SignalR send from Server console App to client
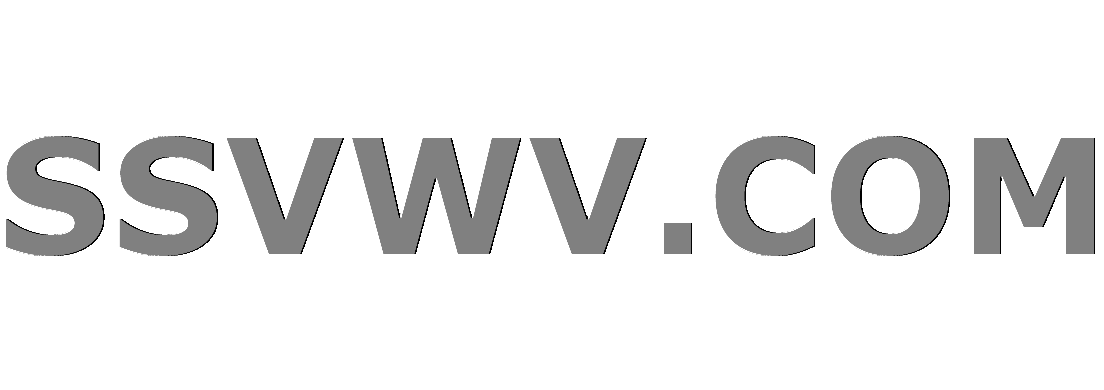
Multi tool use
is it even possible to send a message to all(or selected) connected clients connected to the hub? I mean from the server side to all clients. I can get clients data to server window, but when I enter and try to send from server side nothing happens on clients window. How to push notifications to all straight from server app?
Server:
namespace SignalRHub
{
class Program
{
static void Main(string args)
{
string url = @"http://localhost:8080/";
using (WebApp.Start<Startup>(url))
{
Console.WriteLine(string.Format("Server running at {0}", url));
Console.ReadLine();
while (true)
{
// get text to send
Console.WriteLine("Enter your message:");
string line = Console.ReadLine();
// Get hub context
IHubContext ctx = GlobalHost.ConnectionManager.GetHubContext<TestHub>();
// call addMessage on all clients of context
ctx.Clients.All.addMessage(line);
// pause to allow clients to receive
Console.ReadLine();
}
}
}
class Startup
{
public void Configuration(IAppBuilder app)
{
app.UseCors(CorsOptions.AllowAll);
app.MapSignalR();
}
}
[HubName("TestHub")]
public class TestHub : Hub
{
public void SendMsg(string message)
{
Console.WriteLine(message);
}
}
}
}
Client:
namespace SignalRClient
{
class Program
{
static void Main(string args)
{
IHubProxy _hub;
string url = @"http://localhost:8080/";
try
{
Console.WriteLine("Connecting to: " +url);
var connection = new HubConnection(url);
_hub = connection.CreateHubProxy("TestHub");
connection.Start().Wait();
Console.WriteLine();
Console.WriteLine();
Console.WriteLine("---------- Connection OK.");
Console.WriteLine();
}
catch (Exception e)
{
Console.WriteLine("------------ Connection FAILED: ");
Console.WriteLine(e);
throw;
}
string line = null;
while ((line = System.Console.ReadLine()) != null)
{
_hub.Invoke("SendMsg", line).Wait();
}
Console.Read();
}
}
}
c# console-application signalr-hub
add a comment |
is it even possible to send a message to all(or selected) connected clients connected to the hub? I mean from the server side to all clients. I can get clients data to server window, but when I enter and try to send from server side nothing happens on clients window. How to push notifications to all straight from server app?
Server:
namespace SignalRHub
{
class Program
{
static void Main(string args)
{
string url = @"http://localhost:8080/";
using (WebApp.Start<Startup>(url))
{
Console.WriteLine(string.Format("Server running at {0}", url));
Console.ReadLine();
while (true)
{
// get text to send
Console.WriteLine("Enter your message:");
string line = Console.ReadLine();
// Get hub context
IHubContext ctx = GlobalHost.ConnectionManager.GetHubContext<TestHub>();
// call addMessage on all clients of context
ctx.Clients.All.addMessage(line);
// pause to allow clients to receive
Console.ReadLine();
}
}
}
class Startup
{
public void Configuration(IAppBuilder app)
{
app.UseCors(CorsOptions.AllowAll);
app.MapSignalR();
}
}
[HubName("TestHub")]
public class TestHub : Hub
{
public void SendMsg(string message)
{
Console.WriteLine(message);
}
}
}
}
Client:
namespace SignalRClient
{
class Program
{
static void Main(string args)
{
IHubProxy _hub;
string url = @"http://localhost:8080/";
try
{
Console.WriteLine("Connecting to: " +url);
var connection = new HubConnection(url);
_hub = connection.CreateHubProxy("TestHub");
connection.Start().Wait();
Console.WriteLine();
Console.WriteLine();
Console.WriteLine("---------- Connection OK.");
Console.WriteLine();
}
catch (Exception e)
{
Console.WriteLine("------------ Connection FAILED: ");
Console.WriteLine(e);
throw;
}
string line = null;
while ((line = System.Console.ReadLine()) != null)
{
_hub.Invoke("SendMsg", line).Wait();
}
Console.Read();
}
}
}
c# console-application signalr-hub
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for theClients.All.
call you have server-side. Something like:_hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30
add a comment |
is it even possible to send a message to all(or selected) connected clients connected to the hub? I mean from the server side to all clients. I can get clients data to server window, but when I enter and try to send from server side nothing happens on clients window. How to push notifications to all straight from server app?
Server:
namespace SignalRHub
{
class Program
{
static void Main(string args)
{
string url = @"http://localhost:8080/";
using (WebApp.Start<Startup>(url))
{
Console.WriteLine(string.Format("Server running at {0}", url));
Console.ReadLine();
while (true)
{
// get text to send
Console.WriteLine("Enter your message:");
string line = Console.ReadLine();
// Get hub context
IHubContext ctx = GlobalHost.ConnectionManager.GetHubContext<TestHub>();
// call addMessage on all clients of context
ctx.Clients.All.addMessage(line);
// pause to allow clients to receive
Console.ReadLine();
}
}
}
class Startup
{
public void Configuration(IAppBuilder app)
{
app.UseCors(CorsOptions.AllowAll);
app.MapSignalR();
}
}
[HubName("TestHub")]
public class TestHub : Hub
{
public void SendMsg(string message)
{
Console.WriteLine(message);
}
}
}
}
Client:
namespace SignalRClient
{
class Program
{
static void Main(string args)
{
IHubProxy _hub;
string url = @"http://localhost:8080/";
try
{
Console.WriteLine("Connecting to: " +url);
var connection = new HubConnection(url);
_hub = connection.CreateHubProxy("TestHub");
connection.Start().Wait();
Console.WriteLine();
Console.WriteLine();
Console.WriteLine("---------- Connection OK.");
Console.WriteLine();
}
catch (Exception e)
{
Console.WriteLine("------------ Connection FAILED: ");
Console.WriteLine(e);
throw;
}
string line = null;
while ((line = System.Console.ReadLine()) != null)
{
_hub.Invoke("SendMsg", line).Wait();
}
Console.Read();
}
}
}
c# console-application signalr-hub
is it even possible to send a message to all(or selected) connected clients connected to the hub? I mean from the server side to all clients. I can get clients data to server window, but when I enter and try to send from server side nothing happens on clients window. How to push notifications to all straight from server app?
Server:
namespace SignalRHub
{
class Program
{
static void Main(string args)
{
string url = @"http://localhost:8080/";
using (WebApp.Start<Startup>(url))
{
Console.WriteLine(string.Format("Server running at {0}", url));
Console.ReadLine();
while (true)
{
// get text to send
Console.WriteLine("Enter your message:");
string line = Console.ReadLine();
// Get hub context
IHubContext ctx = GlobalHost.ConnectionManager.GetHubContext<TestHub>();
// call addMessage on all clients of context
ctx.Clients.All.addMessage(line);
// pause to allow clients to receive
Console.ReadLine();
}
}
}
class Startup
{
public void Configuration(IAppBuilder app)
{
app.UseCors(CorsOptions.AllowAll);
app.MapSignalR();
}
}
[HubName("TestHub")]
public class TestHub : Hub
{
public void SendMsg(string message)
{
Console.WriteLine(message);
}
}
}
}
Client:
namespace SignalRClient
{
class Program
{
static void Main(string args)
{
IHubProxy _hub;
string url = @"http://localhost:8080/";
try
{
Console.WriteLine("Connecting to: " +url);
var connection = new HubConnection(url);
_hub = connection.CreateHubProxy("TestHub");
connection.Start().Wait();
Console.WriteLine();
Console.WriteLine();
Console.WriteLine("---------- Connection OK.");
Console.WriteLine();
}
catch (Exception e)
{
Console.WriteLine("------------ Connection FAILED: ");
Console.WriteLine(e);
throw;
}
string line = null;
while ((line = System.Console.ReadLine()) != null)
{
_hub.Invoke("SendMsg", line).Wait();
}
Console.Read();
}
}
}
c# console-application signalr-hub
c# console-application signalr-hub
asked Nov 15 '18 at 20:38
Vyt AutasVyt Autas
164
164
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for theClients.All.
call you have server-side. Something like:_hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30
add a comment |
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for theClients.All.
call you have server-side. Something like:_hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for the
Clients.All.
call you have server-side. Something like: _hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for the
Clients.All.
call you have server-side. Something like: _hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327554%2fsignalr-send-from-server-console-app-to-client%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327554%2fsignalr-send-from-server-console-app-to-client%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uPPDMQD5vErt
Yes what you want to do is possible with SignalR. You need to add some code client-side that explicitly creates registers a callback for the
Clients.All.
call you have server-side. Something like:_hub.on('addMessage', function(line) { console.log(line); });
– David Tansey
Nov 15 '18 at 21:44
Thanks, as I understand client side has to be somehow subscribed to servers side method which pushes notifications? Could you please give a code example which I could use in actual client? Is this the only way or may be there is a way to force push to all of them like do "console.writeline("bla bla") to all clients", and then message appears in their console, they want it or not! possible?
– Vyt Autas
Nov 15 '18 at 22:03
Refer to the following MSDN page for some examples: docs.microsoft.com/en-us/aspnet/signalr/overview/…
– David Tansey
Nov 15 '18 at 22:51
All examples on internet is for webforms only...
– Vyt Autas
Nov 16 '18 at 9:30