How to cancel class decorators in constructor
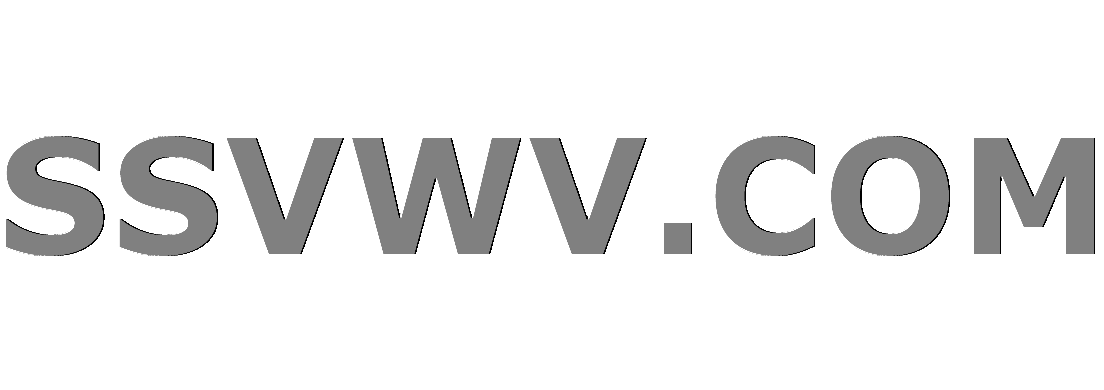
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Lets take the following example of class decorators (origin http://www.informit.com/articles/article.aspx?p=1309289&seqNum=4):
class GenericDescriptor:
def __init__(self, getter, setter):
self.getter = getter
self.setter = setter
def __get__(self, instance, owner=None):
if instance is None:
return self
return self.getter(instance)
def __set__(self, instance, value):
return self.setter(instance, value)
def valid_string(attr_name, empty_allowed=True, regex=None,
acceptable=None):
def decorator(cls):
name = "__" + attr_name
def getter(self):
return getattr(self, name)
def setter(self, value):
assert isinstance(value, str), (attr_name +
" must be a string")
if not empty_allowed and not value:
raise ValueError("{0} may not be empty".format(
attr_name))
if ((acceptable is not None and value not in acceptable) or
(regex is not None and not regex.match(value))):
raise ValueError("{attr_name} cannot be set to "
"{value}".format(**locals()))
setattr(self, name, value)
setattr(cls, attr_name, GenericDescriptor(getter, setter))
return cls
return decorator
@valid_string("name", empty_allowed=False)
class StockItem:
name = None
def __init__(self, **kwargs):
if kwargs.get('second_call'):
pass
# proceed normally without calling @valid_string
self.name = kwargs.get('name', None)
self.price = kwargs.get('price', None)
self.quantity = kwargs.get('quantity', None)
if __name__ == "__main__":
import doctest
doctest.testmod()
# valid value for name
cameras1 = StockItem(name="Camera", price=45.99, quatity=2)
# invalid value for name according to @valid_string but I need this to be also valid if 'second_call'
cameras2 = StockItem(name=67, price=45.99, quatity=2, second_call=True)
The StockItem
class constructor is invoked twice and on the second turn I want the @valid_string decorator to be somehow canceled (I don't want name attribute's value to be altered anymore).
python python-3.x python-decorators python-descriptors class-decorator
|
show 2 more comments
Lets take the following example of class decorators (origin http://www.informit.com/articles/article.aspx?p=1309289&seqNum=4):
class GenericDescriptor:
def __init__(self, getter, setter):
self.getter = getter
self.setter = setter
def __get__(self, instance, owner=None):
if instance is None:
return self
return self.getter(instance)
def __set__(self, instance, value):
return self.setter(instance, value)
def valid_string(attr_name, empty_allowed=True, regex=None,
acceptable=None):
def decorator(cls):
name = "__" + attr_name
def getter(self):
return getattr(self, name)
def setter(self, value):
assert isinstance(value, str), (attr_name +
" must be a string")
if not empty_allowed and not value:
raise ValueError("{0} may not be empty".format(
attr_name))
if ((acceptable is not None and value not in acceptable) or
(regex is not None and not regex.match(value))):
raise ValueError("{attr_name} cannot be set to "
"{value}".format(**locals()))
setattr(self, name, value)
setattr(cls, attr_name, GenericDescriptor(getter, setter))
return cls
return decorator
@valid_string("name", empty_allowed=False)
class StockItem:
name = None
def __init__(self, **kwargs):
if kwargs.get('second_call'):
pass
# proceed normally without calling @valid_string
self.name = kwargs.get('name', None)
self.price = kwargs.get('price', None)
self.quantity = kwargs.get('quantity', None)
if __name__ == "__main__":
import doctest
doctest.testmod()
# valid value for name
cameras1 = StockItem(name="Camera", price=45.99, quatity=2)
# invalid value for name according to @valid_string but I need this to be also valid if 'second_call'
cameras2 = StockItem(name=67, price=45.99, quatity=2, second_call=True)
The StockItem
class constructor is invoked twice and on the second turn I want the @valid_string decorator to be somehow canceled (I don't want name attribute's value to be altered anymore).
python python-3.x python-decorators python-descriptors class-decorator
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know__init__
is called twice?
– juanpa.arrivillaga
Nov 16 '18 at 17:27
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
1
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11
|
show 2 more comments
Lets take the following example of class decorators (origin http://www.informit.com/articles/article.aspx?p=1309289&seqNum=4):
class GenericDescriptor:
def __init__(self, getter, setter):
self.getter = getter
self.setter = setter
def __get__(self, instance, owner=None):
if instance is None:
return self
return self.getter(instance)
def __set__(self, instance, value):
return self.setter(instance, value)
def valid_string(attr_name, empty_allowed=True, regex=None,
acceptable=None):
def decorator(cls):
name = "__" + attr_name
def getter(self):
return getattr(self, name)
def setter(self, value):
assert isinstance(value, str), (attr_name +
" must be a string")
if not empty_allowed and not value:
raise ValueError("{0} may not be empty".format(
attr_name))
if ((acceptable is not None and value not in acceptable) or
(regex is not None and not regex.match(value))):
raise ValueError("{attr_name} cannot be set to "
"{value}".format(**locals()))
setattr(self, name, value)
setattr(cls, attr_name, GenericDescriptor(getter, setter))
return cls
return decorator
@valid_string("name", empty_allowed=False)
class StockItem:
name = None
def __init__(self, **kwargs):
if kwargs.get('second_call'):
pass
# proceed normally without calling @valid_string
self.name = kwargs.get('name', None)
self.price = kwargs.get('price', None)
self.quantity = kwargs.get('quantity', None)
if __name__ == "__main__":
import doctest
doctest.testmod()
# valid value for name
cameras1 = StockItem(name="Camera", price=45.99, quatity=2)
# invalid value for name according to @valid_string but I need this to be also valid if 'second_call'
cameras2 = StockItem(name=67, price=45.99, quatity=2, second_call=True)
The StockItem
class constructor is invoked twice and on the second turn I want the @valid_string decorator to be somehow canceled (I don't want name attribute's value to be altered anymore).
python python-3.x python-decorators python-descriptors class-decorator
Lets take the following example of class decorators (origin http://www.informit.com/articles/article.aspx?p=1309289&seqNum=4):
class GenericDescriptor:
def __init__(self, getter, setter):
self.getter = getter
self.setter = setter
def __get__(self, instance, owner=None):
if instance is None:
return self
return self.getter(instance)
def __set__(self, instance, value):
return self.setter(instance, value)
def valid_string(attr_name, empty_allowed=True, regex=None,
acceptable=None):
def decorator(cls):
name = "__" + attr_name
def getter(self):
return getattr(self, name)
def setter(self, value):
assert isinstance(value, str), (attr_name +
" must be a string")
if not empty_allowed and not value:
raise ValueError("{0} may not be empty".format(
attr_name))
if ((acceptable is not None and value not in acceptable) or
(regex is not None and not regex.match(value))):
raise ValueError("{attr_name} cannot be set to "
"{value}".format(**locals()))
setattr(self, name, value)
setattr(cls, attr_name, GenericDescriptor(getter, setter))
return cls
return decorator
@valid_string("name", empty_allowed=False)
class StockItem:
name = None
def __init__(self, **kwargs):
if kwargs.get('second_call'):
pass
# proceed normally without calling @valid_string
self.name = kwargs.get('name', None)
self.price = kwargs.get('price', None)
self.quantity = kwargs.get('quantity', None)
if __name__ == "__main__":
import doctest
doctest.testmod()
# valid value for name
cameras1 = StockItem(name="Camera", price=45.99, quatity=2)
# invalid value for name according to @valid_string but I need this to be also valid if 'second_call'
cameras2 = StockItem(name=67, price=45.99, quatity=2, second_call=True)
The StockItem
class constructor is invoked twice and on the second turn I want the @valid_string decorator to be somehow canceled (I don't want name attribute's value to be altered anymore).
python python-3.x python-decorators python-descriptors class-decorator
python python-3.x python-decorators python-descriptors class-decorator
edited Nov 16 '18 at 18:15
Andreea Irimies
asked Nov 16 '18 at 17:25


Andreea IrimiesAndreea Irimies
112
112
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know__init__
is called twice?
– juanpa.arrivillaga
Nov 16 '18 at 17:27
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
1
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11
|
show 2 more comments
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know__init__
is called twice?
– juanpa.arrivillaga
Nov 16 '18 at 17:27
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
1
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know
__init__
is called twice?– juanpa.arrivillaga
Nov 16 '18 at 17:27
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know
__init__
is called twice?– juanpa.arrivillaga
Nov 16 '18 at 17:27
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
1
1
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342665%2fhow-to-cancel-class-decorators-in-constructor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53342665%2fhow-to-cancel-class-decorators-in-constructor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LeGj1e0fTgs n9sERBx8Oq1G4 PlNi9iCt,Ledm8q103GFHT37vMt3re6,uCsTT29YVrSM2P9gC72EAV5sl BQi kh
Hell, Andreea, welcome to StackOverflow. Please provide a Minimal, Complete, and Verifiable example in the question itself. Can you also clarify what exactly is the issue you are encountering? How do you know
__init__
is called twice?– juanpa.arrivillaga
Nov 16 '18 at 17:27
No, you shouldn't provide the whole code, you should provide a Minimal, Complete, and Verifiable example. If we cannot reproduce the behavior you are seeing, it is hard to know how to help.
– juanpa.arrivillaga
Nov 16 '18 at 17:42
Ok guys, here is an example that works: jmp.sh/ADC2GvG (let me know if you cannot access the link)
– Andreea Irimies
Nov 16 '18 at 18:02
1
Andreea, please provide the Minimal, Complete, and Verifiable example in the question itself. Check out How to Ask, questions are required to be self-contained. Just copy and paste that code and edit into your question.
– juanpa.arrivillaga
Nov 16 '18 at 18:06
I've gone ahead and done the edit for you. But anyway, decorators cannot really be "canceled", they have already run by the time your class definition is finished executing (well, they are run immediately after). But you can subvert them, I suppose, but that would be easier if you can modify the decorator code somehow, or to do run-time modifications to the class (the latter option seems more brittle, but it may be easier or your only option if you can't touch the decorator code)
– juanpa.arrivillaga
Nov 16 '18 at 18:11