How to pass object through Web client call to a web API controller that expects object as parameter?
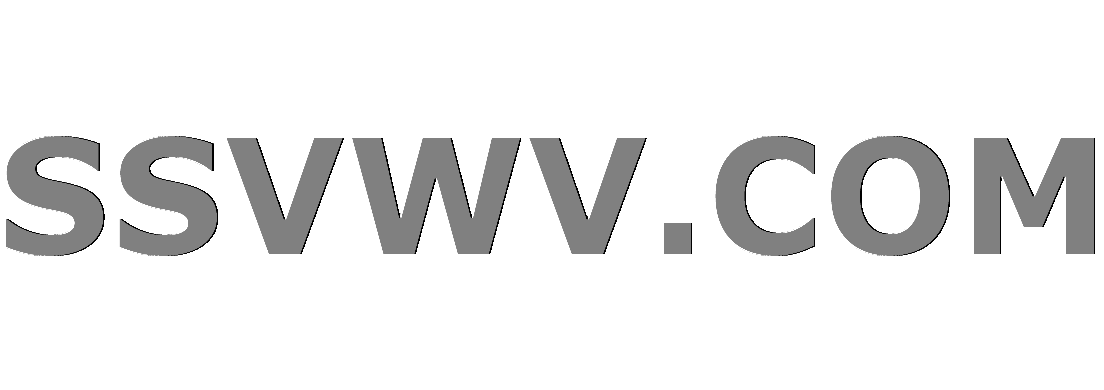
Multi tool use
I have console app as client and am trying to get worksheet data by passing client Id to a web API controller.Then creating a ClientWorkSheet object with received info from previous API call plus some other details. (Code working fine until this point)
Now I want to pass this ClientWorkSheet object as parameter to another API controller that takes the object and prints it out. I am getting media-type not supported error at this pointcall To API:. Controller action method:
Here is the WebClient Code:
using (var client = new WebClient())
{
client.Headers.Add("Content-Type:application/octet-stream");
client.Headers.Add("Origin:http://localhost:50920");
client.Headers.Add("Access-Control-Allow-
Origin:http://localhost:9000");
client.UseDefaultCredentials = true;
client.Credentials = CredentialCache.DefaultCredentials;
}
asp.net-web-api
add a comment |
I have console app as client and am trying to get worksheet data by passing client Id to a web API controller.Then creating a ClientWorkSheet object with received info from previous API call plus some other details. (Code working fine until this point)
Now I want to pass this ClientWorkSheet object as parameter to another API controller that takes the object and prints it out. I am getting media-type not supported error at this pointcall To API:. Controller action method:
Here is the WebClient Code:
using (var client = new WebClient())
{
client.Headers.Add("Content-Type:application/octet-stream");
client.Headers.Add("Origin:http://localhost:50920");
client.Headers.Add("Access-Control-Allow-
Origin:http://localhost:9000");
client.UseDefaultCredentials = true;
client.Credentials = CredentialCache.DefaultCredentials;
}
asp.net-web-api
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
1
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16
add a comment |
I have console app as client and am trying to get worksheet data by passing client Id to a web API controller.Then creating a ClientWorkSheet object with received info from previous API call plus some other details. (Code working fine until this point)
Now I want to pass this ClientWorkSheet object as parameter to another API controller that takes the object and prints it out. I am getting media-type not supported error at this pointcall To API:. Controller action method:
Here is the WebClient Code:
using (var client = new WebClient())
{
client.Headers.Add("Content-Type:application/octet-stream");
client.Headers.Add("Origin:http://localhost:50920");
client.Headers.Add("Access-Control-Allow-
Origin:http://localhost:9000");
client.UseDefaultCredentials = true;
client.Credentials = CredentialCache.DefaultCredentials;
}
asp.net-web-api
I have console app as client and am trying to get worksheet data by passing client Id to a web API controller.Then creating a ClientWorkSheet object with received info from previous API call plus some other details. (Code working fine until this point)
Now I want to pass this ClientWorkSheet object as parameter to another API controller that takes the object and prints it out. I am getting media-type not supported error at this pointcall To API:. Controller action method:
Here is the WebClient Code:
using (var client = new WebClient())
{
client.Headers.Add("Content-Type:application/octet-stream");
client.Headers.Add("Origin:http://localhost:50920");
client.Headers.Add("Access-Control-Allow-
Origin:http://localhost:9000");
client.UseDefaultCredentials = true;
client.Credentials = CredentialCache.DefaultCredentials;
}
asp.net-web-api
asp.net-web-api
edited Nov 15 '18 at 22:44


Nkosi
119k17137201
119k17137201
asked Nov 15 '18 at 18:35
techietechie
15
15
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
1
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16
add a comment |
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
1
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
1
1
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16
add a comment |
1 Answer
1
active
oldest
votes
One of my colleagues suggested the use of RestSharp to pass objects in Web API call and it worked for me. Sharing as it is pretty simple to use.
var reportRequest = new RestSharp.RestRequest("api/ClientWorksheet/CreateClientWorksheetAsPdf", RestSharp.Method.POST)
{
RequestFormat = RestSharp.DataFormat.Json
};
reportRequest.AddBody(sheet);
var restClient = new RestSharp.RestClient
{
BaseUrl = new Uri("http://localhost:50061"),
Authenticator = new RestSharp.Authenticators.NtlmAuthenticator()
};
var response = restClient.Execute<DataSet>(reportRequest);
if (response.StatusCode == HttpStatusCode.OK)
{
//success
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325891%2fhow-to-pass-object-through-web-client-call-to-a-web-api-controller-that-expects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
One of my colleagues suggested the use of RestSharp to pass objects in Web API call and it worked for me. Sharing as it is pretty simple to use.
var reportRequest = new RestSharp.RestRequest("api/ClientWorksheet/CreateClientWorksheetAsPdf", RestSharp.Method.POST)
{
RequestFormat = RestSharp.DataFormat.Json
};
reportRequest.AddBody(sheet);
var restClient = new RestSharp.RestClient
{
BaseUrl = new Uri("http://localhost:50061"),
Authenticator = new RestSharp.Authenticators.NtlmAuthenticator()
};
var response = restClient.Execute<DataSet>(reportRequest);
if (response.StatusCode == HttpStatusCode.OK)
{
//success
}
add a comment |
One of my colleagues suggested the use of RestSharp to pass objects in Web API call and it worked for me. Sharing as it is pretty simple to use.
var reportRequest = new RestSharp.RestRequest("api/ClientWorksheet/CreateClientWorksheetAsPdf", RestSharp.Method.POST)
{
RequestFormat = RestSharp.DataFormat.Json
};
reportRequest.AddBody(sheet);
var restClient = new RestSharp.RestClient
{
BaseUrl = new Uri("http://localhost:50061"),
Authenticator = new RestSharp.Authenticators.NtlmAuthenticator()
};
var response = restClient.Execute<DataSet>(reportRequest);
if (response.StatusCode == HttpStatusCode.OK)
{
//success
}
add a comment |
One of my colleagues suggested the use of RestSharp to pass objects in Web API call and it worked for me. Sharing as it is pretty simple to use.
var reportRequest = new RestSharp.RestRequest("api/ClientWorksheet/CreateClientWorksheetAsPdf", RestSharp.Method.POST)
{
RequestFormat = RestSharp.DataFormat.Json
};
reportRequest.AddBody(sheet);
var restClient = new RestSharp.RestClient
{
BaseUrl = new Uri("http://localhost:50061"),
Authenticator = new RestSharp.Authenticators.NtlmAuthenticator()
};
var response = restClient.Execute<DataSet>(reportRequest);
if (response.StatusCode == HttpStatusCode.OK)
{
//success
}
One of my colleagues suggested the use of RestSharp to pass objects in Web API call and it worked for me. Sharing as it is pretty simple to use.
var reportRequest = new RestSharp.RestRequest("api/ClientWorksheet/CreateClientWorksheetAsPdf", RestSharp.Method.POST)
{
RequestFormat = RestSharp.DataFormat.Json
};
reportRequest.AddBody(sheet);
var restClient = new RestSharp.RestClient
{
BaseUrl = new Uri("http://localhost:50061"),
Authenticator = new RestSharp.Authenticators.NtlmAuthenticator()
};
var response = restClient.Execute<DataSet>(reportRequest);
if (response.StatusCode == HttpStatusCode.OK)
{
//success
}
answered Nov 20 '18 at 23:22
techietechie
15
15
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325891%2fhow-to-pass-object-through-web-client-call-to-a-web-api-controller-that-expects%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sy1cxeXsfli4,qHMeN2,XE wEvM07nRinT ELMXF9f 50TX 7NUE6GT SIH VVB 42t4A96XPYLFZtQQcXOiJQkCU
Logically if the webapi uses json.net to deserialize and object, then you would use json.net to serialize the object... at least that is probably the easiest way to do it.
– Erik Philips
Nov 15 '18 at 18:40
Did not understand your comment. Are you saying that I should deserialize the object in the action method? If yes, then what should the input parameter type be instead of object? Have added controller code img too for reference. Pls help
– techie
Nov 15 '18 at 18:50
1
I won't be looking at your controller code, please provide the actual code not a screen shot. Also, only add the actual web api method that is relevant.
– Erik Philips
Nov 15 '18 at 20:16