keys.Modifiers; // remove modifier keys
if (IsLetterKey(baseKey) && !key.HasFlag(Keys.Control)) // if a letter
{
bool shiftPressed = key.HasFlag(Keys.Shift); // check whether Shift was pressed
bool capital = capsLockState ^ shiftPressed; // if it should be capital
if (capital)
return (char)baseKey;
else
return Char.ToLower((char)baseKey);
}
else
{
return null; // not a letter
}
}
edited Nov 10 at 20:44
answered Nov 10 at 20:06
John B. Lambe
36739
36739
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-MansouriKeyPress
returns the character typed, not the key, so it doesn't provide key codes.
– John B. Lambe
Nov 11 at 15:45
add a comment |
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-MansouriKeyPress
returns the character typed, not the key, so it doesn't provide key codes.
– John B. Lambe
Nov 11 at 15:45
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
Thanks for your replay, but is your solutions includes KeyCode, KeyData ? Cuz they're not showing in KeyPress event except KeyChar.
– Hashim Al-Mansouri
Nov 11 at 14:34
@HashimAl-Mansouri
KeyPress
returns the character typed, not the key, so it doesn't provide key codes.– John B. Lambe
Nov 11 at 15:45
@HashimAl-Mansouri
KeyPress
returns the character typed, not the key, so it doesn't provide key codes.– John B. Lambe
Nov 11 at 15:45
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241551%2fhow-can-i-display-keycode-and-keydata-for-lowercase-letters-in-c-sharp-winforms%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
smn hOanFztq7jn7Bd wE,PmwlnXxy,o3ohOpTHO7d H02ambpXpsGkFvy7L
As you can see,
Keys.[Key]
doesn't have lowercase letters. The Upper/Lower case is determined by the state of Shift and/or CapsLock.– Jimi
Nov 10 at 19:40
As you also noticed,
KeyPressEventArgs
returns an already processed value for the lettersKeys
, including the lowercase representation.– Jimi
Nov 10 at 20:35
The KeyDown and KeyUp events provide the virtual keycode. it is the same anywhere in the world and matches the Keys enum type. What character they produce, if any, depends on the active keyboard layout and the state of the modifier keys. Like Shift, Ctrl, Alt. Extra complications for keyboards that have an AltGr key. You know what that character is going to be, the KeyPress event delivers it.
– Hans Passant
Nov 10 at 21:02