Capturing multi td elements in the table
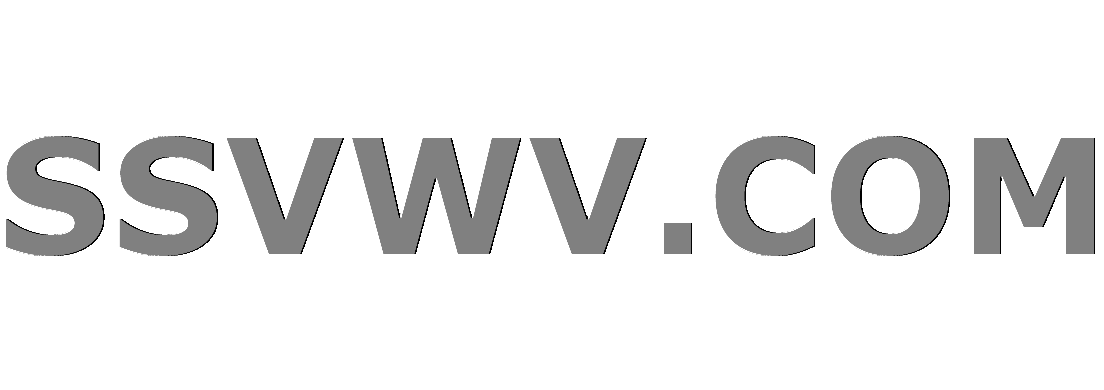
Multi tool use
I am working on implementation of selecting cells in the table.
I found really good solution here:
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
The cell selection works great. After the selection, I want to be able to select more cells, without the previous ones disappearing and also be able to deselect the already selected one.
Now basically every time I click into the table, previous selection disappears and the new one start.
Does anyone have any idea, how I could implement this?
Any thoughts on this much appreciated.
javascript jquery html angularjs
add a comment |
I am working on implementation of selecting cells in the table.
I found really good solution here:
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
The cell selection works great. After the selection, I want to be able to select more cells, without the previous ones disappearing and also be able to deselect the already selected one.
Now basically every time I click into the table, previous selection disappears and the new one start.
Does anyone have any idea, how I could implement this?
Any thoughts on this much appreciated.
javascript jquery html angularjs
What have you tried so far?
– Dekel
Nov 12 at 10:46
You could detect thectrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
The selection works with rectangles. What you could do: onmouseDown
: ifctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.
– Jean-Marc Zimmer
Nov 12 at 10:52
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53
add a comment |
I am working on implementation of selecting cells in the table.
I found really good solution here:
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
The cell selection works great. After the selection, I want to be able to select more cells, without the previous ones disappearing and also be able to deselect the already selected one.
Now basically every time I click into the table, previous selection disappears and the new one start.
Does anyone have any idea, how I could implement this?
Any thoughts on this much appreciated.
javascript jquery html angularjs
I am working on implementation of selecting cells in the table.
I found really good solution here:
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
The cell selection works great. After the selection, I want to be able to select more cells, without the previous ones disappearing and also be able to deselect the already selected one.
Now basically every time I click into the table, previous selection disappears and the new one start.
Does anyone have any idea, how I could implement this?
Any thoughts on this much appreciated.
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
$scope.dragSelectIds = ;
$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
$scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1,x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x<=x2 && y>=y1 && y<=y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {minX:0,minY:0,maxX:0,maxY:0};
bounds.minX = $window.Math.min($(start).offset().left,$(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top,$(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left+$(end).width(),$(start).offset().left+$(start).width());
bounds.maxY = $window.Math.max($(end).offset().top+$(end).height(),$(start).offset().top+$(start).height());
var initiallySelectedTds = rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
for(var i=0;i<initiallySelectedTds.length;i++){
if($(initiallySelectedTds[i]).offset().left<bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if($(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width()>bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left+$(initiallySelectedTds[i]).width();
if($(initiallySelectedTds[i]).offset().top<bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if($(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height()>bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top+$(initiallySelectedTds[i]).height();
}
return rectangleSelect("td",bounds.minX,bounds.maxX,bounds.minY,bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>document.write('<base href="' + document.location + '" />');</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
javascript jquery html angularjs
javascript jquery html angularjs
edited Nov 12 at 12:09


Rohit.007
1,5862419
1,5862419
asked Nov 12 at 10:42
jansv
88117
88117
What have you tried so far?
– Dekel
Nov 12 at 10:46
You could detect thectrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
The selection works with rectangles. What you could do: onmouseDown
: ifctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.
– Jean-Marc Zimmer
Nov 12 at 10:52
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53
add a comment |
What have you tried so far?
– Dekel
Nov 12 at 10:46
You could detect thectrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
The selection works with rectangles. What you could do: onmouseDown
: ifctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.
– Jean-Marc Zimmer
Nov 12 at 10:52
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53
What have you tried so far?
– Dekel
Nov 12 at 10:46
What have you tried so far?
– Dekel
Nov 12 at 10:46
You could detect the
ctrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
You could detect the
ctrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
The selection works with rectangles. What you could do: on
mouseDown
: if ctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.– Jean-Marc Zimmer
Nov 12 at 10:52
The selection works with rectangles. What you could do: on
mouseDown
: if ctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.– Jean-Marc Zimmer
Nov 12 at 10:52
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53
add a comment |
1 Answer
1
active
oldest
votes
Just modify you setEndCell function
like,
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setEndCell(el) {
if (el.hasClass(cls)) { // if added then remove on click
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
// change if added, then not to add twice.
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
|
show 4 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260462%2fcapturing-multi-td-elements-in-the-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Just modify you setEndCell function
like,
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setEndCell(el) {
if (el.hasClass(cls)) { // if added then remove on click
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
// change if added, then not to add twice.
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
|
show 4 more comments
Just modify you setEndCell function
like,
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setEndCell(el) {
if (el.hasClass(cls)) { // if added then remove on click
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
// change if added, then not to add twice.
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
|
show 4 more comments
Just modify you setEndCell function
like,
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setEndCell(el) {
if (el.hasClass(cls)) { // if added then remove on click
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
// change if added, then not to add twice.
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
Just modify you setEndCell function
like,
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setEndCell(el) {
if (el.hasClass(cls)) { // if added then remove on click
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
// change if added, then not to add twice.
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
var app = angular.module('plunker', );
app.controller('MainCtrl', function($scope) {
$scope.ids = ;
});
app.directive('dragSelect', function($window, $document) {
return {
scope: {
dragSelectIds: '='
},
controller: function($scope, $element) {
var cls = 'eng-selected-item';
var startCell = null;
var dragging = false;
function mouseUp(el) {
dragging = false;
}
function mouseDown(el) {
dragging = true;
setStartCell(el);
setEndCell(el);
}
function mouseEnter(el) {
if (!dragging) return;
setEndCell(el);
}
function setStartCell(el) {
startCell = el;
}
function setEndCell(el) {
if (el.hasClass(cls)) {
el.removeClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex !==-1 && $scope.dragSelectIds.splice(elIndex, 1)
return false;
}
if (!$scope.dragSelectIds) {
$scope.dragSelectIds = ;
}
//$element.find('td').removeClass(cls);
$(cellsBetween(startCell, el)).each(function() {
var el = angular.element(this);
el.addClass(cls);
var elIndex = $scope.dragSelectIds.indexOf(el[0].id);
elIndex ===-1 && $scope.dragSelectIds.push(el.attr('id'));
});
}
function rectangleSelect(selector, x1, x2, y1, y2) {
var elements = ;
jQuery(selector).each(function() {
var $this = jQuery(this);
var offset = $this.offset();
var x = offset.left;
var y = offset.top;
var w = $this.width();
var h = $this.height();
if (x >= x1 && x <= x2 && y >= y1 && y <= y2) {
// this element fits inside the selection rectangle
elements.push($this.get(0));
}
});
return elements;
}
function cellsBetween(start, end) {
var bounds = {
minX: 0,
minY: 0,
maxX: 0,
maxY: 0
};
bounds.minX = $window.Math.min($(start).offset().left, $(end).offset().left);
bounds.minY = $window.Math.min($(start).offset().top, $(end).offset().top);
bounds.maxX = $window.Math.max($(end).offset().left + $(end).width(), $(start).offset().left + $(start).width());
bounds.maxY = $window.Math.max($(end).offset().top + $(end).height(), $(start).offset().top + $(start).height());
var initiallySelectedTds = rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
for (var i = 0; i < initiallySelectedTds.length; i++) {
if ($(initiallySelectedTds[i]).offset().left < bounds.minX)
bounds.minX = $(initiallySelectedTds[i]).offset().left;
if ($(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width() > bounds.maxX)
bounds.maxX = $(initiallySelectedTds[i]).offset().left + $(initiallySelectedTds[i]).width();
if ($(initiallySelectedTds[i]).offset().top < bounds.minY)
bounds.minY = $(initiallySelectedTds[i]).offset().top;
if ($(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height() > bounds.maxY)
bounds.maxY = $(initiallySelectedTds[i]).offset().top + $(initiallySelectedTds[i]).height();
}
return rectangleSelect("td", bounds.minX, bounds.maxX, bounds.minY, bounds.maxY);
}
function wrap(fn) {
return function() {
var el = angular.element(this);
$scope.$apply(function() {
fn(el);
});
}
}
$element.delegate('td', 'mousedown', wrap(mouseDown));
$element.delegate('td', 'mouseenter', wrap(mouseEnter));
$document.delegate('body', 'mouseup', wrap(mouseUp));
}
}
});
[drag-select] {
cursor: pointer;
-webkit-touch-callout: none;
-webkit-user-select: none;
-khtml-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
[drag-select] .eng-selected-item {
background: blue;
color: white;
}
td {
padding: 10px;
border: 1px solid gray;
}
<!DOCTYPE html>
<html ng-app="plunker">
<head>
<meta charset="utf-8" />
<title>AngularJS Plunker</title>
<script>
document.write('<base href="' + document.location + '" />');
</script>
<link rel="stylesheet" href="style.css" />
<script data-require="jquery" data-semver="2.0.3" src="http://code.jquery.com/jquery-2.0.3.min.js"></script>
<script data-require="angular.js@1.2.x" src="https://ajax.googleapis.com/ajax/libs/angularjs/1.2.16/angular.min.js" data-semver="1.2.16"></script>
<script src="app.js"></script>
</head>
<body ng-controller="MainCtrl">
<table drag-select drag-select-ids="ids">
<tr>
<td id="td-1-1">1-1</td>
<td id="td-1-2">1-2</td>
<td id="td-1-3">1-3</td>
<td id="td-1-4">1-4</td>
<td id="td-1-5">1-5</td>
<td id="td-1-6">1-6</td>
<td id="td-1-7">1-7</td>
<td id="td-1-8">1-8</td>
<td id="td-1-9">1-9</td>
<td id="td-1-10">1-10</td>
</tr>
<tr>
<td id="td-2-1">2-1</td>
<td id="td-2-2">2-2</td>
<td id="td-2-3">2-3</td>
<td id="td-2-4">2-4</td>
<td id="td-2-5">2-5</td>
<td id="td-2-6">2-6</td>
<td id="td-2-7">2-7</td>
<td id="td-2-8">2-8</td>
<td id="td-2-9">2-9</td>
<td id="td-2-10">2-10</td>
</tr>
<tr>
<td id="td-3-1">3-1</td>
<td id="td-3-2">3-2</td>
<td id="td-3-3">3-3</td>
<td id="td-3-4">3-4</td>
<td id="td-3-5">3-5</td>
<td id="td-3-6">3-6</td>
<td id="td-3-7">3-7</td>
<td id="td-3-8">3-8</td>
<td id="td-3-9">3-9</td>
<td id="td-3-10">3-10</td>
</tr>
<tr>
<td id="td-4-1">4-1</td>
<td id="td-4-2">4-2</td>
<td id="td-4-3">4-3</td>
<td id="td-4-4">4-4</td>
<td id="td-4-5">4-5</td>
<td id="td-4-6">4-6</td>
<td id="td-4-7">4-7</td>
<td id="td-4-8">4-8</td>
<td id="td-4-9">4-9</td>
<td id="td-4-10">4-10</td>
</tr>
<tr>
<td id="td-5-1">5-1</td>
<td id="td-5-2">5-2</td>
<td id="td-5-3">5-3</td>
<td id="td-5-4">5-4</td>
<td id="td-5-5">5-5</td>
<td id="td-5-6">5-6</td>
<td id="td-5-7">5-7</td>
<td id="td-5-8">5-8</td>
<td id="td-5-9">5-9</td>
<td id="td-5-10">5-10</td>
</tr>
</table>
<p>Selected IDs: {{ids | json}}</p>
</body>
</html>
edited Nov 12 at 11:50
answered Nov 12 at 11:05


Rohan Kumar
35k96090
35k96090
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
|
show 4 more comments
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Cheers, this is exactly what I was looking for!
– jansv
Nov 12 at 11:15
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Most welcome (like).
– Rohan Kumar
Nov 12 at 11:16
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Do you know, how I would deselect the cells once clicked?
– jansv
Nov 12 at 11:17
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Now when you drag across items, all of the IDs are added every time (duplicating items in the output). I need to check somehow, if the ID is already in the array and if it is, do not add.
– jansv
Nov 12 at 11:30
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
Again Updated..
– Rohan Kumar
Nov 12 at 11:37
|
show 4 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53260462%2fcapturing-multi-td-elements-in-the-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Gp1o6VaLkdwo8xCz wy,nl
What have you tried so far?
– Dekel
Nov 12 at 10:46
You could detect the
ctrl-click
– Jean-Marc Zimmer
Nov 12 at 10:48
I was trying to store the output in the variable, and than passing it to the function every time the mouse clicks, but with no success. I am new to JavaScript and cant really get my head around it..
– jansv
Nov 12 at 10:50
The selection works with rectangles. What you could do: on
mouseDown
: ifctrl
is down : create a new rectangle, else : clear all rectangles. You need an array to store all rectangles.– Jean-Marc Zimmer
Nov 12 at 10:52
Provide the code you tried so far. Stackoverflow is here to help you, not to solve your problems. Show that you spent some time working on it...
– Dekel
Nov 12 at 10:53