Asynchronous nature of this.setState in React
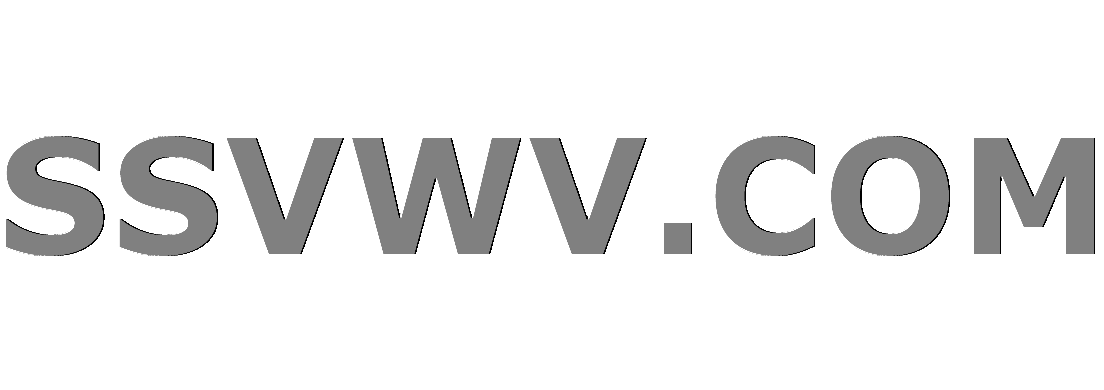
Multi tool use
Let's say that I have these two calls:
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment + 1
}));
Because setState is asynchronous, how is it guaranteed that the first call to it will execute first?
javascript reactjs asynchronous
add a comment |
Let's say that I have these two calls:
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment + 1
}));
Because setState is asynchronous, how is it guaranteed that the first call to it will execute first?
javascript reactjs asynchronous
1
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52
add a comment |
Let's say that I have these two calls:
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment + 1
}));
Because setState is asynchronous, how is it guaranteed that the first call to it will execute first?
javascript reactjs asynchronous
Let's say that I have these two calls:
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment
}));
this.setState((prevState, props) => ({
counter: prevState.counter + props.increment + 1
}));
Because setState is asynchronous, how is it guaranteed that the first call to it will execute first?
javascript reactjs asynchronous
javascript reactjs asynchronous
asked Nov 14 '18 at 3:47


LearningMathLearningMath
373220
373220
1
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52
add a comment |
1
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52
1
1
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52
add a comment |
1 Answer
1
active
oldest
votes
From the react documentation of setState() states that
setState()
enqueues changes to the component state and tells React that this component and its children need to be re-rendered with the updated state. setState() is also asynchronous, and multiple calls during the same cycle may be batched together. For example, if you attempt to increment or add a value to a counter more than once in the same cycle, that will result in the equivalent of:
Object.assign(
previousState,
{counter: previousState.counter + props.increment},
{counter: previousState.counter + props.increment + 1},
...
)
Subsequent calls will override values from previous calls in the same cycle, so the quantity will only be incremented once. If the next state depends on the current state, we recommend using the updater function form, instead:
this.setState((state) => {
return {counter: state.counter + 1};
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292915%2fasynchronous-nature-of-this-setstate-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
From the react documentation of setState() states that
setState()
enqueues changes to the component state and tells React that this component and its children need to be re-rendered with the updated state. setState() is also asynchronous, and multiple calls during the same cycle may be batched together. For example, if you attempt to increment or add a value to a counter more than once in the same cycle, that will result in the equivalent of:
Object.assign(
previousState,
{counter: previousState.counter + props.increment},
{counter: previousState.counter + props.increment + 1},
...
)
Subsequent calls will override values from previous calls in the same cycle, so the quantity will only be incremented once. If the next state depends on the current state, we recommend using the updater function form, instead:
this.setState((state) => {
return {counter: state.counter + 1};
});
add a comment |
From the react documentation of setState() states that
setState()
enqueues changes to the component state and tells React that this component and its children need to be re-rendered with the updated state. setState() is also asynchronous, and multiple calls during the same cycle may be batched together. For example, if you attempt to increment or add a value to a counter more than once in the same cycle, that will result in the equivalent of:
Object.assign(
previousState,
{counter: previousState.counter + props.increment},
{counter: previousState.counter + props.increment + 1},
...
)
Subsequent calls will override values from previous calls in the same cycle, so the quantity will only be incremented once. If the next state depends on the current state, we recommend using the updater function form, instead:
this.setState((state) => {
return {counter: state.counter + 1};
});
add a comment |
From the react documentation of setState() states that
setState()
enqueues changes to the component state and tells React that this component and its children need to be re-rendered with the updated state. setState() is also asynchronous, and multiple calls during the same cycle may be batched together. For example, if you attempt to increment or add a value to a counter more than once in the same cycle, that will result in the equivalent of:
Object.assign(
previousState,
{counter: previousState.counter + props.increment},
{counter: previousState.counter + props.increment + 1},
...
)
Subsequent calls will override values from previous calls in the same cycle, so the quantity will only be incremented once. If the next state depends on the current state, we recommend using the updater function form, instead:
this.setState((state) => {
return {counter: state.counter + 1};
});
From the react documentation of setState() states that
setState()
enqueues changes to the component state and tells React that this component and its children need to be re-rendered with the updated state. setState() is also asynchronous, and multiple calls during the same cycle may be batched together. For example, if you attempt to increment or add a value to a counter more than once in the same cycle, that will result in the equivalent of:
Object.assign(
previousState,
{counter: previousState.counter + props.increment},
{counter: previousState.counter + props.increment + 1},
...
)
Subsequent calls will override values from previous calls in the same cycle, so the quantity will only be incremented once. If the next state depends on the current state, we recommend using the updater function form, instead:
this.setState((state) => {
return {counter: state.counter + 1};
});
edited Nov 14 '18 at 8:25
answered Nov 14 '18 at 4:06
Samuel J MathewSamuel J Mathew
3,62212229
3,62212229
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53292915%2fasynchronous-nature-of-this-setstate-in-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
r4 b7 u5 UUQ3eMd Gu6cortYoL uXsQNt hgjaw eLQFJ33
1
Just imagine a queue - first in, first out, regardless of the precise timing of each.
– CertainPerformance
Nov 14 '18 at 3:49
But this has to do with the Javascript engine, and nothing to do with React itself, right?
– LearningMath
Nov 14 '18 at 3:51
Well, it's React that's implementing the processing of the queue, so it is up to React's code - it would be quite possible to implement something else yourself that accepted items and resolved them asynchronously, in a random order, rather than in FIFO order (but that would be confusing)
– CertainPerformance
Nov 14 '18 at 3:52