Node.js EventEmitter handler memory leak
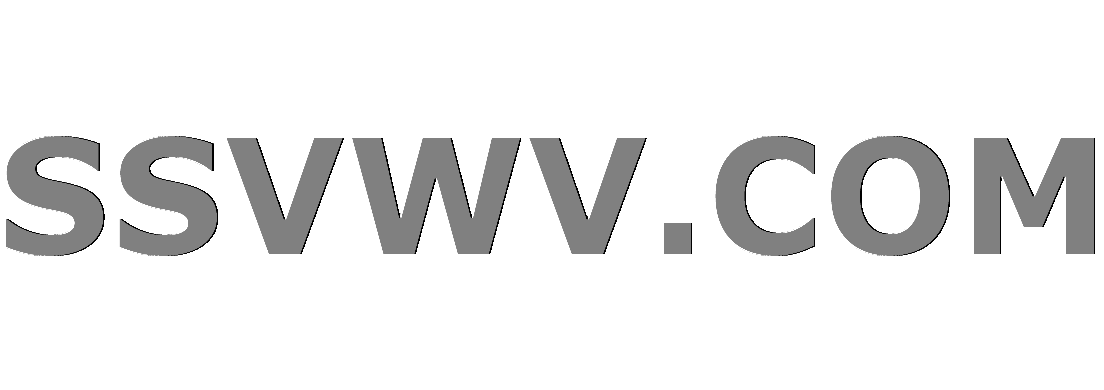
Multi tool use
Is this a memory leak?
class Leaky {
constructor(options) {
process.on('message', function(msg) {
this.do_something(msg)
// ...
}.bind(this))
}
// ...
}
When I call new Leaky()
a reference is bound to the instance through the handler. process
is not going to be GC'd, so did I just leak the Leaky
instance?
How can I prevent this? The only solution I can think of is having a dispose
method that calls process.off
, but I'd like to avoid that. I don't see something like unref
or weak handlers on EventEmitter
.
javascript node.js memory-leaks
|
show 1 more comment
Is this a memory leak?
class Leaky {
constructor(options) {
process.on('message', function(msg) {
this.do_something(msg)
// ...
}.bind(this))
}
// ...
}
When I call new Leaky()
a reference is bound to the instance through the handler. process
is not going to be GC'd, so did I just leak the Leaky
instance?
How can I prevent this? The only solution I can think of is having a dispose
method that calls process.off
, but I'd like to avoid that. I don't see something like unref
or weak handlers on EventEmitter
.
javascript node.js memory-leaks
Do you need to add a new event handler for each instance ofLeaky
? Could you store a list ofLeaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.
– Mike Cluck
Nov 15 '18 at 17:10
1
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Why are you using both an arrow function and.bind(this)
Pick one or the other.
– jfriend00
Nov 15 '18 at 17:28
1
@MikeCluck In the actual code it's notprocess
but another long livedEventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.
– Fozi
Nov 15 '18 at 18:14
@Fozi What exactly is the event emitter if it is notprocess
? What are theLeaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if theLeaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?
– Bergi
Nov 15 '18 at 21:01
|
show 1 more comment
Is this a memory leak?
class Leaky {
constructor(options) {
process.on('message', function(msg) {
this.do_something(msg)
// ...
}.bind(this))
}
// ...
}
When I call new Leaky()
a reference is bound to the instance through the handler. process
is not going to be GC'd, so did I just leak the Leaky
instance?
How can I prevent this? The only solution I can think of is having a dispose
method that calls process.off
, but I'd like to avoid that. I don't see something like unref
or weak handlers on EventEmitter
.
javascript node.js memory-leaks
Is this a memory leak?
class Leaky {
constructor(options) {
process.on('message', function(msg) {
this.do_something(msg)
// ...
}.bind(this))
}
// ...
}
When I call new Leaky()
a reference is bound to the instance through the handler. process
is not going to be GC'd, so did I just leak the Leaky
instance?
How can I prevent this? The only solution I can think of is having a dispose
method that calls process.off
, but I'd like to avoid that. I don't see something like unref
or weak handlers on EventEmitter
.
javascript node.js memory-leaks
javascript node.js memory-leaks
edited Nov 15 '18 at 18:11
Fozi
asked Nov 15 '18 at 17:03
FoziFozi
3,8522450
3,8522450
Do you need to add a new event handler for each instance ofLeaky
? Could you store a list ofLeaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.
– Mike Cluck
Nov 15 '18 at 17:10
1
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Why are you using both an arrow function and.bind(this)
Pick one or the other.
– jfriend00
Nov 15 '18 at 17:28
1
@MikeCluck In the actual code it's notprocess
but another long livedEventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.
– Fozi
Nov 15 '18 at 18:14
@Fozi What exactly is the event emitter if it is notprocess
? What are theLeaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if theLeaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?
– Bergi
Nov 15 '18 at 21:01
|
show 1 more comment
Do you need to add a new event handler for each instance ofLeaky
? Could you store a list ofLeaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.
– Mike Cluck
Nov 15 '18 at 17:10
1
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Why are you using both an arrow function and.bind(this)
Pick one or the other.
– jfriend00
Nov 15 '18 at 17:28
1
@MikeCluck In the actual code it's notprocess
but another long livedEventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.
– Fozi
Nov 15 '18 at 18:14
@Fozi What exactly is the event emitter if it is notprocess
? What are theLeaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if theLeaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?
– Bergi
Nov 15 '18 at 21:01
Do you need to add a new event handler for each instance of
Leaky
? Could you store a list of Leaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.– Mike Cluck
Nov 15 '18 at 17:10
Do you need to add a new event handler for each instance of
Leaky
? Could you store a list of Leaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.– Mike Cluck
Nov 15 '18 at 17:10
1
1
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Why are you using both an arrow function and
.bind(this)
Pick one or the other.– jfriend00
Nov 15 '18 at 17:28
Why are you using both an arrow function and
.bind(this)
Pick one or the other.– jfriend00
Nov 15 '18 at 17:28
1
1
@MikeCluck In the actual code it's not
process
but another long lived EventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.– Fozi
Nov 15 '18 at 18:14
@MikeCluck In the actual code it's not
process
but another long lived EventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.– Fozi
Nov 15 '18 at 18:14
@Fozi What exactly is the event emitter if it is not
process
? What are the Leaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if the Leaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?– Bergi
Nov 15 '18 at 21:01
@Fozi What exactly is the event emitter if it is not
process
? What are the Leaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if the Leaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?– Bergi
Nov 15 '18 at 21:01
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324522%2fnode-js-eventemitter-handler-memory-leak%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324522%2fnode-js-eventemitter-handler-memory-leak%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IQc4r,sqK8iTtSsGS4Q1aFBiwurxJKQC0xeWrjS54IgMPwHfX2NXH5i4ZBn q,ZMOhPLk,l l8EanRh,s
Do you need to add a new event handler for each instance of
Leaky
? Could you store a list ofLeaky
s and have one handler that operates on that list? It would make it easier to remove the event handler when you don't have any more instances to work against.– Mike Cluck
Nov 15 '18 at 17:10
1
Yes, this would prevent your instances from getting garbage collected. Does every instance of Leaky listen for the exact same message? If so, I can think of a different way to implement this that doesn't have this issue.
– jfriend00
Nov 15 '18 at 17:27
Why are you using both an arrow function and
.bind(this)
Pick one or the other.– jfriend00
Nov 15 '18 at 17:28
1
@MikeCluck In the actual code it's not
process
but another long livedEventEmitter
that is passed in the options. I thought about the indirection (only register one handler and execute from a list of handlers) but it does not solve the memory leak problem: in the end there has to be a list of handlers that reference every instance. I looked at WeakMap and WeakSet but they are not enumerable.– Fozi
Nov 15 '18 at 18:14
@Fozi What exactly is the event emitter if it is not
process
? What are theLeaky
entities, and how many are there (do they accumulate over time or is the number limited)? Also if theLeaky
ones are supposed to be shorter-lived than the event emitter, when exactly does their live end (i.e. when are they no longer needed)?– Bergi
Nov 15 '18 at 21:01