How to Skip Span Element Using jQuery Next Function
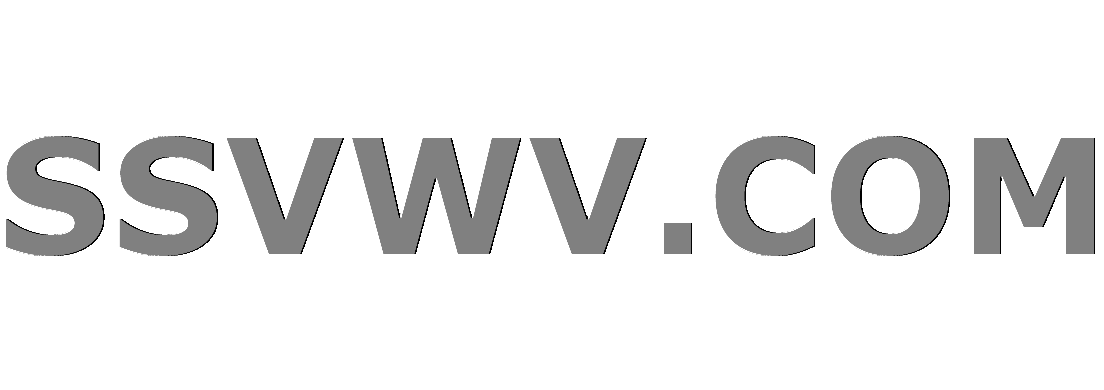
Multi tool use
I have a panel which has to be like this
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
I need to target the dynamically added .prev
by using jQuery next()
function but looks like span is bugging this selection?~
Can you please let me know how to skip the span
here?
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
javascript jquery
add a comment |
I have a panel which has to be like this
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
I need to target the dynamically added .prev
by using jQuery next()
function but looks like span is bugging this selection?~
Can you please let me know how to skip the span
here?
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
javascript jquery
1
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27
add a comment |
I have a panel which has to be like this
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
I need to target the dynamically added .prev
by using jQuery next()
function but looks like span is bugging this selection?~
Can you please let me know how to skip the span
here?
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
javascript jquery
I have a panel which has to be like this
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
I need to target the dynamically added .prev
by using jQuery next()
function but looks like span is bugging this selection?~
Can you please let me know how to skip the span
here?
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).next(".prev").addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
javascript jquery
javascript jquery
asked Nov 13 '18 at 19:09


Mona CoderMona Coder
2,409134083
2,409134083
1
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27
add a comment |
1
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27
1
1
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27
add a comment |
3 Answers
3
active
oldest
votes
To remove the positional based logic of your issue, I would suggest you encapsulate your elements and use parent()
or closest()
and perform a contextual lookup rather than using next() or prev().
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
Using logic like this, you don't care if there is a span, or where it is. You know that the prev belongs to the wrapper.
add a comment |
You can use nextAll(".prev")
to get all siblings of that class (.next()
only fetches the immediate sibling), then isolate the first of that type with .eq(0)
Like in this snippet!
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
add a comment |
.next()
only checks the next element. If you include a selector, it either returns the next element if it matches, or nothing.
Consider using .siblings(".prev")
(all siblings with class prev
) combined with .first()
(the first one only).
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287955%2fhow-to-skip-span-element-using-jquery-next-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
To remove the positional based logic of your issue, I would suggest you encapsulate your elements and use parent()
or closest()
and perform a contextual lookup rather than using next() or prev().
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
Using logic like this, you don't care if there is a span, or where it is. You know that the prev belongs to the wrapper.
add a comment |
To remove the positional based logic of your issue, I would suggest you encapsulate your elements and use parent()
or closest()
and perform a contextual lookup rather than using next() or prev().
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
Using logic like this, you don't care if there is a span, or where it is. You know that the prev belongs to the wrapper.
add a comment |
To remove the positional based logic of your issue, I would suggest you encapsulate your elements and use parent()
or closest()
and perform a contextual lookup rather than using next() or prev().
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
Using logic like this, you don't care if there is a span, or where it is. You know that the prev belongs to the wrapper.
To remove the positional based logic of your issue, I would suggest you encapsulate your elements and use parent()
or closest()
and perform a contextual lookup rather than using next() or prev().
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
Using logic like this, you don't care if there is a span, or where it is. You know that the prev belongs to the wrapper.
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
$(document).on("click", ".next", function() {
$(this).closest('.navigation-wrapper').find('.prev').addClass('red');
});
.red {
background-color: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<span class="navigation-wrapper">
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
</span>
answered Nov 13 '18 at 19:19
TaplarTaplar
16k21529
16k21529
add a comment |
add a comment |
You can use nextAll(".prev")
to get all siblings of that class (.next()
only fetches the immediate sibling), then isolate the first of that type with .eq(0)
Like in this snippet!
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
add a comment |
You can use nextAll(".prev")
to get all siblings of that class (.next()
only fetches the immediate sibling), then isolate the first of that type with .eq(0)
Like in this snippet!
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
add a comment |
You can use nextAll(".prev")
to get all siblings of that class (.next()
only fetches the immediate sibling), then isolate the first of that type with .eq(0)
Like in this snippet!
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
You can use nextAll(".prev")
to get all siblings of that class (.next()
only fetches the immediate sibling), then isolate the first of that type with .eq(0)
Like in this snippet!
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).nextAll(".prev").eq(0).addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
answered Nov 13 '18 at 19:22
Michael BeesonMichael Beeson
1,0942913
1,0942913
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
add a comment |
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
Again, this answer seems to accomplish exactly what OP requested, yet has also received a downvote with no explanation :/
– Tyler Roper
Nov 13 '18 at 19:24
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
I dv your answer, and uv your comment. The code, while answer the question explicity, is also extremely fragile in that it relies on the order of HTML for the functionality to work. For example, wrapping buttons causes your code not to work. See my comment to the question.
– Erik Philips
Nov 13 '18 at 19:28
add a comment |
.next()
only checks the next element. If you include a selector, it either returns the next element if it matches, or nothing.
Consider using .siblings(".prev")
(all siblings with class prev
) combined with .first()
(the first one only).
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
add a comment |
.next()
only checks the next element. If you include a selector, it either returns the next element if it matches, or nothing.
Consider using .siblings(".prev")
(all siblings with class prev
) combined with .first()
(the first one only).
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
add a comment |
.next()
only checks the next element. If you include a selector, it either returns the next element if it matches, or nothing.
Consider using .siblings(".prev")
(all siblings with class prev
) combined with .first()
(the first one only).
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
.next()
only checks the next element. If you include a selector, it either returns the next element if it matches, or nothing.
Consider using .siblings(".prev")
(all siblings with class prev
) combined with .first()
(the first one only).
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
$(document).on("click", ".next", function() {
$(this).siblings(".prev").first().addClass('red');
});
.red {
background: red;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<button class="next">Next</button>
<span>Test</span>
<button class="prev">Prev</button>
answered Nov 13 '18 at 19:12


Tyler RoperTyler Roper
13.6k31941
13.6k31941
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53287955%2fhow-to-skip-span-element-using-jquery-next-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kqSQcStaaG,NmkDt 3C3X7U,d96nkF4T,e K,38d4bvfo,Um NFWj6vy W wm4YL5lpm6pOcVlR OF5 B15uI,x0mU,mWW02GRV8
1
If you know the span is always there, you could just do next().next('.prev') . The selector for the next operates as a filter. It doesn't make it go next until it finds a match. It filters out the next one if it does not match.
– Taplar
Nov 13 '18 at 19:11
I highly recommend reading Decoupling Your HTML, CSS, and JavaScript.
– Erik Philips
Nov 13 '18 at 19:27