Clarity + Angular6: Tree View Node Listener (clr-tree-node)
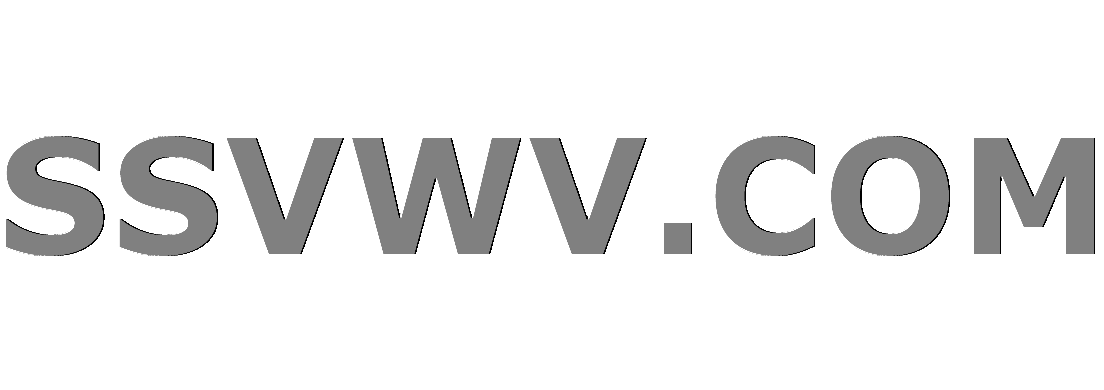
Multi tool use
up vote
2
down vote
favorite
I'm trying to track changes made to the tree nodes data source.
Each node has a selected property which reflects the node selection status, every time I select a node, I need to emit the selection to another component, which will build another tree according to that selection.
I tried with OnChanges interface, but I read later in the doc that ngOnChanges is called only when data is set again.
This is my simple template:
<clr-tree-node [(clrSelected)]="selected" >
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node *ngFor="let asset of assets" [(clrSelected)]="asset.selected">
{{asset.type}}
</clr-tree-node>
</ng-template>
</clr-tree-node>
Is there a tree node event I can bind I can use to emit (with EventEmitter) the changed data? For example onSelectionChange, or onChange or something else?
Or any other mechanism?
Thanks in advance,
Alex.
angular angular6 treenode clarity angular-event-emitter
add a comment |
up vote
2
down vote
favorite
I'm trying to track changes made to the tree nodes data source.
Each node has a selected property which reflects the node selection status, every time I select a node, I need to emit the selection to another component, which will build another tree according to that selection.
I tried with OnChanges interface, but I read later in the doc that ngOnChanges is called only when data is set again.
This is my simple template:
<clr-tree-node [(clrSelected)]="selected" >
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node *ngFor="let asset of assets" [(clrSelected)]="asset.selected">
{{asset.type}}
</clr-tree-node>
</ng-template>
</clr-tree-node>
Is there a tree node event I can bind I can use to emit (with EventEmitter) the changed data? For example onSelectionChange, or onChange or something else?
Or any other mechanism?
Thanks in advance,
Alex.
angular angular6 treenode clarity angular-event-emitter
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm trying to track changes made to the tree nodes data source.
Each node has a selected property which reflects the node selection status, every time I select a node, I need to emit the selection to another component, which will build another tree according to that selection.
I tried with OnChanges interface, but I read later in the doc that ngOnChanges is called only when data is set again.
This is my simple template:
<clr-tree-node [(clrSelected)]="selected" >
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node *ngFor="let asset of assets" [(clrSelected)]="asset.selected">
{{asset.type}}
</clr-tree-node>
</ng-template>
</clr-tree-node>
Is there a tree node event I can bind I can use to emit (with EventEmitter) the changed data? For example onSelectionChange, or onChange or something else?
Or any other mechanism?
Thanks in advance,
Alex.
angular angular6 treenode clarity angular-event-emitter
I'm trying to track changes made to the tree nodes data source.
Each node has a selected property which reflects the node selection status, every time I select a node, I need to emit the selection to another component, which will build another tree according to that selection.
I tried with OnChanges interface, but I read later in the doc that ngOnChanges is called only when data is set again.
This is my simple template:
<clr-tree-node [(clrSelected)]="selected" >
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node *ngFor="let asset of assets" [(clrSelected)]="asset.selected">
{{asset.type}}
</clr-tree-node>
</ng-template>
</clr-tree-node>
Is there a tree node event I can bind I can use to emit (with EventEmitter) the changed data? For example onSelectionChange, or onChange or something else?
Or any other mechanism?
Thanks in advance,
Alex.
angular angular6 treenode clarity angular-event-emitter
angular angular6 treenode clarity angular-event-emitter
asked Nov 10 at 18:46
Alex
726
726
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
As far as I can see, you are already binding to that event. Just use the long form of the two way binding:
template.html
<clr-tree-node [clrSelected]="selected" (clrSelectedChange)="changeSelected($event)">
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node
*ngFor="let asset of assets
[clrSelected]="asset.selected"
(clrSelectedChange)="changeSelectedAsset(asset, $event)"
>{{asset.type}}</clr-tree-node>
</ng-template>
</clr-tree-node>
component.ts
@Component(...)
export class MyComp {
@Output public structureChange:EventEmitter<...> = new EventEmitter<...>();
public changeSelected(data):void {
this.selected = data;
this.structureChange.emit(data);
}
public changeSelectedAsset(asset, data):void {
asset.selected = data;
this.structureChange.emit(this.selected);
}
}
Something like this. Of course, add datatypes wherever possible.
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
As far as I can see, you are already binding to that event. Just use the long form of the two way binding:
template.html
<clr-tree-node [clrSelected]="selected" (clrSelectedChange)="changeSelected($event)">
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node
*ngFor="let asset of assets
[clrSelected]="asset.selected"
(clrSelectedChange)="changeSelectedAsset(asset, $event)"
>{{asset.type}}</clr-tree-node>
</ng-template>
</clr-tree-node>
component.ts
@Component(...)
export class MyComp {
@Output public structureChange:EventEmitter<...> = new EventEmitter<...>();
public changeSelected(data):void {
this.selected = data;
this.structureChange.emit(data);
}
public changeSelectedAsset(asset, data):void {
asset.selected = data;
this.structureChange.emit(this.selected);
}
}
Something like this. Of course, add datatypes wherever possible.
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
add a comment |
up vote
2
down vote
accepted
As far as I can see, you are already binding to that event. Just use the long form of the two way binding:
template.html
<clr-tree-node [clrSelected]="selected" (clrSelectedChange)="changeSelected($event)">
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node
*ngFor="let asset of assets
[clrSelected]="asset.selected"
(clrSelectedChange)="changeSelectedAsset(asset, $event)"
>{{asset.type}}</clr-tree-node>
</ng-template>
</clr-tree-node>
component.ts
@Component(...)
export class MyComp {
@Output public structureChange:EventEmitter<...> = new EventEmitter<...>();
public changeSelected(data):void {
this.selected = data;
this.structureChange.emit(data);
}
public changeSelectedAsset(asset, data):void {
asset.selected = data;
this.structureChange.emit(this.selected);
}
}
Something like this. Of course, add datatypes wherever possible.
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
As far as I can see, you are already binding to that event. Just use the long form of the two way binding:
template.html
<clr-tree-node [clrSelected]="selected" (clrSelectedChange)="changeSelected($event)">
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node
*ngFor="let asset of assets
[clrSelected]="asset.selected"
(clrSelectedChange)="changeSelectedAsset(asset, $event)"
>{{asset.type}}</clr-tree-node>
</ng-template>
</clr-tree-node>
component.ts
@Component(...)
export class MyComp {
@Output public structureChange:EventEmitter<...> = new EventEmitter<...>();
public changeSelected(data):void {
this.selected = data;
this.structureChange.emit(data);
}
public changeSelectedAsset(asset, data):void {
asset.selected = data;
this.structureChange.emit(this.selected);
}
}
Something like this. Of course, add datatypes wherever possible.
As far as I can see, you are already binding to that event. Just use the long form of the two way binding:
template.html
<clr-tree-node [clrSelected]="selected" (clrSelectedChange)="changeSelected($event)">
Asset
<ng-template [clrIfExpanded]="true">
<clr-tree-node
*ngFor="let asset of assets
[clrSelected]="asset.selected"
(clrSelectedChange)="changeSelectedAsset(asset, $event)"
>{{asset.type}}</clr-tree-node>
</ng-template>
</clr-tree-node>
component.ts
@Component(...)
export class MyComp {
@Output public structureChange:EventEmitter<...> = new EventEmitter<...>();
public changeSelected(data):void {
this.selected = data;
this.structureChange.emit(data);
}
public changeSelectedAsset(asset, data):void {
asset.selected = data;
this.structureChange.emit(this.selected);
}
}
Something like this. Of course, add datatypes wherever possible.
answered Nov 10 at 21:30
pascalpuetz
34618
34618
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
add a comment |
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
Ok thanks! I was missing the (clrSelectedChange) binding :-)
– Alex
Nov 11 at 13:01
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242274%2fclarity-angular6-tree-view-node-listener-clr-tree-node%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
C5PtqD4BP1InGC5M1m i2dC QwUg0KaLmaWYh,572K Kw40tT3S jdQ5ial4vkvIO