How do I highlight an entire trace upon hover in Plotly for Python?
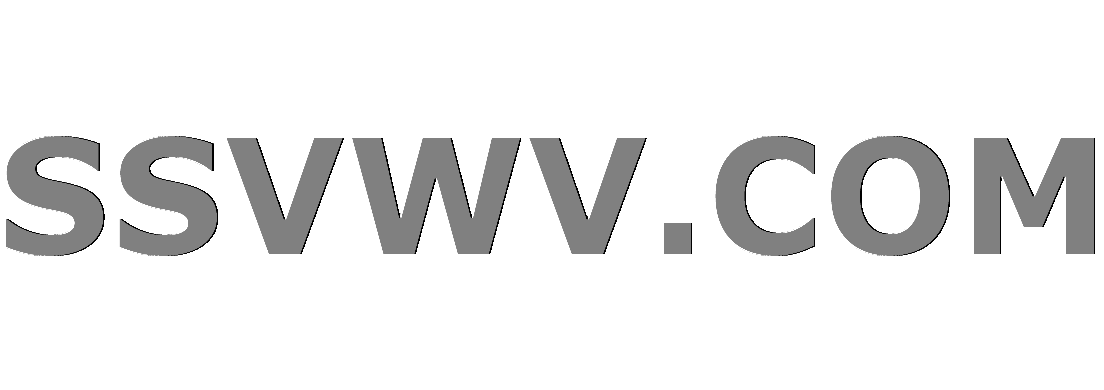
Multi tool use
I want a trace to be highlighted (color or opacity change) when selected with mouse hover. I have looked into restyle
functionality, but it may not be appropriate for my use case.
It looks like this has been discussed on Github, but I'm not sure if it has been resolved/implemented.
Here is an example in Bokeh of what I want to accomplish in Plotly Python:
from bokeh.plotting import figure, show, output_notebook
from bokeh.models import HoverTool
from bokeh.models import ColumnDataSource
output_notebook()
p = figure(plot_width=400, plot_height=400,y_range=(0.2,0.5))
y_vals = [0.22,0.22,0.25,0.25,0.26,0.26,0.27,0.27]
y_vals2 = [y*1.4 for y in y_vals]
x_vals = [0,1,1,2,2,2,2,3]
data_dict = {'x':[x_vals,x_vals],
'y':[y_vals,y_vals2],
'color':["firebrick", "navy"],
'alpha':[0.1, 0.1]}
source = ColumnDataSource(data_dict)
p.multi_line('x','y',source=source,
color='color', alpha='alpha', line_width=4,
hover_line_alpha=1.0,hover_line_color='color')
p.add_tools(HoverTool(show_arrow=True,
line_policy='nearest',
))
show(p)
python plotly
add a comment |
I want a trace to be highlighted (color or opacity change) when selected with mouse hover. I have looked into restyle
functionality, but it may not be appropriate for my use case.
It looks like this has been discussed on Github, but I'm not sure if it has been resolved/implemented.
Here is an example in Bokeh of what I want to accomplish in Plotly Python:
from bokeh.plotting import figure, show, output_notebook
from bokeh.models import HoverTool
from bokeh.models import ColumnDataSource
output_notebook()
p = figure(plot_width=400, plot_height=400,y_range=(0.2,0.5))
y_vals = [0.22,0.22,0.25,0.25,0.26,0.26,0.27,0.27]
y_vals2 = [y*1.4 for y in y_vals]
x_vals = [0,1,1,2,2,2,2,3]
data_dict = {'x':[x_vals,x_vals],
'y':[y_vals,y_vals2],
'color':["firebrick", "navy"],
'alpha':[0.1, 0.1]}
source = ColumnDataSource(data_dict)
p.multi_line('x','y',source=source,
color='color', alpha='alpha', line_width=4,
hover_line_alpha=1.0,hover_line_color='color')
p.add_tools(HoverTool(show_arrow=True,
line_policy='nearest',
))
show(p)
python plotly
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17
add a comment |
I want a trace to be highlighted (color or opacity change) when selected with mouse hover. I have looked into restyle
functionality, but it may not be appropriate for my use case.
It looks like this has been discussed on Github, but I'm not sure if it has been resolved/implemented.
Here is an example in Bokeh of what I want to accomplish in Plotly Python:
from bokeh.plotting import figure, show, output_notebook
from bokeh.models import HoverTool
from bokeh.models import ColumnDataSource
output_notebook()
p = figure(plot_width=400, plot_height=400,y_range=(0.2,0.5))
y_vals = [0.22,0.22,0.25,0.25,0.26,0.26,0.27,0.27]
y_vals2 = [y*1.4 for y in y_vals]
x_vals = [0,1,1,2,2,2,2,3]
data_dict = {'x':[x_vals,x_vals],
'y':[y_vals,y_vals2],
'color':["firebrick", "navy"],
'alpha':[0.1, 0.1]}
source = ColumnDataSource(data_dict)
p.multi_line('x','y',source=source,
color='color', alpha='alpha', line_width=4,
hover_line_alpha=1.0,hover_line_color='color')
p.add_tools(HoverTool(show_arrow=True,
line_policy='nearest',
))
show(p)
python plotly
I want a trace to be highlighted (color or opacity change) when selected with mouse hover. I have looked into restyle
functionality, but it may not be appropriate for my use case.
It looks like this has been discussed on Github, but I'm not sure if it has been resolved/implemented.
Here is an example in Bokeh of what I want to accomplish in Plotly Python:
from bokeh.plotting import figure, show, output_notebook
from bokeh.models import HoverTool
from bokeh.models import ColumnDataSource
output_notebook()
p = figure(plot_width=400, plot_height=400,y_range=(0.2,0.5))
y_vals = [0.22,0.22,0.25,0.25,0.26,0.26,0.27,0.27]
y_vals2 = [y*1.4 for y in y_vals]
x_vals = [0,1,1,2,2,2,2,3]
data_dict = {'x':[x_vals,x_vals],
'y':[y_vals,y_vals2],
'color':["firebrick", "navy"],
'alpha':[0.1, 0.1]}
source = ColumnDataSource(data_dict)
p.multi_line('x','y',source=source,
color='color', alpha='alpha', line_width=4,
hover_line_alpha=1.0,hover_line_color='color')
p.add_tools(HoverTool(show_arrow=True,
line_policy='nearest',
))
show(p)
python plotly
python plotly
edited Dec 19 '18 at 21:53
scottlittle
asked Nov 15 '18 at 20:39
scottlittlescottlittle
5,78532546
5,78532546
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17
add a comment |
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17
add a comment |
1 Answer
1
active
oldest
votes
You can use Plotly's FigureWidget
functionality.
import plotly.graph_objs as go
import random
f = go.FigureWidget()
f.layout.hovermode = 'closest'
f.layout.hoverdistance = -1 #ensures no "gaps" for selecting sparse data
default_linewidth = 2
highlighted_linewidth_delta = 2
# just some traces with random data points
num_of_traces = 5
random.seed = 42
for i in range(num_of_traces):
y = [random.random() + i / 2 for _ in range(100)]
trace = go.Scatter(y=y, mode='lines', line={ 'width': default_linewidth })
f.add_trace(trace)
# our custom event handler
def update_trace(trace, points, selector):
# this list stores the points which were clicked on
# in all but one event they it be empty
if len(points.point_inds) > 0:
for i in range( len(f.data) ):
f.data[i]['line']['width'] = default_linewidth + highlighted_linewidth_delta * (i == points.trace_index)
# we need to add the on_click event to each trace separately
for i in range( len(f.data) ):
f.data[i].on_click(update_trace)
# let's show the figure
f
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327572%2fhow-do-i-highlight-an-entire-trace-upon-hover-in-plotly-for-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use Plotly's FigureWidget
functionality.
import plotly.graph_objs as go
import random
f = go.FigureWidget()
f.layout.hovermode = 'closest'
f.layout.hoverdistance = -1 #ensures no "gaps" for selecting sparse data
default_linewidth = 2
highlighted_linewidth_delta = 2
# just some traces with random data points
num_of_traces = 5
random.seed = 42
for i in range(num_of_traces):
y = [random.random() + i / 2 for _ in range(100)]
trace = go.Scatter(y=y, mode='lines', line={ 'width': default_linewidth })
f.add_trace(trace)
# our custom event handler
def update_trace(trace, points, selector):
# this list stores the points which were clicked on
# in all but one event they it be empty
if len(points.point_inds) > 0:
for i in range( len(f.data) ):
f.data[i]['line']['width'] = default_linewidth + highlighted_linewidth_delta * (i == points.trace_index)
# we need to add the on_click event to each trace separately
for i in range( len(f.data) ):
f.data[i].on_click(update_trace)
# let's show the figure
f
add a comment |
You can use Plotly's FigureWidget
functionality.
import plotly.graph_objs as go
import random
f = go.FigureWidget()
f.layout.hovermode = 'closest'
f.layout.hoverdistance = -1 #ensures no "gaps" for selecting sparse data
default_linewidth = 2
highlighted_linewidth_delta = 2
# just some traces with random data points
num_of_traces = 5
random.seed = 42
for i in range(num_of_traces):
y = [random.random() + i / 2 for _ in range(100)]
trace = go.Scatter(y=y, mode='lines', line={ 'width': default_linewidth })
f.add_trace(trace)
# our custom event handler
def update_trace(trace, points, selector):
# this list stores the points which were clicked on
# in all but one event they it be empty
if len(points.point_inds) > 0:
for i in range( len(f.data) ):
f.data[i]['line']['width'] = default_linewidth + highlighted_linewidth_delta * (i == points.trace_index)
# we need to add the on_click event to each trace separately
for i in range( len(f.data) ):
f.data[i].on_click(update_trace)
# let's show the figure
f
add a comment |
You can use Plotly's FigureWidget
functionality.
import plotly.graph_objs as go
import random
f = go.FigureWidget()
f.layout.hovermode = 'closest'
f.layout.hoverdistance = -1 #ensures no "gaps" for selecting sparse data
default_linewidth = 2
highlighted_linewidth_delta = 2
# just some traces with random data points
num_of_traces = 5
random.seed = 42
for i in range(num_of_traces):
y = [random.random() + i / 2 for _ in range(100)]
trace = go.Scatter(y=y, mode='lines', line={ 'width': default_linewidth })
f.add_trace(trace)
# our custom event handler
def update_trace(trace, points, selector):
# this list stores the points which were clicked on
# in all but one event they it be empty
if len(points.point_inds) > 0:
for i in range( len(f.data) ):
f.data[i]['line']['width'] = default_linewidth + highlighted_linewidth_delta * (i == points.trace_index)
# we need to add the on_click event to each trace separately
for i in range( len(f.data) ):
f.data[i].on_click(update_trace)
# let's show the figure
f
You can use Plotly's FigureWidget
functionality.
import plotly.graph_objs as go
import random
f = go.FigureWidget()
f.layout.hovermode = 'closest'
f.layout.hoverdistance = -1 #ensures no "gaps" for selecting sparse data
default_linewidth = 2
highlighted_linewidth_delta = 2
# just some traces with random data points
num_of_traces = 5
random.seed = 42
for i in range(num_of_traces):
y = [random.random() + i / 2 for _ in range(100)]
trace = go.Scatter(y=y, mode='lines', line={ 'width': default_linewidth })
f.add_trace(trace)
# our custom event handler
def update_trace(trace, points, selector):
# this list stores the points which were clicked on
# in all but one event they it be empty
if len(points.point_inds) > 0:
for i in range( len(f.data) ):
f.data[i]['line']['width'] = default_linewidth + highlighted_linewidth_delta * (i == points.trace_index)
# we need to add the on_click event to each trace separately
for i in range( len(f.data) ):
f.data[i].on_click(update_trace)
# let's show the figure
f
edited Jan 14 at 19:59
scottlittle
5,78532546
5,78532546
answered Dec 27 '18 at 17:38


Maximilian PetersMaximilian Peters
15.5k63353
15.5k63353
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53327572%2fhow-do-i-highlight-an-entire-trace-upon-hover-in-plotly-for-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
prpS,SB8,dHUuCB2hbwyTRUKe9 fJhVYF,FqxoEyP1 6wN5UZS5hnA38GROuJI W1ndIL6dOvjwX5 3cjXKQJVNsZNRD66K 3Wwl4h
Are you trying to use it an IPython notebook or using Dash?
– Maximilian Peters
Dec 27 '18 at 14:10
Jupyter notebook or lab
– scottlittle
Dec 27 '18 at 15:17