CRC16 with hex long array Data type java
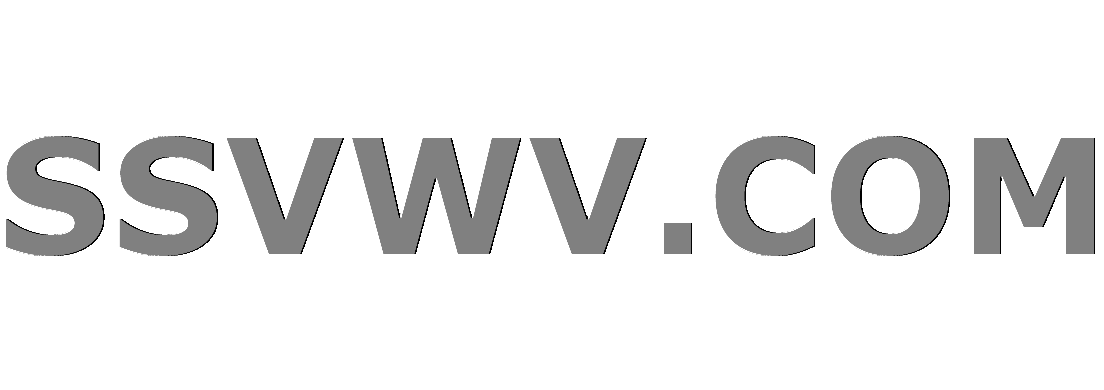
Multi tool use
I am trying to get right CRC16 with using the following code,
public static int GenCrc16(final byte buffer) {
int crc = 0xffff;
for (int j = 0; j < buffer.length ; j++) {
crc = ((crc >>> 8) | (crc << 8) )& 0xffff;
crc ^= (buffer[j] & 0xff);//byte to int, trunc sign
crc ^= ((crc & 0xff) >> 4);
crc ^= (crc << 12) & 0xffff;
crc ^= ((crc & 0xFF) << 5) & 0xffff;
}
crc &= 0xffff;
return crc;
}
if I pass a string,
String input = "00000140 0000000 000000002 0000001";
I get 0x17B5 which is correct but I want pass data as
as raw data
long inputLongArray= {0x00000140, 0x00000000, 0x00000002, 0x00000001}
public static byte toByte(long longArray) {
ByteBuffer bb = ByteBuffer.allocate(longArray.length * Long.BYTES);
bb.asLongBuffer().put(longArray);
return bb.array();
}
I am expecting 0x1F19 as per https://crccalc.com with following
00000140 00000000 00000002 00000001 and choose hex data type and CRC-16/CCITT-FALSE.
public static void main(String args) {
long intputarray = {0x00000140, 0x0000000, 0x000000002, 0x0000001};
System.out.println(Integer.toHexString(GenCrc16(toByte(intputarray)));
}
What I am doing wrong. Thanks in advance for help.
java crc16
add a comment |
I am trying to get right CRC16 with using the following code,
public static int GenCrc16(final byte buffer) {
int crc = 0xffff;
for (int j = 0; j < buffer.length ; j++) {
crc = ((crc >>> 8) | (crc << 8) )& 0xffff;
crc ^= (buffer[j] & 0xff);//byte to int, trunc sign
crc ^= ((crc & 0xff) >> 4);
crc ^= (crc << 12) & 0xffff;
crc ^= ((crc & 0xFF) << 5) & 0xffff;
}
crc &= 0xffff;
return crc;
}
if I pass a string,
String input = "00000140 0000000 000000002 0000001";
I get 0x17B5 which is correct but I want pass data as
as raw data
long inputLongArray= {0x00000140, 0x00000000, 0x00000002, 0x00000001}
public static byte toByte(long longArray) {
ByteBuffer bb = ByteBuffer.allocate(longArray.length * Long.BYTES);
bb.asLongBuffer().put(longArray);
return bb.array();
}
I am expecting 0x1F19 as per https://crccalc.com with following
00000140 00000000 00000002 00000001 and choose hex data type and CRC-16/CCITT-FALSE.
public static void main(String args) {
long intputarray = {0x00000140, 0x0000000, 0x000000002, 0x0000001};
System.out.println(Integer.toHexString(GenCrc16(toByte(intputarray)));
}
What I am doing wrong. Thanks in advance for help.
java crc16
add a comment |
I am trying to get right CRC16 with using the following code,
public static int GenCrc16(final byte buffer) {
int crc = 0xffff;
for (int j = 0; j < buffer.length ; j++) {
crc = ((crc >>> 8) | (crc << 8) )& 0xffff;
crc ^= (buffer[j] & 0xff);//byte to int, trunc sign
crc ^= ((crc & 0xff) >> 4);
crc ^= (crc << 12) & 0xffff;
crc ^= ((crc & 0xFF) << 5) & 0xffff;
}
crc &= 0xffff;
return crc;
}
if I pass a string,
String input = "00000140 0000000 000000002 0000001";
I get 0x17B5 which is correct but I want pass data as
as raw data
long inputLongArray= {0x00000140, 0x00000000, 0x00000002, 0x00000001}
public static byte toByte(long longArray) {
ByteBuffer bb = ByteBuffer.allocate(longArray.length * Long.BYTES);
bb.asLongBuffer().put(longArray);
return bb.array();
}
I am expecting 0x1F19 as per https://crccalc.com with following
00000140 00000000 00000002 00000001 and choose hex data type and CRC-16/CCITT-FALSE.
public static void main(String args) {
long intputarray = {0x00000140, 0x0000000, 0x000000002, 0x0000001};
System.out.println(Integer.toHexString(GenCrc16(toByte(intputarray)));
}
What I am doing wrong. Thanks in advance for help.
java crc16
I am trying to get right CRC16 with using the following code,
public static int GenCrc16(final byte buffer) {
int crc = 0xffff;
for (int j = 0; j < buffer.length ; j++) {
crc = ((crc >>> 8) | (crc << 8) )& 0xffff;
crc ^= (buffer[j] & 0xff);//byte to int, trunc sign
crc ^= ((crc & 0xff) >> 4);
crc ^= (crc << 12) & 0xffff;
crc ^= ((crc & 0xFF) << 5) & 0xffff;
}
crc &= 0xffff;
return crc;
}
if I pass a string,
String input = "00000140 0000000 000000002 0000001";
I get 0x17B5 which is correct but I want pass data as
as raw data
long inputLongArray= {0x00000140, 0x00000000, 0x00000002, 0x00000001}
public static byte toByte(long longArray) {
ByteBuffer bb = ByteBuffer.allocate(longArray.length * Long.BYTES);
bb.asLongBuffer().put(longArray);
return bb.array();
}
I am expecting 0x1F19 as per https://crccalc.com with following
00000140 00000000 00000002 00000001 and choose hex data type and CRC-16/CCITT-FALSE.
public static void main(String args) {
long intputarray = {0x00000140, 0x0000000, 0x000000002, 0x0000001};
System.out.println(Integer.toHexString(GenCrc16(toByte(intputarray)));
}
What I am doing wrong. Thanks in advance for help.
java crc16
java crc16
asked Nov 15 '18 at 21:33
Muhammad Rizwan nycghumanMuhammad Rizwan nycghuman
66
66
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
long
values are 8 bytes, aka 16 hex digits, solong {0x00000140, 0x00000000, 0x00000002, 0x00000001}
is same as"0000000000000140 0000000000000000 0000000000000002 0000000000000001"
and both have CRC-16/CCITT-FALSE value
E47B
The string"00000140 0000000 000000002 0000001"
should really be"00000140 00000000 00000002 00000001"
which is the same asint {0x00000140, 0x00000000, 0x00000002, 0x00000001}
and both have CRC-16/CCITT-FALSE value
1F19
public static byte toByte(final int... intArray) {
ByteBuffer bb = ByteBuffer.allocate(intArray.length * Integer.BYTES);
bb.asIntBuffer().put(intArray);
return bb.array();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328209%2fcrc16-with-hex-long-array-data-type-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
long
values are 8 bytes, aka 16 hex digits, solong {0x00000140, 0x00000000, 0x00000002, 0x00000001}
is same as"0000000000000140 0000000000000000 0000000000000002 0000000000000001"
and both have CRC-16/CCITT-FALSE value
E47B
The string"00000140 0000000 000000002 0000001"
should really be"00000140 00000000 00000002 00000001"
which is the same asint {0x00000140, 0x00000000, 0x00000002, 0x00000001}
and both have CRC-16/CCITT-FALSE value
1F19
public static byte toByte(final int... intArray) {
ByteBuffer bb = ByteBuffer.allocate(intArray.length * Integer.BYTES);
bb.asIntBuffer().put(intArray);
return bb.array();
}
add a comment |
long
values are 8 bytes, aka 16 hex digits, solong {0x00000140, 0x00000000, 0x00000002, 0x00000001}
is same as"0000000000000140 0000000000000000 0000000000000002 0000000000000001"
and both have CRC-16/CCITT-FALSE value
E47B
The string"00000140 0000000 000000002 0000001"
should really be"00000140 00000000 00000002 00000001"
which is the same asint {0x00000140, 0x00000000, 0x00000002, 0x00000001}
and both have CRC-16/CCITT-FALSE value
1F19
public static byte toByte(final int... intArray) {
ByteBuffer bb = ByteBuffer.allocate(intArray.length * Integer.BYTES);
bb.asIntBuffer().put(intArray);
return bb.array();
}
add a comment |
long
values are 8 bytes, aka 16 hex digits, solong {0x00000140, 0x00000000, 0x00000002, 0x00000001}
is same as"0000000000000140 0000000000000000 0000000000000002 0000000000000001"
and both have CRC-16/CCITT-FALSE value
E47B
The string"00000140 0000000 000000002 0000001"
should really be"00000140 00000000 00000002 00000001"
which is the same asint {0x00000140, 0x00000000, 0x00000002, 0x00000001}
and both have CRC-16/CCITT-FALSE value
1F19
public static byte toByte(final int... intArray) {
ByteBuffer bb = ByteBuffer.allocate(intArray.length * Integer.BYTES);
bb.asIntBuffer().put(intArray);
return bb.array();
}
long
values are 8 bytes, aka 16 hex digits, solong {0x00000140, 0x00000000, 0x00000002, 0x00000001}
is same as"0000000000000140 0000000000000000 0000000000000002 0000000000000001"
and both have CRC-16/CCITT-FALSE value
E47B
The string"00000140 0000000 000000002 0000001"
should really be"00000140 00000000 00000002 00000001"
which is the same asint {0x00000140, 0x00000000, 0x00000002, 0x00000001}
and both have CRC-16/CCITT-FALSE value
1F19
public static byte toByte(final int... intArray) {
ByteBuffer bb = ByteBuffer.allocate(intArray.length * Integer.BYTES);
bb.asIntBuffer().put(intArray);
return bb.array();
}
edited Nov 16 '18 at 0:37
answered Nov 16 '18 at 0:28


AndreasAndreas
78.8k464129
78.8k464129
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53328209%2fcrc16-with-hex-long-array-data-type-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
r,xoX o3YWRI Ca57LFYQfuu6y50slvYdu