Inverse of Fourier transform gives “data type not supported” error
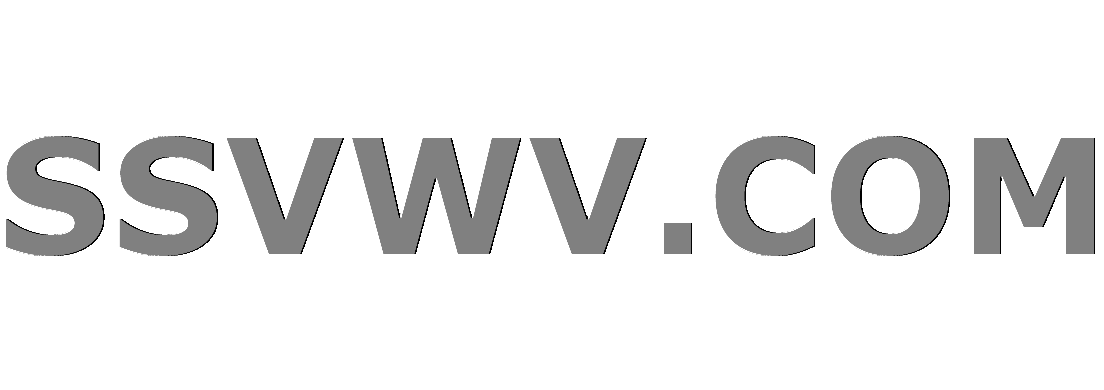
Multi tool use
I am trying to implementing the inverse of Fourier transform. Here's my code:
import numpy as np
import cv2 as cv
import math
import cmath
from matplotlib import pyplot as plt
image="test2.bmp"
img=cv.imread(image,cv.IMREAD_GRAYSCALE)
#foruior transform
dft = cv.dft(np.float32(img),flags = cv.DFT_COMPLEX_OUTPUT)
#shifting for displaying
dft_shift = np.fft.fftshift(dft)
#get the filter contains real and complex part
t=1
a=0.1
b=0.1
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
for x in range(img.shape[0]):
for y in range(img.shape[1]):
if x==0 and y==0:
const1=math.pi*(1e-10)
else:
const1=math.pi*((x*a)+(y*b))
#for real number
motion_filter[x,y,0]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).real
#for complex number
motion_filter[x,y,1]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).imag
#processing
fshift = dft_shift*motion_filter
#shift back
f_ishift = np.fft.ifftshift(fshift)
#inverse
img_back = cv.idft(f_ishift)
#take real part
img_back=img_back[:,:,0]
#show image
plt.imshow(img_back,cmap='gray')
plt.show()
And the error occurs when doing:
img_back = cv.idft(f_ishift)
The error message is:
src data type = 15 is not supported
How can I fix the code?
python opencv image-processing fft opencv3.0
add a comment |
I am trying to implementing the inverse of Fourier transform. Here's my code:
import numpy as np
import cv2 as cv
import math
import cmath
from matplotlib import pyplot as plt
image="test2.bmp"
img=cv.imread(image,cv.IMREAD_GRAYSCALE)
#foruior transform
dft = cv.dft(np.float32(img),flags = cv.DFT_COMPLEX_OUTPUT)
#shifting for displaying
dft_shift = np.fft.fftshift(dft)
#get the filter contains real and complex part
t=1
a=0.1
b=0.1
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
for x in range(img.shape[0]):
for y in range(img.shape[1]):
if x==0 and y==0:
const1=math.pi*(1e-10)
else:
const1=math.pi*((x*a)+(y*b))
#for real number
motion_filter[x,y,0]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).real
#for complex number
motion_filter[x,y,1]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).imag
#processing
fshift = dft_shift*motion_filter
#shift back
f_ishift = np.fft.ifftshift(fshift)
#inverse
img_back = cv.idft(f_ishift)
#take real part
img_back=img_back[:,:,0]
#show image
plt.imshow(img_back,cmap='gray')
plt.show()
And the error occurs when doing:
img_back = cv.idft(f_ishift)
The error message is:
src data type = 15 is not supported
How can I fix the code?
python opencv image-processing fft opencv3.0
2
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02
add a comment |
I am trying to implementing the inverse of Fourier transform. Here's my code:
import numpy as np
import cv2 as cv
import math
import cmath
from matplotlib import pyplot as plt
image="test2.bmp"
img=cv.imread(image,cv.IMREAD_GRAYSCALE)
#foruior transform
dft = cv.dft(np.float32(img),flags = cv.DFT_COMPLEX_OUTPUT)
#shifting for displaying
dft_shift = np.fft.fftshift(dft)
#get the filter contains real and complex part
t=1
a=0.1
b=0.1
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
for x in range(img.shape[0]):
for y in range(img.shape[1]):
if x==0 and y==0:
const1=math.pi*(1e-10)
else:
const1=math.pi*((x*a)+(y*b))
#for real number
motion_filter[x,y,0]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).real
#for complex number
motion_filter[x,y,1]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).imag
#processing
fshift = dft_shift*motion_filter
#shift back
f_ishift = np.fft.ifftshift(fshift)
#inverse
img_back = cv.idft(f_ishift)
#take real part
img_back=img_back[:,:,0]
#show image
plt.imshow(img_back,cmap='gray')
plt.show()
And the error occurs when doing:
img_back = cv.idft(f_ishift)
The error message is:
src data type = 15 is not supported
How can I fix the code?
python opencv image-processing fft opencv3.0
I am trying to implementing the inverse of Fourier transform. Here's my code:
import numpy as np
import cv2 as cv
import math
import cmath
from matplotlib import pyplot as plt
image="test2.bmp"
img=cv.imread(image,cv.IMREAD_GRAYSCALE)
#foruior transform
dft = cv.dft(np.float32(img),flags = cv.DFT_COMPLEX_OUTPUT)
#shifting for displaying
dft_shift = np.fft.fftshift(dft)
#get the filter contains real and complex part
t=1
a=0.1
b=0.1
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
for x in range(img.shape[0]):
for y in range(img.shape[1]):
if x==0 and y==0:
const1=math.pi*(1e-10)
else:
const1=math.pi*((x*a)+(y*b))
#for real number
motion_filter[x,y,0]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).real
#for complex number
motion_filter[x,y,1]=((t/const1)*(math.sin(const1))*(cmath.exp((0-1j)*(const1)))).imag
#processing
fshift = dft_shift*motion_filter
#shift back
f_ishift = np.fft.ifftshift(fshift)
#inverse
img_back = cv.idft(f_ishift)
#take real part
img_back=img_back[:,:,0]
#show image
plt.imshow(img_back,cmap='gray')
plt.show()
And the error occurs when doing:
img_back = cv.idft(f_ishift)
The error message is:
src data type = 15 is not supported
How can I fix the code?
python opencv image-processing fft opencv3.0
python opencv image-processing fft opencv3.0
edited Nov 13 '18 at 16:30


Cris Luengo
19.8k52249
19.8k52249
asked Nov 13 '18 at 13:15
kriskris
345
345
2
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02
add a comment |
2
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02
2
2
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02
add a comment |
1 Answer
1
active
oldest
votes
According to the answer in this other question, the OpenCV idft
requires a real-valued matrix where the real and imaginary components are stored along a third dimension. You create this matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
It is of the right sizes (2 along the 3rd dimension, for the real and imaginary components), but it is complex-valued. Next you multiply your Fourier-domain image (a real-valued matrix with real and imaginary components along the 3rd dimension) with this complex matrix, creating a complex-valued output:
fshift = dft_shift*motion_filter
This complex-valued output cannot be used in cv.idft
. Instead, create your filter matrix as a real-valued matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2))
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281835%2finverse-of-fourier-transform-gives-data-type-not-supported-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
According to the answer in this other question, the OpenCV idft
requires a real-valued matrix where the real and imaginary components are stored along a third dimension. You create this matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
It is of the right sizes (2 along the 3rd dimension, for the real and imaginary components), but it is complex-valued. Next you multiply your Fourier-domain image (a real-valued matrix with real and imaginary components along the 3rd dimension) with this complex matrix, creating a complex-valued output:
fshift = dft_shift*motion_filter
This complex-valued output cannot be used in cv.idft
. Instead, create your filter matrix as a real-valued matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2))
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
add a comment |
According to the answer in this other question, the OpenCV idft
requires a real-valued matrix where the real and imaginary components are stored along a third dimension. You create this matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
It is of the right sizes (2 along the 3rd dimension, for the real and imaginary components), but it is complex-valued. Next you multiply your Fourier-domain image (a real-valued matrix with real and imaginary components along the 3rd dimension) with this complex matrix, creating a complex-valued output:
fshift = dft_shift*motion_filter
This complex-valued output cannot be used in cv.idft
. Instead, create your filter matrix as a real-valued matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2))
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
add a comment |
According to the answer in this other question, the OpenCV idft
requires a real-valued matrix where the real and imaginary components are stored along a third dimension. You create this matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
It is of the right sizes (2 along the 3rd dimension, for the real and imaginary components), but it is complex-valued. Next you multiply your Fourier-domain image (a real-valued matrix with real and imaginary components along the 3rd dimension) with this complex matrix, creating a complex-valued output:
fshift = dft_shift*motion_filter
This complex-valued output cannot be used in cv.idft
. Instead, create your filter matrix as a real-valued matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2))
According to the answer in this other question, the OpenCV idft
requires a real-valued matrix where the real and imaginary components are stored along a third dimension. You create this matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2),dtype=np.complex_)
It is of the right sizes (2 along the 3rd dimension, for the real and imaginary components), but it is complex-valued. Next you multiply your Fourier-domain image (a real-valued matrix with real and imaginary components along the 3rd dimension) with this complex matrix, creating a complex-valued output:
fshift = dft_shift*motion_filter
This complex-valued output cannot be used in cv.idft
. Instead, create your filter matrix as a real-valued matrix:
motion_filter=np.empty((img.shape[0],img.shape[1],2))
answered Nov 13 '18 at 16:29


Cris LuengoCris Luengo
19.8k52249
19.8k52249
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
add a comment |
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
Am I doing the correct way to store the real part and complex part for kernel?
– kris
Nov 14 '18 at 4:21
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
@kris: Looks correct to me, but I haven't tried running your code. But you are computing that complex value twice, which is not efficient.
– Cris Luengo
Nov 14 '18 at 4:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
Okay I’ll post an example later, I tried this but not working out....
– kris
Nov 14 '18 at 6:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53281835%2finverse-of-fourier-transform-gives-data-type-not-supported-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MMJFBLR97Qipa8
2
Did you try to search on SO before posting the question? Exact (not possible) Duplicate: stackoverflow.com/questions/30989915/…
– Rick M.
Nov 13 '18 at 13:48
Not working . I tried before
– kris
Nov 13 '18 at 13:53
Possible duplicate of TypeError: src data type = 15 is not supported
– n00dle
Nov 13 '18 at 16:02