How To Solve NodeJS Method Priority Problem
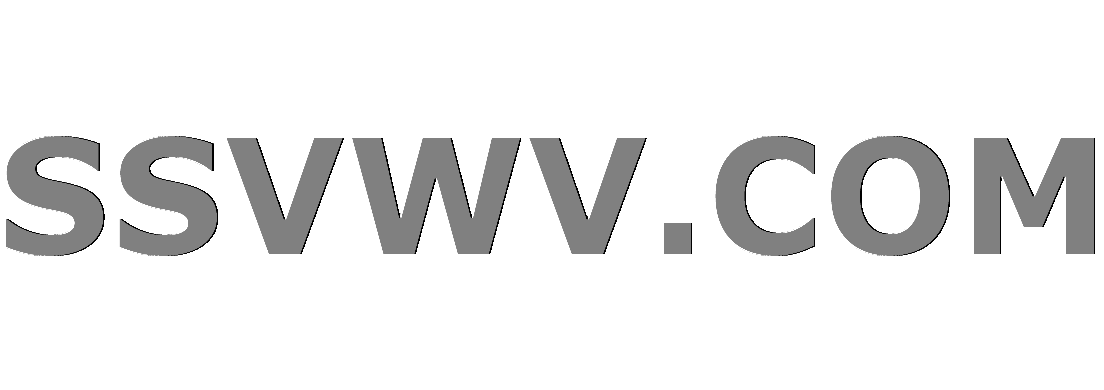
Multi tool use
up vote
3
down vote
favorite
In this case, method 3 works first and i am getting error. Its priority has to be like method 1, method 2, and method 3. Are these methods promises ? And promises work as asynchronous.
I want check if new user's username and email in use or not. If username or email are not in use, then register it.
How to i solve this problem ? I'm new at nodejs.
module.exports.addUser = function(newUser, callback) {
// method 1
User.countDocuments({username: newUser.username}).then(count => {
if(count > 0) {
console.log("username in use");
callback("username in use", null);
return;
}});
// method 2
User.countDocuments({email: newUser.email}).then(count => {
if(count > 0) {
console.log("email in use");
callback("email in use", null);
return;
}});
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if(err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
Output:
salt here
username in use
email in use
(node:7972) UnhandledPromiseRejectionWarning: Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:20:17)
at User.countDocuments.then.count (C:UserscycloneDesktopmy_authmodelsuser.js:48:13)
at <anonymous>
at process._tickCallback (internal/process/next_tick.js:189:7)
(node:7972) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 1)
(node:7972) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled
will terminate the Node.js process with a non-zero exit code.
events.js:183
throw er; // Unhandled 'error' event
^
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:22:17)
at C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:4518:16
at model.$__save.error (C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:422:7)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:315:21
at next (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:209:27)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:182:9
at process.nextTick (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:499:38)
at _combinedTickCallback (internal/process/next_tick.js:132:7)
at process._tickCallback (internal/process/next_tick.js:181:9)
[nodemon] app crashed - waiting for file changes before starting...
javascript node.js mongodb mongoose
add a comment |
up vote
3
down vote
favorite
In this case, method 3 works first and i am getting error. Its priority has to be like method 1, method 2, and method 3. Are these methods promises ? And promises work as asynchronous.
I want check if new user's username and email in use or not. If username or email are not in use, then register it.
How to i solve this problem ? I'm new at nodejs.
module.exports.addUser = function(newUser, callback) {
// method 1
User.countDocuments({username: newUser.username}).then(count => {
if(count > 0) {
console.log("username in use");
callback("username in use", null);
return;
}});
// method 2
User.countDocuments({email: newUser.email}).then(count => {
if(count > 0) {
console.log("email in use");
callback("email in use", null);
return;
}});
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if(err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
Output:
salt here
username in use
email in use
(node:7972) UnhandledPromiseRejectionWarning: Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:20:17)
at User.countDocuments.then.count (C:UserscycloneDesktopmy_authmodelsuser.js:48:13)
at <anonymous>
at process._tickCallback (internal/process/next_tick.js:189:7)
(node:7972) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 1)
(node:7972) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled
will terminate the Node.js process with a non-zero exit code.
events.js:183
throw er; // Unhandled 'error' event
^
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:22:17)
at C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:4518:16
at model.$__save.error (C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:422:7)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:315:21
at next (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:209:27)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:182:9
at process.nextTick (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:499:38)
at _combinedTickCallback (internal/process/next_tick.js:132:7)
at process._tickCallback (internal/process/next_tick.js:181:9)
[nodemon] app crashed - waiting for file changes before starting...
javascript node.js mongodb mongoose
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
In this case, method 3 works first and i am getting error. Its priority has to be like method 1, method 2, and method 3. Are these methods promises ? And promises work as asynchronous.
I want check if new user's username and email in use or not. If username or email are not in use, then register it.
How to i solve this problem ? I'm new at nodejs.
module.exports.addUser = function(newUser, callback) {
// method 1
User.countDocuments({username: newUser.username}).then(count => {
if(count > 0) {
console.log("username in use");
callback("username in use", null);
return;
}});
// method 2
User.countDocuments({email: newUser.email}).then(count => {
if(count > 0) {
console.log("email in use");
callback("email in use", null);
return;
}});
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if(err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
Output:
salt here
username in use
email in use
(node:7972) UnhandledPromiseRejectionWarning: Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:20:17)
at User.countDocuments.then.count (C:UserscycloneDesktopmy_authmodelsuser.js:48:13)
at <anonymous>
at process._tickCallback (internal/process/next_tick.js:189:7)
(node:7972) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 1)
(node:7972) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled
will terminate the Node.js process with a non-zero exit code.
events.js:183
throw er; // Unhandled 'error' event
^
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:22:17)
at C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:4518:16
at model.$__save.error (C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:422:7)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:315:21
at next (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:209:27)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:182:9
at process.nextTick (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:499:38)
at _combinedTickCallback (internal/process/next_tick.js:132:7)
at process._tickCallback (internal/process/next_tick.js:181:9)
[nodemon] app crashed - waiting for file changes before starting...
javascript node.js mongodb mongoose
In this case, method 3 works first and i am getting error. Its priority has to be like method 1, method 2, and method 3. Are these methods promises ? And promises work as asynchronous.
I want check if new user's username and email in use or not. If username or email are not in use, then register it.
How to i solve this problem ? I'm new at nodejs.
module.exports.addUser = function(newUser, callback) {
// method 1
User.countDocuments({username: newUser.username}).then(count => {
if(count > 0) {
console.log("username in use");
callback("username in use", null);
return;
}});
// method 2
User.countDocuments({email: newUser.email}).then(count => {
if(count > 0) {
console.log("email in use");
callback("email in use", null);
return;
}});
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if(err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
Output:
salt here
username in use
email in use
(node:7972) UnhandledPromiseRejectionWarning: Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:20:17)
at User.countDocuments.then.count (C:UserscycloneDesktopmy_authmodelsuser.js:48:13)
at <anonymous>
at process._tickCallback (internal/process/next_tick.js:189:7)
(node:7972) UnhandledPromiseRejectionWarning: Unhandled promise rejection. This error originated either by throwing inside of an async function without a catch block, or by rejecting a promise which was not handled with .catch(). (rejection id: 1)
(node:7972) [DEP0018] DeprecationWarning: Unhandled promise rejections are deprecated. In the future, promise rejections that are not handled
will terminate the Node.js process with a non-zero exit code.
events.js:183
throw er; // Unhandled 'error' event
^
Error: Can't set headers after they are sent.
at validateHeader (_http_outgoing.js:491:11)
at ServerResponse.setHeader (_http_outgoing.js:498:3)
at ServerResponse.header (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:767:10)
at ServerResponse.send (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:170:12)
at ServerResponse.json (C:UserscycloneDesktopmy_authnode_modulesexpresslibresponse.js:267:15)
at User.addUser (C:UserscycloneDesktopmy_authroutesusers.js:22:17)
at C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:4518:16
at model.$__save.error (C:UserscycloneDesktopmy_authnode_modulesmongooselibmodel.js:422:7)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:315:21
at next (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:209:27)
at C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:182:9
at process.nextTick (C:UserscycloneDesktopmy_authnode_moduleskareemindex.js:499:38)
at _combinedTickCallback (internal/process/next_tick.js:132:7)
at process._tickCallback (internal/process/next_tick.js:181:9)
[nodemon] app crashed - waiting for file changes before starting...
javascript node.js mongodb mongoose
javascript node.js mongodb mongoose
asked Nov 10 at 21:32
Şeref Can Muştu
192114
192114
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
The single fact that "then" is used after countDocuments
does indicate that it's a promise and thus asynchronous.
The easiest solution at this point, would be to define your addUser
function as async
.
module.exports.addUser = async function(newUser, callback) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
console.log("username in use");
callback("username in use", null);
return;
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
console.log("email in use");
callback("email in use", null);
return;
};
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
However, now it's quite pointless to have a callback function for addUser since an async function automatically returns a promise. I'd recommend doing something like so...
module.exports.addUser = async function(newUser) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
throw Error("username is in use");
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
throw Error("email in use");
};
let result = null;
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
result = await newUser.save(callback);
});
});
return result;
};
and in use it would look like:
addUser(someUserObject).then(result=>console.log(result)).catch(error=>{
//Example: username in use
console.log(error.message)
});
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
The single fact that "then" is used after countDocuments
does indicate that it's a promise and thus asynchronous.
The easiest solution at this point, would be to define your addUser
function as async
.
module.exports.addUser = async function(newUser, callback) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
console.log("username in use");
callback("username in use", null);
return;
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
console.log("email in use");
callback("email in use", null);
return;
};
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
However, now it's quite pointless to have a callback function for addUser since an async function automatically returns a promise. I'd recommend doing something like so...
module.exports.addUser = async function(newUser) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
throw Error("username is in use");
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
throw Error("email in use");
};
let result = null;
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
result = await newUser.save(callback);
});
});
return result;
};
and in use it would look like:
addUser(someUserObject).then(result=>console.log(result)).catch(error=>{
//Example: username in use
console.log(error.message)
});
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
add a comment |
up vote
2
down vote
accepted
The single fact that "then" is used after countDocuments
does indicate that it's a promise and thus asynchronous.
The easiest solution at this point, would be to define your addUser
function as async
.
module.exports.addUser = async function(newUser, callback) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
console.log("username in use");
callback("username in use", null);
return;
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
console.log("email in use");
callback("email in use", null);
return;
};
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
However, now it's quite pointless to have a callback function for addUser since an async function automatically returns a promise. I'd recommend doing something like so...
module.exports.addUser = async function(newUser) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
throw Error("username is in use");
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
throw Error("email in use");
};
let result = null;
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
result = await newUser.save(callback);
});
});
return result;
};
and in use it would look like:
addUser(someUserObject).then(result=>console.log(result)).catch(error=>{
//Example: username in use
console.log(error.message)
});
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
The single fact that "then" is used after countDocuments
does indicate that it's a promise and thus asynchronous.
The easiest solution at this point, would be to define your addUser
function as async
.
module.exports.addUser = async function(newUser, callback) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
console.log("username in use");
callback("username in use", null);
return;
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
console.log("email in use");
callback("email in use", null);
return;
};
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
However, now it's quite pointless to have a callback function for addUser since an async function automatically returns a promise. I'd recommend doing something like so...
module.exports.addUser = async function(newUser) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
throw Error("username is in use");
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
throw Error("email in use");
};
let result = null;
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
result = await newUser.save(callback);
});
});
return result;
};
and in use it would look like:
addUser(someUserObject).then(result=>console.log(result)).catch(error=>{
//Example: username in use
console.log(error.message)
});
The single fact that "then" is used after countDocuments
does indicate that it's a promise and thus asynchronous.
The easiest solution at this point, would be to define your addUser
function as async
.
module.exports.addUser = async function(newUser, callback) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
console.log("username in use");
callback("username in use", null);
return;
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
console.log("email in use");
callback("email in use", null);
return;
};
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
newUser.save(callback);
});
});
};
However, now it's quite pointless to have a callback function for addUser since an async function automatically returns a promise. I'd recommend doing something like so...
module.exports.addUser = async function(newUser) {
// method 1
const count1 = await User.countDocuments({
username: newUser.username
});
if (count1 > 0) {
throw Error("username is in use");
};
// method 2
const count2 = await User.countDocuments({
email: newUser.email
});
if (count2 > 0) {
throw Error("email in use");
};
let result = null;
// method 3 , this method works first
bcrypt.genSalt(10, (err, salt) => {
console.log("salt here");
bcrypt.hash(newUser.password, salt, (err, hash) => {
if (err) throw err;
newUser.password = hash;
result = await newUser.save(callback);
});
});
return result;
};
and in use it would look like:
addUser(someUserObject).then(result=>console.log(result)).catch(error=>{
//Example: username in use
console.log(error.message)
});
edited Nov 10 at 22:05
answered Nov 10 at 21:48


kemicofa
7,79343477
7,79343477
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
add a comment |
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
It solved my problem. Thank you so much. But i have a question. Do i have to use asynchronous methods while using mongoose ?
– Şeref Can Muştu
Nov 10 at 22:20
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
@ŞerefCanMuştu mongoose is asynchronous in nature, they offer a few synchronous method variants but not for all methods. So in general, if you're working with mongoose you'll be working with promises/asynchronous methods. You could just use promises everywhere... but for readability I'd recommend working with async/await. You could also work with co (npmjs.com/package/co) which is also recommended by mongoose documentation... though it's pretty much another way to do asynchronous functions.
– kemicofa
Nov 10 at 22:31
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53243614%2fhow-to-solve-nodejs-method-priority-problem%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UbtX071DUAlu HQXO VxM9bqr2kNd3CJba yJ,rHlnUg9