How to bind the event in html template?
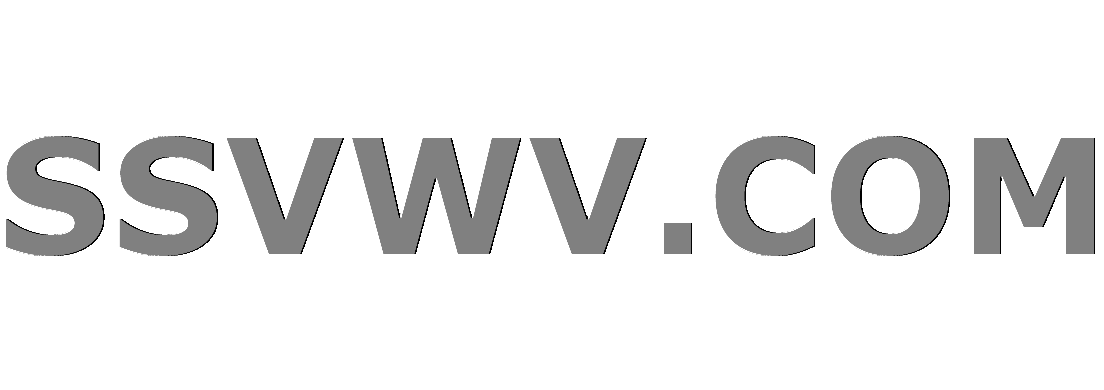
Multi tool use
Please refer to my previous question: How to get element in template html?.
Firstly, I follow the solution in How to get element in template html? to obtain the element.
var template = templateContent.content;
Then I try to bind a click
event for searchBtn
:
searchBtn.addEventListener('click', function() {
console.log('click'); // does not work
});
This binding fails, so I use the event delegation to handle event:
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click');
console.log(template.querySelector('#results'));
template.querySelector('#results').innerText = 'Foo';
}
});
The two logs are expected, but the last command to update the innerText
does not work. On the other hand, I can update its content outside the click event. Any solution?
The final version of my code:
var link = document.querySelector('link[rel="import"][href="search.html"]');
var templateContent = link.import.querySelector('template');
var template = templateContent.content;
var searchBtn = template.querySelector('#searchBtn');
console.log(searchBtn);
template.querySelector('#results').innerText = 'Bar'; // ok
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
template.querySelector('#results').innerText = 'Foo'; // does not work
}
});
Updates
Now I retrieve the results
element outside the function:
var results = template.querySelector('#results');
Then inside the function, results.innerText = 'Foo';
works. However, this only updates the results
itself, and the UI is not updated correspondingly.
How to update the UI inside the template?
javascript html
|
show 4 more comments
Please refer to my previous question: How to get element in template html?.
Firstly, I follow the solution in How to get element in template html? to obtain the element.
var template = templateContent.content;
Then I try to bind a click
event for searchBtn
:
searchBtn.addEventListener('click', function() {
console.log('click'); // does not work
});
This binding fails, so I use the event delegation to handle event:
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click');
console.log(template.querySelector('#results'));
template.querySelector('#results').innerText = 'Foo';
}
});
The two logs are expected, but the last command to update the innerText
does not work. On the other hand, I can update its content outside the click event. Any solution?
The final version of my code:
var link = document.querySelector('link[rel="import"][href="search.html"]');
var templateContent = link.import.querySelector('template');
var template = templateContent.content;
var searchBtn = template.querySelector('#searchBtn');
console.log(searchBtn);
template.querySelector('#results').innerText = 'Bar'; // ok
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
template.querySelector('#results').innerText = 'Foo'; // does not work
}
});
Updates
Now I retrieve the results
element outside the function:
var results = template.querySelector('#results');
Then inside the function, results.innerText = 'Foo';
works. However, this only updates the results
itself, and the UI is not updated correspondingly.
How to update the UI inside the template?
javascript html
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
@NarendraMongiya I log theresults.innerText
inside the click function, and it is updated. But the nothing happens in the UI.
– chenzhongpu
Nov 13 '18 at 6:12
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16
|
show 4 more comments
Please refer to my previous question: How to get element in template html?.
Firstly, I follow the solution in How to get element in template html? to obtain the element.
var template = templateContent.content;
Then I try to bind a click
event for searchBtn
:
searchBtn.addEventListener('click', function() {
console.log('click'); // does not work
});
This binding fails, so I use the event delegation to handle event:
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click');
console.log(template.querySelector('#results'));
template.querySelector('#results').innerText = 'Foo';
}
});
The two logs are expected, but the last command to update the innerText
does not work. On the other hand, I can update its content outside the click event. Any solution?
The final version of my code:
var link = document.querySelector('link[rel="import"][href="search.html"]');
var templateContent = link.import.querySelector('template');
var template = templateContent.content;
var searchBtn = template.querySelector('#searchBtn');
console.log(searchBtn);
template.querySelector('#results').innerText = 'Bar'; // ok
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
template.querySelector('#results').innerText = 'Foo'; // does not work
}
});
Updates
Now I retrieve the results
element outside the function:
var results = template.querySelector('#results');
Then inside the function, results.innerText = 'Foo';
works. However, this only updates the results
itself, and the UI is not updated correspondingly.
How to update the UI inside the template?
javascript html
Please refer to my previous question: How to get element in template html?.
Firstly, I follow the solution in How to get element in template html? to obtain the element.
var template = templateContent.content;
Then I try to bind a click
event for searchBtn
:
searchBtn.addEventListener('click', function() {
console.log('click'); // does not work
});
This binding fails, so I use the event delegation to handle event:
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click');
console.log(template.querySelector('#results'));
template.querySelector('#results').innerText = 'Foo';
}
});
The two logs are expected, but the last command to update the innerText
does not work. On the other hand, I can update its content outside the click event. Any solution?
The final version of my code:
var link = document.querySelector('link[rel="import"][href="search.html"]');
var templateContent = link.import.querySelector('template');
var template = templateContent.content;
var searchBtn = template.querySelector('#searchBtn');
console.log(searchBtn);
template.querySelector('#results').innerText = 'Bar'; // ok
document.addEventListener('click',function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
template.querySelector('#results').innerText = 'Foo'; // does not work
}
});
Updates
Now I retrieve the results
element outside the function:
var results = template.querySelector('#results');
Then inside the function, results.innerText = 'Foo';
works. However, this only updates the results
itself, and the UI is not updated correspondingly.
How to update the UI inside the template?
javascript html
javascript html
edited Nov 13 '18 at 5:58
chenzhongpu
asked Nov 13 '18 at 3:48


chenzhongpuchenzhongpu
2,25732451
2,25732451
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
@NarendraMongiya I log theresults.innerText
inside the click function, and it is updated. But the nothing happens in the UI.
– chenzhongpu
Nov 13 '18 at 6:12
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16
|
show 4 more comments
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
@NarendraMongiya I log theresults.innerText
inside the click function, and it is updated. But the nothing happens in the UI.
– chenzhongpu
Nov 13 '18 at 6:12
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
@NarendraMongiya I log the
results.innerText
inside the click function, and it is updated. But the nothing happens in the UI.– chenzhongpu
Nov 13 '18 at 6:12
@NarendraMongiya I log the
results.innerText
inside the click function, and it is updated. But the nothing happens in the UI.– chenzhongpu
Nov 13 '18 at 6:12
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16
|
show 4 more comments
3 Answers
3
active
oldest
votes
You got it right, it is the binding issue. template is not available inside click function. You need to bind this. There are many ways to do this e.g.
document.addEventListener('click',onClick.bind(this));
onClick = function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
this.template.querySelector('#results').innerText = 'Foo'; // should work
}
}
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. Theresults
value is updated, but the UI remains unchanged.
– chenzhongpu
Nov 13 '18 at 5:59
add a comment |
There are many ways to get the HTML elements
You can get use
var sbtnElement = document.getElementById('searchBtn');
then add eventlistener to it
sbtnElement.addEventlistener('click', function() {
console.log('click'); // does not work
});
Naturally getElement by id will give you one element, and getElementsByClassName will return you an array of elements with the same classname. Same goes for getElementsByTagName.
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
add a comment |
hi i don't know what are you try to create for. but have you ever try to use template in script ? like this one
<script id="sampleTemplate" type="text/template">
// do the html here
</script>
So in that case you can bind event using that one. just a normal creating of html and binding javascript function.
You can refer to this blog. https://jonsuh.com/blog/javascript-templating-without-a-library/
I hope, i helped you thru this. :)
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273505%2fhow-to-bind-the-event-in-html-template%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You got it right, it is the binding issue. template is not available inside click function. You need to bind this. There are many ways to do this e.g.
document.addEventListener('click',onClick.bind(this));
onClick = function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
this.template.querySelector('#results').innerText = 'Foo'; // should work
}
}
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. Theresults
value is updated, but the UI remains unchanged.
– chenzhongpu
Nov 13 '18 at 5:59
add a comment |
You got it right, it is the binding issue. template is not available inside click function. You need to bind this. There are many ways to do this e.g.
document.addEventListener('click',onClick.bind(this));
onClick = function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
this.template.querySelector('#results').innerText = 'Foo'; // should work
}
}
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. Theresults
value is updated, but the UI remains unchanged.
– chenzhongpu
Nov 13 '18 at 5:59
add a comment |
You got it right, it is the binding issue. template is not available inside click function. You need to bind this. There are many ways to do this e.g.
document.addEventListener('click',onClick.bind(this));
onClick = function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
this.template.querySelector('#results').innerText = 'Foo'; // should work
}
}
You got it right, it is the binding issue. template is not available inside click function. You need to bind this. There are many ways to do this e.g.
document.addEventListener('click',onClick.bind(this));
onClick = function(e){
if(e.target && e.target.id== 'searchBtn'){
console.log('click'); // ok
console.log(template.querySelector('#results')); // ok
this.template.querySelector('#results').innerText = 'Foo'; // should work
}
}
edited Nov 13 '18 at 5:52
answered Nov 13 '18 at 4:30
Narendra MongiyaNarendra Mongiya
1,470719
1,470719
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. Theresults
value is updated, but the UI remains unchanged.
– chenzhongpu
Nov 13 '18 at 5:59
add a comment |
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. Theresults
value is updated, but the UI remains unchanged.
– chenzhongpu
Nov 13 '18 at 5:59
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
double functions?
– chenzhongpu
Nov 13 '18 at 5:35
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
that was the typo, edited the answer.
– Narendra Mongiya
Nov 13 '18 at 5:52
Please see my updated question. The
results
value is updated, but the UI remains unchanged.– chenzhongpu
Nov 13 '18 at 5:59
Please see my updated question. The
results
value is updated, but the UI remains unchanged.– chenzhongpu
Nov 13 '18 at 5:59
add a comment |
There are many ways to get the HTML elements
You can get use
var sbtnElement = document.getElementById('searchBtn');
then add eventlistener to it
sbtnElement.addEventlistener('click', function() {
console.log('click'); // does not work
});
Naturally getElement by id will give you one element, and getElementsByClassName will return you an array of elements with the same classname. Same goes for getElementsByTagName.
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
add a comment |
There are many ways to get the HTML elements
You can get use
var sbtnElement = document.getElementById('searchBtn');
then add eventlistener to it
sbtnElement.addEventlistener('click', function() {
console.log('click'); // does not work
});
Naturally getElement by id will give you one element, and getElementsByClassName will return you an array of elements with the same classname. Same goes for getElementsByTagName.
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
add a comment |
There are many ways to get the HTML elements
You can get use
var sbtnElement = document.getElementById('searchBtn');
then add eventlistener to it
sbtnElement.addEventlistener('click', function() {
console.log('click'); // does not work
});
Naturally getElement by id will give you one element, and getElementsByClassName will return you an array of elements with the same classname. Same goes for getElementsByTagName.
There are many ways to get the HTML elements
You can get use
var sbtnElement = document.getElementById('searchBtn');
then add eventlistener to it
sbtnElement.addEventlistener('click', function() {
console.log('click'); // does not work
});
Naturally getElement by id will give you one element, and getElementsByClassName will return you an array of elements with the same classname. Same goes for getElementsByTagName.
answered Nov 13 '18 at 4:00


Scott LeeScott Lee
11
11
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
add a comment |
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
You may refer to my previous question: How to get element in template html?. I have to access the element in a template html.
– chenzhongpu
Nov 13 '18 at 4:01
add a comment |
hi i don't know what are you try to create for. but have you ever try to use template in script ? like this one
<script id="sampleTemplate" type="text/template">
// do the html here
</script>
So in that case you can bind event using that one. just a normal creating of html and binding javascript function.
You can refer to this blog. https://jonsuh.com/blog/javascript-templating-without-a-library/
I hope, i helped you thru this. :)
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
add a comment |
hi i don't know what are you try to create for. but have you ever try to use template in script ? like this one
<script id="sampleTemplate" type="text/template">
// do the html here
</script>
So in that case you can bind event using that one. just a normal creating of html and binding javascript function.
You can refer to this blog. https://jonsuh.com/blog/javascript-templating-without-a-library/
I hope, i helped you thru this. :)
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
add a comment |
hi i don't know what are you try to create for. but have you ever try to use template in script ? like this one
<script id="sampleTemplate" type="text/template">
// do the html here
</script>
So in that case you can bind event using that one. just a normal creating of html and binding javascript function.
You can refer to this blog. https://jonsuh.com/blog/javascript-templating-without-a-library/
I hope, i helped you thru this. :)
hi i don't know what are you try to create for. but have you ever try to use template in script ? like this one
<script id="sampleTemplate" type="text/template">
// do the html here
</script>
So in that case you can bind event using that one. just a normal creating of html and binding javascript function.
You can refer to this blog. https://jonsuh.com/blog/javascript-templating-without-a-library/
I hope, i helped you thru this. :)
answered Nov 13 '18 at 3:56


Agent DroidAgent Droid
916
916
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
add a comment |
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
You may refer to my previous question How to get element in template html?. Now I can access the element, but there are some problems with the event bindings.
– chenzhongpu
Nov 13 '18 at 4:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273505%2fhow-to-bind-the-event-in-html-template%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
X1F,3Z N,o,84dFwq,t
What do you mean by 'However, this only updates the results itself, and the UI is not updated correspondingly' ? what is exactly not working here? Have you tried with binding this here as described in answer?
– Narendra Mongiya
Nov 13 '18 at 6:04
this might be useful quirksmode.org/js/events_advanced.html
– Narendra Mongiya
Nov 13 '18 at 6:08
@NarendraMongiya I log the
results.innerText
inside the click function, and it is updated. But the nothing happens in the UI.– chenzhongpu
Nov 13 '18 at 6:12
yes, because that is inside the scope of function.
– Narendra Mongiya
Nov 13 '18 at 6:13
but template does not have any reference inside eventlisterner without binding. The bind() method creates a new function that, when called, has its this keyword set to the provided value, with a given sequence of arguments preceding any provided when the new function is called.
– Narendra Mongiya
Nov 13 '18 at 6:16