What are the best practices for appium framework using page opject model
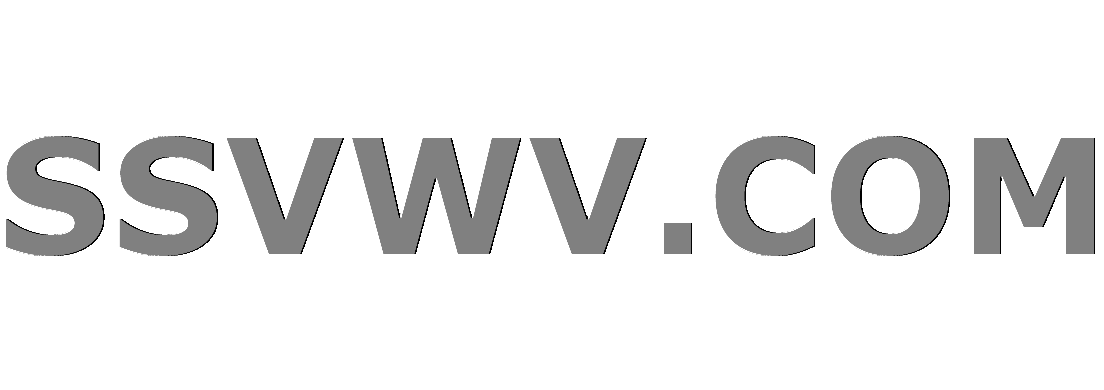
Multi tool use
I am developing an automation framework for a mobile application using Appium (in java). I started with creating a class per view, in each class, I find and initialize elements of that class. Now after a while I understand my configuration for the framework is not correct and I'm not able to expand it. It would be great if anyone can introduce me an implemented framework on Github.
here is my config class, I use setdriver() to set a driver wherever I need.
public class Config {
public AndroidDriver<AndroidElement> driver;
public static AndroidDriver<AndroidElement> SetDriver() throws MalformedURLException {
File appPath= new File("src");
File app = new File(appPath,"My-debug.apk");
DesiredCapabilities cap = new DesiredCapabilities();
cap.setCapability(MobileCapabilityType.DEVICE_NAME, "myDevice");
cap.setCapability(MobileCapabilityType.APP,app.getAbsolutePath());
cap.setCapability("autoGrantPermissions",true);
cap.setCapability("appWaitActivity","com.xxxx.xxxx.ui.launch.LaunchActivity");
AndroidDriver<AndroidElement> driver = new AndroidDriver<AndroidElement>(new URL("http://127.0.0.1:4723/wd/hub"),cap);
return driver;
}
Thank you in advance.
selenium testing automation appium
add a comment |
I am developing an automation framework for a mobile application using Appium (in java). I started with creating a class per view, in each class, I find and initialize elements of that class. Now after a while I understand my configuration for the framework is not correct and I'm not able to expand it. It would be great if anyone can introduce me an implemented framework on Github.
here is my config class, I use setdriver() to set a driver wherever I need.
public class Config {
public AndroidDriver<AndroidElement> driver;
public static AndroidDriver<AndroidElement> SetDriver() throws MalformedURLException {
File appPath= new File("src");
File app = new File(appPath,"My-debug.apk");
DesiredCapabilities cap = new DesiredCapabilities();
cap.setCapability(MobileCapabilityType.DEVICE_NAME, "myDevice");
cap.setCapability(MobileCapabilityType.APP,app.getAbsolutePath());
cap.setCapability("autoGrantPermissions",true);
cap.setCapability("appWaitActivity","com.xxxx.xxxx.ui.launch.LaunchActivity");
AndroidDriver<AndroidElement> driver = new AndroidDriver<AndroidElement>(new URL("http://127.0.0.1:4723/wd/hub"),cap);
return driver;
}
Thank you in advance.
selenium testing automation appium
add a comment |
I am developing an automation framework for a mobile application using Appium (in java). I started with creating a class per view, in each class, I find and initialize elements of that class. Now after a while I understand my configuration for the framework is not correct and I'm not able to expand it. It would be great if anyone can introduce me an implemented framework on Github.
here is my config class, I use setdriver() to set a driver wherever I need.
public class Config {
public AndroidDriver<AndroidElement> driver;
public static AndroidDriver<AndroidElement> SetDriver() throws MalformedURLException {
File appPath= new File("src");
File app = new File(appPath,"My-debug.apk");
DesiredCapabilities cap = new DesiredCapabilities();
cap.setCapability(MobileCapabilityType.DEVICE_NAME, "myDevice");
cap.setCapability(MobileCapabilityType.APP,app.getAbsolutePath());
cap.setCapability("autoGrantPermissions",true);
cap.setCapability("appWaitActivity","com.xxxx.xxxx.ui.launch.LaunchActivity");
AndroidDriver<AndroidElement> driver = new AndroidDriver<AndroidElement>(new URL("http://127.0.0.1:4723/wd/hub"),cap);
return driver;
}
Thank you in advance.
selenium testing automation appium
I am developing an automation framework for a mobile application using Appium (in java). I started with creating a class per view, in each class, I find and initialize elements of that class. Now after a while I understand my configuration for the framework is not correct and I'm not able to expand it. It would be great if anyone can introduce me an implemented framework on Github.
here is my config class, I use setdriver() to set a driver wherever I need.
public class Config {
public AndroidDriver<AndroidElement> driver;
public static AndroidDriver<AndroidElement> SetDriver() throws MalformedURLException {
File appPath= new File("src");
File app = new File(appPath,"My-debug.apk");
DesiredCapabilities cap = new DesiredCapabilities();
cap.setCapability(MobileCapabilityType.DEVICE_NAME, "myDevice");
cap.setCapability(MobileCapabilityType.APP,app.getAbsolutePath());
cap.setCapability("autoGrantPermissions",true);
cap.setCapability("appWaitActivity","com.xxxx.xxxx.ui.launch.LaunchActivity");
AndroidDriver<AndroidElement> driver = new AndroidDriver<AndroidElement>(new URL("http://127.0.0.1:4723/wd/hub"),cap);
return driver;
}
Thank you in advance.
selenium testing automation appium
selenium testing automation appium
asked Nov 13 '18 at 22:11
HamidHamid
539
539
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
First you have to create base class which load the driver.
Note: If you are using thread local, we can achieve the parallel execution without any issues
package com.vg.ui.utils.mobile;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.android.AndroidDriver;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Mobile {
public static ThreadLocal<AppiumDriver> driverThread = new ThreadLocal<AppiumDriver>();
public void setDriver(String deviceName, String platformVersion)
throws MalformedURLException, InterruptedException {
// TODO Auto-generated method stub
File classpathRoot = new File(System.getProperty("user.dir"));
File appDir = new File(classpathRoot, "Apps");
File app = new File(appDir, "android-debug.apk");
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.BROWSER_NAME, "");
capabilities.setCapability("deviceName", deviceName);
capabilities.setCapability("platformVersion", platformVersion);
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("app", app.getAbsolutePath());
if (deviceName.equals("Nexus6")) {
driverThread.set(new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities));
} else if (deviceName.equals("Nexus7")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4724/wd/hub"), capabilities));
} else if (deviceName.equals("Lenovo")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4723/wd/hub"), capabilities));
} else {
System.out.println("Check the device name and platformversion");
}
}
public static AppiumDriver getDriver() {
return driverThread.get();
}
public static void closeDriver() {
if (!getDriver().equals(null)) {
getDriver().quit();
}
}
}
Second: Create a object class for the particular page using PageFactory methodology.
package com.vg.ui.pageobjects;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LandingPageObject {
@FindBy(xpath = "//*[@text='Account Create']")
WebElement btn_accountCreate;
public void click_AccountCreate(){
/*driverUtil.clickandWait(btn_accountCreate);*/
btn_accountCreate.click();
}
}
Third: Then in your step definition class extend the Mobile driver class like below with the PageFactory of respective screens.
package com.vg.ui.stepdefinitions;
import org.openqa.selenium.support.PageFactory;
import com.vg.ui.pageobjects.LandingPageObject;
import com.vg.ui.utils.mobile.Mobile;
import cucumber.api.java.en.When;
public class LandingPage extends Mobile{
LandingPageObject lp = PageFactory.initElements(getDriver(), LandingPageObject.class);
@When("^click on the button account create\.$")
public void click_on_the_button_account_create() throws Throwable {
// Write code here that turns the phrase above into concrete actions
lp.click_AccountCreate();
}
}
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
add a comment |
First define your page as follow:
public class WelcomeScreen{
@AndroidFindBy(accessibility = "") //you can use id, accessibility-id or xpath
@iOSFindBy(accessibility = "")
private MobileElement element1;
@AndroidFindBy(accessibility = "")
@iOSFindBy(accessibility = "")
private MobileElement element2;
AppiumDriver<MoblieElement> driver;
public WelcomeScreen(AppiumDriver<MobileElement> driver) {
this.driver=driver;
PageFactory.initElements(new AppiumFieldDecorator(driver), this);
}
public void clickElement2(){
element2.click()
}
}
Then setup the DesiredCapabilities and AppiumDriver.
After that use page object model in other class.
WelcomeScreen screen=new WelcomeScreen(driver);
screen.clickElement2();
Make sure your driver is global.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290266%2fwhat-are-the-best-practices-for-appium-framework-using-page-opject-model%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
First you have to create base class which load the driver.
Note: If you are using thread local, we can achieve the parallel execution without any issues
package com.vg.ui.utils.mobile;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.android.AndroidDriver;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Mobile {
public static ThreadLocal<AppiumDriver> driverThread = new ThreadLocal<AppiumDriver>();
public void setDriver(String deviceName, String platformVersion)
throws MalformedURLException, InterruptedException {
// TODO Auto-generated method stub
File classpathRoot = new File(System.getProperty("user.dir"));
File appDir = new File(classpathRoot, "Apps");
File app = new File(appDir, "android-debug.apk");
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.BROWSER_NAME, "");
capabilities.setCapability("deviceName", deviceName);
capabilities.setCapability("platformVersion", platformVersion);
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("app", app.getAbsolutePath());
if (deviceName.equals("Nexus6")) {
driverThread.set(new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities));
} else if (deviceName.equals("Nexus7")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4724/wd/hub"), capabilities));
} else if (deviceName.equals("Lenovo")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4723/wd/hub"), capabilities));
} else {
System.out.println("Check the device name and platformversion");
}
}
public static AppiumDriver getDriver() {
return driverThread.get();
}
public static void closeDriver() {
if (!getDriver().equals(null)) {
getDriver().quit();
}
}
}
Second: Create a object class for the particular page using PageFactory methodology.
package com.vg.ui.pageobjects;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LandingPageObject {
@FindBy(xpath = "//*[@text='Account Create']")
WebElement btn_accountCreate;
public void click_AccountCreate(){
/*driverUtil.clickandWait(btn_accountCreate);*/
btn_accountCreate.click();
}
}
Third: Then in your step definition class extend the Mobile driver class like below with the PageFactory of respective screens.
package com.vg.ui.stepdefinitions;
import org.openqa.selenium.support.PageFactory;
import com.vg.ui.pageobjects.LandingPageObject;
import com.vg.ui.utils.mobile.Mobile;
import cucumber.api.java.en.When;
public class LandingPage extends Mobile{
LandingPageObject lp = PageFactory.initElements(getDriver(), LandingPageObject.class);
@When("^click on the button account create\.$")
public void click_on_the_button_account_create() throws Throwable {
// Write code here that turns the phrase above into concrete actions
lp.click_AccountCreate();
}
}
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
add a comment |
First you have to create base class which load the driver.
Note: If you are using thread local, we can achieve the parallel execution without any issues
package com.vg.ui.utils.mobile;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.android.AndroidDriver;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Mobile {
public static ThreadLocal<AppiumDriver> driverThread = new ThreadLocal<AppiumDriver>();
public void setDriver(String deviceName, String platformVersion)
throws MalformedURLException, InterruptedException {
// TODO Auto-generated method stub
File classpathRoot = new File(System.getProperty("user.dir"));
File appDir = new File(classpathRoot, "Apps");
File app = new File(appDir, "android-debug.apk");
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.BROWSER_NAME, "");
capabilities.setCapability("deviceName", deviceName);
capabilities.setCapability("platformVersion", platformVersion);
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("app", app.getAbsolutePath());
if (deviceName.equals("Nexus6")) {
driverThread.set(new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities));
} else if (deviceName.equals("Nexus7")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4724/wd/hub"), capabilities));
} else if (deviceName.equals("Lenovo")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4723/wd/hub"), capabilities));
} else {
System.out.println("Check the device name and platformversion");
}
}
public static AppiumDriver getDriver() {
return driverThread.get();
}
public static void closeDriver() {
if (!getDriver().equals(null)) {
getDriver().quit();
}
}
}
Second: Create a object class for the particular page using PageFactory methodology.
package com.vg.ui.pageobjects;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LandingPageObject {
@FindBy(xpath = "//*[@text='Account Create']")
WebElement btn_accountCreate;
public void click_AccountCreate(){
/*driverUtil.clickandWait(btn_accountCreate);*/
btn_accountCreate.click();
}
}
Third: Then in your step definition class extend the Mobile driver class like below with the PageFactory of respective screens.
package com.vg.ui.stepdefinitions;
import org.openqa.selenium.support.PageFactory;
import com.vg.ui.pageobjects.LandingPageObject;
import com.vg.ui.utils.mobile.Mobile;
import cucumber.api.java.en.When;
public class LandingPage extends Mobile{
LandingPageObject lp = PageFactory.initElements(getDriver(), LandingPageObject.class);
@When("^click on the button account create\.$")
public void click_on_the_button_account_create() throws Throwable {
// Write code here that turns the phrase above into concrete actions
lp.click_AccountCreate();
}
}
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
add a comment |
First you have to create base class which load the driver.
Note: If you are using thread local, we can achieve the parallel execution without any issues
package com.vg.ui.utils.mobile;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.android.AndroidDriver;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Mobile {
public static ThreadLocal<AppiumDriver> driverThread = new ThreadLocal<AppiumDriver>();
public void setDriver(String deviceName, String platformVersion)
throws MalformedURLException, InterruptedException {
// TODO Auto-generated method stub
File classpathRoot = new File(System.getProperty("user.dir"));
File appDir = new File(classpathRoot, "Apps");
File app = new File(appDir, "android-debug.apk");
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.BROWSER_NAME, "");
capabilities.setCapability("deviceName", deviceName);
capabilities.setCapability("platformVersion", platformVersion);
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("app", app.getAbsolutePath());
if (deviceName.equals("Nexus6")) {
driverThread.set(new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities));
} else if (deviceName.equals("Nexus7")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4724/wd/hub"), capabilities));
} else if (deviceName.equals("Lenovo")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4723/wd/hub"), capabilities));
} else {
System.out.println("Check the device name and platformversion");
}
}
public static AppiumDriver getDriver() {
return driverThread.get();
}
public static void closeDriver() {
if (!getDriver().equals(null)) {
getDriver().quit();
}
}
}
Second: Create a object class for the particular page using PageFactory methodology.
package com.vg.ui.pageobjects;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LandingPageObject {
@FindBy(xpath = "//*[@text='Account Create']")
WebElement btn_accountCreate;
public void click_AccountCreate(){
/*driverUtil.clickandWait(btn_accountCreate);*/
btn_accountCreate.click();
}
}
Third: Then in your step definition class extend the Mobile driver class like below with the PageFactory of respective screens.
package com.vg.ui.stepdefinitions;
import org.openqa.selenium.support.PageFactory;
import com.vg.ui.pageobjects.LandingPageObject;
import com.vg.ui.utils.mobile.Mobile;
import cucumber.api.java.en.When;
public class LandingPage extends Mobile{
LandingPageObject lp = PageFactory.initElements(getDriver(), LandingPageObject.class);
@When("^click on the button account create\.$")
public void click_on_the_button_account_create() throws Throwable {
// Write code here that turns the phrase above into concrete actions
lp.click_AccountCreate();
}
}
First you have to create base class which load the driver.
Note: If you are using thread local, we can achieve the parallel execution without any issues
package com.vg.ui.utils.mobile;
import io.appium.java_client.AppiumDriver;
import io.appium.java_client.android.AndroidDriver;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import org.openqa.selenium.remote.CapabilityType;
import org.openqa.selenium.remote.DesiredCapabilities;
public class Mobile {
public static ThreadLocal<AppiumDriver> driverThread = new ThreadLocal<AppiumDriver>();
public void setDriver(String deviceName, String platformVersion)
throws MalformedURLException, InterruptedException {
// TODO Auto-generated method stub
File classpathRoot = new File(System.getProperty("user.dir"));
File appDir = new File(classpathRoot, "Apps");
File app = new File(appDir, "android-debug.apk");
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(CapabilityType.BROWSER_NAME, "");
capabilities.setCapability("deviceName", deviceName);
capabilities.setCapability("platformVersion", platformVersion);
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("app", app.getAbsolutePath());
if (deviceName.equals("Nexus6")) {
driverThread.set(new AndroidDriver(new URL("http://127.0.0.1:4723/wd/hub"), capabilities));
} else if (deviceName.equals("Nexus7")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4724/wd/hub"), capabilities));
} else if (deviceName.equals("Lenovo")) {
driverThread.set(new AndroidDriver(new URL(
"http://127.0.0.1:4723/wd/hub"), capabilities));
} else {
System.out.println("Check the device name and platformversion");
}
}
public static AppiumDriver getDriver() {
return driverThread.get();
}
public static void closeDriver() {
if (!getDriver().equals(null)) {
getDriver().quit();
}
}
}
Second: Create a object class for the particular page using PageFactory methodology.
package com.vg.ui.pageobjects;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.support.FindBy;
public class LandingPageObject {
@FindBy(xpath = "//*[@text='Account Create']")
WebElement btn_accountCreate;
public void click_AccountCreate(){
/*driverUtil.clickandWait(btn_accountCreate);*/
btn_accountCreate.click();
}
}
Third: Then in your step definition class extend the Mobile driver class like below with the PageFactory of respective screens.
package com.vg.ui.stepdefinitions;
import org.openqa.selenium.support.PageFactory;
import com.vg.ui.pageobjects.LandingPageObject;
import com.vg.ui.utils.mobile.Mobile;
import cucumber.api.java.en.When;
public class LandingPage extends Mobile{
LandingPageObject lp = PageFactory.initElements(getDriver(), LandingPageObject.class);
@When("^click on the button account create\.$")
public void click_on_the_button_account_create() throws Throwable {
// Write code here that turns the phrase above into concrete actions
lp.click_AccountCreate();
}
}
answered Nov 14 '18 at 11:28


Balakrishnan ABalakrishnan A
1215
1215
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
add a comment |
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Would you please explain more about PageFactory, how it works?
– Hamid
Nov 15 '18 at 20:36
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
Please refer this links. guru99.com/…
– Balakrishnan A
Nov 19 '18 at 7:02
add a comment |
First define your page as follow:
public class WelcomeScreen{
@AndroidFindBy(accessibility = "") //you can use id, accessibility-id or xpath
@iOSFindBy(accessibility = "")
private MobileElement element1;
@AndroidFindBy(accessibility = "")
@iOSFindBy(accessibility = "")
private MobileElement element2;
AppiumDriver<MoblieElement> driver;
public WelcomeScreen(AppiumDriver<MobileElement> driver) {
this.driver=driver;
PageFactory.initElements(new AppiumFieldDecorator(driver), this);
}
public void clickElement2(){
element2.click()
}
}
Then setup the DesiredCapabilities and AppiumDriver.
After that use page object model in other class.
WelcomeScreen screen=new WelcomeScreen(driver);
screen.clickElement2();
Make sure your driver is global.
add a comment |
First define your page as follow:
public class WelcomeScreen{
@AndroidFindBy(accessibility = "") //you can use id, accessibility-id or xpath
@iOSFindBy(accessibility = "")
private MobileElement element1;
@AndroidFindBy(accessibility = "")
@iOSFindBy(accessibility = "")
private MobileElement element2;
AppiumDriver<MoblieElement> driver;
public WelcomeScreen(AppiumDriver<MobileElement> driver) {
this.driver=driver;
PageFactory.initElements(new AppiumFieldDecorator(driver), this);
}
public void clickElement2(){
element2.click()
}
}
Then setup the DesiredCapabilities and AppiumDriver.
After that use page object model in other class.
WelcomeScreen screen=new WelcomeScreen(driver);
screen.clickElement2();
Make sure your driver is global.
add a comment |
First define your page as follow:
public class WelcomeScreen{
@AndroidFindBy(accessibility = "") //you can use id, accessibility-id or xpath
@iOSFindBy(accessibility = "")
private MobileElement element1;
@AndroidFindBy(accessibility = "")
@iOSFindBy(accessibility = "")
private MobileElement element2;
AppiumDriver<MoblieElement> driver;
public WelcomeScreen(AppiumDriver<MobileElement> driver) {
this.driver=driver;
PageFactory.initElements(new AppiumFieldDecorator(driver), this);
}
public void clickElement2(){
element2.click()
}
}
Then setup the DesiredCapabilities and AppiumDriver.
After that use page object model in other class.
WelcomeScreen screen=new WelcomeScreen(driver);
screen.clickElement2();
Make sure your driver is global.
First define your page as follow:
public class WelcomeScreen{
@AndroidFindBy(accessibility = "") //you can use id, accessibility-id or xpath
@iOSFindBy(accessibility = "")
private MobileElement element1;
@AndroidFindBy(accessibility = "")
@iOSFindBy(accessibility = "")
private MobileElement element2;
AppiumDriver<MoblieElement> driver;
public WelcomeScreen(AppiumDriver<MobileElement> driver) {
this.driver=driver;
PageFactory.initElements(new AppiumFieldDecorator(driver), this);
}
public void clickElement2(){
element2.click()
}
}
Then setup the DesiredCapabilities and AppiumDriver.
After that use page object model in other class.
WelcomeScreen screen=new WelcomeScreen(driver);
screen.clickElement2();
Make sure your driver is global.
answered Nov 14 '18 at 5:59


Suban DhyakoSuban Dhyako
658417
658417
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290266%2fwhat-are-the-best-practices-for-appium-framework-using-page-opject-model%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N3Y1rSBc4V MqFNiSgSoAANeXOV,4rfYnSC8qgkslc9ysZ