Why doesn't this storyboard defined in code work?
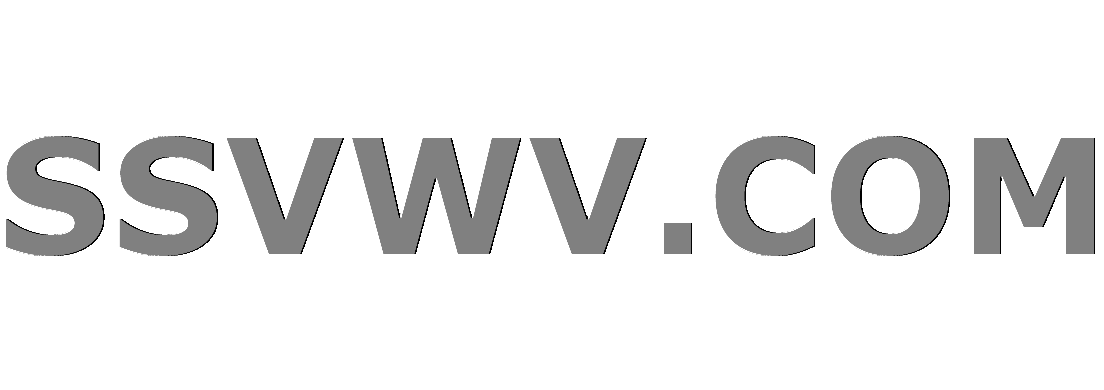
Multi tool use
I'm attempting to create an animation for a GradientBrush
that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
Because of the complex nature of this task, I've taken to handling everything in the code behind, rather than in XAML.
Fortunately, I'm getting no errors or exceptions with my code.
Unfortunately, it's also doing absolutely nothing.
In compliance with the minimal, complete and verifiable example requirements:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Animation;
namespace MCVE {
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class Program {
private static LinearGradientBrush TestBrush { get; } =
new LinearGradientBrush(
new GradientStopCollection( new[ ]{
new GradientStop( Colors.Black, 0 / 1.0D ),
new GradientStop( Colors.White, 1 / 1.0D )
} ), new Point( 0.0D, 0.0D ), new Point( 1.0D, 1.0D ) );
[STAThread]
public static int Main( ) {
Storyboard board = CreateStoryboard( TestBrush );
Window w = new Window( ){
Background = TestBrush
};
w.Loaded += new RoutedEventHandler(
( S, E ) => board.Begin( w, true ) );
Program program = new Program( );
program.InitializeComponent( );
return program.Run( w );
}
public static Storyboard CreateStoryboard( GradientBrush brush ) {
Storyboard board = new Storyboard( ){
Duration = new Duration( TimeSpan.FromSeconds( 1.0D ) ),
RepeatBehavior = RepeatBehavior.Forever
};
foreach (
var animation
in brush.GradientStops.Select(
GS => _CreateGradientStopAnimation(
GS, brush.GradientStops.SkipWhile( G => G != GS
).Concat( brush.GradientStops.TakeWhile( G => G != GS )
).Concat( new[ ] { GS } ).Select( G => G.Color ) ) ) )
board.Children.Add( animation );
return board;
ColorAnimationUsingKeyFrames _CreateGradientStopAnimation(
GradientStop stop, IEnumerable<Color> colors ) {
ColorAnimationUsingKeyFrames animation =
new ColorAnimationUsingKeyFrames( );
Storyboard.SetTarget( animation, stop );
Storyboard.SetTargetProperty(
animation, new PropertyPath(
GradientStop.ColorProperty ) );
foreach ( var keyFrame in colors.Select(
C => new EasingColorKeyFrame( C ) ) )
animation.KeyFrames.Add( keyFrame );
return animation;
}
}
}
}
I've tried using just the ColorAnimationUsingKeyFrames
for each gradient within the brush, and that DOES work, but I would prefer to use a Storyboard
( if possible ) so that all of the animations can be started together.
Is what I am trying to do possible? If so, what am I doing wrong?
If not, what can I do to accomplish what I am trying to get done here ( starting up many different animations simultaneously )?
c# wpf xaml storyboard code-behind
add a comment |
I'm attempting to create an animation for a GradientBrush
that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
Because of the complex nature of this task, I've taken to handling everything in the code behind, rather than in XAML.
Fortunately, I'm getting no errors or exceptions with my code.
Unfortunately, it's also doing absolutely nothing.
In compliance with the minimal, complete and verifiable example requirements:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Animation;
namespace MCVE {
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class Program {
private static LinearGradientBrush TestBrush { get; } =
new LinearGradientBrush(
new GradientStopCollection( new[ ]{
new GradientStop( Colors.Black, 0 / 1.0D ),
new GradientStop( Colors.White, 1 / 1.0D )
} ), new Point( 0.0D, 0.0D ), new Point( 1.0D, 1.0D ) );
[STAThread]
public static int Main( ) {
Storyboard board = CreateStoryboard( TestBrush );
Window w = new Window( ){
Background = TestBrush
};
w.Loaded += new RoutedEventHandler(
( S, E ) => board.Begin( w, true ) );
Program program = new Program( );
program.InitializeComponent( );
return program.Run( w );
}
public static Storyboard CreateStoryboard( GradientBrush brush ) {
Storyboard board = new Storyboard( ){
Duration = new Duration( TimeSpan.FromSeconds( 1.0D ) ),
RepeatBehavior = RepeatBehavior.Forever
};
foreach (
var animation
in brush.GradientStops.Select(
GS => _CreateGradientStopAnimation(
GS, brush.GradientStops.SkipWhile( G => G != GS
).Concat( brush.GradientStops.TakeWhile( G => G != GS )
).Concat( new[ ] { GS } ).Select( G => G.Color ) ) ) )
board.Children.Add( animation );
return board;
ColorAnimationUsingKeyFrames _CreateGradientStopAnimation(
GradientStop stop, IEnumerable<Color> colors ) {
ColorAnimationUsingKeyFrames animation =
new ColorAnimationUsingKeyFrames( );
Storyboard.SetTarget( animation, stop );
Storyboard.SetTargetProperty(
animation, new PropertyPath(
GradientStop.ColorProperty ) );
foreach ( var keyFrame in colors.Select(
C => new EasingColorKeyFrame( C ) ) )
animation.KeyFrames.Add( keyFrame );
return animation;
}
}
}
}
I've tried using just the ColorAnimationUsingKeyFrames
for each gradient within the brush, and that DOES work, but I would prefer to use a Storyboard
( if possible ) so that all of the animations can be started together.
Is what I am trying to do possible? If so, what am I doing wrong?
If not, what can I do to accomplish what I am trying to get done here ( starting up many different animations simultaneously )?
c# wpf xaml storyboard code-behind
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
@ChrisW. Board gets returned before the local function_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
– Will
Nov 13 '18 at 18:45
add a comment |
I'm attempting to create an animation for a GradientBrush
that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
Because of the complex nature of this task, I've taken to handling everything in the code behind, rather than in XAML.
Fortunately, I'm getting no errors or exceptions with my code.
Unfortunately, it's also doing absolutely nothing.
In compliance with the minimal, complete and verifiable example requirements:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Animation;
namespace MCVE {
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class Program {
private static LinearGradientBrush TestBrush { get; } =
new LinearGradientBrush(
new GradientStopCollection( new[ ]{
new GradientStop( Colors.Black, 0 / 1.0D ),
new GradientStop( Colors.White, 1 / 1.0D )
} ), new Point( 0.0D, 0.0D ), new Point( 1.0D, 1.0D ) );
[STAThread]
public static int Main( ) {
Storyboard board = CreateStoryboard( TestBrush );
Window w = new Window( ){
Background = TestBrush
};
w.Loaded += new RoutedEventHandler(
( S, E ) => board.Begin( w, true ) );
Program program = new Program( );
program.InitializeComponent( );
return program.Run( w );
}
public static Storyboard CreateStoryboard( GradientBrush brush ) {
Storyboard board = new Storyboard( ){
Duration = new Duration( TimeSpan.FromSeconds( 1.0D ) ),
RepeatBehavior = RepeatBehavior.Forever
};
foreach (
var animation
in brush.GradientStops.Select(
GS => _CreateGradientStopAnimation(
GS, brush.GradientStops.SkipWhile( G => G != GS
).Concat( brush.GradientStops.TakeWhile( G => G != GS )
).Concat( new[ ] { GS } ).Select( G => G.Color ) ) ) )
board.Children.Add( animation );
return board;
ColorAnimationUsingKeyFrames _CreateGradientStopAnimation(
GradientStop stop, IEnumerable<Color> colors ) {
ColorAnimationUsingKeyFrames animation =
new ColorAnimationUsingKeyFrames( );
Storyboard.SetTarget( animation, stop );
Storyboard.SetTargetProperty(
animation, new PropertyPath(
GradientStop.ColorProperty ) );
foreach ( var keyFrame in colors.Select(
C => new EasingColorKeyFrame( C ) ) )
animation.KeyFrames.Add( keyFrame );
return animation;
}
}
}
}
I've tried using just the ColorAnimationUsingKeyFrames
for each gradient within the brush, and that DOES work, but I would prefer to use a Storyboard
( if possible ) so that all of the animations can be started together.
Is what I am trying to do possible? If so, what am I doing wrong?
If not, what can I do to accomplish what I am trying to get done here ( starting up many different animations simultaneously )?
c# wpf xaml storyboard code-behind
I'm attempting to create an animation for a GradientBrush
that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
Because of the complex nature of this task, I've taken to handling everything in the code behind, rather than in XAML.
Fortunately, I'm getting no errors or exceptions with my code.
Unfortunately, it's also doing absolutely nothing.
In compliance with the minimal, complete and verifiable example requirements:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows;
using System.Windows.Media;
using System.Windows.Media.Animation;
namespace MCVE {
/// <summary>
/// Interaction logic for App.xaml
/// </summary>
public partial class Program {
private static LinearGradientBrush TestBrush { get; } =
new LinearGradientBrush(
new GradientStopCollection( new[ ]{
new GradientStop( Colors.Black, 0 / 1.0D ),
new GradientStop( Colors.White, 1 / 1.0D )
} ), new Point( 0.0D, 0.0D ), new Point( 1.0D, 1.0D ) );
[STAThread]
public static int Main( ) {
Storyboard board = CreateStoryboard( TestBrush );
Window w = new Window( ){
Background = TestBrush
};
w.Loaded += new RoutedEventHandler(
( S, E ) => board.Begin( w, true ) );
Program program = new Program( );
program.InitializeComponent( );
return program.Run( w );
}
public static Storyboard CreateStoryboard( GradientBrush brush ) {
Storyboard board = new Storyboard( ){
Duration = new Duration( TimeSpan.FromSeconds( 1.0D ) ),
RepeatBehavior = RepeatBehavior.Forever
};
foreach (
var animation
in brush.GradientStops.Select(
GS => _CreateGradientStopAnimation(
GS, brush.GradientStops.SkipWhile( G => G != GS
).Concat( brush.GradientStops.TakeWhile( G => G != GS )
).Concat( new[ ] { GS } ).Select( G => G.Color ) ) ) )
board.Children.Add( animation );
return board;
ColorAnimationUsingKeyFrames _CreateGradientStopAnimation(
GradientStop stop, IEnumerable<Color> colors ) {
ColorAnimationUsingKeyFrames animation =
new ColorAnimationUsingKeyFrames( );
Storyboard.SetTarget( animation, stop );
Storyboard.SetTargetProperty(
animation, new PropertyPath(
GradientStop.ColorProperty ) );
foreach ( var keyFrame in colors.Select(
C => new EasingColorKeyFrame( C ) ) )
animation.KeyFrames.Add( keyFrame );
return animation;
}
}
}
}
I've tried using just the ColorAnimationUsingKeyFrames
for each gradient within the brush, and that DOES work, but I would prefer to use a Storyboard
( if possible ) so that all of the animations can be started together.
Is what I am trying to do possible? If so, what am I doing wrong?
If not, what can I do to accomplish what I am trying to get done here ( starting up many different animations simultaneously )?
c# wpf xaml storyboard code-behind
c# wpf xaml storyboard code-behind
edited Nov 13 '18 at 8:03
Will
asked Nov 13 '18 at 2:26
WillWill
1,03612563
1,03612563
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
@ChrisW. Board gets returned before the local function_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
– Will
Nov 13 '18 at 18:45
add a comment |
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
@ChrisW. Board gets returned before the local function_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
– Will
Nov 13 '18 at 18:45
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
@ChrisW. Board gets returned before the local function
_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.– Will
Nov 13 '18 at 18:45
@ChrisW. Board gets returned before the local function
_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.– Will
Nov 13 '18 at 18:45
add a comment |
2 Answers
2
active
oldest
votes
Not sure what exactly the problem is, but it seems sufficient to freeze the Storyboard:
var storyboard = CreateStoryboard(TestBrush);
storyboard.Freeze();
Loaded += (s, e) => storyboard.Begin();
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
add a comment |
I'm attempting to create an animation for a GradientBrush that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
I could give you a leads how to do this with XAML
. As far as i understood your Storyboard
, a Color
moves slowly through the Background
with gradients to the foregoing and following Color
.
Concept
Fotunately only the Colors
with an Offset
between 0
and 1
are visible, therefore we can use this like a limiter on a Cologradient
with mutliple Colors
(see left figure).
If we now move the Offsets
of the GradientStops
from n
to -1
, it looks like the Color
wanders across the Window. To do this in a loop, we need to put the first two Colors
at the end of the gradient to start anew properly (see right figure).
XAML
This XAML
animates the Background of a Grid
:
<Grid >
<!-- Set Background (this is similar to your TestBrush, only the Offsets will be set later) -->
<Grid.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1">
<GradientStop Color="Red"/>
<GradientStop Color="Blue"/>
<GradientStop Color="Yellow"/>
</LinearGradientBrush>
</Grid.Background>
<!-- Start Storyboard when Element is loaded -->
<Grid.Triggers>
<EventTrigger RoutedEvent="Grid.Loaded">
<BeginStoryboard>
<!-- Ensure the Storyboard is repeated forever -->
<Storyboard RepeatBehavior="Forever">
<!-- Set Offsets of the Colors and animate them to move slowly (Linear) or be set immediately (Discrete) -->
<!-- Red (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[0].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="0" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:03"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:03"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="0" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Blue (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[1].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="1" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:06"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:06"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Yellow (just moves through once) -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[2].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Grid.Triggers>
</Grid>
In this example we have 3 Colors and every Color moves across the Grid
in 3 Seconds
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272888%2fwhy-doesnt-this-storyboard-defined-in-code-work%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Not sure what exactly the problem is, but it seems sufficient to freeze the Storyboard:
var storyboard = CreateStoryboard(TestBrush);
storyboard.Freeze();
Loaded += (s, e) => storyboard.Begin();
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
add a comment |
Not sure what exactly the problem is, but it seems sufficient to freeze the Storyboard:
var storyboard = CreateStoryboard(TestBrush);
storyboard.Freeze();
Loaded += (s, e) => storyboard.Begin();
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
add a comment |
Not sure what exactly the problem is, but it seems sufficient to freeze the Storyboard:
var storyboard = CreateStoryboard(TestBrush);
storyboard.Freeze();
Loaded += (s, e) => storyboard.Begin();
Not sure what exactly the problem is, but it seems sufficient to freeze the Storyboard:
var storyboard = CreateStoryboard(TestBrush);
storyboard.Freeze();
Loaded += (s, e) => storyboard.Begin();
answered Nov 15 '18 at 11:18
ClemensClemens
88k887171
88k887171
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
add a comment |
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
1
1
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
Perfect. Thank you.
– Will
Nov 15 '18 at 17:32
add a comment |
I'm attempting to create an animation for a GradientBrush that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
I could give you a leads how to do this with XAML
. As far as i understood your Storyboard
, a Color
moves slowly through the Background
with gradients to the foregoing and following Color
.
Concept
Fotunately only the Colors
with an Offset
between 0
and 1
are visible, therefore we can use this like a limiter on a Cologradient
with mutliple Colors
(see left figure).
If we now move the Offsets
of the GradientStops
from n
to -1
, it looks like the Color
wanders across the Window. To do this in a loop, we need to put the first two Colors
at the end of the gradient to start anew properly (see right figure).
XAML
This XAML
animates the Background of a Grid
:
<Grid >
<!-- Set Background (this is similar to your TestBrush, only the Offsets will be set later) -->
<Grid.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1">
<GradientStop Color="Red"/>
<GradientStop Color="Blue"/>
<GradientStop Color="Yellow"/>
</LinearGradientBrush>
</Grid.Background>
<!-- Start Storyboard when Element is loaded -->
<Grid.Triggers>
<EventTrigger RoutedEvent="Grid.Loaded">
<BeginStoryboard>
<!-- Ensure the Storyboard is repeated forever -->
<Storyboard RepeatBehavior="Forever">
<!-- Set Offsets of the Colors and animate them to move slowly (Linear) or be set immediately (Discrete) -->
<!-- Red (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[0].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="0" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:03"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:03"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="0" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Blue (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[1].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="1" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:06"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:06"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Yellow (just moves through once) -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[2].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Grid.Triggers>
</Grid>
In this example we have 3 Colors and every Color moves across the Grid
in 3 Seconds
add a comment |
I'm attempting to create an animation for a GradientBrush that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
I could give you a leads how to do this with XAML
. As far as i understood your Storyboard
, a Color
moves slowly through the Background
with gradients to the foregoing and following Color
.
Concept
Fotunately only the Colors
with an Offset
between 0
and 1
are visible, therefore we can use this like a limiter on a Cologradient
with mutliple Colors
(see left figure).
If we now move the Offsets
of the GradientStops
from n
to -1
, it looks like the Color
wanders across the Window. To do this in a loop, we need to put the first two Colors
at the end of the gradient to start anew properly (see right figure).
XAML
This XAML
animates the Background of a Grid
:
<Grid >
<!-- Set Background (this is similar to your TestBrush, only the Offsets will be set later) -->
<Grid.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1">
<GradientStop Color="Red"/>
<GradientStop Color="Blue"/>
<GradientStop Color="Yellow"/>
</LinearGradientBrush>
</Grid.Background>
<!-- Start Storyboard when Element is loaded -->
<Grid.Triggers>
<EventTrigger RoutedEvent="Grid.Loaded">
<BeginStoryboard>
<!-- Ensure the Storyboard is repeated forever -->
<Storyboard RepeatBehavior="Forever">
<!-- Set Offsets of the Colors and animate them to move slowly (Linear) or be set immediately (Discrete) -->
<!-- Red (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[0].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="0" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:03"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:03"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="0" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Blue (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[1].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="1" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:06"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:06"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Yellow (just moves through once) -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[2].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Grid.Triggers>
</Grid>
In this example we have 3 Colors and every Color moves across the Grid
in 3 Seconds
add a comment |
I'm attempting to create an animation for a GradientBrush that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
I could give you a leads how to do this with XAML
. As far as i understood your Storyboard
, a Color
moves slowly through the Background
with gradients to the foregoing and following Color
.
Concept
Fotunately only the Colors
with an Offset
between 0
and 1
are visible, therefore we can use this like a limiter on a Cologradient
with mutliple Colors
(see left figure).
If we now move the Offsets
of the GradientStops
from n
to -1
, it looks like the Color
wanders across the Window. To do this in a loop, we need to put the first two Colors
at the end of the gradient to start anew properly (see right figure).
XAML
This XAML
animates the Background of a Grid
:
<Grid >
<!-- Set Background (this is similar to your TestBrush, only the Offsets will be set later) -->
<Grid.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1">
<GradientStop Color="Red"/>
<GradientStop Color="Blue"/>
<GradientStop Color="Yellow"/>
</LinearGradientBrush>
</Grid.Background>
<!-- Start Storyboard when Element is loaded -->
<Grid.Triggers>
<EventTrigger RoutedEvent="Grid.Loaded">
<BeginStoryboard>
<!-- Ensure the Storyboard is repeated forever -->
<Storyboard RepeatBehavior="Forever">
<!-- Set Offsets of the Colors and animate them to move slowly (Linear) or be set immediately (Discrete) -->
<!-- Red (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[0].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="0" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:03"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:03"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="0" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Blue (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[1].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="1" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:06"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:06"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Yellow (just moves through once) -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[2].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Grid.Triggers>
</Grid>
In this example we have 3 Colors and every Color moves across the Grid
in 3 Seconds
I'm attempting to create an animation for a GradientBrush that will animate each gradient to cycle through all of the colors contained within the gradient indefinitely.
I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.
I could give you a leads how to do this with XAML
. As far as i understood your Storyboard
, a Color
moves slowly through the Background
with gradients to the foregoing and following Color
.
Concept
Fotunately only the Colors
with an Offset
between 0
and 1
are visible, therefore we can use this like a limiter on a Cologradient
with mutliple Colors
(see left figure).
If we now move the Offsets
of the GradientStops
from n
to -1
, it looks like the Color
wanders across the Window. To do this in a loop, we need to put the first two Colors
at the end of the gradient to start anew properly (see right figure).
XAML
This XAML
animates the Background of a Grid
:
<Grid >
<!-- Set Background (this is similar to your TestBrush, only the Offsets will be set later) -->
<Grid.Background>
<LinearGradientBrush StartPoint="0,0" EndPoint="1,1">
<GradientStop Color="Red"/>
<GradientStop Color="Blue"/>
<GradientStop Color="Yellow"/>
</LinearGradientBrush>
</Grid.Background>
<!-- Start Storyboard when Element is loaded -->
<Grid.Triggers>
<EventTrigger RoutedEvent="Grid.Loaded">
<BeginStoryboard>
<!-- Ensure the Storyboard is repeated forever -->
<Storyboard RepeatBehavior="Forever">
<!-- Set Offsets of the Colors and animate them to move slowly (Linear) or be set immediately (Discrete) -->
<!-- Red (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[0].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="0" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:03"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:03"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="0" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Blue (needs to be placed at the 'end' of the gradientbrush for a neat loop -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[1].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="1" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:06"/>
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:06"/> <!-- Move (jump) to end (n) when -1 is reached -->
<LinearDoubleKeyFrame Value="1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
<!-- Yellow (just moves through once) -->
<DoubleAnimationUsingKeyFrames Storyboard.TargetProperty="(Background).(LinearGradientBrush.GradientStops)[2].(GradientStop.Offset)">
<DiscreteDoubleKeyFrame Value="2" KeyTime="00:00:00"/>
<LinearDoubleKeyFrame Value="-1" KeyTime="00:00:09"/>
</DoubleAnimationUsingKeyFrames>
</Storyboard>
</BeginStoryboard>
</EventTrigger>
</Grid.Triggers>
</Grid>
In this example we have 3 Colors and every Color moves across the Grid
in 3 Seconds
answered Nov 15 '18 at 14:37
LittleBitLittleBit
51511
51511
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53272888%2fwhy-doesnt-this-storyboard-defined-in-code-work%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jM Or 624klDDRbGdkX t6TXEiCjNmL0iCBxfYEJ
At a quick glance, wouldn't animation not even get returned after board? Personally I very much prefer xaml for this sort of thing...
– Chris W.
Nov 13 '18 at 17:59
@ChrisW. Board gets returned before the local function
_CreateGradientStopAnimation(...
is declared. I would love to use XAML but the requirements are too complex for me to implement in XAML, mostly because I don't know how to make it work in XAML.– Will
Nov 13 '18 at 18:45