model relationships dependant on others
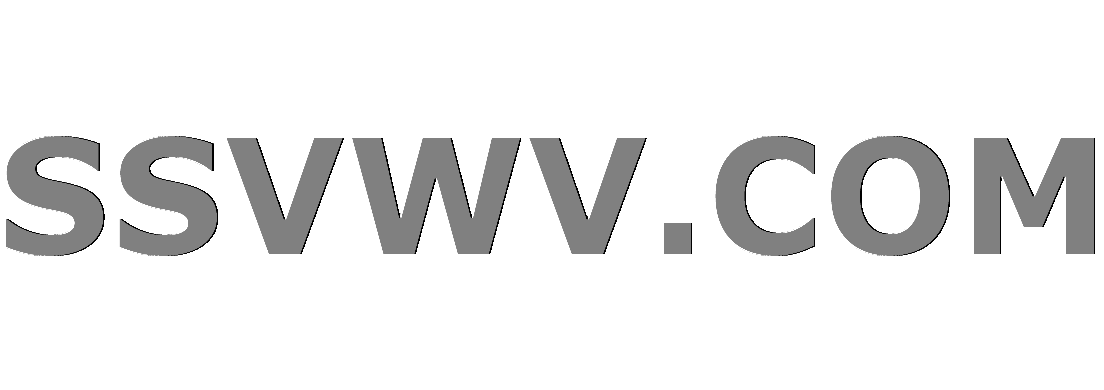
Multi tool use
I'm trying to do something, I'm not sure if it's possible...
I need a User, who needs a Manager. BUT, if the User has a Pod, their manager can be derived from the Pod.owner (in fact, it must be).
So, something like:
class User
belongs_to :pod, optional: true
belongs_to :manager, class_name: "User", foreign_key: "report_id", optional: true
???
end
Essentially, if the :pod exists, a manager is not needed because :manager will be pod.owner.
Because I'm an idiot I tried to monkey patch it thusly:
def manager
(pod.nil?) ? manager : pod.owner
end
I'll leave it as an exercise to the user as to why this is nonsense :D
Any thoughts?
ruby-on-rails activerecord relational-database
add a comment |
I'm trying to do something, I'm not sure if it's possible...
I need a User, who needs a Manager. BUT, if the User has a Pod, their manager can be derived from the Pod.owner (in fact, it must be).
So, something like:
class User
belongs_to :pod, optional: true
belongs_to :manager, class_name: "User", foreign_key: "report_id", optional: true
???
end
Essentially, if the :pod exists, a manager is not needed because :manager will be pod.owner.
Because I'm an idiot I tried to monkey patch it thusly:
def manager
(pod.nil?) ? manager : pod.owner
end
I'll leave it as an exercise to the user as to why this is nonsense :D
Any thoughts?
ruby-on-rails activerecord relational-database
add a comment |
I'm trying to do something, I'm not sure if it's possible...
I need a User, who needs a Manager. BUT, if the User has a Pod, their manager can be derived from the Pod.owner (in fact, it must be).
So, something like:
class User
belongs_to :pod, optional: true
belongs_to :manager, class_name: "User", foreign_key: "report_id", optional: true
???
end
Essentially, if the :pod exists, a manager is not needed because :manager will be pod.owner.
Because I'm an idiot I tried to monkey patch it thusly:
def manager
(pod.nil?) ? manager : pod.owner
end
I'll leave it as an exercise to the user as to why this is nonsense :D
Any thoughts?
ruby-on-rails activerecord relational-database
I'm trying to do something, I'm not sure if it's possible...
I need a User, who needs a Manager. BUT, if the User has a Pod, their manager can be derived from the Pod.owner (in fact, it must be).
So, something like:
class User
belongs_to :pod, optional: true
belongs_to :manager, class_name: "User", foreign_key: "report_id", optional: true
???
end
Essentially, if the :pod exists, a manager is not needed because :manager will be pod.owner.
Because I'm an idiot I tried to monkey patch it thusly:
def manager
(pod.nil?) ? manager : pod.owner
end
I'll leave it as an exercise to the user as to why this is nonsense :D
Any thoughts?
ruby-on-rails activerecord relational-database
ruby-on-rails activerecord relational-database
asked Nov 14 '18 at 18:01


Paul HarkerPaul Harker
5618
5618
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
That's an interesting design problem in my opinion and I'm sure there are plenty of valid solutions. Here is the first that comes to my mind. Instead of overriding the manager method that's defined by the association, I would rename the manager
association to direct_manager
. I makes its role explicit. Then, you can define manager
as it's no longer defined. The end result would be:
class User
belongs_to :pod, optional: true
belongs_to :direct_manager, class_name: "User", foreign_key: "report_id", optional: true
def manager
return pod.owner if pod.present?
direct_manager
end
end
```
Note that I used a guard clause instead of the ternary operator, but it's only a matter of personal taste on the code style.
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've trieddef manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able toUser.create!(manager: boris)
and have it assign direct_manager...
– Paul Harker
Nov 15 '18 at 10:07
1
Figured it out!def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.
– Paul Harker
Nov 15 '18 at 10:14
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306260%2fmodel-relationships-dependant-on-others%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
That's an interesting design problem in my opinion and I'm sure there are plenty of valid solutions. Here is the first that comes to my mind. Instead of overriding the manager method that's defined by the association, I would rename the manager
association to direct_manager
. I makes its role explicit. Then, you can define manager
as it's no longer defined. The end result would be:
class User
belongs_to :pod, optional: true
belongs_to :direct_manager, class_name: "User", foreign_key: "report_id", optional: true
def manager
return pod.owner if pod.present?
direct_manager
end
end
```
Note that I used a guard clause instead of the ternary operator, but it's only a matter of personal taste on the code style.
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've trieddef manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able toUser.create!(manager: boris)
and have it assign direct_manager...
– Paul Harker
Nov 15 '18 at 10:07
1
Figured it out!def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.
– Paul Harker
Nov 15 '18 at 10:14
add a comment |
That's an interesting design problem in my opinion and I'm sure there are plenty of valid solutions. Here is the first that comes to my mind. Instead of overriding the manager method that's defined by the association, I would rename the manager
association to direct_manager
. I makes its role explicit. Then, you can define manager
as it's no longer defined. The end result would be:
class User
belongs_to :pod, optional: true
belongs_to :direct_manager, class_name: "User", foreign_key: "report_id", optional: true
def manager
return pod.owner if pod.present?
direct_manager
end
end
```
Note that I used a guard clause instead of the ternary operator, but it's only a matter of personal taste on the code style.
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've trieddef manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able toUser.create!(manager: boris)
and have it assign direct_manager...
– Paul Harker
Nov 15 '18 at 10:07
1
Figured it out!def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.
– Paul Harker
Nov 15 '18 at 10:14
add a comment |
That's an interesting design problem in my opinion and I'm sure there are plenty of valid solutions. Here is the first that comes to my mind. Instead of overriding the manager method that's defined by the association, I would rename the manager
association to direct_manager
. I makes its role explicit. Then, you can define manager
as it's no longer defined. The end result would be:
class User
belongs_to :pod, optional: true
belongs_to :direct_manager, class_name: "User", foreign_key: "report_id", optional: true
def manager
return pod.owner if pod.present?
direct_manager
end
end
```
Note that I used a guard clause instead of the ternary operator, but it's only a matter of personal taste on the code style.
That's an interesting design problem in my opinion and I'm sure there are plenty of valid solutions. Here is the first that comes to my mind. Instead of overriding the manager method that's defined by the association, I would rename the manager
association to direct_manager
. I makes its role explicit. Then, you can define manager
as it's no longer defined. The end result would be:
class User
belongs_to :pod, optional: true
belongs_to :direct_manager, class_name: "User", foreign_key: "report_id", optional: true
def manager
return pod.owner if pod.present?
direct_manager
end
end
```
Note that I used a guard clause instead of the ternary operator, but it's only a matter of personal taste on the code style.
answered Nov 14 '18 at 18:49
Sophie DézielSophie Déziel
404211
404211
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've trieddef manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able toUser.create!(manager: boris)
and have it assign direct_manager...
– Paul Harker
Nov 15 '18 at 10:07
1
Figured it out!def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.
– Paul Harker
Nov 15 '18 at 10:14
add a comment |
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've trieddef manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able toUser.create!(manager: boris)
and have it assign direct_manager...
– Paul Harker
Nov 15 '18 at 10:07
1
Figured it out!def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.
– Paul Harker
Nov 15 '18 at 10:14
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
This is great! Thanks so much
– Paul Harker
Nov 15 '18 at 8:50
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've tried
def manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able to User.create!(manager: boris)
and have it assign direct_manager...– Paul Harker
Nov 15 '18 at 10:07
Hi Sophie - or anyone else who see this - I now need to overload manager= to create users... I've tried
def manager= (user); direct_manager = user; save; end
but this doesn't work. I need to be able to User.create!(manager: boris)
and have it assign direct_manager...– Paul Harker
Nov 15 '18 at 10:07
1
1
Figured it out!
def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.– Paul Harker
Nov 15 '18 at 10:14
Figured it out!
def manager= (user); self[:report_id] = user.id; end
Thanks again for the direction.– Paul Harker
Nov 15 '18 at 10:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53306260%2fmodel-relationships-dependant-on-others%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2qD7aL,RU QErTKEepWQ,hONIaxS1MFxB60f0UJO tW c,YX74CVPz4,21beesaM3r1PRQWa,MMmSP,Ur6wBn 6PbCQmvRqwEjT2OUYlzsps