C# async/await with/without awaiting (fire and forget)
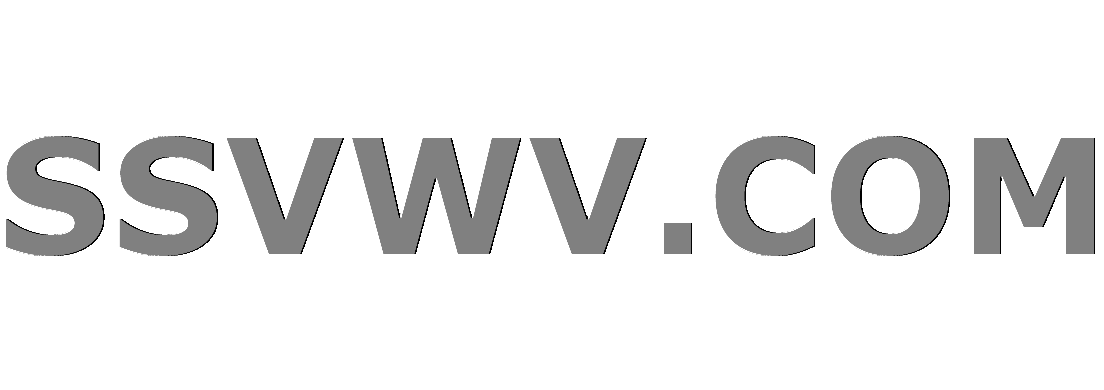
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have the following code:
static async Task Callee()
{
await Task.Delay(1000);
}
static async Task Caller()
{
Callee(); // #1 fire and forget
await Callee(); // #2 >1s
Task.Run(() => Callee()); // #3 fire and forget
await Task.Run(() => Callee()); // #4 >1s
Task.Run(async () => await Callee()); // #5 fire and forget
await Task.Run(async () => await Callee()); // #6 >1s
}
static void Main(string args)
{
var stopWatch = new Stopwatch();
stopWatch.Start();
Caller().Wait();
stopWatch.Stop();
Console.WriteLine($"Elapsed: {stopWatch.ElapsedMilliseconds}");
Console.ReadKey();
}
#1 fires and forgets in the most simple way. #2 simply waits. Interesting stuff begins from #3 on. What's the in-depth logic behind the calls?
I'm aware of using fire'n'forget caveats in ASP.NET as pointed here. I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync());
and the advice is to simply replace it with Task.Run
.
I see that Task.Run runs a new thread, what would be the difference between #3 and #5 then?
c# asynchronous async-await
|
show 10 more comments
I have the following code:
static async Task Callee()
{
await Task.Delay(1000);
}
static async Task Caller()
{
Callee(); // #1 fire and forget
await Callee(); // #2 >1s
Task.Run(() => Callee()); // #3 fire and forget
await Task.Run(() => Callee()); // #4 >1s
Task.Run(async () => await Callee()); // #5 fire and forget
await Task.Run(async () => await Callee()); // #6 >1s
}
static void Main(string args)
{
var stopWatch = new Stopwatch();
stopWatch.Start();
Caller().Wait();
stopWatch.Stop();
Console.WriteLine($"Elapsed: {stopWatch.ElapsedMilliseconds}");
Console.ReadKey();
}
#1 fires and forgets in the most simple way. #2 simply waits. Interesting stuff begins from #3 on. What's the in-depth logic behind the calls?
I'm aware of using fire'n'forget caveats in ASP.NET as pointed here. I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync());
and the advice is to simply replace it with Task.Run
.
I see that Task.Run runs a new thread, what would be the difference between #3 and #5 then?
c# asynchronous async-await
3
Please define "interesting stuff."Task.Run
uses a separate thread.
– GSerg
Sep 5 '17 at 10:54
4
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
2
Tl;Dr don't useasync
/await
if you want fire and forget.
– Liam
Sep 5 '17 at 10:56
2
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (Task
) created new threads to do processing separate to the main thread.
– Liam
Sep 5 '17 at 10:58
2
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09
|
show 10 more comments
I have the following code:
static async Task Callee()
{
await Task.Delay(1000);
}
static async Task Caller()
{
Callee(); // #1 fire and forget
await Callee(); // #2 >1s
Task.Run(() => Callee()); // #3 fire and forget
await Task.Run(() => Callee()); // #4 >1s
Task.Run(async () => await Callee()); // #5 fire and forget
await Task.Run(async () => await Callee()); // #6 >1s
}
static void Main(string args)
{
var stopWatch = new Stopwatch();
stopWatch.Start();
Caller().Wait();
stopWatch.Stop();
Console.WriteLine($"Elapsed: {stopWatch.ElapsedMilliseconds}");
Console.ReadKey();
}
#1 fires and forgets in the most simple way. #2 simply waits. Interesting stuff begins from #3 on. What's the in-depth logic behind the calls?
I'm aware of using fire'n'forget caveats in ASP.NET as pointed here. I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync());
and the advice is to simply replace it with Task.Run
.
I see that Task.Run runs a new thread, what would be the difference between #3 and #5 then?
c# asynchronous async-await
I have the following code:
static async Task Callee()
{
await Task.Delay(1000);
}
static async Task Caller()
{
Callee(); // #1 fire and forget
await Callee(); // #2 >1s
Task.Run(() => Callee()); // #3 fire and forget
await Task.Run(() => Callee()); // #4 >1s
Task.Run(async () => await Callee()); // #5 fire and forget
await Task.Run(async () => await Callee()); // #6 >1s
}
static void Main(string args)
{
var stopWatch = new Stopwatch();
stopWatch.Start();
Caller().Wait();
stopWatch.Stop();
Console.WriteLine($"Elapsed: {stopWatch.ElapsedMilliseconds}");
Console.ReadKey();
}
#1 fires and forgets in the most simple way. #2 simply waits. Interesting stuff begins from #3 on. What's the in-depth logic behind the calls?
I'm aware of using fire'n'forget caveats in ASP.NET as pointed here. I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync());
and the advice is to simply replace it with Task.Run
.
I see that Task.Run runs a new thread, what would be the difference between #3 and #5 then?
c# asynchronous async-await
c# asynchronous async-await
edited Sep 5 '17 at 11:05
mcs_dodo
asked Sep 5 '17 at 10:51
mcs_dodomcs_dodo
454713
454713
3
Please define "interesting stuff."Task.Run
uses a separate thread.
– GSerg
Sep 5 '17 at 10:54
4
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
2
Tl;Dr don't useasync
/await
if you want fire and forget.
– Liam
Sep 5 '17 at 10:56
2
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (Task
) created new threads to do processing separate to the main thread.
– Liam
Sep 5 '17 at 10:58
2
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09
|
show 10 more comments
3
Please define "interesting stuff."Task.Run
uses a separate thread.
– GSerg
Sep 5 '17 at 10:54
4
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
2
Tl;Dr don't useasync
/await
if you want fire and forget.
– Liam
Sep 5 '17 at 10:56
2
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (Task
) created new threads to do processing separate to the main thread.
– Liam
Sep 5 '17 at 10:58
2
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09
3
3
Please define "interesting stuff."
Task.Run
uses a separate thread.– GSerg
Sep 5 '17 at 10:54
Please define "interesting stuff."
Task.Run
uses a separate thread.– GSerg
Sep 5 '17 at 10:54
4
4
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
2
2
Tl;Dr don't use
async
/await
if you want fire and forget.– Liam
Sep 5 '17 at 10:56
Tl;Dr don't use
async
/await
if you want fire and forget.– Liam
Sep 5 '17 at 10:56
2
2
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (
Task
) created new threads to do processing separate to the main thread.– Liam
Sep 5 '17 at 10:58
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (
Task
) created new threads to do processing separate to the main thread.– Liam
Sep 5 '17 at 10:58
2
2
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09
|
show 10 more comments
2 Answers
2
active
oldest
votes
I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync()); and the advice is to simply replace it with Task.Run.
That's bad advice. You should use a separate background process separated from your web frontend by a queue.
What's the in-depth logic behind the calls?
- Starts the asynchronous method on the current thread. Ignores all results (including exceptions).
- Starts the asynchronous method on the current thread. Asynchronously waits for it to complete. This is the standard way of calling asynchronous code.
- Starts the asynchronous method on a thread pool thread. Ignores all results (including exceptions).
- Starts the asynchronous method on a thread pool thread. Asynchronously waits for it to complete.
- Exactly the same as #3.
- Exactly the same as #4.
add a comment |
"26. "Fire and Forget" is fine, provided you never actually forget." Maxim 26.
If you do any kind of fire and forget scenario, you have a massive risk of swallowing Exceptions. Swallowing any exception - but especially fatal ones - is a deadly sin of exception handling. All you end up is with a programm in memory that will produce even less understandable and reproduceable Exceptions. So do not ever start. Here is are two nice articles to read on the mater:
http://blogs.msdn.com/b/ericlippert/archive/2008/09/10/vexing-exceptions.aspx
http://www.codeproject.com/Articles/9538/Exception-Handling-Best-Practices-in-NET
Full on Threads are notoriously capable of swallowing exceptions. Indeed you have to do work to not swallow them and then check if they had one after they finished. You should have at least some followup task that does logging (and possible exposure) of exceptions. Or you will really regret that "fire and forget".
Hope that helps.
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46053175%2fc-sharp-async-await-with-without-awaiting-fire-and-forget%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync()); and the advice is to simply replace it with Task.Run.
That's bad advice. You should use a separate background process separated from your web frontend by a queue.
What's the in-depth logic behind the calls?
- Starts the asynchronous method on the current thread. Ignores all results (including exceptions).
- Starts the asynchronous method on the current thread. Asynchronously waits for it to complete. This is the standard way of calling asynchronous code.
- Starts the asynchronous method on a thread pool thread. Ignores all results (including exceptions).
- Starts the asynchronous method on a thread pool thread. Asynchronously waits for it to complete.
- Exactly the same as #3.
- Exactly the same as #4.
add a comment |
I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync()); and the advice is to simply replace it with Task.Run.
That's bad advice. You should use a separate background process separated from your web frontend by a queue.
What's the in-depth logic behind the calls?
- Starts the asynchronous method on the current thread. Ignores all results (including exceptions).
- Starts the asynchronous method on the current thread. Asynchronously waits for it to complete. This is the standard way of calling asynchronous code.
- Starts the asynchronous method on a thread pool thread. Ignores all results (including exceptions).
- Starts the asynchronous method on a thread pool thread. Asynchronously waits for it to complete.
- Exactly the same as #3.
- Exactly the same as #4.
add a comment |
I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync()); and the advice is to simply replace it with Task.Run.
That's bad advice. You should use a separate background process separated from your web frontend by a queue.
What's the in-depth logic behind the calls?
- Starts the asynchronous method on the current thread. Ignores all results (including exceptions).
- Starts the asynchronous method on the current thread. Asynchronously waits for it to complete. This is the standard way of calling asynchronous code.
- Starts the asynchronous method on a thread pool thread. Ignores all results (including exceptions).
- Starts the asynchronous method on a thread pool thread. Asynchronously waits for it to complete.
- Exactly the same as #3.
- Exactly the same as #4.
I'm asking this, because we're moving our app to service fabric where we no longer can use HostingEnvironment.QueueBackgroundWorkItem(async cancellationToken => await LongMethodAsync()); and the advice is to simply replace it with Task.Run.
That's bad advice. You should use a separate background process separated from your web frontend by a queue.
What's the in-depth logic behind the calls?
- Starts the asynchronous method on the current thread. Ignores all results (including exceptions).
- Starts the asynchronous method on the current thread. Asynchronously waits for it to complete. This is the standard way of calling asynchronous code.
- Starts the asynchronous method on a thread pool thread. Ignores all results (including exceptions).
- Starts the asynchronous method on a thread pool thread. Asynchronously waits for it to complete.
- Exactly the same as #3.
- Exactly the same as #4.
answered Sep 15 '17 at 18:48
Stephen ClearyStephen Cleary
289k49484607
289k49484607
add a comment |
add a comment |
"26. "Fire and Forget" is fine, provided you never actually forget." Maxim 26.
If you do any kind of fire and forget scenario, you have a massive risk of swallowing Exceptions. Swallowing any exception - but especially fatal ones - is a deadly sin of exception handling. All you end up is with a programm in memory that will produce even less understandable and reproduceable Exceptions. So do not ever start. Here is are two nice articles to read on the mater:
http://blogs.msdn.com/b/ericlippert/archive/2008/09/10/vexing-exceptions.aspx
http://www.codeproject.com/Articles/9538/Exception-Handling-Best-Practices-in-NET
Full on Threads are notoriously capable of swallowing exceptions. Indeed you have to do work to not swallow them and then check if they had one after they finished. You should have at least some followup task that does logging (and possible exposure) of exceptions. Or you will really regret that "fire and forget".
Hope that helps.
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
add a comment |
"26. "Fire and Forget" is fine, provided you never actually forget." Maxim 26.
If you do any kind of fire and forget scenario, you have a massive risk of swallowing Exceptions. Swallowing any exception - but especially fatal ones - is a deadly sin of exception handling. All you end up is with a programm in memory that will produce even less understandable and reproduceable Exceptions. So do not ever start. Here is are two nice articles to read on the mater:
http://blogs.msdn.com/b/ericlippert/archive/2008/09/10/vexing-exceptions.aspx
http://www.codeproject.com/Articles/9538/Exception-Handling-Best-Practices-in-NET
Full on Threads are notoriously capable of swallowing exceptions. Indeed you have to do work to not swallow them and then check if they had one after they finished. You should have at least some followup task that does logging (and possible exposure) of exceptions. Or you will really regret that "fire and forget".
Hope that helps.
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
add a comment |
"26. "Fire and Forget" is fine, provided you never actually forget." Maxim 26.
If you do any kind of fire and forget scenario, you have a massive risk of swallowing Exceptions. Swallowing any exception - but especially fatal ones - is a deadly sin of exception handling. All you end up is with a programm in memory that will produce even less understandable and reproduceable Exceptions. So do not ever start. Here is are two nice articles to read on the mater:
http://blogs.msdn.com/b/ericlippert/archive/2008/09/10/vexing-exceptions.aspx
http://www.codeproject.com/Articles/9538/Exception-Handling-Best-Practices-in-NET
Full on Threads are notoriously capable of swallowing exceptions. Indeed you have to do work to not swallow them and then check if they had one after they finished. You should have at least some followup task that does logging (and possible exposure) of exceptions. Or you will really regret that "fire and forget".
Hope that helps.
"26. "Fire and Forget" is fine, provided you never actually forget." Maxim 26.
If you do any kind of fire and forget scenario, you have a massive risk of swallowing Exceptions. Swallowing any exception - but especially fatal ones - is a deadly sin of exception handling. All you end up is with a programm in memory that will produce even less understandable and reproduceable Exceptions. So do not ever start. Here is are two nice articles to read on the mater:
http://blogs.msdn.com/b/ericlippert/archive/2008/09/10/vexing-exceptions.aspx
http://www.codeproject.com/Articles/9538/Exception-Handling-Best-Practices-in-NET
Full on Threads are notoriously capable of swallowing exceptions. Indeed you have to do work to not swallow them and then check if they had one after they finished. You should have at least some followup task that does logging (and possible exposure) of exceptions. Or you will really regret that "fire and forget".
Hope that helps.
answered Sep 5 '17 at 11:13
ChristopherChristopher
2,9842824
2,9842824
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
add a comment |
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
That’s an awesome quote.
– poke
Sep 5 '17 at 11:16
2
2
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
For ASP add : fire-and-forget-on-asp-net
– Fildor
Sep 7 '17 at 7:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f46053175%2fc-sharp-async-await-with-without-awaiting-fire-and-forget%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
asI ejQ,t0x7cWli,eFuBlXPLaehA8t,4jp3saH8 o38s31,0UZ QZ
3
Please define "interesting stuff."
Task.Run
uses a separate thread.– GSerg
Sep 5 '17 at 10:54
4
Possible duplicate of Fire-and-forget with async vs "old async delegate"
– Liam
Sep 5 '17 at 10:55
2
Tl;Dr don't use
async
/await
if you want fire and forget.– Liam
Sep 5 '17 at 10:56
2
No it doesn't @Christopher it's asynchronous programming as opposed to multi threaded programming. Aync reuses threads that are idle, multi threaded (
Task
) created new threads to do processing separate to the main thread.– Liam
Sep 5 '17 at 10:58
2
In words async and await is the difference, you run new async method in separate thread that awaits for something, it doesnt freze your main thread as it is async itself
– Markiian Benovskyi
Sep 5 '17 at 11:09