OpenGL applying glMaterial to only one object
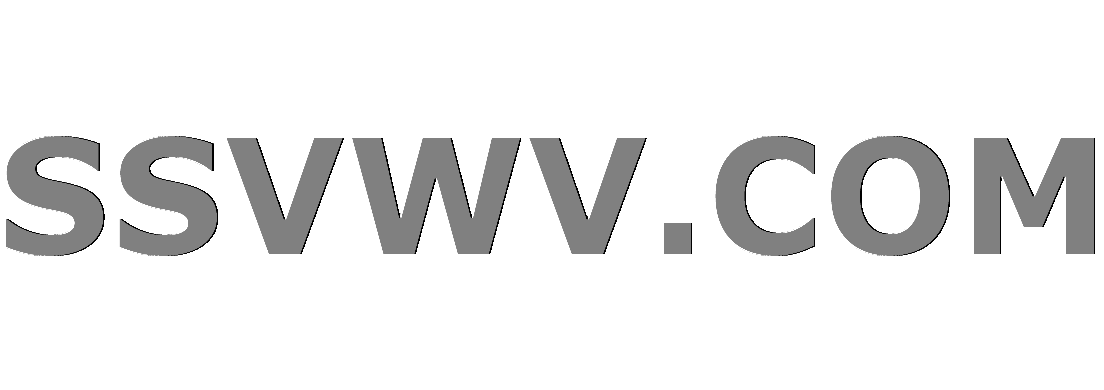
Multi tool use
With the code below I am trying to apply lighting to the sun. When I apply GL_EMISSION it seems to apply the lighting to the other objects that are created too.
GL11.glPushMatrix();
{
// how shiny are the front faces of the sun (specular exponent)
float sunFrontShininess = 2.0f;
// specular reflection of the front faces of the sun
float sunFrontSpecular = {0.1f, 0.1f, 0.0f, 1.0f};
// diffuse reflection of the front faces of the sun
float sunFrontDiffuse = {1.0f, 1.0f, 1.0f, 1.0f};
float sunEmission = {0.2f, 0.2f, 0.2f, 1f};
// set the material properties for the sun using OpenGL
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_EMISSION, FloatBuffer.wrap(sunEmission));
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterialf(GL11.GL_FRONT, GL11.GL_SHININESS, sunFrontShininess);
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_SPECULAR, FloatBuffer.wrap(sunFrontSpecular));
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_DIFFUSE, FloatBuffer.wrap(sunFrontDiffuse));
// position and draw the sun using a sphere quadric object
GL11.glTranslatef(4.0f, 7.0f, -19.0f);
new Sphere().draw(0.5f,10,10);
}
GL11.glPopMatrix();
opengl
add a comment |
With the code below I am trying to apply lighting to the sun. When I apply GL_EMISSION it seems to apply the lighting to the other objects that are created too.
GL11.glPushMatrix();
{
// how shiny are the front faces of the sun (specular exponent)
float sunFrontShininess = 2.0f;
// specular reflection of the front faces of the sun
float sunFrontSpecular = {0.1f, 0.1f, 0.0f, 1.0f};
// diffuse reflection of the front faces of the sun
float sunFrontDiffuse = {1.0f, 1.0f, 1.0f, 1.0f};
float sunEmission = {0.2f, 0.2f, 0.2f, 1f};
// set the material properties for the sun using OpenGL
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_EMISSION, FloatBuffer.wrap(sunEmission));
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterialf(GL11.GL_FRONT, GL11.GL_SHININESS, sunFrontShininess);
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_SPECULAR, FloatBuffer.wrap(sunFrontSpecular));
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_DIFFUSE, FloatBuffer.wrap(sunFrontDiffuse));
// position and draw the sun using a sphere quadric object
GL11.glTranslatef(4.0f, 7.0f, -19.0f);
new Sphere().draw(0.5f,10,10);
}
GL11.glPopMatrix();
opengl
2
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.glMaterial
,glPushMatrix
,glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.
– Nico Schertler
Nov 14 '18 at 19:14
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20
add a comment |
With the code below I am trying to apply lighting to the sun. When I apply GL_EMISSION it seems to apply the lighting to the other objects that are created too.
GL11.glPushMatrix();
{
// how shiny are the front faces of the sun (specular exponent)
float sunFrontShininess = 2.0f;
// specular reflection of the front faces of the sun
float sunFrontSpecular = {0.1f, 0.1f, 0.0f, 1.0f};
// diffuse reflection of the front faces of the sun
float sunFrontDiffuse = {1.0f, 1.0f, 1.0f, 1.0f};
float sunEmission = {0.2f, 0.2f, 0.2f, 1f};
// set the material properties for the sun using OpenGL
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_EMISSION, FloatBuffer.wrap(sunEmission));
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterialf(GL11.GL_FRONT, GL11.GL_SHININESS, sunFrontShininess);
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_SPECULAR, FloatBuffer.wrap(sunFrontSpecular));
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_DIFFUSE, FloatBuffer.wrap(sunFrontDiffuse));
// position and draw the sun using a sphere quadric object
GL11.glTranslatef(4.0f, 7.0f, -19.0f);
new Sphere().draw(0.5f,10,10);
}
GL11.glPopMatrix();
opengl
With the code below I am trying to apply lighting to the sun. When I apply GL_EMISSION it seems to apply the lighting to the other objects that are created too.
GL11.glPushMatrix();
{
// how shiny are the front faces of the sun (specular exponent)
float sunFrontShininess = 2.0f;
// specular reflection of the front faces of the sun
float sunFrontSpecular = {0.1f, 0.1f, 0.0f, 1.0f};
// diffuse reflection of the front faces of the sun
float sunFrontDiffuse = {1.0f, 1.0f, 1.0f, 1.0f};
float sunEmission = {0.2f, 0.2f, 0.2f, 1f};
// set the material properties for the sun using OpenGL
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_EMISSION, FloatBuffer.wrap(sunEmission));
//???????????????????????????????????????????????????????????????????????????????????????
GL11.glMaterialf(GL11.GL_FRONT, GL11.GL_SHININESS, sunFrontShininess);
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_SPECULAR, FloatBuffer.wrap(sunFrontSpecular));
GL11.glMaterial(GL11.GL_FRONT, GL11.GL_DIFFUSE, FloatBuffer.wrap(sunFrontDiffuse));
// position and draw the sun using a sphere quadric object
GL11.glTranslatef(4.0f, 7.0f, -19.0f);
new Sphere().draw(0.5f,10,10);
}
GL11.glPopMatrix();
opengl
opengl
asked Nov 14 '18 at 18:06
Marius KuzmMarius Kuzm
273
273
2
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.glMaterial
,glPushMatrix
,glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.
– Nico Schertler
Nov 14 '18 at 19:14
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20
add a comment |
2
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.glMaterial
,glPushMatrix
,glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.
– Nico Schertler
Nov 14 '18 at 19:14
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20
2
2
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.
glMaterial
, glPushMatrix
, glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.– Nico Schertler
Nov 14 '18 at 19:14
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.
glMaterial
, glPushMatrix
, glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.– Nico Schertler
Nov 14 '18 at 19:14
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306342%2fopengl-applying-glmaterial-to-only-one-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306342%2fopengl-applying-glmaterial-to-only-one-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lEGsCsSul,KdFlwKuM88UV6S0TYwBXqFji,sA9h n2
2
OpenGL is a state machine. If you change a state it is kept stated, until you change it again, even beyond frames.
– Rabbid76
Nov 14 '18 at 18:12
General comment: If you are just starting to learn OpenGL, use a more recent tutorial.
glMaterial
,glPushMatrix
,glTranslate
and so on have been deprecated for over 10 years. If you learn OpenGL, learn the current version with shaders.– Nico Schertler
Nov 14 '18 at 19:14
@NicoSchertler my university lecturer is teaching us this way, therefore thats the way we have to do it
– Marius Kuzm
Nov 14 '18 at 19:16
Then this is a perfectly fine reason. Just wanted to mention it in case you just picked a random OpenGL tutorial.
– Nico Schertler
Nov 14 '18 at 19:18
Just set the emissive material to black after you have rendered the sun. Edit Rabbid76 already pointed it out
– Nadir
Nov 15 '18 at 13:20