SQLite query not creating a table; no errors generated
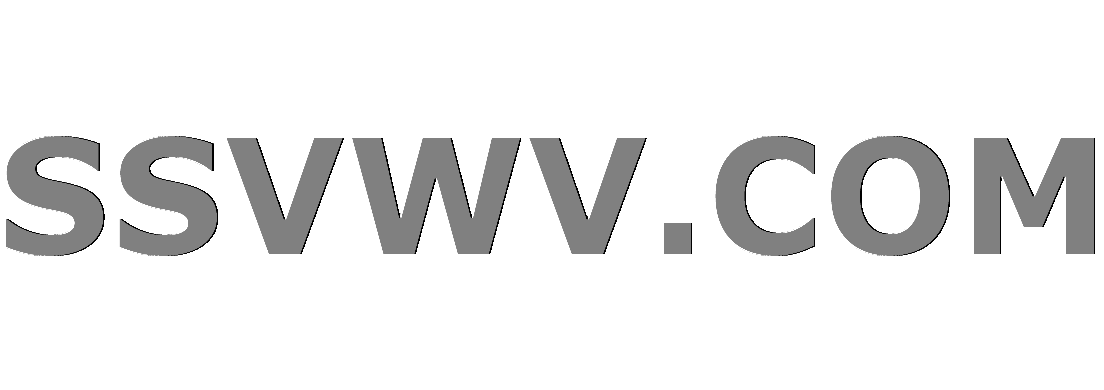
Multi tool use
I am trying to create a table using SQLite however the Create Table query does not seem to be working. When i open the .db file in an online viewer there is no data/table in the file (attached). I am not getting any errors when debugging; the issue. I have been deleting old .db files and making sure the onCreate code is running.
https://wsi.li/OzOSD2tTsB1qua/
Database Helper Code below:
package com.example.lewis.food;
import android.content.Context;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
java

add a comment |
I am trying to create a table using SQLite however the Create Table query does not seem to be working. When i open the .db file in an online viewer there is no data/table in the file (attached). I am not getting any errors when debugging; the issue. I have been deleting old .db files and making sure the onCreate code is running.
https://wsi.li/OzOSD2tTsB1qua/
Database Helper Code below:
package com.example.lewis.food;
import android.content.Context;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
java

add a comment |
I am trying to create a table using SQLite however the Create Table query does not seem to be working. When i open the .db file in an online viewer there is no data/table in the file (attached). I am not getting any errors when debugging; the issue. I have been deleting old .db files and making sure the onCreate code is running.
https://wsi.li/OzOSD2tTsB1qua/
Database Helper Code below:
package com.example.lewis.food;
import android.content.Context;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
java

I am trying to create a table using SQLite however the Create Table query does not seem to be working. When i open the .db file in an online viewer there is no data/table in the file (attached). I am not getting any errors when debugging; the issue. I have been deleting old .db files and making sure the onCreate code is running.
https://wsi.li/OzOSD2tTsB1qua/
Database Helper Code below:
package com.example.lewis.food;
import android.content.Context;
import android.database.SQLException;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
java

java

asked Nov 13 '18 at 23:43
L StubbsL Stubbs
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Issue 1.
The onUpgrade method is part of the onCreate method move it to be out side the onCreate method. e.g. :-
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
}
@Override
public void onUpgrade (SQLiteDatabase db,int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
- Note this may well just be that you dropped in the wrong code, as the code you have shown would very likely not compile.
Issue 2.
You are likely looking in the wrong place for the database/table.
The following code as the invoking activity (MainActivity used in the code) will confirm that the database is being created as well as the table, it will also print out the location of the database :-
public class MainActivity extends AppCompatActivity {
DatabaseHelper mDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDBHlpr = new DatabaseHelper(this);
Cursor csr = mDBHlpr.getReadableDatabase().query("sqlite_master",null,null,null,null,null,null);
Log.d("DBLOCATION",this.getDatabasePath(DatabaseHelper.DATABASE_NAME).getAbsolutePath());
while (csr.moveToNext()) {
Log.d(
"DBINFO",
"Found a " + csr.getString(csr.getColumnIndex("type")) +
" named " + csr.getString(csr.getColumnIndex("name"))
);
}
csr.close();
}
}
When run (using only the above code) the result is :-
11-14 00:33:23.565 1246-1246/? D/DBLOCATION: /data/data/so53291104.so53291104/databases/Food.db
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named android_metadata
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named food_table
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a index named sqlite_autoindex_food_table_1
That is the above code creates the database, the table and the index on the food column (unless it already exists) and then logs some information about the actual database.
Note the DBLOCATION will depend upon your package and will not be the same as above.
You may wish to delete the App's data or uninstall the App before running any amended code.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291104%2fsqlite-query-not-creating-a-table-no-errors-generated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Issue 1.
The onUpgrade method is part of the onCreate method move it to be out side the onCreate method. e.g. :-
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
}
@Override
public void onUpgrade (SQLiteDatabase db,int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
- Note this may well just be that you dropped in the wrong code, as the code you have shown would very likely not compile.
Issue 2.
You are likely looking in the wrong place for the database/table.
The following code as the invoking activity (MainActivity used in the code) will confirm that the database is being created as well as the table, it will also print out the location of the database :-
public class MainActivity extends AppCompatActivity {
DatabaseHelper mDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDBHlpr = new DatabaseHelper(this);
Cursor csr = mDBHlpr.getReadableDatabase().query("sqlite_master",null,null,null,null,null,null);
Log.d("DBLOCATION",this.getDatabasePath(DatabaseHelper.DATABASE_NAME).getAbsolutePath());
while (csr.moveToNext()) {
Log.d(
"DBINFO",
"Found a " + csr.getString(csr.getColumnIndex("type")) +
" named " + csr.getString(csr.getColumnIndex("name"))
);
}
csr.close();
}
}
When run (using only the above code) the result is :-
11-14 00:33:23.565 1246-1246/? D/DBLOCATION: /data/data/so53291104.so53291104/databases/Food.db
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named android_metadata
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named food_table
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a index named sqlite_autoindex_food_table_1
That is the above code creates the database, the table and the index on the food column (unless it already exists) and then logs some information about the actual database.
Note the DBLOCATION will depend upon your package and will not be the same as above.
You may wish to delete the App's data or uninstall the App before running any amended code.
add a comment |
Issue 1.
The onUpgrade method is part of the onCreate method move it to be out side the onCreate method. e.g. :-
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
}
@Override
public void onUpgrade (SQLiteDatabase db,int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
- Note this may well just be that you dropped in the wrong code, as the code you have shown would very likely not compile.
Issue 2.
You are likely looking in the wrong place for the database/table.
The following code as the invoking activity (MainActivity used in the code) will confirm that the database is being created as well as the table, it will also print out the location of the database :-
public class MainActivity extends AppCompatActivity {
DatabaseHelper mDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDBHlpr = new DatabaseHelper(this);
Cursor csr = mDBHlpr.getReadableDatabase().query("sqlite_master",null,null,null,null,null,null);
Log.d("DBLOCATION",this.getDatabasePath(DatabaseHelper.DATABASE_NAME).getAbsolutePath());
while (csr.moveToNext()) {
Log.d(
"DBINFO",
"Found a " + csr.getString(csr.getColumnIndex("type")) +
" named " + csr.getString(csr.getColumnIndex("name"))
);
}
csr.close();
}
}
When run (using only the above code) the result is :-
11-14 00:33:23.565 1246-1246/? D/DBLOCATION: /data/data/so53291104.so53291104/databases/Food.db
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named android_metadata
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named food_table
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a index named sqlite_autoindex_food_table_1
That is the above code creates the database, the table and the index on the food column (unless it already exists) and then logs some information about the actual database.
Note the DBLOCATION will depend upon your package and will not be the same as above.
You may wish to delete the App's data or uninstall the App before running any amended code.
add a comment |
Issue 1.
The onUpgrade method is part of the onCreate method move it to be out side the onCreate method. e.g. :-
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
}
@Override
public void onUpgrade (SQLiteDatabase db,int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
- Note this may well just be that you dropped in the wrong code, as the code you have shown would very likely not compile.
Issue 2.
You are likely looking in the wrong place for the database/table.
The following code as the invoking activity (MainActivity used in the code) will confirm that the database is being created as well as the table, it will also print out the location of the database :-
public class MainActivity extends AppCompatActivity {
DatabaseHelper mDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDBHlpr = new DatabaseHelper(this);
Cursor csr = mDBHlpr.getReadableDatabase().query("sqlite_master",null,null,null,null,null,null);
Log.d("DBLOCATION",this.getDatabasePath(DatabaseHelper.DATABASE_NAME).getAbsolutePath());
while (csr.moveToNext()) {
Log.d(
"DBINFO",
"Found a " + csr.getString(csr.getColumnIndex("type")) +
" named " + csr.getString(csr.getColumnIndex("name"))
);
}
csr.close();
}
}
When run (using only the above code) the result is :-
11-14 00:33:23.565 1246-1246/? D/DBLOCATION: /data/data/so53291104.so53291104/databases/Food.db
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named android_metadata
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named food_table
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a index named sqlite_autoindex_food_table_1
That is the above code creates the database, the table and the index on the food column (unless it already exists) and then logs some information about the actual database.
Note the DBLOCATION will depend upon your package and will not be the same as above.
You may wish to delete the App's data or uninstall the App before running any amended code.
Issue 1.
The onUpgrade method is part of the onCreate method move it to be out side the onCreate method. e.g. :-
public class DatabaseHelper extends SQLiteOpenHelper {
public static final String DATABASE_NAME = "Food.db";
public static final String TABLE_NAME = "food_table";
public static final String ID = "ID";
public static final String DESCRIPTION = "DESCRIPTION";
public static final String INGREDIENTS = "INGREDIENTS";
public static final String METHOD = "METHOD";
public static final String NOTES = "NOTES";
public DatabaseHelper(Context context) {
super(context, DATABASE_NAME, null, 1);
try {
SQLiteDatabase db = this.getWritableDatabase();
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public void onCreate(SQLiteDatabase db) {
db.execSQL(" CREATE TABLE " + TABLE_NAME + " (" +
ID + " TEXT PRIMARY KEY, " +
DESCRIPTION + " TEXT, " +
INGREDIENTS + " TEXT, " +
METHOD + " TEXT, " +
NOTES + " TEXT NOT NULL);"
);
}
@Override
public void onUpgrade (SQLiteDatabase db,int oldVersion, int newVersion){
db.execSQL("DROP TABLE IF EXISTS " + TABLE_NAME);
onCreate(db);
}
}
- Note this may well just be that you dropped in the wrong code, as the code you have shown would very likely not compile.
Issue 2.
You are likely looking in the wrong place for the database/table.
The following code as the invoking activity (MainActivity used in the code) will confirm that the database is being created as well as the table, it will also print out the location of the database :-
public class MainActivity extends AppCompatActivity {
DatabaseHelper mDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mDBHlpr = new DatabaseHelper(this);
Cursor csr = mDBHlpr.getReadableDatabase().query("sqlite_master",null,null,null,null,null,null);
Log.d("DBLOCATION",this.getDatabasePath(DatabaseHelper.DATABASE_NAME).getAbsolutePath());
while (csr.moveToNext()) {
Log.d(
"DBINFO",
"Found a " + csr.getString(csr.getColumnIndex("type")) +
" named " + csr.getString(csr.getColumnIndex("name"))
);
}
csr.close();
}
}
When run (using only the above code) the result is :-
11-14 00:33:23.565 1246-1246/? D/DBLOCATION: /data/data/so53291104.so53291104/databases/Food.db
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named android_metadata
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a table named food_table
11-14 00:33:23.565 1246-1246/? D/DBINFO: Found a index named sqlite_autoindex_food_table_1
That is the above code creates the database, the table and the index on the food column (unless it already exists) and then logs some information about the actual database.
Note the DBLOCATION will depend upon your package and will not be the same as above.
You may wish to delete the App's data or uninstall the App before running any amended code.
answered Nov 14 '18 at 0:36


MikeTMikeT
16k112642
16k112642
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291104%2fsqlite-query-not-creating-a-table-no-errors-generated%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xf0LNF,T5jg3G5UsQF 1yCyVy,4 MdDJ,iwZK4PRU6xhE