How to deploy reactJS + nodeJS app on Heroku?
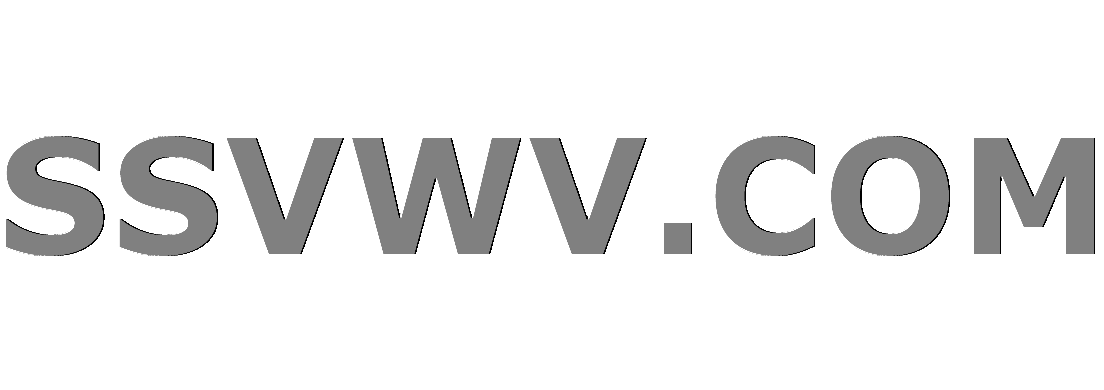
Multi tool use
I have problem with deploying my app to heroku. I have output of react app in built folder (path: /client/build/index.html)
server.js
const express = require('express');
const path = require('path');
const cors = require('cors');
const passport = require('passport');
app.use(express.static(path.resolve(__dirname + '/client/build')));
app.get('*', (req, res) => {
res.sendFile(path.join(__dirname+'/client/build/index.html'));
});
const port = process.env.PORT || 5000;
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
app.use(cors())
mongoose.connect(process.env.MONGODB_URI)
.then(() => console.log("success"))
.catch(err => console.log(err))
app.use(passport.initialize());
require('./config/passport')(passport);
const server = app.listen(port, function(err) {
if (err) {
return;
}
console.log('server listening on port: %s', port);
});
Procfile
web: node server.js
package.json (server)
{
"description": "",
"main": "index.js",
"scripts": {
"start": "node server.js",
"server": "nodemon server.js",
"client-install": "npm install --prefix client",
"client": "npm start --prefix client",
"dev": "concurrently "npm run client" "npm run server""
},
"author": "",
"license": "ISC",
"dependencies": {
"concurrently": "^4.0.1",
"express": "^4.16.4",
"jsonwebtoken": "^8.3.0",
"mongoose": "^5.3.4",
"node": "^8.10.0",
"nodemon": "^1.18.4",
"passport": "^0.4.0",
"passport-jwt": "^4.0.0",
"react-scripts": "1.0.11",
},
"devDependencies": {
"nodemon": "^1.18.4"
}
}
Is it ok to show my reactApp from build folder? How can I deploy my server? Is it enough to put command in Procfile or should I add for example "heroku-postbuild" in package.json? Thanks for help
PS. After pushing to heroku I got this index.html file, but blank page and server is not working.
node.js reactjs heroku
add a comment |
I have problem with deploying my app to heroku. I have output of react app in built folder (path: /client/build/index.html)
server.js
const express = require('express');
const path = require('path');
const cors = require('cors');
const passport = require('passport');
app.use(express.static(path.resolve(__dirname + '/client/build')));
app.get('*', (req, res) => {
res.sendFile(path.join(__dirname+'/client/build/index.html'));
});
const port = process.env.PORT || 5000;
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
app.use(cors())
mongoose.connect(process.env.MONGODB_URI)
.then(() => console.log("success"))
.catch(err => console.log(err))
app.use(passport.initialize());
require('./config/passport')(passport);
const server = app.listen(port, function(err) {
if (err) {
return;
}
console.log('server listening on port: %s', port);
});
Procfile
web: node server.js
package.json (server)
{
"description": "",
"main": "index.js",
"scripts": {
"start": "node server.js",
"server": "nodemon server.js",
"client-install": "npm install --prefix client",
"client": "npm start --prefix client",
"dev": "concurrently "npm run client" "npm run server""
},
"author": "",
"license": "ISC",
"dependencies": {
"concurrently": "^4.0.1",
"express": "^4.16.4",
"jsonwebtoken": "^8.3.0",
"mongoose": "^5.3.4",
"node": "^8.10.0",
"nodemon": "^1.18.4",
"passport": "^0.4.0",
"passport-jwt": "^4.0.0",
"react-scripts": "1.0.11",
},
"devDependencies": {
"nodemon": "^1.18.4"
}
}
Is it ok to show my reactApp from build folder? How can I deploy my server? Is it enough to put command in Procfile or should I add for example "heroku-postbuild" in package.json? Thanks for help
PS. After pushing to heroku I got this index.html file, but blank page and server is not working.
node.js reactjs heroku
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57
add a comment |
I have problem with deploying my app to heroku. I have output of react app in built folder (path: /client/build/index.html)
server.js
const express = require('express');
const path = require('path');
const cors = require('cors');
const passport = require('passport');
app.use(express.static(path.resolve(__dirname + '/client/build')));
app.get('*', (req, res) => {
res.sendFile(path.join(__dirname+'/client/build/index.html'));
});
const port = process.env.PORT || 5000;
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
app.use(cors())
mongoose.connect(process.env.MONGODB_URI)
.then(() => console.log("success"))
.catch(err => console.log(err))
app.use(passport.initialize());
require('./config/passport')(passport);
const server = app.listen(port, function(err) {
if (err) {
return;
}
console.log('server listening on port: %s', port);
});
Procfile
web: node server.js
package.json (server)
{
"description": "",
"main": "index.js",
"scripts": {
"start": "node server.js",
"server": "nodemon server.js",
"client-install": "npm install --prefix client",
"client": "npm start --prefix client",
"dev": "concurrently "npm run client" "npm run server""
},
"author": "",
"license": "ISC",
"dependencies": {
"concurrently": "^4.0.1",
"express": "^4.16.4",
"jsonwebtoken": "^8.3.0",
"mongoose": "^5.3.4",
"node": "^8.10.0",
"nodemon": "^1.18.4",
"passport": "^0.4.0",
"passport-jwt": "^4.0.0",
"react-scripts": "1.0.11",
},
"devDependencies": {
"nodemon": "^1.18.4"
}
}
Is it ok to show my reactApp from build folder? How can I deploy my server? Is it enough to put command in Procfile or should I add for example "heroku-postbuild" in package.json? Thanks for help
PS. After pushing to heroku I got this index.html file, but blank page and server is not working.
node.js reactjs heroku
I have problem with deploying my app to heroku. I have output of react app in built folder (path: /client/build/index.html)
server.js
const express = require('express');
const path = require('path');
const cors = require('cors');
const passport = require('passport');
app.use(express.static(path.resolve(__dirname + '/client/build')));
app.get('*', (req, res) => {
res.sendFile(path.join(__dirname+'/client/build/index.html'));
});
const port = process.env.PORT || 5000;
const bodyParser = require('body-parser');
const mongoose = require('mongoose');
app.use(bodyParser.urlencoded({extended:false}));
app.use(bodyParser.json());
app.use(cors())
mongoose.connect(process.env.MONGODB_URI)
.then(() => console.log("success"))
.catch(err => console.log(err))
app.use(passport.initialize());
require('./config/passport')(passport);
const server = app.listen(port, function(err) {
if (err) {
return;
}
console.log('server listening on port: %s', port);
});
Procfile
web: node server.js
package.json (server)
{
"description": "",
"main": "index.js",
"scripts": {
"start": "node server.js",
"server": "nodemon server.js",
"client-install": "npm install --prefix client",
"client": "npm start --prefix client",
"dev": "concurrently "npm run client" "npm run server""
},
"author": "",
"license": "ISC",
"dependencies": {
"concurrently": "^4.0.1",
"express": "^4.16.4",
"jsonwebtoken": "^8.3.0",
"mongoose": "^5.3.4",
"node": "^8.10.0",
"nodemon": "^1.18.4",
"passport": "^0.4.0",
"passport-jwt": "^4.0.0",
"react-scripts": "1.0.11",
},
"devDependencies": {
"nodemon": "^1.18.4"
}
}
Is it ok to show my reactApp from build folder? How can I deploy my server? Is it enough to put command in Procfile or should I add for example "heroku-postbuild" in package.json? Thanks for help
PS. After pushing to heroku I got this index.html file, but blank page and server is not working.
node.js reactjs heroku
node.js reactjs heroku
edited Nov 15 '18 at 11:42
Kasia1
asked Nov 15 '18 at 11:26
Kasia1Kasia1
84
84
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57
add a comment |
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57
add a comment |
1 Answer
1
active
oldest
votes
In server.js
your app
object is not defined as in app = express()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53318428%2fhow-to-deploy-reactjs-nodejs-app-on-heroku%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In server.js
your app
object is not defined as in app = express()
add a comment |
In server.js
your app
object is not defined as in app = express()
add a comment |
In server.js
your app
object is not defined as in app = express()
In server.js
your app
object is not defined as in app = express()
answered Nov 15 '18 at 13:22


elightelight
809
809
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53318428%2fhow-to-deploy-reactjs-nodejs-app-on-heroku%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8,X,7BX,MV3 W4kkV zE,dkxVt1 Q2ZRGj3y,6Ct,JtZURLnmpohy,uqxi J69MCW4wiJDvny fxAj2SWa G34 R2M1s
Check through the documentation listed here and follow this tutorial to ensure that your app is setup correctly. Sounds like your react app isn't deployed.
– elken
Nov 15 '18 at 11:48
Have a look at this answer to see if it helps
– c-chavez
Nov 15 '18 at 11:57