Python: Any optimized way to check that 2 lists increase together?
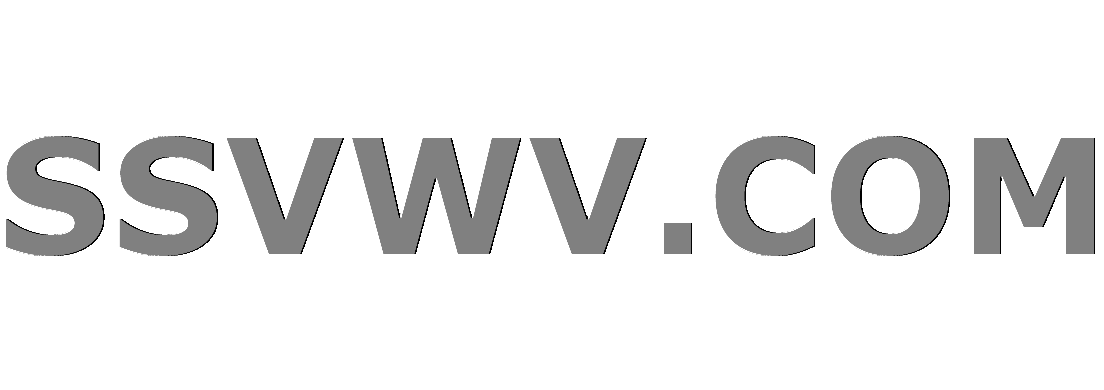
Multi tool use
Let's assume we have 2 lists a = [1,2,4,3,5]
and b = [103,122,800,500,1000]
is there an optimized way that I can check that they are "increasing together"?
My current solution, employs a loop:
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
print('wrong')
Is there a better way?
Notes:
- The solution does not need to be list specific (really any data structure would work)
- The two iterables do not need to increase by the same number of units, just have to increase together.
python sequence
|
show 1 more comment
Let's assume we have 2 lists a = [1,2,4,3,5]
and b = [103,122,800,500,1000]
is there an optimized way that I can check that they are "increasing together"?
My current solution, employs a loop:
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
print('wrong')
Is there a better way?
Notes:
- The solution does not need to be list specific (really any data structure would work)
- The two iterables do not need to increase by the same number of units, just have to increase together.
python sequence
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44
|
show 1 more comment
Let's assume we have 2 lists a = [1,2,4,3,5]
and b = [103,122,800,500,1000]
is there an optimized way that I can check that they are "increasing together"?
My current solution, employs a loop:
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
print('wrong')
Is there a better way?
Notes:
- The solution does not need to be list specific (really any data structure would work)
- The two iterables do not need to increase by the same number of units, just have to increase together.
python sequence
Let's assume we have 2 lists a = [1,2,4,3,5]
and b = [103,122,800,500,1000]
is there an optimized way that I can check that they are "increasing together"?
My current solution, employs a loop:
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
print('wrong')
Is there a better way?
Notes:
- The solution does not need to be list specific (really any data structure would work)
- The two iterables do not need to increase by the same number of units, just have to increase together.
python sequence
python sequence
asked Nov 14 '18 at 10:30
dozyaustindozyaustin
14411
14411
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44
|
show 1 more comment
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44
|
show 1 more comment
4 Answers
4
active
oldest
votes
You can't really get any faster than O(n), but you could make your code a bit shorter and maybe more readable by using numpy.diff
and comparing the sign
of the diffs of a
and b
:
>>> from numpy import diff, sign
>>> a, b = [1,2,4,3,5], [103,122,800,500,1000]
>>> sign(diff(a))
array([ 1, 1, -1, 1])
>>> all(sign(diff(a)) == sign(diff(b)))
True
>>> a, b = [1,2,4,3,5], [103,122,800,500,100]
>>> all(sign(diff(a)) == sign(diff(b)))
False
The downside of this solution is that it does not use lazy-evaluation, i.e. it calculates and compares the entire sign(diff(...))
array even if the "increasingness" of a
and b
differs in the very first position. If the list is very long, you should consider using another approach.
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
add a comment |
In terms of O(order notation), you can't get better than linear, assuming lists don't have some order. But, you can use some python compiler like cython, numba to speed up your code. Your code using numba:
import numpy as np
import numba as nb
@nb.njit()
def vary_together(a, b):
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
return False
return True
You have to use large lists to see the performance benefit. For example, if:
a = np.array([randint(0,100) for i in range(10000000)])
Then,
vary_together(a, a) # a as both arguments so as to make it complete the loop
Has has the performance comparison to your solution as :
Your solution: 8.09s
vary_together: 0.2 (on second run to discount for compile time).
If you need to run the code again and again in the script, do cache=True
in the nb.njit
decorator.
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
add a comment |
We can use the lazy evaluation provided by python iterators, meaning we don't need to continue traversing both lists ( structures ) once they don't have the same variation sign
def compare_variation( a, b ):
a_variations = ( a[ i - 1 ] < a[ i ] for i in range( 1, len( a ) ) )
b_variations = ( b[ i - 1 ] < b[ i ] for i in range( 1, len( b ) ) )
return all( x == y for x, y in zip( a_variations, b_variations ) )
1
If you still calculate thediff
andsign
for the entire listsa
andb
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.
– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
You can just writea[i-1] < a[i]
instead ofTrue if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just userange
andzip
, which now return iterators instead of lists.
– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
add a comment |
Here is a way with list comprehension:
c = [(a[x + 1] - a[x]) * (b[x + 1] - b[x]) for x in range(len(a) - 1)]
if any([x < 0 for x in c]):
print('Wrong')
Comparing all the previous approaches (except for the numba one), tobias_k's answer looks like most efficient when dealing with large enough lists (at list 40 elements roughly).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298045%2fpython-any-optimized-way-to-check-that-2-lists-increase-together%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can't really get any faster than O(n), but you could make your code a bit shorter and maybe more readable by using numpy.diff
and comparing the sign
of the diffs of a
and b
:
>>> from numpy import diff, sign
>>> a, b = [1,2,4,3,5], [103,122,800,500,1000]
>>> sign(diff(a))
array([ 1, 1, -1, 1])
>>> all(sign(diff(a)) == sign(diff(b)))
True
>>> a, b = [1,2,4,3,5], [103,122,800,500,100]
>>> all(sign(diff(a)) == sign(diff(b)))
False
The downside of this solution is that it does not use lazy-evaluation, i.e. it calculates and compares the entire sign(diff(...))
array even if the "increasingness" of a
and b
differs in the very first position. If the list is very long, you should consider using another approach.
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
add a comment |
You can't really get any faster than O(n), but you could make your code a bit shorter and maybe more readable by using numpy.diff
and comparing the sign
of the diffs of a
and b
:
>>> from numpy import diff, sign
>>> a, b = [1,2,4,3,5], [103,122,800,500,1000]
>>> sign(diff(a))
array([ 1, 1, -1, 1])
>>> all(sign(diff(a)) == sign(diff(b)))
True
>>> a, b = [1,2,4,3,5], [103,122,800,500,100]
>>> all(sign(diff(a)) == sign(diff(b)))
False
The downside of this solution is that it does not use lazy-evaluation, i.e. it calculates and compares the entire sign(diff(...))
array even if the "increasingness" of a
and b
differs in the very first position. If the list is very long, you should consider using another approach.
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
add a comment |
You can't really get any faster than O(n), but you could make your code a bit shorter and maybe more readable by using numpy.diff
and comparing the sign
of the diffs of a
and b
:
>>> from numpy import diff, sign
>>> a, b = [1,2,4,3,5], [103,122,800,500,1000]
>>> sign(diff(a))
array([ 1, 1, -1, 1])
>>> all(sign(diff(a)) == sign(diff(b)))
True
>>> a, b = [1,2,4,3,5], [103,122,800,500,100]
>>> all(sign(diff(a)) == sign(diff(b)))
False
The downside of this solution is that it does not use lazy-evaluation, i.e. it calculates and compares the entire sign(diff(...))
array even if the "increasingness" of a
and b
differs in the very first position. If the list is very long, you should consider using another approach.
You can't really get any faster than O(n), but you could make your code a bit shorter and maybe more readable by using numpy.diff
and comparing the sign
of the diffs of a
and b
:
>>> from numpy import diff, sign
>>> a, b = [1,2,4,3,5], [103,122,800,500,1000]
>>> sign(diff(a))
array([ 1, 1, -1, 1])
>>> all(sign(diff(a)) == sign(diff(b)))
True
>>> a, b = [1,2,4,3,5], [103,122,800,500,100]
>>> all(sign(diff(a)) == sign(diff(b)))
False
The downside of this solution is that it does not use lazy-evaluation, i.e. it calculates and compares the entire sign(diff(...))
array even if the "increasingness" of a
and b
differs in the very first position. If the list is very long, you should consider using another approach.
edited Nov 14 '18 at 11:32
answered Nov 14 '18 at 10:53
tobias_ktobias_k
58.1k969106
58.1k969106
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
add a comment |
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
I find this the most elegant, and it avoid an explicit loop. Thanks. It worked like a charm.
– dozyaustin
Nov 14 '18 at 14:22
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
Your approach is actually fastest when one compares two large lists which increase together.
– Patol75
Nov 14 '18 at 22:50
add a comment |
In terms of O(order notation), you can't get better than linear, assuming lists don't have some order. But, you can use some python compiler like cython, numba to speed up your code. Your code using numba:
import numpy as np
import numba as nb
@nb.njit()
def vary_together(a, b):
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
return False
return True
You have to use large lists to see the performance benefit. For example, if:
a = np.array([randint(0,100) for i in range(10000000)])
Then,
vary_together(a, a) # a as both arguments so as to make it complete the loop
Has has the performance comparison to your solution as :
Your solution: 8.09s
vary_together: 0.2 (on second run to discount for compile time).
If you need to run the code again and again in the script, do cache=True
in the nb.njit
decorator.
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
add a comment |
In terms of O(order notation), you can't get better than linear, assuming lists don't have some order. But, you can use some python compiler like cython, numba to speed up your code. Your code using numba:
import numpy as np
import numba as nb
@nb.njit()
def vary_together(a, b):
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
return False
return True
You have to use large lists to see the performance benefit. For example, if:
a = np.array([randint(0,100) for i in range(10000000)])
Then,
vary_together(a, a) # a as both arguments so as to make it complete the loop
Has has the performance comparison to your solution as :
Your solution: 8.09s
vary_together: 0.2 (on second run to discount for compile time).
If you need to run the code again and again in the script, do cache=True
in the nb.njit
decorator.
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
add a comment |
In terms of O(order notation), you can't get better than linear, assuming lists don't have some order. But, you can use some python compiler like cython, numba to speed up your code. Your code using numba:
import numpy as np
import numba as nb
@nb.njit()
def vary_together(a, b):
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
return False
return True
You have to use large lists to see the performance benefit. For example, if:
a = np.array([randint(0,100) for i in range(10000000)])
Then,
vary_together(a, a) # a as both arguments so as to make it complete the loop
Has has the performance comparison to your solution as :
Your solution: 8.09s
vary_together: 0.2 (on second run to discount for compile time).
If you need to run the code again and again in the script, do cache=True
in the nb.njit
decorator.
In terms of O(order notation), you can't get better than linear, assuming lists don't have some order. But, you can use some python compiler like cython, numba to speed up your code. Your code using numba:
import numpy as np
import numba as nb
@nb.njit()
def vary_together(a, b):
for i in range(1,len(a)):
if (a[i-1] < a[i] and b[i-1] > b[i]) or (a[i-1] > a[i] and b[i-1] < b[i]):
return False
return True
You have to use large lists to see the performance benefit. For example, if:
a = np.array([randint(0,100) for i in range(10000000)])
Then,
vary_together(a, a) # a as both arguments so as to make it complete the loop
Has has the performance comparison to your solution as :
Your solution: 8.09s
vary_together: 0.2 (on second run to discount for compile time).
If you need to run the code again and again in the script, do cache=True
in the nb.njit
decorator.
edited Nov 14 '18 at 11:40
answered Nov 14 '18 at 10:52
Deepak SainiDeepak Saini
1,597814
1,597814
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
add a comment |
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
Good answer. But numba can look exotic for beginners. Add vary_together (a,ma) with ma=-a to enforce the lazy aspect. and compare with and without numba (4 cases)
– B. M.
Nov 14 '18 at 12:24
add a comment |
We can use the lazy evaluation provided by python iterators, meaning we don't need to continue traversing both lists ( structures ) once they don't have the same variation sign
def compare_variation( a, b ):
a_variations = ( a[ i - 1 ] < a[ i ] for i in range( 1, len( a ) ) )
b_variations = ( b[ i - 1 ] < b[ i ] for i in range( 1, len( b ) ) )
return all( x == y for x, y in zip( a_variations, b_variations ) )
1
If you still calculate thediff
andsign
for the entire listsa
andb
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.
– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
You can just writea[i-1] < a[i]
instead ofTrue if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just userange
andzip
, which now return iterators instead of lists.
– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
add a comment |
We can use the lazy evaluation provided by python iterators, meaning we don't need to continue traversing both lists ( structures ) once they don't have the same variation sign
def compare_variation( a, b ):
a_variations = ( a[ i - 1 ] < a[ i ] for i in range( 1, len( a ) ) )
b_variations = ( b[ i - 1 ] < b[ i ] for i in range( 1, len( b ) ) )
return all( x == y for x, y in zip( a_variations, b_variations ) )
1
If you still calculate thediff
andsign
for the entire listsa
andb
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.
– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
You can just writea[i-1] < a[i]
instead ofTrue if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just userange
andzip
, which now return iterators instead of lists.
– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
add a comment |
We can use the lazy evaluation provided by python iterators, meaning we don't need to continue traversing both lists ( structures ) once they don't have the same variation sign
def compare_variation( a, b ):
a_variations = ( a[ i - 1 ] < a[ i ] for i in range( 1, len( a ) ) )
b_variations = ( b[ i - 1 ] < b[ i ] for i in range( 1, len( b ) ) )
return all( x == y for x, y in zip( a_variations, b_variations ) )
We can use the lazy evaluation provided by python iterators, meaning we don't need to continue traversing both lists ( structures ) once they don't have the same variation sign
def compare_variation( a, b ):
a_variations = ( a[ i - 1 ] < a[ i ] for i in range( 1, len( a ) ) )
b_variations = ( b[ i - 1 ] < b[ i ] for i in range( 1, len( b ) ) )
return all( x == y for x, y in zip( a_variations, b_variations ) )
edited Nov 14 '18 at 11:35
answered Nov 14 '18 at 11:03


rachid el kedmirirachid el kedmiri
973522
973522
1
If you still calculate thediff
andsign
for the entire listsa
andb
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.
– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
You can just writea[i-1] < a[i]
instead ofTrue if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just userange
andzip
, which now return iterators instead of lists.
– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
add a comment |
1
If you still calculate thediff
andsign
for the entire listsa
andb
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.
– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
You can just writea[i-1] < a[i]
instead ofTrue if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just userange
andzip
, which now return iterators instead of lists.
– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
1
1
If you still calculate the
diff
and sign
for the entire lists a
and b
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.– tobias_k
Nov 14 '18 at 11:25
If you still calculate the
diff
and sign
for the entire lists a
and b
, lazy-evaluating whether they are equal does not lower the number of operations significantly, even if they differ in the very first comparison.– tobias_k
Nov 14 '18 at 11:25
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
@tobias_k valid point i have updated my code accordingly, thanks a lot
– rachid el kedmiri
Nov 14 '18 at 11:27
1
1
You can just write
a[i-1] < a[i]
instead of True if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just use range
and zip
, which now return iterators instead of lists.– tobias_k
Nov 14 '18 at 11:29
You can just write
a[i-1] < a[i]
instead of True if a[ i - 1 ] < a[ i ] else False
. Also, I'd suggest switching to Python 3, i.e. just use range
and zip
, which now return iterators instead of lists.– tobias_k
Nov 14 '18 at 11:29
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k I have changed my code following your recommendations, thanks a loot
– rachid el kedmiri
Nov 14 '18 at 11:33
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
@tobias_k totally forgot about the xrange, thanks a lot, much appreciated
– rachid el kedmiri
Nov 14 '18 at 11:35
add a comment |
Here is a way with list comprehension:
c = [(a[x + 1] - a[x]) * (b[x + 1] - b[x]) for x in range(len(a) - 1)]
if any([x < 0 for x in c]):
print('Wrong')
Comparing all the previous approaches (except for the numba one), tobias_k's answer looks like most efficient when dealing with large enough lists (at list 40 elements roughly).
add a comment |
Here is a way with list comprehension:
c = [(a[x + 1] - a[x]) * (b[x + 1] - b[x]) for x in range(len(a) - 1)]
if any([x < 0 for x in c]):
print('Wrong')
Comparing all the previous approaches (except for the numba one), tobias_k's answer looks like most efficient when dealing with large enough lists (at list 40 elements roughly).
add a comment |
Here is a way with list comprehension:
c = [(a[x + 1] - a[x]) * (b[x + 1] - b[x]) for x in range(len(a) - 1)]
if any([x < 0 for x in c]):
print('Wrong')
Comparing all the previous approaches (except for the numba one), tobias_k's answer looks like most efficient when dealing with large enough lists (at list 40 elements roughly).
Here is a way with list comprehension:
c = [(a[x + 1] - a[x]) * (b[x + 1] - b[x]) for x in range(len(a) - 1)]
if any([x < 0 for x in c]):
print('Wrong')
Comparing all the previous approaches (except for the numba one), tobias_k's answer looks like most efficient when dealing with large enough lists (at list 40 elements roughly).
answered Nov 14 '18 at 11:56
Patol75Patol75
6236
6236
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298045%2fpython-any-optimized-way-to-check-that-2-lists-increase-together%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
m8,nnTw4r1Rm,3,ya1qyAWerYtXZY
By optimize you want faster than current O(N)?
– awesoon
Nov 14 '18 at 10:32
What do you mean with "increasing together"? That the elements in both lists are sorted in increasing order? Or that element[i] in one list is bigger then element[i] in the other list for all possible indices? Something else?
– quant
Nov 14 '18 at 10:37
well there is the loop, and then there is the comparison operation itself. So really both. Looking whether I am oblivious to CS approach that has handled this more elegantly.
– dozyaustin
Nov 14 '18 at 10:38
You might get some improvement in performance by phrasing this in terms of numpy ndarrays.
– Ketil Tveiten
Nov 14 '18 at 10:42
@quant what I mean is that regardless of initial sort of item that if element a[i] is larger than a[i-1] then the same is true for items at same indices in list b.
– dozyaustin
Nov 14 '18 at 10:44