How to continue processing next items on exception for the following code snippet?
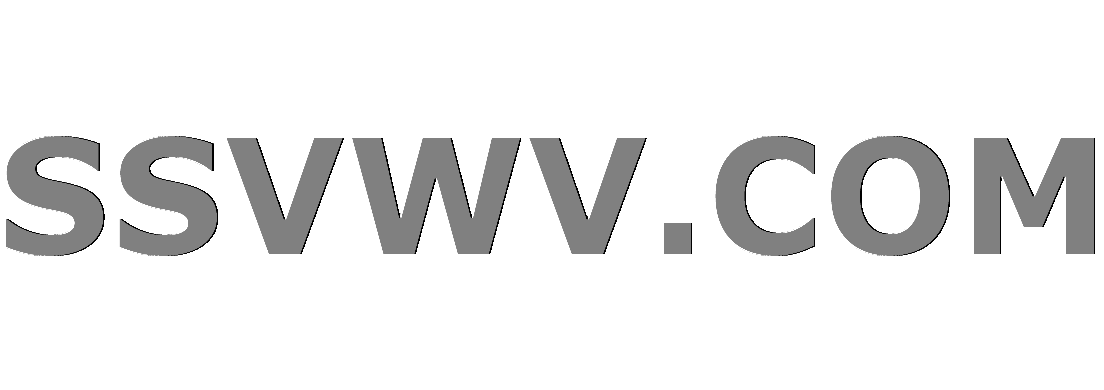
Multi tool use
When I run the code, the output coming is as follows
(a)Test(a=100)
(b)error here- java.lang.NullPointerException
In the current output, whenever the second item throws an exception, the flowable stop processing. But I want to process the third object like these
(a)Test(a=100)
(b)error here {} java.lang.NullPointerException
(c)Test(a=200)
public class Test {
Integer a;
public Integer getA() {
return a * 10;
}
public static void main(String args) {
Test test = new Test(10);
Test test2 = new Test(null); //this object throws exception when it enters flowable
Test test3 = new Test(20);
List<Test> strings = Arrays.asList(test, test2, test3);
Flowable.fromCallable(() -> strings)
.flatMap(s -> Flowable.fromIterable(s)
.flatMap(a -> {
System.out.println(a);
return Flowable.just(a);
})
.onErrorResumeNext(r -> {
System.out.println("error here " + r);
return Flowable.empty();
})
)
.onErrorResumeNext(s -> {
return Flowable.empty();
})
.subscribe();
}`
}
I tried above approach but does not work.
java error-handling nullpointerexception rx-java2
add a comment |
When I run the code, the output coming is as follows
(a)Test(a=100)
(b)error here- java.lang.NullPointerException
In the current output, whenever the second item throws an exception, the flowable stop processing. But I want to process the third object like these
(a)Test(a=100)
(b)error here {} java.lang.NullPointerException
(c)Test(a=200)
public class Test {
Integer a;
public Integer getA() {
return a * 10;
}
public static void main(String args) {
Test test = new Test(10);
Test test2 = new Test(null); //this object throws exception when it enters flowable
Test test3 = new Test(20);
List<Test> strings = Arrays.asList(test, test2, test3);
Flowable.fromCallable(() -> strings)
.flatMap(s -> Flowable.fromIterable(s)
.flatMap(a -> {
System.out.println(a);
return Flowable.just(a);
})
.onErrorResumeNext(r -> {
System.out.println("error here " + r);
return Flowable.empty();
})
)
.onErrorResumeNext(s -> {
return Flowable.empty();
})
.subscribe();
}`
}
I tried above approach but does not work.
java error-handling nullpointerexception rx-java2
1
Why you don't want filterTest(null)
items? That should solve your problem without any complicated error handling
– ConstOrVar
Nov 14 '18 at 18:56
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
There is overloadedflatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.
– ConstOrVar
Nov 15 '18 at 19:44
add a comment |
When I run the code, the output coming is as follows
(a)Test(a=100)
(b)error here- java.lang.NullPointerException
In the current output, whenever the second item throws an exception, the flowable stop processing. But I want to process the third object like these
(a)Test(a=100)
(b)error here {} java.lang.NullPointerException
(c)Test(a=200)
public class Test {
Integer a;
public Integer getA() {
return a * 10;
}
public static void main(String args) {
Test test = new Test(10);
Test test2 = new Test(null); //this object throws exception when it enters flowable
Test test3 = new Test(20);
List<Test> strings = Arrays.asList(test, test2, test3);
Flowable.fromCallable(() -> strings)
.flatMap(s -> Flowable.fromIterable(s)
.flatMap(a -> {
System.out.println(a);
return Flowable.just(a);
})
.onErrorResumeNext(r -> {
System.out.println("error here " + r);
return Flowable.empty();
})
)
.onErrorResumeNext(s -> {
return Flowable.empty();
})
.subscribe();
}`
}
I tried above approach but does not work.
java error-handling nullpointerexception rx-java2
When I run the code, the output coming is as follows
(a)Test(a=100)
(b)error here- java.lang.NullPointerException
In the current output, whenever the second item throws an exception, the flowable stop processing. But I want to process the third object like these
(a)Test(a=100)
(b)error here {} java.lang.NullPointerException
(c)Test(a=200)
public class Test {
Integer a;
public Integer getA() {
return a * 10;
}
public static void main(String args) {
Test test = new Test(10);
Test test2 = new Test(null); //this object throws exception when it enters flowable
Test test3 = new Test(20);
List<Test> strings = Arrays.asList(test, test2, test3);
Flowable.fromCallable(() -> strings)
.flatMap(s -> Flowable.fromIterable(s)
.flatMap(a -> {
System.out.println(a);
return Flowable.just(a);
})
.onErrorResumeNext(r -> {
System.out.println("error here " + r);
return Flowable.empty();
})
)
.onErrorResumeNext(s -> {
return Flowable.empty();
})
.subscribe();
}`
}
I tried above approach but does not work.
java error-handling nullpointerexception rx-java2
java error-handling nullpointerexception rx-java2
edited Dec 8 '18 at 18:30
Filip
3,30931226
3,30931226
asked Nov 14 '18 at 18:00
prabhuprabhu
1
1
1
Why you don't want filterTest(null)
items? That should solve your problem without any complicated error handling
– ConstOrVar
Nov 14 '18 at 18:56
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
There is overloadedflatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.
– ConstOrVar
Nov 15 '18 at 19:44
add a comment |
1
Why you don't want filterTest(null)
items? That should solve your problem without any complicated error handling
– ConstOrVar
Nov 14 '18 at 18:56
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
There is overloadedflatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.
– ConstOrVar
Nov 15 '18 at 19:44
1
1
Why you don't want filter
Test(null)
items? That should solve your problem without any complicated error handling– ConstOrVar
Nov 14 '18 at 18:56
Why you don't want filter
Test(null)
items? That should solve your problem without any complicated error handling– ConstOrVar
Nov 14 '18 at 18:56
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
There is overloaded
flatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.– ConstOrVar
Nov 15 '18 at 19:44
There is overloaded
flatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.– ConstOrVar
Nov 15 '18 at 19:44
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306244%2fhow-to-continue-processing-next-items-on-exception-for-the-following-code-snippe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306244%2fhow-to-continue-processing-next-items-on-exception-for-the-following-code-snippe%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
G3FFHi1Bk Lz4UY5zNH5gYBXz5lMjHr9xTLfyRDN78ChR1rWtXxkE2SXIrSf2
1
Why you don't want filter
Test(null)
items? That should solve your problem without any complicated error handling– ConstOrVar
Nov 14 '18 at 18:56
Here, Test(null) is just for example. I am facing an exception where I can't add a filter. So, I need to handle exception when it comes and continue processing other items
– prabhu
Nov 15 '18 at 19:17
There is overloaded
flatMap(Function<? super T,? extends ObservableSource<? extends R>> mapper, boolean delayErrors)
that allows delay errors . You can pass delayErros = true in that case.– ConstOrVar
Nov 15 '18 at 19:44