How to retrieve image from Firebase Storage using Python?
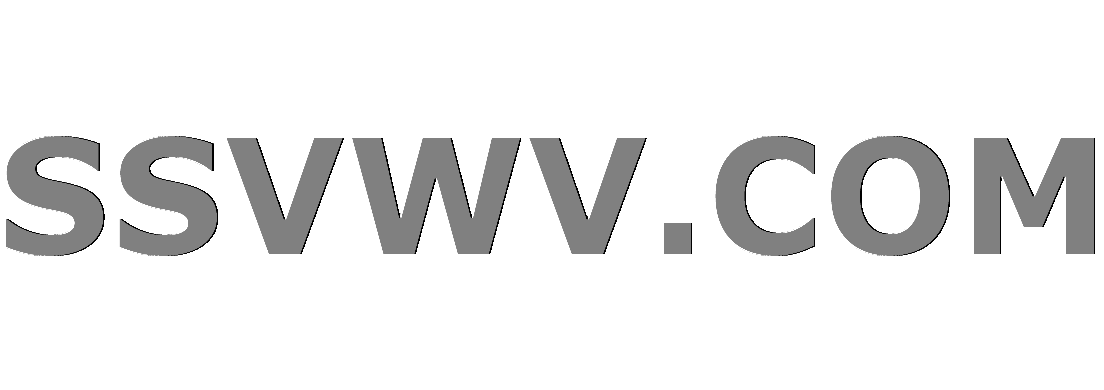
Multi tool use
I have already store my image to Firebase Storage, and I need to take it out by using Python code. Can I retrieve the image by using any URL? Or is there any way to retrieve it out?
Here are the image of how I store it in Firebase Storage:
python firebase firebase-storage
add a comment |
I have already store my image to Firebase Storage, and I need to take it out by using Python code. Can I retrieve the image by using any URL? Or is there any way to retrieve it out?
Here are the image of how I store it in Firebase Storage:
python firebase firebase-storage
add a comment |
I have already store my image to Firebase Storage, and I need to take it out by using Python code. Can I retrieve the image by using any URL? Or is there any way to retrieve it out?
Here are the image of how I store it in Firebase Storage:
python firebase firebase-storage
I have already store my image to Firebase Storage, and I need to take it out by using Python code. Can I retrieve the image by using any URL? Or is there any way to retrieve it out?
Here are the image of how I store it in Firebase Storage:
python firebase firebase-storage
python firebase firebase-storage
edited Nov 14 '18 at 17:25


Grimthorr
4,45442235
4,45442235
asked Nov 14 '18 at 16:15


KaiKai
145
145
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
This is what I use. Hope it helps.
import firebase_admin
from firebase_admin import credentials
from firebase_admin import storage
# Fetch the service account key JSON file contents
cred = credentials.Certificate("credentials.json")
# Initialize the app with a service account, granting admin privileges
app = firebase_admin.initialize_app(cred, {
'storageBucket': '<BUCKET_NAME>.appspot.com',
}, name='storage')
bucket = storage.bucket(app=app)
blob = bucket.blob("<your_blob_path>")
print(blob.generate_signed_url(datetime.timedelta(seconds=300), method='GET'))
It generates a public URL (for 300 secs) for you to download your files.
For example, in my case, I use that URL to display stored pictures in my django website with <img>
tag.
Here is the doc for more usefull functions.
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304517%2fhow-to-retrieve-image-from-firebase-storage-using-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is what I use. Hope it helps.
import firebase_admin
from firebase_admin import credentials
from firebase_admin import storage
# Fetch the service account key JSON file contents
cred = credentials.Certificate("credentials.json")
# Initialize the app with a service account, granting admin privileges
app = firebase_admin.initialize_app(cred, {
'storageBucket': '<BUCKET_NAME>.appspot.com',
}, name='storage')
bucket = storage.bucket(app=app)
blob = bucket.blob("<your_blob_path>")
print(blob.generate_signed_url(datetime.timedelta(seconds=300), method='GET'))
It generates a public URL (for 300 secs) for you to download your files.
For example, in my case, I use that URL to display stored pictures in my django website with <img>
tag.
Here is the doc for more usefull functions.
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
add a comment |
This is what I use. Hope it helps.
import firebase_admin
from firebase_admin import credentials
from firebase_admin import storage
# Fetch the service account key JSON file contents
cred = credentials.Certificate("credentials.json")
# Initialize the app with a service account, granting admin privileges
app = firebase_admin.initialize_app(cred, {
'storageBucket': '<BUCKET_NAME>.appspot.com',
}, name='storage')
bucket = storage.bucket(app=app)
blob = bucket.blob("<your_blob_path>")
print(blob.generate_signed_url(datetime.timedelta(seconds=300), method='GET'))
It generates a public URL (for 300 secs) for you to download your files.
For example, in my case, I use that URL to display stored pictures in my django website with <img>
tag.
Here is the doc for more usefull functions.
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
add a comment |
This is what I use. Hope it helps.
import firebase_admin
from firebase_admin import credentials
from firebase_admin import storage
# Fetch the service account key JSON file contents
cred = credentials.Certificate("credentials.json")
# Initialize the app with a service account, granting admin privileges
app = firebase_admin.initialize_app(cred, {
'storageBucket': '<BUCKET_NAME>.appspot.com',
}, name='storage')
bucket = storage.bucket(app=app)
blob = bucket.blob("<your_blob_path>")
print(blob.generate_signed_url(datetime.timedelta(seconds=300), method='GET'))
It generates a public URL (for 300 secs) for you to download your files.
For example, in my case, I use that URL to display stored pictures in my django website with <img>
tag.
Here is the doc for more usefull functions.
This is what I use. Hope it helps.
import firebase_admin
from firebase_admin import credentials
from firebase_admin import storage
# Fetch the service account key JSON file contents
cred = credentials.Certificate("credentials.json")
# Initialize the app with a service account, granting admin privileges
app = firebase_admin.initialize_app(cred, {
'storageBucket': '<BUCKET_NAME>.appspot.com',
}, name='storage')
bucket = storage.bucket(app=app)
blob = bucket.blob("<your_blob_path>")
print(blob.generate_signed_url(datetime.timedelta(seconds=300), method='GET'))
It generates a public URL (for 300 secs) for you to download your files.
For example, in my case, I use that URL to display stored pictures in my django website with <img>
tag.
Here is the doc for more usefull functions.
edited Nov 15 '18 at 20:07
answered Nov 14 '18 at 17:53


ChecheCheche
816218
816218
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
add a comment |
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
I don't understand that "Fetch the service account key JSON file contents" part, what is that use for, and how to do it?
– Kai
Nov 15 '18 at 15:17
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
In your Firebase console, go to Project Overview (left panel) > Project Configuration. Then go to service accounts and press "Generate new private key". Check this picture.
– Cheche
Nov 15 '18 at 20:06
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Ohh it works. Thank you so much. Lol By the way did you have any idea on downloading that image ?
– Kai
Nov 16 '18 at 3:58
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
Yeah, check the docs link I've added. Or just try here.
– Cheche
Nov 16 '18 at 14:11
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
console.cloud.google.com/… get the credentials from that link
– Sohaib Aslam
Jan 15 at 18:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53304517%2fhow-to-retrieve-image-from-firebase-storage-using-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cTNTjGOO,D,5V5Ac2aP2EFmUYf,Ie Jm6Iph