How can I get the user's local time in Swift?
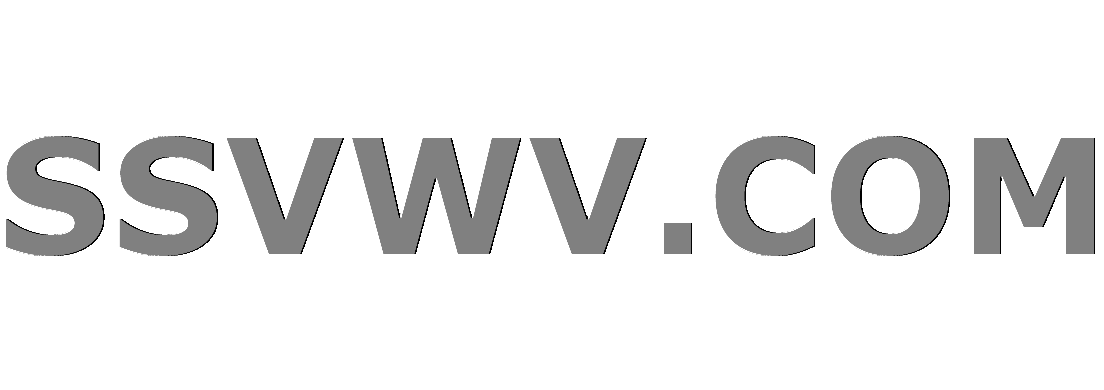
Multi tool use
up vote
0
down vote
favorite
I am making an app which among other things, it determines the sunset and sunrise. Nevertheless I've found some difficulties to get them using the user's local time. Therefore, I would like to know: how can I get the user's time zone in Swift?
The coordinates of my test location (Puerto Vallarta, Mex) that I load in the iPhone simulator are these:
Latitude: 20.61
Longitude: -106.23
The next is part of my code to determine the sunrise and the sunset of any location in the world:
//First we get the sunrise time.
let sunriseDate = Date(timeIntervalSince1970: sunriseValue.doubleValue)
let formatter = DateFormatter()
formatter.dateStyle = .none
formatter.timeStyle = .medium
formatter.timeZone = NSTimeZone(name: "UTC")! as TimeZone
formatter.dateFormat = "HH:mm:ss"
let sunrise = formatter.string(from: sunriseDate)
print(sunrise)
//Second, we get the sunset time
let sunsetDate = Date(timeIntervalSince1970: sunsetValue.doubleValue)
let sunset = formatter.string(from: sunsetDate)
print(sunset)
And this is what I get from the console:
Sunrise: 13:08:12
Sunset: 00:21:22
This is more than evident that the printed sunrise and sunset correspond to the UTC, nevertheless I haven't found any direct information that could help me to get the user's local time, wherever that person is.
Basically the sunrise and sunset that you see in the code above I got it from an API (openweather) from which I got their values in unix time (which I converted to date and time using this link . Now I see that I effectively got the correct sunset and sunrise but in UTC format, now I'm missing one step to go from UTC to local time.
So, in summary, I got:
from API: time in unix time (e.g. sunrise = 1541855294; sunset = 1541895681;), extract from it the time using DateFormatter() (see code above) and I got the UTC time (formatter.dateFormat = "HH:mm:ss").
I hope that with this modification the question is more clear now.
That's why I wanted to ask you if you cand lend me a hand with this, please :)
Thanks in advance and I apologize for any misunderstanding!
swift timezone
|
show 5 more comments
up vote
0
down vote
favorite
I am making an app which among other things, it determines the sunset and sunrise. Nevertheless I've found some difficulties to get them using the user's local time. Therefore, I would like to know: how can I get the user's time zone in Swift?
The coordinates of my test location (Puerto Vallarta, Mex) that I load in the iPhone simulator are these:
Latitude: 20.61
Longitude: -106.23
The next is part of my code to determine the sunrise and the sunset of any location in the world:
//First we get the sunrise time.
let sunriseDate = Date(timeIntervalSince1970: sunriseValue.doubleValue)
let formatter = DateFormatter()
formatter.dateStyle = .none
formatter.timeStyle = .medium
formatter.timeZone = NSTimeZone(name: "UTC")! as TimeZone
formatter.dateFormat = "HH:mm:ss"
let sunrise = formatter.string(from: sunriseDate)
print(sunrise)
//Second, we get the sunset time
let sunsetDate = Date(timeIntervalSince1970: sunsetValue.doubleValue)
let sunset = formatter.string(from: sunsetDate)
print(sunset)
And this is what I get from the console:
Sunrise: 13:08:12
Sunset: 00:21:22
This is more than evident that the printed sunrise and sunset correspond to the UTC, nevertheless I haven't found any direct information that could help me to get the user's local time, wherever that person is.
Basically the sunrise and sunset that you see in the code above I got it from an API (openweather) from which I got their values in unix time (which I converted to date and time using this link . Now I see that I effectively got the correct sunset and sunrise but in UTC format, now I'm missing one step to go from UTC to local time.
So, in summary, I got:
from API: time in unix time (e.g. sunrise = 1541855294; sunset = 1541895681;), extract from it the time using DateFormatter() (see code above) and I got the UTC time (formatter.dateFormat = "HH:mm:ss").
I hope that with this modification the question is more clear now.
That's why I wanted to ask you if you cand lend me a hand with this, please :)
Thanks in advance and I apologize for any misunderstanding!
swift timezone
1
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
BTW - If you ever do use a time zone, useTimeZone
, notNSTimeZone
.
– rmaddy
Nov 10 at 17:42
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58
|
show 5 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am making an app which among other things, it determines the sunset and sunrise. Nevertheless I've found some difficulties to get them using the user's local time. Therefore, I would like to know: how can I get the user's time zone in Swift?
The coordinates of my test location (Puerto Vallarta, Mex) that I load in the iPhone simulator are these:
Latitude: 20.61
Longitude: -106.23
The next is part of my code to determine the sunrise and the sunset of any location in the world:
//First we get the sunrise time.
let sunriseDate = Date(timeIntervalSince1970: sunriseValue.doubleValue)
let formatter = DateFormatter()
formatter.dateStyle = .none
formatter.timeStyle = .medium
formatter.timeZone = NSTimeZone(name: "UTC")! as TimeZone
formatter.dateFormat = "HH:mm:ss"
let sunrise = formatter.string(from: sunriseDate)
print(sunrise)
//Second, we get the sunset time
let sunsetDate = Date(timeIntervalSince1970: sunsetValue.doubleValue)
let sunset = formatter.string(from: sunsetDate)
print(sunset)
And this is what I get from the console:
Sunrise: 13:08:12
Sunset: 00:21:22
This is more than evident that the printed sunrise and sunset correspond to the UTC, nevertheless I haven't found any direct information that could help me to get the user's local time, wherever that person is.
Basically the sunrise and sunset that you see in the code above I got it from an API (openweather) from which I got their values in unix time (which I converted to date and time using this link . Now I see that I effectively got the correct sunset and sunrise but in UTC format, now I'm missing one step to go from UTC to local time.
So, in summary, I got:
from API: time in unix time (e.g. sunrise = 1541855294; sunset = 1541895681;), extract from it the time using DateFormatter() (see code above) and I got the UTC time (formatter.dateFormat = "HH:mm:ss").
I hope that with this modification the question is more clear now.
That's why I wanted to ask you if you cand lend me a hand with this, please :)
Thanks in advance and I apologize for any misunderstanding!
swift timezone
I am making an app which among other things, it determines the sunset and sunrise. Nevertheless I've found some difficulties to get them using the user's local time. Therefore, I would like to know: how can I get the user's time zone in Swift?
The coordinates of my test location (Puerto Vallarta, Mex) that I load in the iPhone simulator are these:
Latitude: 20.61
Longitude: -106.23
The next is part of my code to determine the sunrise and the sunset of any location in the world:
//First we get the sunrise time.
let sunriseDate = Date(timeIntervalSince1970: sunriseValue.doubleValue)
let formatter = DateFormatter()
formatter.dateStyle = .none
formatter.timeStyle = .medium
formatter.timeZone = NSTimeZone(name: "UTC")! as TimeZone
formatter.dateFormat = "HH:mm:ss"
let sunrise = formatter.string(from: sunriseDate)
print(sunrise)
//Second, we get the sunset time
let sunsetDate = Date(timeIntervalSince1970: sunsetValue.doubleValue)
let sunset = formatter.string(from: sunsetDate)
print(sunset)
And this is what I get from the console:
Sunrise: 13:08:12
Sunset: 00:21:22
This is more than evident that the printed sunrise and sunset correspond to the UTC, nevertheless I haven't found any direct information that could help me to get the user's local time, wherever that person is.
Basically the sunrise and sunset that you see in the code above I got it from an API (openweather) from which I got their values in unix time (which I converted to date and time using this link . Now I see that I effectively got the correct sunset and sunrise but in UTC format, now I'm missing one step to go from UTC to local time.
So, in summary, I got:
from API: time in unix time (e.g. sunrise = 1541855294; sunset = 1541895681;), extract from it the time using DateFormatter() (see code above) and I got the UTC time (formatter.dateFormat = "HH:mm:ss").
I hope that with this modification the question is more clear now.
That's why I wanted to ask you if you cand lend me a hand with this, please :)
Thanks in advance and I apologize for any misunderstanding!
swift timezone
swift timezone
edited Nov 10 at 21:49
asked Nov 10 at 17:21


J_A_F_Casillas
12
12
1
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
BTW - If you ever do use a time zone, useTimeZone
, notNSTimeZone
.
– rmaddy
Nov 10 at 17:42
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58
|
show 5 more comments
1
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
BTW - If you ever do use a time zone, useTimeZone
, notNSTimeZone
.
– rmaddy
Nov 10 at 17:42
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58
1
1
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
BTW - If you ever do use a time zone, use
TimeZone
, not NSTimeZone
.– rmaddy
Nov 10 at 17:42
BTW - If you ever do use a time zone, use
TimeZone
, not NSTimeZone
.– rmaddy
Nov 10 at 17:42
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58
|
show 5 more comments
1 Answer
1
active
oldest
votes
up vote
-1
down vote
Did you try to use the Date method description(with locale: Locale?) to get user's localized time ? This will give you the time of the device
let date = Date().description(with: .current)
print(date) //Saturday, November 10, 2018 at 11:30:58 AM Central Standard Time
A string representation of the Date, using the given locale, or if the locale argument is nil, in the international format YYYY-MM-DD HH:MM:SS ±HHMM, where ±HHMM represents the time zone offset in hours and minutes from UTC (for example, “2001-03-24 10:45:32 +0600”).
After that you can give it any formatt that you want...
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with thedescription(with:)
method anddate
is aString
, not aDate
. Please clarify how using thedescription(with:)
method ofDate
gives the results desired by the OP.
– rmaddy
Nov 10 at 18:57
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
-1
down vote
Did you try to use the Date method description(with locale: Locale?) to get user's localized time ? This will give you the time of the device
let date = Date().description(with: .current)
print(date) //Saturday, November 10, 2018 at 11:30:58 AM Central Standard Time
A string representation of the Date, using the given locale, or if the locale argument is nil, in the international format YYYY-MM-DD HH:MM:SS ±HHMM, where ±HHMM represents the time zone offset in hours and minutes from UTC (for example, “2001-03-24 10:45:32 +0600”).
After that you can give it any formatt that you want...
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with thedescription(with:)
method anddate
is aString
, not aDate
. Please clarify how using thedescription(with:)
method ofDate
gives the results desired by the OP.
– rmaddy
Nov 10 at 18:57
add a comment |
up vote
-1
down vote
Did you try to use the Date method description(with locale: Locale?) to get user's localized time ? This will give you the time of the device
let date = Date().description(with: .current)
print(date) //Saturday, November 10, 2018 at 11:30:58 AM Central Standard Time
A string representation of the Date, using the given locale, or if the locale argument is nil, in the international format YYYY-MM-DD HH:MM:SS ±HHMM, where ±HHMM represents the time zone offset in hours and minutes from UTC (for example, “2001-03-24 10:45:32 +0600”).
After that you can give it any formatt that you want...
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with thedescription(with:)
method anddate
is aString
, not aDate
. Please clarify how using thedescription(with:)
method ofDate
gives the results desired by the OP.
– rmaddy
Nov 10 at 18:57
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Did you try to use the Date method description(with locale: Locale?) to get user's localized time ? This will give you the time of the device
let date = Date().description(with: .current)
print(date) //Saturday, November 10, 2018 at 11:30:58 AM Central Standard Time
A string representation of the Date, using the given locale, or if the locale argument is nil, in the international format YYYY-MM-DD HH:MM:SS ±HHMM, where ±HHMM represents the time zone offset in hours and minutes from UTC (for example, “2001-03-24 10:45:32 +0600”).
After that you can give it any formatt that you want...
Did you try to use the Date method description(with locale: Locale?) to get user's localized time ? This will give you the time of the device
let date = Date().description(with: .current)
print(date) //Saturday, November 10, 2018 at 11:30:58 AM Central Standard Time
A string representation of the Date, using the given locale, or if the locale argument is nil, in the international format YYYY-MM-DD HH:MM:SS ±HHMM, where ±HHMM represents the time zone offset in hours and minutes from UTC (for example, “2001-03-24 10:45:32 +0600”).
After that you can give it any formatt that you want...
answered Nov 10 at 17:47


Gabo MC
86
86
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with thedescription(with:)
method anddate
is aString
, not aDate
. Please clarify how using thedescription(with:)
method ofDate
gives the results desired by the OP.
– rmaddy
Nov 10 at 18:57
add a comment |
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with thedescription(with:)
method anddate
is aString
, not aDate
. Please clarify how using thedescription(with:)
method ofDate
gives the results desired by the OP.
– rmaddy
Nov 10 at 18:57
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
This should only be used for debugging, not for display to a user. And the OP only wants the time, not the date.
– rmaddy
Nov 10 at 17:54
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
as i wrote at the end of my comment..."you can give the the format that you want..." (HH:MM:SS) and work with that values to compare with sunrise or sunset values..
– Gabo MC
Nov 10 at 18:51
You can't set a format with the
description(with:)
method and date
is a String
, not a Date
. Please clarify how using the description(with:)
method of Date
gives the results desired by the OP.– rmaddy
Nov 10 at 18:57
You can't set a format with the
description(with:)
method and date
is a String
, not a Date
. Please clarify how using the description(with:)
method of Date
gives the results desired by the OP.– rmaddy
Nov 10 at 18:57
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53241504%2fhow-can-i-get-the-users-local-time-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tbdsHGVQFoMLl7plVgT TjG,o1
1
Did you try not setting formatter.timeZone? Then the timezone from the user's settings should be used.
– Martin R
Nov 10 at 17:25
BTW - If you ever do use a time zone, use
TimeZone
, notNSTimeZone
.– rmaddy
Nov 10 at 17:42
Do you want to show the times in the user's local time or in the local time of the coordinate? For the latter you would need to know the timezone of the coordinate.
– rmaddy
Nov 10 at 17:44
Hello rmaddy. I want to keep it simple so I just want to show the user's time (only the time HH:mm:ss) @M
– J_A_F_Casillas
Nov 10 at 18:15
@JorgeAlbertoFuentesCasillas Then simply remove the line where you set the formatter's timeZone property and your are done, just like Martin stated in the 1st comment.
– rmaddy
Nov 10 at 18:58