Compress a folder into separated parts ( no need to be all parts together to extract)
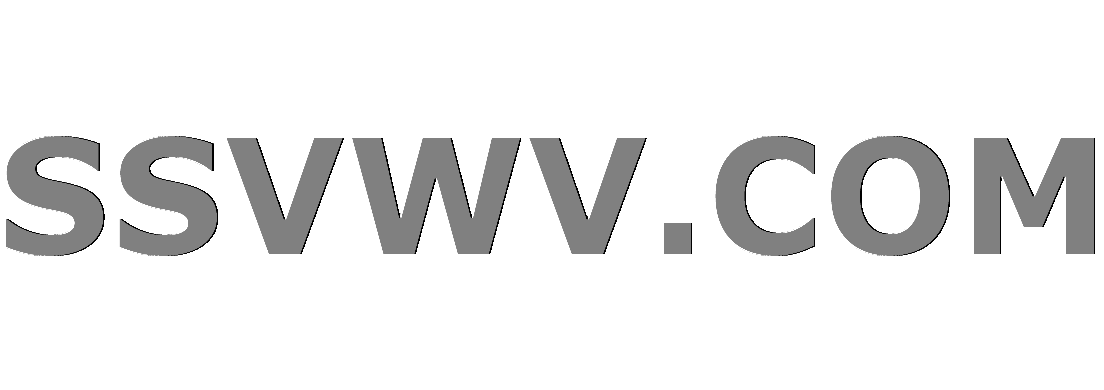
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
In my application I can compress a folder and its contents,
this content is the result of manipulation of pdf, jpg and xls. However
I needed to split it into pieces (the compressed file can not be
greater than for example 10 MB), but in such a way that a part can be
extracted without the need of the other. I can also with another
class compact in parts but with the need of all parts
together to be able to extract. This class is the one I use to create a single file.
The question is how to achieve to zip the folder into parts that can be later extracted wiithout the need of the other parts.My code so far can just zip the folder into one single part.
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipCompress {
public static void compress(String dirPath, String zipName,String path) {
Path sourceDir = Paths.get(dirPath);
String zipFileName = path+zipName.concat(".zip");
try {
ZipOutputStream outputStream = new ZipOutputStream(new FileOutputStream(zipFileName));
Files.walkFileTree(sourceDir, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attributes) {
try {
Path targetFile = sourceDir.relativize(file);
outputStream.putNextEntry(new ZipEntry(targetFile.toString()));
byte bytes = Files.readAllBytes(file);
outputStream.write(bytes, 0, bytes.length);
outputStream.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
return FileVisitResult.CONTINUE;
}
});
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Update:
Solved to list the files in the folder (folder containing the files I want to compress), after this create a new folder and move the files until the accumulated size is "5 MB", each time the accumulated size of the files is larger than "5 mb" create a new folder and move the files to this new folder.
I am sure my codes can be improved, so i accept suggestions.
public void listNovo() throws IOException {
File directory = new File("D:\Faturas\Gerados\");
String pasta = "\Pasta";
long length = 0;
long fileSizeInBytes = 0;
long fileSizeInMB = 0;
long fileSizeInKB = 0;
int count = 0;
File files = directory.listFiles();
for (File file : directory.listFiles()) {
String fileName1 = file.getName();
if (file.isFile()) {
length += file.length();
System.out.println("file:" + file.getName());
fileSizeInBytes = length;
fileSizeInKB = fileSizeInBytes / 1024;
fileSizeInMB = fileSizeInKB / 1024;
System.out.println("fileSizeInMB:" + fileSizeInMB);
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
if (fileSizeInMB >= 5) {
fileSizeInMB = 0;
length=0;
count++;
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
}
}//fim isFile
}//fim directoryListfile
}
java zip
add a comment |
In my application I can compress a folder and its contents,
this content is the result of manipulation of pdf, jpg and xls. However
I needed to split it into pieces (the compressed file can not be
greater than for example 10 MB), but in such a way that a part can be
extracted without the need of the other. I can also with another
class compact in parts but with the need of all parts
together to be able to extract. This class is the one I use to create a single file.
The question is how to achieve to zip the folder into parts that can be later extracted wiithout the need of the other parts.My code so far can just zip the folder into one single part.
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipCompress {
public static void compress(String dirPath, String zipName,String path) {
Path sourceDir = Paths.get(dirPath);
String zipFileName = path+zipName.concat(".zip");
try {
ZipOutputStream outputStream = new ZipOutputStream(new FileOutputStream(zipFileName));
Files.walkFileTree(sourceDir, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attributes) {
try {
Path targetFile = sourceDir.relativize(file);
outputStream.putNextEntry(new ZipEntry(targetFile.toString()));
byte bytes = Files.readAllBytes(file);
outputStream.write(bytes, 0, bytes.length);
outputStream.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
return FileVisitResult.CONTINUE;
}
});
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Update:
Solved to list the files in the folder (folder containing the files I want to compress), after this create a new folder and move the files until the accumulated size is "5 MB", each time the accumulated size of the files is larger than "5 mb" create a new folder and move the files to this new folder.
I am sure my codes can be improved, so i accept suggestions.
public void listNovo() throws IOException {
File directory = new File("D:\Faturas\Gerados\");
String pasta = "\Pasta";
long length = 0;
long fileSizeInBytes = 0;
long fileSizeInMB = 0;
long fileSizeInKB = 0;
int count = 0;
File files = directory.listFiles();
for (File file : directory.listFiles()) {
String fileName1 = file.getName();
if (file.isFile()) {
length += file.length();
System.out.println("file:" + file.getName());
fileSizeInBytes = length;
fileSizeInKB = fileSizeInBytes / 1024;
fileSizeInMB = fileSizeInKB / 1024;
System.out.println("fileSizeInMB:" + fileSizeInMB);
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
if (fileSizeInMB >= 5) {
fileSizeInMB = 0;
length=0;
count++;
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
}
}//fim isFile
}//fim directoryListfile
}
java zip
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
1
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57
add a comment |
In my application I can compress a folder and its contents,
this content is the result of manipulation of pdf, jpg and xls. However
I needed to split it into pieces (the compressed file can not be
greater than for example 10 MB), but in such a way that a part can be
extracted without the need of the other. I can also with another
class compact in parts but with the need of all parts
together to be able to extract. This class is the one I use to create a single file.
The question is how to achieve to zip the folder into parts that can be later extracted wiithout the need of the other parts.My code so far can just zip the folder into one single part.
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipCompress {
public static void compress(String dirPath, String zipName,String path) {
Path sourceDir = Paths.get(dirPath);
String zipFileName = path+zipName.concat(".zip");
try {
ZipOutputStream outputStream = new ZipOutputStream(new FileOutputStream(zipFileName));
Files.walkFileTree(sourceDir, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attributes) {
try {
Path targetFile = sourceDir.relativize(file);
outputStream.putNextEntry(new ZipEntry(targetFile.toString()));
byte bytes = Files.readAllBytes(file);
outputStream.write(bytes, 0, bytes.length);
outputStream.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
return FileVisitResult.CONTINUE;
}
});
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Update:
Solved to list the files in the folder (folder containing the files I want to compress), after this create a new folder and move the files until the accumulated size is "5 MB", each time the accumulated size of the files is larger than "5 mb" create a new folder and move the files to this new folder.
I am sure my codes can be improved, so i accept suggestions.
public void listNovo() throws IOException {
File directory = new File("D:\Faturas\Gerados\");
String pasta = "\Pasta";
long length = 0;
long fileSizeInBytes = 0;
long fileSizeInMB = 0;
long fileSizeInKB = 0;
int count = 0;
File files = directory.listFiles();
for (File file : directory.listFiles()) {
String fileName1 = file.getName();
if (file.isFile()) {
length += file.length();
System.out.println("file:" + file.getName());
fileSizeInBytes = length;
fileSizeInKB = fileSizeInBytes / 1024;
fileSizeInMB = fileSizeInKB / 1024;
System.out.println("fileSizeInMB:" + fileSizeInMB);
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
if (fileSizeInMB >= 5) {
fileSizeInMB = 0;
length=0;
count++;
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
}
}//fim isFile
}//fim directoryListfile
}
java zip
In my application I can compress a folder and its contents,
this content is the result of manipulation of pdf, jpg and xls. However
I needed to split it into pieces (the compressed file can not be
greater than for example 10 MB), but in such a way that a part can be
extracted without the need of the other. I can also with another
class compact in parts but with the need of all parts
together to be able to extract. This class is the one I use to create a single file.
The question is how to achieve to zip the folder into parts that can be later extracted wiithout the need of the other parts.My code so far can just zip the folder into one single part.
import java.io.FileOutputStream;
import java.io.IOException;
import java.nio.file.*;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipCompress {
public static void compress(String dirPath, String zipName,String path) {
Path sourceDir = Paths.get(dirPath);
String zipFileName = path+zipName.concat(".zip");
try {
ZipOutputStream outputStream = new ZipOutputStream(new FileOutputStream(zipFileName));
Files.walkFileTree(sourceDir, new SimpleFileVisitor<Path>() {
@Override
public FileVisitResult visitFile(Path file, BasicFileAttributes attributes) {
try {
Path targetFile = sourceDir.relativize(file);
outputStream.putNextEntry(new ZipEntry(targetFile.toString()));
byte bytes = Files.readAllBytes(file);
outputStream.write(bytes, 0, bytes.length);
outputStream.closeEntry();
} catch (IOException e) {
e.printStackTrace();
}
return FileVisitResult.CONTINUE;
}
});
outputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Update:
Solved to list the files in the folder (folder containing the files I want to compress), after this create a new folder and move the files until the accumulated size is "5 MB", each time the accumulated size of the files is larger than "5 mb" create a new folder and move the files to this new folder.
I am sure my codes can be improved, so i accept suggestions.
public void listNovo() throws IOException {
File directory = new File("D:\Faturas\Gerados\");
String pasta = "\Pasta";
long length = 0;
long fileSizeInBytes = 0;
long fileSizeInMB = 0;
long fileSizeInKB = 0;
int count = 0;
File files = directory.listFiles();
for (File file : directory.listFiles()) {
String fileName1 = file.getName();
if (file.isFile()) {
length += file.length();
System.out.println("file:" + file.getName());
fileSizeInBytes = length;
fileSizeInKB = fileSizeInBytes / 1024;
fileSizeInMB = fileSizeInKB / 1024;
System.out.println("fileSizeInMB:" + fileSizeInMB);
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
if (fileSizeInMB >= 5) {
fileSizeInMB = 0;
length=0;
count++;
if (fileSizeInMB < 5) {
File diretorio = new File(jTCaminho.getText() + pasta + count);
if (!diretorio.exists()) {
new File(jTCaminho.getText() + pasta + count).mkdir();
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
else if (diretorio.exists()) {
Files.move(file.toPath(), Paths.get(diretorio.toString(), fileName1), REPLACE_EXISTING);
length += fileSizeInMB;
}
}
}
}//fim isFile
}//fim directoryListfile
}
java zip
java zip
edited Nov 17 '18 at 15:13
Rodrigo
asked Nov 16 '18 at 16:04
RodrigoRodrigo
2328
2328
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
1
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57
add a comment |
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
1
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
1
1
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53341478%2fcompress-a-folder-into-separated-parts-no-need-to-be-all-parts-together-to-ext%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53341478%2fcompress-a-folder-into-separated-parts-no-need-to-be-all-parts-together-to-ext%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ISFV1R,LS5Or,fTmlIhMJoN k9lj0,fczvVwJSIHVJs91dw
What is your question? Does the code not work, what specifically does not work, does it throw an exception or behaves unexpected in a different way?
– luk2302
Nov 16 '18 at 16:06
Question updated.
– Rodrigo
Nov 16 '18 at 16:09
1
you need to write the logic to split the files into groups of files that are at most 10mb big and zip each one independently.
– luk2302
Nov 16 '18 at 16:13
Working on it, as soon as i can will add the code here, emaybe not fully working
– Rodrigo
Nov 16 '18 at 23:30
Code updated on que question
– Rodrigo
Nov 17 '18 at 15:57