or-tools : Python employee scheduling does not give any solutions if `num_nurses` is not equal to...
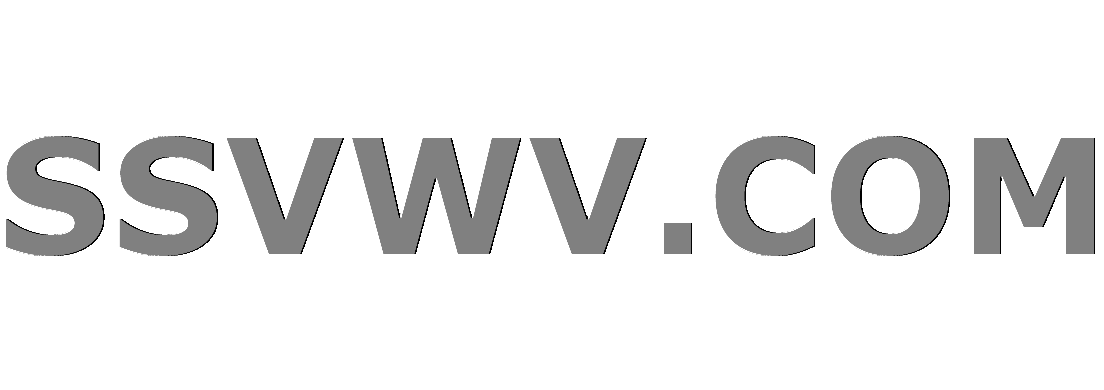
Multi tool use
I am trying to use get familiar with google or-tools. I tried a simplified version of the Employee scheduling python example.
from __future__ import print_function
import sys
from ortools.constraint_solver import pywrapcp
def main():
# Creates the solver.
solver = pywrapcp.Solver("employee_scheduling")
num_nurses = 3
num_shifts = 3
num_days = 1
# [START]
# Create shift variables.
shifts = {}
for j in range(num_nurses):
for i in range(num_days):
shifts[(j, i)] = solver.IntVar(
0, num_shifts - 1, "shifts(%i,%i)" % (j, i))
shifts_flat = [shifts[(j, i)] for j in range(num_nurses)
for i in range(num_days)]
# Create nurse variables.
nurses = {}
for j in range(num_shifts):
for i in range(num_days):
nurses[(j, i)] = solver.IntVar(
0, num_nurses - 1, "shift%d day%d" % (j, i))
# Set relationships between shifts and nurses.
for day in range(num_days):
nurses_for_day = [nurses[(j, day)] for j in range(num_shifts)]
for j in range(num_nurses):
s = shifts[(j, day)]
solver.Add(s.IndexOf(nurses_for_day) == j)
# Create the decision builder.
db = solver.Phase(shifts_flat, solver.CHOOSE_FIRST_UNBOUND,
solver.ASSIGN_MIN_VALUE)
# Create the solution collector.
solution = solver.Assignment()
solution.Add(shifts_flat)
collector = solver.AllSolutionCollector(solution)
solver.Solve(db, [collector])
print("Solutions found:", collector.SolutionCount())
print("Time:", solver.WallTime(), "ms")
print()
if __name__ == "__main__":
main()
As you can see the only constraints I have kept is the relationships between shifts and nurses.
With num_nurses = 3, num_shifts = 3 and num_days = 1, the solver is able to find 6 solutions. However, if I change num_shifts to 2, the solver returns 0 solutions.
Shouldn't this have 3 solutions as well (assign one nurse, leave the other two unassigned) ?
or-tools
add a comment |
I am trying to use get familiar with google or-tools. I tried a simplified version of the Employee scheduling python example.
from __future__ import print_function
import sys
from ortools.constraint_solver import pywrapcp
def main():
# Creates the solver.
solver = pywrapcp.Solver("employee_scheduling")
num_nurses = 3
num_shifts = 3
num_days = 1
# [START]
# Create shift variables.
shifts = {}
for j in range(num_nurses):
for i in range(num_days):
shifts[(j, i)] = solver.IntVar(
0, num_shifts - 1, "shifts(%i,%i)" % (j, i))
shifts_flat = [shifts[(j, i)] for j in range(num_nurses)
for i in range(num_days)]
# Create nurse variables.
nurses = {}
for j in range(num_shifts):
for i in range(num_days):
nurses[(j, i)] = solver.IntVar(
0, num_nurses - 1, "shift%d day%d" % (j, i))
# Set relationships between shifts and nurses.
for day in range(num_days):
nurses_for_day = [nurses[(j, day)] for j in range(num_shifts)]
for j in range(num_nurses):
s = shifts[(j, day)]
solver.Add(s.IndexOf(nurses_for_day) == j)
# Create the decision builder.
db = solver.Phase(shifts_flat, solver.CHOOSE_FIRST_UNBOUND,
solver.ASSIGN_MIN_VALUE)
# Create the solution collector.
solution = solver.Assignment()
solution.Add(shifts_flat)
collector = solver.AllSolutionCollector(solution)
solver.Solve(db, [collector])
print("Solutions found:", collector.SolutionCount())
print("Time:", solver.WallTime(), "ms")
print()
if __name__ == "__main__":
main()
As you can see the only constraints I have kept is the relationships between shifts and nurses.
With num_nurses = 3, num_shifts = 3 and num_days = 1, the solver is able to find 6 solutions. However, if I change num_shifts to 2, the solver returns 0 solutions.
Shouldn't this have 3 solutions as well (assign one nurse, leave the other two unassigned) ?
or-tools
add a comment |
I am trying to use get familiar with google or-tools. I tried a simplified version of the Employee scheduling python example.
from __future__ import print_function
import sys
from ortools.constraint_solver import pywrapcp
def main():
# Creates the solver.
solver = pywrapcp.Solver("employee_scheduling")
num_nurses = 3
num_shifts = 3
num_days = 1
# [START]
# Create shift variables.
shifts = {}
for j in range(num_nurses):
for i in range(num_days):
shifts[(j, i)] = solver.IntVar(
0, num_shifts - 1, "shifts(%i,%i)" % (j, i))
shifts_flat = [shifts[(j, i)] for j in range(num_nurses)
for i in range(num_days)]
# Create nurse variables.
nurses = {}
for j in range(num_shifts):
for i in range(num_days):
nurses[(j, i)] = solver.IntVar(
0, num_nurses - 1, "shift%d day%d" % (j, i))
# Set relationships between shifts and nurses.
for day in range(num_days):
nurses_for_day = [nurses[(j, day)] for j in range(num_shifts)]
for j in range(num_nurses):
s = shifts[(j, day)]
solver.Add(s.IndexOf(nurses_for_day) == j)
# Create the decision builder.
db = solver.Phase(shifts_flat, solver.CHOOSE_FIRST_UNBOUND,
solver.ASSIGN_MIN_VALUE)
# Create the solution collector.
solution = solver.Assignment()
solution.Add(shifts_flat)
collector = solver.AllSolutionCollector(solution)
solver.Solve(db, [collector])
print("Solutions found:", collector.SolutionCount())
print("Time:", solver.WallTime(), "ms")
print()
if __name__ == "__main__":
main()
As you can see the only constraints I have kept is the relationships between shifts and nurses.
With num_nurses = 3, num_shifts = 3 and num_days = 1, the solver is able to find 6 solutions. However, if I change num_shifts to 2, the solver returns 0 solutions.
Shouldn't this have 3 solutions as well (assign one nurse, leave the other two unassigned) ?
or-tools
I am trying to use get familiar with google or-tools. I tried a simplified version of the Employee scheduling python example.
from __future__ import print_function
import sys
from ortools.constraint_solver import pywrapcp
def main():
# Creates the solver.
solver = pywrapcp.Solver("employee_scheduling")
num_nurses = 3
num_shifts = 3
num_days = 1
# [START]
# Create shift variables.
shifts = {}
for j in range(num_nurses):
for i in range(num_days):
shifts[(j, i)] = solver.IntVar(
0, num_shifts - 1, "shifts(%i,%i)" % (j, i))
shifts_flat = [shifts[(j, i)] for j in range(num_nurses)
for i in range(num_days)]
# Create nurse variables.
nurses = {}
for j in range(num_shifts):
for i in range(num_days):
nurses[(j, i)] = solver.IntVar(
0, num_nurses - 1, "shift%d day%d" % (j, i))
# Set relationships between shifts and nurses.
for day in range(num_days):
nurses_for_day = [nurses[(j, day)] for j in range(num_shifts)]
for j in range(num_nurses):
s = shifts[(j, day)]
solver.Add(s.IndexOf(nurses_for_day) == j)
# Create the decision builder.
db = solver.Phase(shifts_flat, solver.CHOOSE_FIRST_UNBOUND,
solver.ASSIGN_MIN_VALUE)
# Create the solution collector.
solution = solver.Assignment()
solution.Add(shifts_flat)
collector = solver.AllSolutionCollector(solution)
solver.Solve(db, [collector])
print("Solutions found:", collector.SolutionCount())
print("Time:", solver.WallTime(), "ms")
print()
if __name__ == "__main__":
main()
As you can see the only constraints I have kept is the relationships between shifts and nurses.
With num_nurses = 3, num_shifts = 3 and num_days = 1, the solver is able to find 6 solutions. However, if I change num_shifts to 2, the solver returns 0 solutions.
Shouldn't this have 3 solutions as well (assign one nurse, leave the other two unassigned) ?
or-tools
or-tools
asked Nov 14 '18 at 14:55


Pravesh JainPravesh Jain
1,36541731
1,36541731
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
As it turns out, this is a limitation with the way employee_scheduling is written right now. A rewrite of it is currently underway and should be done in a couple of weeks.
https://github.com/google/or-tools/issues/932
add a comment |
I recommend this version of shift scheduling that implements a few different constraints:
https://github.com/google/or-tools/blob/master/examples/python/shift_scheduling_sat.py
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303025%2for-tools-python-employee-scheduling-does-not-give-any-solutions-if-num-nurses%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As it turns out, this is a limitation with the way employee_scheduling is written right now. A rewrite of it is currently underway and should be done in a couple of weeks.
https://github.com/google/or-tools/issues/932
add a comment |
As it turns out, this is a limitation with the way employee_scheduling is written right now. A rewrite of it is currently underway and should be done in a couple of weeks.
https://github.com/google/or-tools/issues/932
add a comment |
As it turns out, this is a limitation with the way employee_scheduling is written right now. A rewrite of it is currently underway and should be done in a couple of weeks.
https://github.com/google/or-tools/issues/932
As it turns out, this is a limitation with the way employee_scheduling is written right now. A rewrite of it is currently underway and should be done in a couple of weeks.
https://github.com/google/or-tools/issues/932
answered Nov 14 '18 at 17:40


Pravesh JainPravesh Jain
1,36541731
1,36541731
add a comment |
add a comment |
I recommend this version of shift scheduling that implements a few different constraints:
https://github.com/google/or-tools/blob/master/examples/python/shift_scheduling_sat.py
add a comment |
I recommend this version of shift scheduling that implements a few different constraints:
https://github.com/google/or-tools/blob/master/examples/python/shift_scheduling_sat.py
add a comment |
I recommend this version of shift scheduling that implements a few different constraints:
https://github.com/google/or-tools/blob/master/examples/python/shift_scheduling_sat.py
I recommend this version of shift scheduling that implements a few different constraints:
https://github.com/google/or-tools/blob/master/examples/python/shift_scheduling_sat.py
answered Nov 19 '18 at 18:29


Laurent PerronLaurent Perron
2316
2316
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53303025%2for-tools-python-employee-scheduling-does-not-give-any-solutions-if-num-nurses%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
M,u4hm y2czXwb,DcxM2Rh,yAur4LQ