PHPMailer sends duplicate emails
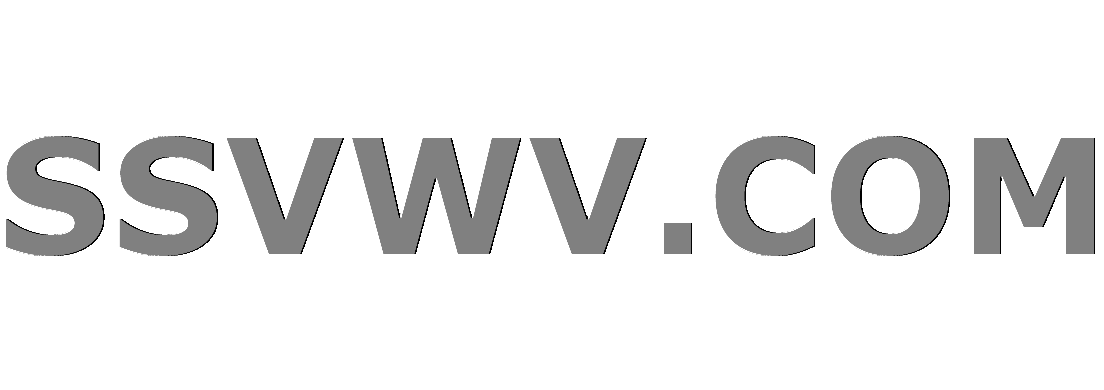
Multi tool use
PROBLEM: I'm receiving three duplicate emails for each user who signs up with their email address. My guest list is being overrun by duplicate emails, and I don't know what's wrong with my code.
Probable Cause: I have 3 signup forms, so I think somehow when I submit one signup form they all submit at the same time. The problem lies in either the Bootstrap 4.1.3 JS or the HTML.
PHP Code:
$email = $_REQUEST['email'] ;
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
exit;
}
I also have other Forms that open different PHP files, but they shouldn't be the issue here. I just added it here just in case anyone can figure something out.
FORM 1 (Same Landing Page)
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0">Get Started</button>
</div>
</form>
FORM 2 (Same Landing Page)
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" value="Get Started"/>
</div>
</form>
I'm not a JavaScript or PHP mailer expert someone please show me how to fix this problem, I think it might be a Bootstrap JS 4.13. Any suggestion or help will be greatly appreciated. Thanks!
javascript php twitter-bootstrap bootstrap-4 phpmailer
|
show 10 more comments
PROBLEM: I'm receiving three duplicate emails for each user who signs up with their email address. My guest list is being overrun by duplicate emails, and I don't know what's wrong with my code.
Probable Cause: I have 3 signup forms, so I think somehow when I submit one signup form they all submit at the same time. The problem lies in either the Bootstrap 4.1.3 JS or the HTML.
PHP Code:
$email = $_REQUEST['email'] ;
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
exit;
}
I also have other Forms that open different PHP files, but they shouldn't be the issue here. I just added it here just in case anyone can figure something out.
FORM 1 (Same Landing Page)
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0">Get Started</button>
</div>
</form>
FORM 2 (Same Landing Page)
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" value="Get Started"/>
</div>
</form>
I'm not a JavaScript or PHP mailer expert someone please show me how to fix this problem, I think it might be a Bootstrap JS 4.13. Any suggestion or help will be greatly appreciated. Thanks!
javascript php twitter-bootstrap bootstrap-4 phpmailer
2
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
1
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
1
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46
|
show 10 more comments
PROBLEM: I'm receiving three duplicate emails for each user who signs up with their email address. My guest list is being overrun by duplicate emails, and I don't know what's wrong with my code.
Probable Cause: I have 3 signup forms, so I think somehow when I submit one signup form they all submit at the same time. The problem lies in either the Bootstrap 4.1.3 JS or the HTML.
PHP Code:
$email = $_REQUEST['email'] ;
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
exit;
}
I also have other Forms that open different PHP files, but they shouldn't be the issue here. I just added it here just in case anyone can figure something out.
FORM 1 (Same Landing Page)
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0">Get Started</button>
</div>
</form>
FORM 2 (Same Landing Page)
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" value="Get Started"/>
</div>
</form>
I'm not a JavaScript or PHP mailer expert someone please show me how to fix this problem, I think it might be a Bootstrap JS 4.13. Any suggestion or help will be greatly appreciated. Thanks!
javascript php twitter-bootstrap bootstrap-4 phpmailer
PROBLEM: I'm receiving three duplicate emails for each user who signs up with their email address. My guest list is being overrun by duplicate emails, and I don't know what's wrong with my code.
Probable Cause: I have 3 signup forms, so I think somehow when I submit one signup form they all submit at the same time. The problem lies in either the Bootstrap 4.1.3 JS or the HTML.
PHP Code:
$email = $_REQUEST['email'] ;
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
exit;
}
I also have other Forms that open different PHP files, but they shouldn't be the issue here. I just added it here just in case anyone can figure something out.
FORM 1 (Same Landing Page)
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0">Get Started</button>
</div>
</form>
FORM 2 (Same Landing Page)
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" value="Get Started"/>
</div>
</form>
I'm not a JavaScript or PHP mailer expert someone please show me how to fix this problem, I think it might be a Bootstrap JS 4.13. Any suggestion or help will be greatly appreciated. Thanks!
javascript php twitter-bootstrap bootstrap-4 phpmailer
javascript php twitter-bootstrap bootstrap-4 phpmailer
edited Nov 22 '18 at 4:29
ChosenJuan
asked Nov 13 '18 at 23:39


ChosenJuanChosenJuan
3001330
3001330
2
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
1
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
1
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46
|
show 10 more comments
2
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
1
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
1
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46
2
2
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
1
1
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
1
1
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46
|
show 10 more comments
8 Answers
8
active
oldest
votes
Try creating a seperate submit()-function (with the onclick-handler) for each button and removing the value='submit'
from them, like so:
<!--signupform1-->
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started</button>
</div>
</form>
<!--signupform2-->
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();" value="Get Started"/>
</div>
</form>
And then in your js:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
</script>
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
add a comment |
First off: Bootstrap ain't the culprit here, JS neither. And if if PHP (or php mailer) are clean too, then you may want to take a look at the mail server settings (check with your host).
However, maybe the form is submitted twice? Let's check:
- open google chrome debugger (F12)
- Click on "Network", tick "Preserve log"
- Click on "Clear" (2nd icon left)
- Run your regular script (i.e. fill the form and submit)
- "Network" tab will show you any duplicate call of your PHP page
If it still fails, try this:
download https://github.com/PHPMailer/PHPMailer
create a completely blank file, directly put your PHP emailing lines in it. Remove the form, input, javascript, isset and the rest. Run this file by simply calling it, so it would look like:
require_once("PHPMailerAutoload.php"); // <---- only this will be required (put your path properly)
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again.
<p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
godd luck
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
add a comment |
I don't know which version of PHPMailer you're using but you're also using PHP's mail()
method, which is quite ironic because you're also using PHPMailer. But nevertheless, this is how I did it in one of my projects :
function sendAuthEmail($email, $token, $role) {
try {
$mail = new PHPMailer(true);
$mail->SMTPDebug = 0; //SMTP Debug
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = true;
$mail->Username = 'example@example.com';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = '587';
/**
* SMTPOptions work-around by @author : Synchro
* This setting should removed on server and
* mailing should be working on the server
*/
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
// Recipients
$mail->setFrom('no-reply@confirmation.com', 'Do Not Reply');
$mail->addAddress($email, 'Yash Karanke'); // Add a recipient
$mail->addReplyTo('no-reply@confirmation.com');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Authentication Email';
$mail->Body = 'text to send';
if ($mail->send()) {
return true;
}
else {
return false;
exit();
}
}
catch(Exception $e) {
return $mail->ErrorInfo;
}
}
If you're looking for a simpler example, remove the wrapped function and use only code which you need. More details on Docs
In your code you're using if(!$mail->send())
which I'm not sure, how it is working, but you should use if($mail->send())
which is recommended way from the docs
This code snippet is from verion 6.0.3.
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
add a comment |
I think that a deep dive into your jQuery / JS code could bring the answer, but my guess is that the button
elements in your forms trigger some event that affects the other forms.
Did you try changing all the buttons to <input type='submit'/>
?
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
add a comment |
start a session in your server and store the mail sent information
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$_SESSION['is_mail_1_send']){//check mail sended once
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
$_SESSION['is_mail_1_send']=false;
exit;
}else{
$_SESSION['is_mail_1_send']=true;
}
}
if(!$_SESSION['is_mail_2_send']){//check mail sended once
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
$_SESSION['is_mail_2_send']=false;
exit;
}else{
$_SESSION['is_mail_2_send']=true;
}
}
?>
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
add a comment |
Where you are handling form submit event? Create a separate function
for sending mail. call that function when user click on specific form
submit button. like below
// Your separate Mail sending function
sendMail(arg1, arg2, arg3){
// PHP Mailer Configurations
}
// Calling your first form
if(isset($_POST['topsubmit']){
sendMail(arg1, arg2, arg3);
}
// Calling your second form
if(isset($_POST['footersubmit']){
sendMail(arg1, arg2, arg3);
}
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
add a comment |
Did I understand it correctly? o_O
Of course, with that php code, you are sending multiple emails.
just change your php code like this, so you parse the mail1
and mail2
conditionally separated:
if (!empty($_POST['topsubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
if (!empty($_POST['footersubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
thus, all of the emails wont be sent on the same pageload.
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
add a comment |
This is the complete answer to my question, thanks to @SearchingSolutions for showing me the correct way to solve this issue.
What I did to fix this problem was to create 2 different buttons with separate onclick-handlers for each button. Each button has different ids and I added the TYPE="Submit" on both. I gave every form a different name, and added a small JS script to submit the specific form when the specific onclick handler is called.
So far, every email is coming in as intended, and everything is running smoothly again.
Thank you all for the great answers!
The HTML:
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email2" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email2" type="email" name="email2" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started"</button>
</div>
</form>
<form class="signupForm2 input-group mt-1" method="post" action="footerconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email3" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email3" type="email" name="email3" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();">Get Started"</button>
</div>
</form>
The JS Script:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291081%2fphpmailer-sends-duplicate-emails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try creating a seperate submit()-function (with the onclick-handler) for each button and removing the value='submit'
from them, like so:
<!--signupform1-->
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started</button>
</div>
</form>
<!--signupform2-->
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();" value="Get Started"/>
</div>
</form>
And then in your js:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
</script>
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
add a comment |
Try creating a seperate submit()-function (with the onclick-handler) for each button and removing the value='submit'
from them, like so:
<!--signupform1-->
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started</button>
</div>
</form>
<!--signupform2-->
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();" value="Get Started"/>
</div>
</form>
And then in your js:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
</script>
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
add a comment |
Try creating a seperate submit()-function (with the onclick-handler) for each button and removing the value='submit'
from them, like so:
<!--signupform1-->
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started</button>
</div>
</form>
<!--signupform2-->
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();" value="Get Started"/>
</div>
</form>
And then in your js:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
</script>
Try creating a seperate submit()-function (with the onclick-handler) for each button and removing the value='submit'
from them, like so:
<!--signupform1-->
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started</button>
</div>
</form>
<!--signupform2-->
<form class="signupForm2 input-group mt-1" method="post" action="bottomconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email" type="email" name="email" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<input style="font-size:17px;" name="footersubmit" type="button" class="ctabutton semibolder submitButton sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();" value="Get Started"/>
</div>
</form>
And then in your js:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
</script>
answered Nov 21 '18 at 10:13


SearchingSolutionsSearchingSolutions
13119
13119
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
add a comment |
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
This answer works best, it was the submit button that was causing the issue. However, in order to get it to work, I had to add the type="submit" to the buttons on both the forms. So far, I've been getting individual email from each form. Cheers!!! Thank you for the answer!
– ChosenJuan
Nov 22 '18 at 0:00
add a comment |
First off: Bootstrap ain't the culprit here, JS neither. And if if PHP (or php mailer) are clean too, then you may want to take a look at the mail server settings (check with your host).
However, maybe the form is submitted twice? Let's check:
- open google chrome debugger (F12)
- Click on "Network", tick "Preserve log"
- Click on "Clear" (2nd icon left)
- Run your regular script (i.e. fill the form and submit)
- "Network" tab will show you any duplicate call of your PHP page
If it still fails, try this:
download https://github.com/PHPMailer/PHPMailer
create a completely blank file, directly put your PHP emailing lines in it. Remove the form, input, javascript, isset and the rest. Run this file by simply calling it, so it would look like:
require_once("PHPMailerAutoload.php"); // <---- only this will be required (put your path properly)
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again.
<p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
godd luck
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
add a comment |
First off: Bootstrap ain't the culprit here, JS neither. And if if PHP (or php mailer) are clean too, then you may want to take a look at the mail server settings (check with your host).
However, maybe the form is submitted twice? Let's check:
- open google chrome debugger (F12)
- Click on "Network", tick "Preserve log"
- Click on "Clear" (2nd icon left)
- Run your regular script (i.e. fill the form and submit)
- "Network" tab will show you any duplicate call of your PHP page
If it still fails, try this:
download https://github.com/PHPMailer/PHPMailer
create a completely blank file, directly put your PHP emailing lines in it. Remove the form, input, javascript, isset and the rest. Run this file by simply calling it, so it would look like:
require_once("PHPMailerAutoload.php"); // <---- only this will be required (put your path properly)
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again.
<p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
godd luck
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
add a comment |
First off: Bootstrap ain't the culprit here, JS neither. And if if PHP (or php mailer) are clean too, then you may want to take a look at the mail server settings (check with your host).
However, maybe the form is submitted twice? Let's check:
- open google chrome debugger (F12)
- Click on "Network", tick "Preserve log"
- Click on "Clear" (2nd icon left)
- Run your regular script (i.e. fill the form and submit)
- "Network" tab will show you any duplicate call of your PHP page
If it still fails, try this:
download https://github.com/PHPMailer/PHPMailer
create a completely blank file, directly put your PHP emailing lines in it. Remove the form, input, javascript, isset and the rest. Run this file by simply calling it, so it would look like:
require_once("PHPMailerAutoload.php"); // <---- only this will be required (put your path properly)
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again.
<p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
godd luck
First off: Bootstrap ain't the culprit here, JS neither. And if if PHP (or php mailer) are clean too, then you may want to take a look at the mail server settings (check with your host).
However, maybe the form is submitted twice? Let's check:
- open google chrome debugger (F12)
- Click on "Network", tick "Preserve log"
- Click on "Clear" (2nd icon left)
- Run your regular script (i.e. fill the form and submit)
- "Network" tab will show you any duplicate call of your PHP page
If it still fails, try this:
download https://github.com/PHPMailer/PHPMailer
create a completely blank file, directly put your PHP emailing lines in it. Remove the form, input, javascript, isset and the rest. Run this file by simply calling it, so it would look like:
require_once("PHPMailerAutoload.php"); // <---- only this will be required (put your path properly)
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again.
<p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
exit;
}
godd luck
edited Nov 21 '18 at 10:42
answered Nov 21 '18 at 10:14
DingDongDingDong
43110
43110
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
add a comment |
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
Thanks for the debugger tutorial, it's very valuable. However, my log didn't particularly show anything wrong with the code as I submitted the email to the form. Seems like it needed a JS solution to fix it. Thanks anyway!
– ChosenJuan
Nov 22 '18 at 0:02
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
@ChosenJuan I guess most of us missed it: one should never bind a JS form submit event to an html submit button to begin with. :D
– DingDong
Nov 22 '18 at 6:37
add a comment |
I don't know which version of PHPMailer you're using but you're also using PHP's mail()
method, which is quite ironic because you're also using PHPMailer. But nevertheless, this is how I did it in one of my projects :
function sendAuthEmail($email, $token, $role) {
try {
$mail = new PHPMailer(true);
$mail->SMTPDebug = 0; //SMTP Debug
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = true;
$mail->Username = 'example@example.com';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = '587';
/**
* SMTPOptions work-around by @author : Synchro
* This setting should removed on server and
* mailing should be working on the server
*/
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
// Recipients
$mail->setFrom('no-reply@confirmation.com', 'Do Not Reply');
$mail->addAddress($email, 'Yash Karanke'); // Add a recipient
$mail->addReplyTo('no-reply@confirmation.com');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Authentication Email';
$mail->Body = 'text to send';
if ($mail->send()) {
return true;
}
else {
return false;
exit();
}
}
catch(Exception $e) {
return $mail->ErrorInfo;
}
}
If you're looking for a simpler example, remove the wrapped function and use only code which you need. More details on Docs
In your code you're using if(!$mail->send())
which I'm not sure, how it is working, but you should use if($mail->send())
which is recommended way from the docs
This code snippet is from verion 6.0.3.
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
add a comment |
I don't know which version of PHPMailer you're using but you're also using PHP's mail()
method, which is quite ironic because you're also using PHPMailer. But nevertheless, this is how I did it in one of my projects :
function sendAuthEmail($email, $token, $role) {
try {
$mail = new PHPMailer(true);
$mail->SMTPDebug = 0; //SMTP Debug
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = true;
$mail->Username = 'example@example.com';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = '587';
/**
* SMTPOptions work-around by @author : Synchro
* This setting should removed on server and
* mailing should be working on the server
*/
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
// Recipients
$mail->setFrom('no-reply@confirmation.com', 'Do Not Reply');
$mail->addAddress($email, 'Yash Karanke'); // Add a recipient
$mail->addReplyTo('no-reply@confirmation.com');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Authentication Email';
$mail->Body = 'text to send';
if ($mail->send()) {
return true;
}
else {
return false;
exit();
}
}
catch(Exception $e) {
return $mail->ErrorInfo;
}
}
If you're looking for a simpler example, remove the wrapped function and use only code which you need. More details on Docs
In your code you're using if(!$mail->send())
which I'm not sure, how it is working, but you should use if($mail->send())
which is recommended way from the docs
This code snippet is from verion 6.0.3.
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
add a comment |
I don't know which version of PHPMailer you're using but you're also using PHP's mail()
method, which is quite ironic because you're also using PHPMailer. But nevertheless, this is how I did it in one of my projects :
function sendAuthEmail($email, $token, $role) {
try {
$mail = new PHPMailer(true);
$mail->SMTPDebug = 0; //SMTP Debug
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = true;
$mail->Username = 'example@example.com';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = '587';
/**
* SMTPOptions work-around by @author : Synchro
* This setting should removed on server and
* mailing should be working on the server
*/
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
// Recipients
$mail->setFrom('no-reply@confirmation.com', 'Do Not Reply');
$mail->addAddress($email, 'Yash Karanke'); // Add a recipient
$mail->addReplyTo('no-reply@confirmation.com');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Authentication Email';
$mail->Body = 'text to send';
if ($mail->send()) {
return true;
}
else {
return false;
exit();
}
}
catch(Exception $e) {
return $mail->ErrorInfo;
}
}
If you're looking for a simpler example, remove the wrapped function and use only code which you need. More details on Docs
In your code you're using if(!$mail->send())
which I'm not sure, how it is working, but you should use if($mail->send())
which is recommended way from the docs
This code snippet is from verion 6.0.3.
I don't know which version of PHPMailer you're using but you're also using PHP's mail()
method, which is quite ironic because you're also using PHPMailer. But nevertheless, this is how I did it in one of my projects :
function sendAuthEmail($email, $token, $role) {
try {
$mail = new PHPMailer(true);
$mail->SMTPDebug = 0; //SMTP Debug
$mail->isSMTP();
$mail->Host = "smtp.gmail.com";
$mail->SMTPAuth = true;
$mail->Username = 'example@example.com';
$mail->Password = 'password';
$mail->SMTPSecure = 'tls';
$mail->Port = '587';
/**
* SMTPOptions work-around by @author : Synchro
* This setting should removed on server and
* mailing should be working on the server
*/
$mail->SMTPOptions = array(
'ssl' => array(
'verify_peer' => false,
'verify_peer_name' => false,
'allow_self_signed' => true
)
);
// Recipients
$mail->setFrom('no-reply@confirmation.com', 'Do Not Reply');
$mail->addAddress($email, 'Yash Karanke'); // Add a recipient
$mail->addReplyTo('no-reply@confirmation.com');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Authentication Email';
$mail->Body = 'text to send';
if ($mail->send()) {
return true;
}
else {
return false;
exit();
}
}
catch(Exception $e) {
return $mail->ErrorInfo;
}
}
If you're looking for a simpler example, remove the wrapped function and use only code which you need. More details on Docs
In your code you're using if(!$mail->send())
which I'm not sure, how it is working, but you should use if($mail->send())
which is recommended way from the docs
This code snippet is from verion 6.0.3.
edited Nov 14 '18 at 0:15
answered Nov 14 '18 at 0:04
user10415043
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
add a comment |
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
When I comment out mail($to, $subject, $message, $headers); it doesn't work, but I did remove the $email = $_REQUEST['email'] ; and it worked without it. However, your above solution is not working and I'm still receiving duplicate emails.
– ChosenJuan
Nov 14 '18 at 0:48
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
Please check the new updated PHPMailer code, now I think the issue has to do with jQuery submit script. Let me know what you think.
– ChosenJuan
Nov 14 '18 at 23:31
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
@ChosenJuan Yes, I checked and there is no conflict with jQuery Code.
– user10415043
Nov 15 '18 at 4:44
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
yes, I actually removed the jQuery completely, it wasn't even necessary. I think the problem is that it submits all three forms at the same time, but I have no idea how to fix this issue while keeping all three forms. Any ideas?
– ChosenJuan
Nov 15 '18 at 15:48
add a comment |
I think that a deep dive into your jQuery / JS code could bring the answer, but my guess is that the button
elements in your forms trigger some event that affects the other forms.
Did you try changing all the buttons to <input type='submit'/>
?
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
add a comment |
I think that a deep dive into your jQuery / JS code could bring the answer, but my guess is that the button
elements in your forms trigger some event that affects the other forms.
Did you try changing all the buttons to <input type='submit'/>
?
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
add a comment |
I think that a deep dive into your jQuery / JS code could bring the answer, but my guess is that the button
elements in your forms trigger some event that affects the other forms.
Did you try changing all the buttons to <input type='submit'/>
?
I think that a deep dive into your jQuery / JS code could bring the answer, but my guess is that the button
elements in your forms trigger some event that affects the other forms.
Did you try changing all the buttons to <input type='submit'/>
?
answered Nov 16 '18 at 0:45
LisLis
19911
19911
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
add a comment |
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
I tried changing the input to submit, but that isn't working either. The only JQuery code in question could be the Jquery Validation code I will post it soon
– ChosenJuan
Nov 19 '18 at 2:41
add a comment |
start a session in your server and store the mail sent information
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$_SESSION['is_mail_1_send']){//check mail sended once
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
$_SESSION['is_mail_1_send']=false;
exit;
}else{
$_SESSION['is_mail_1_send']=true;
}
}
if(!$_SESSION['is_mail_2_send']){//check mail sended once
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
$_SESSION['is_mail_2_send']=false;
exit;
}else{
$_SESSION['is_mail_2_send']=true;
}
}
?>
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
add a comment |
start a session in your server and store the mail sent information
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$_SESSION['is_mail_1_send']){//check mail sended once
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
$_SESSION['is_mail_1_send']=false;
exit;
}else{
$_SESSION['is_mail_1_send']=true;
}
}
if(!$_SESSION['is_mail_2_send']){//check mail sended once
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
$_SESSION['is_mail_2_send']=false;
exit;
}else{
$_SESSION['is_mail_2_send']=true;
}
}
?>
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
add a comment |
start a session in your server and store the mail sent information
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$_SESSION['is_mail_1_send']){//check mail sended once
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
$_SESSION['is_mail_1_send']=false;
exit;
}else{
$_SESSION['is_mail_1_send']=true;
}
}
if(!$_SESSION['is_mail_2_send']){//check mail sended once
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
$_SESSION['is_mail_2_send']=false;
exit;
}else{
$_SESSION['is_mail_2_send']=true;
}
}
?>
start a session in your server and store the mail sent information
// Import PHPMailer classes into the global namespace
// These must be at the top of your script, not inside a function
use PHPMailerPHPMailerPHPMailer;
use PHPMailerPHPMailerException;
require './PHPMailer-master/src/Exception.php';
require './PHPMailer-master/src/PHPMailer.php';
require './PHPMailer-master/src/SMTP.php';
// Popup Form Signup
$mail = new PHPMailer();
$mail->IsSMTP();
$mail->Host = "mail.domain.com";
$mail->SMTPAuth = true;
$mail->Username = "user@domain.com";
$mail->Password = "password";
$mail->SMTPSecure = 'TLS';
$mail->Port = 587;
$mail->From = $email;
$mail->setFrom('$email', 'Guest');
$mail->addAddress('admin@domain.com', 'Admin');
$mail->IsHTML(true);
$mail->Subject = "New Member!";
$mail->Body = $email;
$mail->AltBody = $email;
$mail2 = new PHPMailer();
$mail2->IsSMTP();
$mail2->Host = "mail.domain.com";
$mail2->SMTPAuth = true;
$mail2->Username = "user@domain.com";
$mail2->Password = "password";
$mail2->SMTPSecure = 'TLS';
$mail2->Port = 587;
$mail2->setFrom('support@domain.com', 'Support');
$mail2->AddAddress("$email");
$mail2->AddReplyTo('support@domain.com');
$mail2->Subject = "Thanks for signing up!";
$message = '';
$mail2->Body = $message;
$mail2->AltBody = $message;
if(!$_SESSION['is_mail_1_send']){//check mail sended once
if(!$mail2->Send())
{
echo "Message could not be sent. Please reload the page and try again. <p>";
echo "Mailer Error: " . $mail2->ErrorInfo;
$_SESSION['is_mail_1_send']=false;
exit;
}else{
$_SESSION['is_mail_1_send']=true;
}
}
if(!$_SESSION['is_mail_2_send']){//check mail sended once
if(!$mail->Send())
{
echo "Message was not received. Please reload the page and try again.<p>";
echo "Mailer Error: " . $mail->ErrorInfo;
$_SESSION['is_mail_2_send']=false;
exit;
}else{
$_SESSION['is_mail_2_send']=true;
}
}
?>
answered Nov 16 '18 at 5:20


Mahfuzar RahmanMahfuzar Rahman
14919
14919
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
add a comment |
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
This doesn't work, It still send the duplicate emails. Is this supposed to send the logs to my email?
– ChosenJuan
Nov 19 '18 at 2:43
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
how many emails you received by using my session setting i tried my code it sending only 2mails
– Mahfuzar Rahman
Nov 19 '18 at 3:39
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
It keeps sending three duplicate emails to the user from support@domain.com and three duplicate emails to my admin@domain.com
– ChosenJuan
Nov 19 '18 at 12:32
add a comment |
Where you are handling form submit event? Create a separate function
for sending mail. call that function when user click on specific form
submit button. like below
// Your separate Mail sending function
sendMail(arg1, arg2, arg3){
// PHP Mailer Configurations
}
// Calling your first form
if(isset($_POST['topsubmit']){
sendMail(arg1, arg2, arg3);
}
// Calling your second form
if(isset($_POST['footersubmit']){
sendMail(arg1, arg2, arg3);
}
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
add a comment |
Where you are handling form submit event? Create a separate function
for sending mail. call that function when user click on specific form
submit button. like below
// Your separate Mail sending function
sendMail(arg1, arg2, arg3){
// PHP Mailer Configurations
}
// Calling your first form
if(isset($_POST['topsubmit']){
sendMail(arg1, arg2, arg3);
}
// Calling your second form
if(isset($_POST['footersubmit']){
sendMail(arg1, arg2, arg3);
}
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
add a comment |
Where you are handling form submit event? Create a separate function
for sending mail. call that function when user click on specific form
submit button. like below
// Your separate Mail sending function
sendMail(arg1, arg2, arg3){
// PHP Mailer Configurations
}
// Calling your first form
if(isset($_POST['topsubmit']){
sendMail(arg1, arg2, arg3);
}
// Calling your second form
if(isset($_POST['footersubmit']){
sendMail(arg1, arg2, arg3);
}
Where you are handling form submit event? Create a separate function
for sending mail. call that function when user click on specific form
submit button. like below
// Your separate Mail sending function
sendMail(arg1, arg2, arg3){
// PHP Mailer Configurations
}
// Calling your first form
if(isset($_POST['topsubmit']){
sendMail(arg1, arg2, arg3);
}
// Calling your second form
if(isset($_POST['footersubmit']){
sendMail(arg1, arg2, arg3);
}
answered Nov 21 '18 at 0:04
Himel RanaHimel Rana
41129
41129
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
add a comment |
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
Can you elaborate on how to incorporate the above code? Should I just copy the sendmail part and place it above or should it replace something? And the second code should just replace the if if(!$mail->Send()) code? Can you please update your sample?
– ChosenJuan
Nov 21 '18 at 0:57
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
if(isset($_POST['topsubmit']){ if(!$mail->Send()) // like this should work. } // But if you send mail related full configuration to a separate function it's a lot batter.
– Himel Rana
Nov 21 '18 at 1:06
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
Can I just add this one send function replacing both if(!$mail->Send()) functions // Calling your first form if(isset($_POST['topsubmit']){ sendMail($mail, $mail2); echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail, $mail2->ErrorInfo; exit; }
– ChosenJuan
Nov 21 '18 at 2:31
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
if(isset($_POST['footersubmit']){ if(!$mail2->Send()) { echo "Message could not be sent. Please go back to voozlr.com and try again. If problem persists contact us at voozlr.com/contact. Thank you for your patience!<p>"; echo "Mailer Error: " . $mail2->ErrorInfo; exit; } it's not working. Am I doing something wrong? Or am I missing something?
– ChosenJuan
Nov 21 '18 at 3:14
add a comment |
Did I understand it correctly? o_O
Of course, with that php code, you are sending multiple emails.
just change your php code like this, so you parse the mail1
and mail2
conditionally separated:
if (!empty($_POST['topsubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
if (!empty($_POST['footersubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
thus, all of the emails wont be sent on the same pageload.
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
add a comment |
Did I understand it correctly? o_O
Of course, with that php code, you are sending multiple emails.
just change your php code like this, so you parse the mail1
and mail2
conditionally separated:
if (!empty($_POST['topsubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
if (!empty($_POST['footersubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
thus, all of the emails wont be sent on the same pageload.
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
add a comment |
Did I understand it correctly? o_O
Of course, with that php code, you are sending multiple emails.
just change your php code like this, so you parse the mail1
and mail2
conditionally separated:
if (!empty($_POST['topsubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
if (!empty($_POST['footersubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
thus, all of the emails wont be sent on the same pageload.
Did I understand it correctly? o_O
Of course, with that php code, you are sending multiple emails.
just change your php code like this, so you parse the mail1
and mail2
conditionally separated:
if (!empty($_POST['topsubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
if (!empty($_POST['footersubmit']))
{
$mail2 = new PHPMailer();
...
if(!$mail2->Send())
...
}
thus, all of the emails wont be sent on the same pageload.
answered Nov 21 '18 at 10:57


T.ToduaT.Todua
30.4k12132131
30.4k12132131
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
add a comment |
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
I'm sending two emails at the same time. One email: $mail to myself at admin.domain.com and another email: $mail2 to the user who entered their email. But what you answered is splitting the top submit and footersubmit, but I split the topsubmit and footersubmit into separate PHP files labelled topconfirm.php and footerconfirm.php both of these php files send two emails each at mail and mail2 one to the new signup and one to me. How do I set it up?
– ChosenJuan
Nov 21 '18 at 21:09
add a comment |
This is the complete answer to my question, thanks to @SearchingSolutions for showing me the correct way to solve this issue.
What I did to fix this problem was to create 2 different buttons with separate onclick-handlers for each button. Each button has different ids and I added the TYPE="Submit" on both. I gave every form a different name, and added a small JS script to submit the specific form when the specific onclick handler is called.
So far, every email is coming in as intended, and everything is running smoothly again.
Thank you all for the great answers!
The HTML:
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email2" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email2" type="email" name="email2" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started"</button>
</div>
</form>
<form class="signupForm2 input-group mt-1" method="post" action="footerconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email3" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email3" type="email" name="email3" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();">Get Started"</button>
</div>
</form>
The JS Script:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
add a comment |
This is the complete answer to my question, thanks to @SearchingSolutions for showing me the correct way to solve this issue.
What I did to fix this problem was to create 2 different buttons with separate onclick-handlers for each button. Each button has different ids and I added the TYPE="Submit" on both. I gave every form a different name, and added a small JS script to submit the specific form when the specific onclick handler is called.
So far, every email is coming in as intended, and everything is running smoothly again.
Thank you all for the great answers!
The HTML:
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email2" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email2" type="email" name="email2" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started"</button>
</div>
</form>
<form class="signupForm2 input-group mt-1" method="post" action="footerconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email3" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email3" type="email" name="email3" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();">Get Started"</button>
</div>
</form>
The JS Script:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
add a comment |
This is the complete answer to my question, thanks to @SearchingSolutions for showing me the correct way to solve this issue.
What I did to fix this problem was to create 2 different buttons with separate onclick-handlers for each button. Each button has different ids and I added the TYPE="Submit" on both. I gave every form a different name, and added a small JS script to submit the specific form when the specific onclick handler is called.
So far, every email is coming in as intended, and everything is running smoothly again.
Thank you all for the great answers!
The HTML:
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email2" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email2" type="email" name="email2" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started"</button>
</div>
</form>
<form class="signupForm2 input-group mt-1" method="post" action="footerconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email3" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email3" type="email" name="email3" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();">Get Started"</button>
</div>
</form>
The JS Script:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
This is the complete answer to my question, thanks to @SearchingSolutions for showing me the correct way to solve this issue.
What I did to fix this problem was to create 2 different buttons with separate onclick-handlers for each button. Each button has different ids and I added the TYPE="Submit" on both. I gave every form a different name, and added a small JS script to submit the specific form when the specific onclick handler is called.
So far, every email is coming in as intended, and everything is running smoothly again.
Thank you all for the great answers!
The HTML:
<form class="signupForm1 input-group mt-1" method="post" action="topconfirm">
<div class="input-group">
<label for="email2" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email2" type="email" name="email2" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="topsubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit1();">Get Started"</button>
</div>
</form>
<form class="signupForm2 input-group mt-1" method="post" action="footerconfirm">
<div class="input-group">
<label style="font-weight:normal!important;" for="email3" class="sr-only">Enter your email address</label>
<input style ="overflow:hidden;" id="email3" type="email" name="email3" required class="sentence form-control" aria-label="Large" placeholder="enter your email address">
<button style="font-size:17px;" name="footersubmit" type="submit" class="ctabutton semibolder sentence text-white btn btn-secondary px-lg-3 px-md-1 px-sm-1 btn-lg rounded-0" onclick="submit2();">Get Started"</button>
</div>
</form>
The JS Script:
<script>
function submit1() {
$(".signupForm1").submit();
}
function submit2() {
$(".signupForm2").submit();
}
answered Nov 22 '18 at 4:29


ChosenJuanChosenJuan
3001330
3001330
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291081%2fphpmailer-sends-duplicate-emails%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eiFgFgf0g1JYtET2u,Sc
2
you dont need to initialise PHPMailer twice to send 2 emails. your also using php's mail() as well as phpmailer, this is a bit of a mess to be honest.
– user10226920
Nov 13 '18 at 23:42
1
Having an input with the same name in different forms shouldn't matter. You can only submit one form at a time.
– Don't Panic
Nov 13 '18 at 23:43
whats your jquery looking like? you have same #id on the same page. what is the confirm in your action for? you should leave it empty
– comphonia
Nov 13 '18 at 23:44
The confirm triggers the confirm.php page that sends the email upon entering a valid email and hitting the submit button
– ChosenJuan
Nov 13 '18 at 23:45
1
phpmailer can be cleaned up to like half the code if you use variables and swap them out based on a if(isset($_POST['variable']))
– comphonia
Nov 13 '18 at 23:46