Laravel seeder - check if unique slug exists already
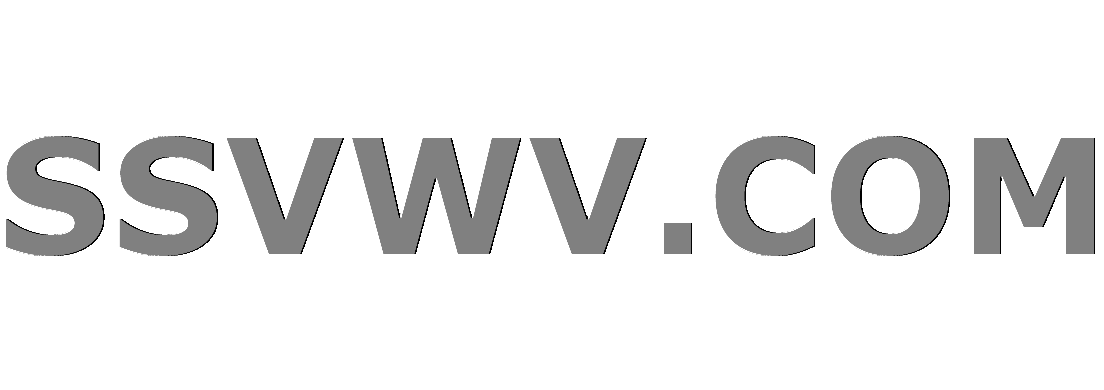
Multi tool use
up vote
1
down vote
favorite
I am seeding a database table ('games') from several csv files. The table has a many-to-many-relationship with another table ('consoles'). The code works for the first csv file...
I am expecting to encounter duplicates (one game may release on multiple platforms).
Rather than an error exception, I would first like to check if the record (unique slug) already exists within the database table,
- if slug has already been used, just update relationships to existing record, syncing the pivot table against new console (I believe this is attach or sync?)
- if not - create a new record.
The model/schema is setup as such:
<?php
use IlluminateSupportFacadesSchema;
use IlluminateDatabaseSchemaBlueprint;
use IlluminateDatabaseMigrationsMigration;
class CreateGamesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('games', function (Blueprint $table) {
$table->increments('id');
$table->string('title');
$table->string('slug')->unique();
...
An example seeder code is
use IlluminateDatabaseSeeder;
use IlluminateDatabaseEloquentModel;
use IlluminateSupportStr;
class SnesGamesSeeder extends Seeder
{
public function run()
{
// Fetch the data from our CSV File
$data = $this->seedFromCSV(base_path('/database/seeds/csvs/snes-csv-filtered.csv'), ',');
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
$date = strtotime($game['release_date']);
$format_date = date('Y-m-d',$date);
DB::table('games')->insert(
[
'title' => $title,
'slug' => $slug,
'release_date' => $format_date,
...
I would like to be able to do something like
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
**if(*** $slug already exists in 'game' table){
(find ID) -> ->consoles()->attach(5);
} else {**
DB::table('games')->insert(
[
'title' => $game['Title'],
'slug' => Str::slug($game['Title']),
'release_date' => $format_date,
...
laravel seeding csv-import
add a comment |
up vote
1
down vote
favorite
I am seeding a database table ('games') from several csv files. The table has a many-to-many-relationship with another table ('consoles'). The code works for the first csv file...
I am expecting to encounter duplicates (one game may release on multiple platforms).
Rather than an error exception, I would first like to check if the record (unique slug) already exists within the database table,
- if slug has already been used, just update relationships to existing record, syncing the pivot table against new console (I believe this is attach or sync?)
- if not - create a new record.
The model/schema is setup as such:
<?php
use IlluminateSupportFacadesSchema;
use IlluminateDatabaseSchemaBlueprint;
use IlluminateDatabaseMigrationsMigration;
class CreateGamesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('games', function (Blueprint $table) {
$table->increments('id');
$table->string('title');
$table->string('slug')->unique();
...
An example seeder code is
use IlluminateDatabaseSeeder;
use IlluminateDatabaseEloquentModel;
use IlluminateSupportStr;
class SnesGamesSeeder extends Seeder
{
public function run()
{
// Fetch the data from our CSV File
$data = $this->seedFromCSV(base_path('/database/seeds/csvs/snes-csv-filtered.csv'), ',');
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
$date = strtotime($game['release_date']);
$format_date = date('Y-m-d',$date);
DB::table('games')->insert(
[
'title' => $title,
'slug' => $slug,
'release_date' => $format_date,
...
I would like to be able to do something like
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
**if(*** $slug already exists in 'game' table){
(find ID) -> ->consoles()->attach(5);
} else {**
DB::table('games')->insert(
[
'title' => $game['Title'],
'slug' => Str::slug($game['Title']),
'release_date' => $format_date,
...
laravel seeding csv-import
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am seeding a database table ('games') from several csv files. The table has a many-to-many-relationship with another table ('consoles'). The code works for the first csv file...
I am expecting to encounter duplicates (one game may release on multiple platforms).
Rather than an error exception, I would first like to check if the record (unique slug) already exists within the database table,
- if slug has already been used, just update relationships to existing record, syncing the pivot table against new console (I believe this is attach or sync?)
- if not - create a new record.
The model/schema is setup as such:
<?php
use IlluminateSupportFacadesSchema;
use IlluminateDatabaseSchemaBlueprint;
use IlluminateDatabaseMigrationsMigration;
class CreateGamesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('games', function (Blueprint $table) {
$table->increments('id');
$table->string('title');
$table->string('slug')->unique();
...
An example seeder code is
use IlluminateDatabaseSeeder;
use IlluminateDatabaseEloquentModel;
use IlluminateSupportStr;
class SnesGamesSeeder extends Seeder
{
public function run()
{
// Fetch the data from our CSV File
$data = $this->seedFromCSV(base_path('/database/seeds/csvs/snes-csv-filtered.csv'), ',');
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
$date = strtotime($game['release_date']);
$format_date = date('Y-m-d',$date);
DB::table('games')->insert(
[
'title' => $title,
'slug' => $slug,
'release_date' => $format_date,
...
I would like to be able to do something like
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
**if(*** $slug already exists in 'game' table){
(find ID) -> ->consoles()->attach(5);
} else {**
DB::table('games')->insert(
[
'title' => $game['Title'],
'slug' => Str::slug($game['Title']),
'release_date' => $format_date,
...
laravel seeding csv-import
I am seeding a database table ('games') from several csv files. The table has a many-to-many-relationship with another table ('consoles'). The code works for the first csv file...
I am expecting to encounter duplicates (one game may release on multiple platforms).
Rather than an error exception, I would first like to check if the record (unique slug) already exists within the database table,
- if slug has already been used, just update relationships to existing record, syncing the pivot table against new console (I believe this is attach or sync?)
- if not - create a new record.
The model/schema is setup as such:
<?php
use IlluminateSupportFacadesSchema;
use IlluminateDatabaseSchemaBlueprint;
use IlluminateDatabaseMigrationsMigration;
class CreateGamesTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('games', function (Blueprint $table) {
$table->increments('id');
$table->string('title');
$table->string('slug')->unique();
...
An example seeder code is
use IlluminateDatabaseSeeder;
use IlluminateDatabaseEloquentModel;
use IlluminateSupportStr;
class SnesGamesSeeder extends Seeder
{
public function run()
{
// Fetch the data from our CSV File
$data = $this->seedFromCSV(base_path('/database/seeds/csvs/snes-csv-filtered.csv'), ',');
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
$date = strtotime($game['release_date']);
$format_date = date('Y-m-d',$date);
DB::table('games')->insert(
[
'title' => $title,
'slug' => $slug,
'release_date' => $format_date,
...
I would like to be able to do something like
foreach ($data as $game)
{
$title = $game['Title'];
$slug = Str::slug($title)
**if(*** $slug already exists in 'game' table){
(find ID) -> ->consoles()->attach(5);
} else {**
DB::table('games')->insert(
[
'title' => $game['Title'],
'slug' => Str::slug($game['Title']),
'release_date' => $format_date,
...
laravel seeding csv-import
laravel seeding csv-import
edited Nov 11 at 0:39
asked Nov 11 at 0:25


BeardyMrDeaks
364
364
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You can use the first
method:
DB::table('game')->where('slug', $slug)->first();
Example
foreach ($data as $game) {
$title = $game['Title'];
$slug = Str::slug($title);
if ($game = DB::table('game')->where('slug', $slug)->first()) {
// update existing record
} else {
// insert new record
}
}
If you've created the corresponding Eloquent models, the updateOrCreate
method allows you to do:
$attributes = ['foo' => 'bar'];
// if a game exists with the given slug, update the attributes, otherwise create it.
$game = Game::updateOrCreate(
['slug' => $slug],
$attributes
);
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You can use the first
method:
DB::table('game')->where('slug', $slug)->first();
Example
foreach ($data as $game) {
$title = $game['Title'];
$slug = Str::slug($title);
if ($game = DB::table('game')->where('slug', $slug)->first()) {
// update existing record
} else {
// insert new record
}
}
If you've created the corresponding Eloquent models, the updateOrCreate
method allows you to do:
$attributes = ['foo' => 'bar'];
// if a game exists with the given slug, update the attributes, otherwise create it.
$game = Game::updateOrCreate(
['slug' => $slug],
$attributes
);
add a comment |
up vote
0
down vote
You can use the first
method:
DB::table('game')->where('slug', $slug)->first();
Example
foreach ($data as $game) {
$title = $game['Title'];
$slug = Str::slug($title);
if ($game = DB::table('game')->where('slug', $slug)->first()) {
// update existing record
} else {
// insert new record
}
}
If you've created the corresponding Eloquent models, the updateOrCreate
method allows you to do:
$attributes = ['foo' => 'bar'];
// if a game exists with the given slug, update the attributes, otherwise create it.
$game = Game::updateOrCreate(
['slug' => $slug],
$attributes
);
add a comment |
up vote
0
down vote
up vote
0
down vote
You can use the first
method:
DB::table('game')->where('slug', $slug)->first();
Example
foreach ($data as $game) {
$title = $game['Title'];
$slug = Str::slug($title);
if ($game = DB::table('game')->where('slug', $slug)->first()) {
// update existing record
} else {
// insert new record
}
}
If you've created the corresponding Eloquent models, the updateOrCreate
method allows you to do:
$attributes = ['foo' => 'bar'];
// if a game exists with the given slug, update the attributes, otherwise create it.
$game = Game::updateOrCreate(
['slug' => $slug],
$attributes
);
You can use the first
method:
DB::table('game')->where('slug', $slug)->first();
Example
foreach ($data as $game) {
$title = $game['Title'];
$slug = Str::slug($title);
if ($game = DB::table('game')->where('slug', $slug)->first()) {
// update existing record
} else {
// insert new record
}
}
If you've created the corresponding Eloquent models, the updateOrCreate
method allows you to do:
$attributes = ['foo' => 'bar'];
// if a game exists with the given slug, update the attributes, otherwise create it.
$game = Game::updateOrCreate(
['slug' => $slug],
$attributes
);
edited Nov 11 at 2:01
answered Nov 11 at 1:54
DigitalDrifter
6,1112422
6,1112422
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244738%2flaravel-seeder-check-if-unique-slug-exists-already%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zegCL2p3JNLTSWrWI,6nnsGO FQhEhNsiH N