How to delete all nodes in circular doubly linked list?
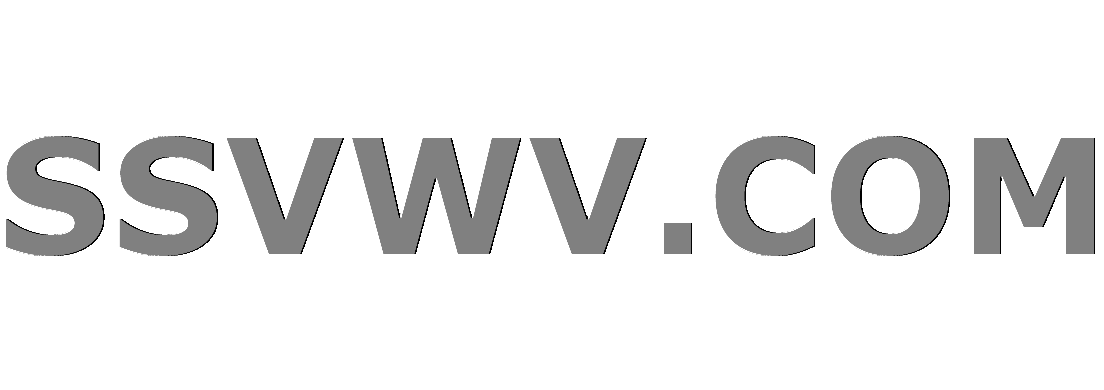
Multi tool use
I've got a problem, because I don't know how to delete all nodes in that kind of list.
What do you think about my solution? Debugger shows me a problem "(...) x was 0xDDDDDDDD"
void del(List *&head) {
List* ptr = head;
List* help;
do {
List *x = ptr;
List *y = ptr;
x = y;
help = x;
x = x->next;
delete y;
head = NULL;
ptr = ptr->next;
} while (help!=NULL);
c++
|
show 3 more comments
I've got a problem, because I don't know how to delete all nodes in that kind of list.
What do you think about my solution? Debugger shows me a problem "(...) x was 0xDDDDDDDD"
void del(List *&head) {
List* ptr = head;
List* help;
do {
List *x = ptr;
List *y = ptr;
x = y;
help = x;
x = x->next;
delete y;
head = NULL;
ptr = ptr->next;
} while (help!=NULL);
c++
1
Onlydelete
what younew
. How is yourList
allocated? Please provide Minimal, Complete, and Verifiable example.
– Algirdas Preidžius
Nov 12 '18 at 13:16
1
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
1
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
2
Removing the unnecessary variable juggling, your loop is equivalent todo { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?
– molbdnilo
Nov 12 '18 at 13:34
|
show 3 more comments
I've got a problem, because I don't know how to delete all nodes in that kind of list.
What do you think about my solution? Debugger shows me a problem "(...) x was 0xDDDDDDDD"
void del(List *&head) {
List* ptr = head;
List* help;
do {
List *x = ptr;
List *y = ptr;
x = y;
help = x;
x = x->next;
delete y;
head = NULL;
ptr = ptr->next;
} while (help!=NULL);
c++
I've got a problem, because I don't know how to delete all nodes in that kind of list.
What do you think about my solution? Debugger shows me a problem "(...) x was 0xDDDDDDDD"
void del(List *&head) {
List* ptr = head;
List* help;
do {
List *x = ptr;
List *y = ptr;
x = y;
help = x;
x = x->next;
delete y;
head = NULL;
ptr = ptr->next;
} while (help!=NULL);
c++
c++
edited Nov 12 '18 at 13:23
asked Nov 12 '18 at 13:13


user76234
183
183
1
Onlydelete
what younew
. How is yourList
allocated? Please provide Minimal, Complete, and Verifiable example.
– Algirdas Preidžius
Nov 12 '18 at 13:16
1
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
1
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
2
Removing the unnecessary variable juggling, your loop is equivalent todo { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?
– molbdnilo
Nov 12 '18 at 13:34
|
show 3 more comments
1
Onlydelete
what younew
. How is yourList
allocated? Please provide Minimal, Complete, and Verifiable example.
– Algirdas Preidžius
Nov 12 '18 at 13:16
1
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
1
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
2
Removing the unnecessary variable juggling, your loop is equivalent todo { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?
– molbdnilo
Nov 12 '18 at 13:34
1
1
Only
delete
what you new
. How is your List
allocated? Please provide Minimal, Complete, and Verifiable example.– Algirdas Preidžius
Nov 12 '18 at 13:16
Only
delete
what you new
. How is your List
allocated? Please provide Minimal, Complete, and Verifiable example.– Algirdas Preidžius
Nov 12 '18 at 13:16
1
1
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
1
1
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
2
2
Removing the unnecessary variable juggling, your loop is equivalent to
do { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?– molbdnilo
Nov 12 '18 at 13:34
Removing the unnecessary variable juggling, your loop is equivalent to
do { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?– molbdnilo
Nov 12 '18 at 13:34
|
show 3 more comments
2 Answers
2
active
oldest
votes
Here is the complete example with the test
struct Node
{
Node(Node* prev, int val)
: prev(prev), next(NULL), value(val)
{ }
Node *prev, *next;
int value;
};
void clear_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head->next;
while (curr != NULL && curr != head)
{
std::cout << "Deleting " << curr->value << std::endl;
Node* temp = curr;
curr = curr->next;
delete temp;
};
delete head;
head = NULL;
}
}
void print_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head;
do
{
std::cout << (curr == head ? "Head: " : "") << curr->value << std::endl;
curr = curr->next;
} while (curr != NULL && curr != head);
}
}
int main()
{
Node* head = new Node(NULL, 0);
Node* curr = head;
for (int i = 1; i <= 10; i++)
{
Node* prev = curr;
curr = new Node(prev, i);
prev->next = curr;
}
// Link end to head
curr->next = head;
//
print_list(head);
clear_list(head);
print_list(head);
}
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
add a comment |
List *y = ptr;
delete y;
ptr = ptr->next;
The memory allocated at ptr is gone after delete y
. You can not call ptr->next
as ptr is dangling at this point and de-referencing it is undefined behavior. Try reordering the last two lines i.e.
ptr = ptr->next;
delete y;
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53262943%2fhow-to-delete-all-nodes-in-circular-doubly-linked-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is the complete example with the test
struct Node
{
Node(Node* prev, int val)
: prev(prev), next(NULL), value(val)
{ }
Node *prev, *next;
int value;
};
void clear_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head->next;
while (curr != NULL && curr != head)
{
std::cout << "Deleting " << curr->value << std::endl;
Node* temp = curr;
curr = curr->next;
delete temp;
};
delete head;
head = NULL;
}
}
void print_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head;
do
{
std::cout << (curr == head ? "Head: " : "") << curr->value << std::endl;
curr = curr->next;
} while (curr != NULL && curr != head);
}
}
int main()
{
Node* head = new Node(NULL, 0);
Node* curr = head;
for (int i = 1; i <= 10; i++)
{
Node* prev = curr;
curr = new Node(prev, i);
prev->next = curr;
}
// Link end to head
curr->next = head;
//
print_list(head);
clear_list(head);
print_list(head);
}
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
add a comment |
Here is the complete example with the test
struct Node
{
Node(Node* prev, int val)
: prev(prev), next(NULL), value(val)
{ }
Node *prev, *next;
int value;
};
void clear_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head->next;
while (curr != NULL && curr != head)
{
std::cout << "Deleting " << curr->value << std::endl;
Node* temp = curr;
curr = curr->next;
delete temp;
};
delete head;
head = NULL;
}
}
void print_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head;
do
{
std::cout << (curr == head ? "Head: " : "") << curr->value << std::endl;
curr = curr->next;
} while (curr != NULL && curr != head);
}
}
int main()
{
Node* head = new Node(NULL, 0);
Node* curr = head;
for (int i = 1; i <= 10; i++)
{
Node* prev = curr;
curr = new Node(prev, i);
prev->next = curr;
}
// Link end to head
curr->next = head;
//
print_list(head);
clear_list(head);
print_list(head);
}
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
add a comment |
Here is the complete example with the test
struct Node
{
Node(Node* prev, int val)
: prev(prev), next(NULL), value(val)
{ }
Node *prev, *next;
int value;
};
void clear_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head->next;
while (curr != NULL && curr != head)
{
std::cout << "Deleting " << curr->value << std::endl;
Node* temp = curr;
curr = curr->next;
delete temp;
};
delete head;
head = NULL;
}
}
void print_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head;
do
{
std::cout << (curr == head ? "Head: " : "") << curr->value << std::endl;
curr = curr->next;
} while (curr != NULL && curr != head);
}
}
int main()
{
Node* head = new Node(NULL, 0);
Node* curr = head;
for (int i = 1; i <= 10; i++)
{
Node* prev = curr;
curr = new Node(prev, i);
prev->next = curr;
}
// Link end to head
curr->next = head;
//
print_list(head);
clear_list(head);
print_list(head);
}
Here is the complete example with the test
struct Node
{
Node(Node* prev, int val)
: prev(prev), next(NULL), value(val)
{ }
Node *prev, *next;
int value;
};
void clear_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head->next;
while (curr != NULL && curr != head)
{
std::cout << "Deleting " << curr->value << std::endl;
Node* temp = curr;
curr = curr->next;
delete temp;
};
delete head;
head = NULL;
}
}
void print_list(Node*& head)
{
if (head != NULL)
{
Node* curr = head;
do
{
std::cout << (curr == head ? "Head: " : "") << curr->value << std::endl;
curr = curr->next;
} while (curr != NULL && curr != head);
}
}
int main()
{
Node* head = new Node(NULL, 0);
Node* curr = head;
for (int i = 1; i <= 10; i++)
{
Node* prev = curr;
curr = new Node(prev, i);
prev->next = curr;
}
// Link end to head
curr->next = head;
//
print_list(head);
clear_list(head);
print_list(head);
}
answered Nov 12 '18 at 14:08
serge
59537
59537
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
add a comment |
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
thank you so much!
– user76234
Nov 12 '18 at 14:32
thank you so much!
– user76234
Nov 12 '18 at 14:32
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
@user76234 you are welcome, don't hesitate to mark the answer as solution if it helps.
– serge
Nov 12 '18 at 15:54
add a comment |
List *y = ptr;
delete y;
ptr = ptr->next;
The memory allocated at ptr is gone after delete y
. You can not call ptr->next
as ptr is dangling at this point and de-referencing it is undefined behavior. Try reordering the last two lines i.e.
ptr = ptr->next;
delete y;
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
add a comment |
List *y = ptr;
delete y;
ptr = ptr->next;
The memory allocated at ptr is gone after delete y
. You can not call ptr->next
as ptr is dangling at this point and de-referencing it is undefined behavior. Try reordering the last two lines i.e.
ptr = ptr->next;
delete y;
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
add a comment |
List *y = ptr;
delete y;
ptr = ptr->next;
The memory allocated at ptr is gone after delete y
. You can not call ptr->next
as ptr is dangling at this point and de-referencing it is undefined behavior. Try reordering the last two lines i.e.
ptr = ptr->next;
delete y;
List *y = ptr;
delete y;
ptr = ptr->next;
The memory allocated at ptr is gone after delete y
. You can not call ptr->next
as ptr is dangling at this point and de-referencing it is undefined behavior. Try reordering the last two lines i.e.
ptr = ptr->next;
delete y;
answered Nov 12 '18 at 13:53
Manoj R
2,67211329
2,67211329
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
add a comment |
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
Ok, now I understand, thank you.
– user76234
Nov 12 '18 at 14:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53262943%2fhow-to-delete-all-nodes-in-circular-doubly-linked-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QbJHc P9cUIgVN
1
Only
delete
what younew
. How is yourList
allocated? Please provide Minimal, Complete, and Verifiable example.– Algirdas Preidžius
Nov 12 '18 at 13:16
1
Please give your variables names that make sense. See: stackoverflow.com/a/853187/3212865
– spectras
Nov 12 '18 at 13:20
1
You have a node pointer called "help"? What does that signify?
– Lightness Races in Orbit
Nov 12 '18 at 13:24
There does not seem to be a need for so many variables. Could you please clarify what is your intended purpose for each?
– GoodDeeds
Nov 12 '18 at 13:29
2
Removing the unnecessary variable juggling, your loop is equivalent to
do { help = ptr; delete ptr; ptr = ptr->next; } while (help != NULL);
. Can you spot the bad dereference?– molbdnilo
Nov 12 '18 at 13:34