How do I make an ascending function in C++?
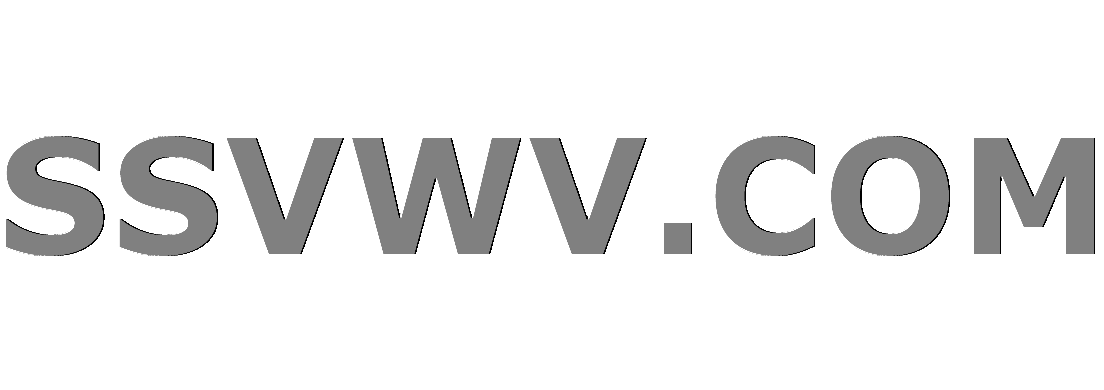
Multi tool use
I need to make a simple function in c++ that will say if an entered integer has its digits ascending from left to right. Ex, 123 is ascending. We just started learning recurssion, which is what I'm supposed to use, but I'm confused. So far what I was thinking is that you store the last digit as a temp, then compare that to the next digit, but how would you manage to do that?
bool ascending(int n) {
int temp = n % 10;
while (n / 10 > 0) {
n = n / 10;
if (temp > n % 10) {
return false;
break;
}
temp = n % 10;
}
}
This is the code I have so far, but I'm definitely messing up. I'm not even using recurrsion.
c++ recursion
add a comment |
I need to make a simple function in c++ that will say if an entered integer has its digits ascending from left to right. Ex, 123 is ascending. We just started learning recurssion, which is what I'm supposed to use, but I'm confused. So far what I was thinking is that you store the last digit as a temp, then compare that to the next digit, but how would you manage to do that?
bool ascending(int n) {
int temp = n % 10;
while (n / 10 > 0) {
n = n / 10;
if (temp > n % 10) {
return false;
break;
}
temp = n % 10;
}
}
This is the code I have so far, but I'm definitely messing up. I'm not even using recurrsion.
c++ recursion
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
1
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05
add a comment |
I need to make a simple function in c++ that will say if an entered integer has its digits ascending from left to right. Ex, 123 is ascending. We just started learning recurssion, which is what I'm supposed to use, but I'm confused. So far what I was thinking is that you store the last digit as a temp, then compare that to the next digit, but how would you manage to do that?
bool ascending(int n) {
int temp = n % 10;
while (n / 10 > 0) {
n = n / 10;
if (temp > n % 10) {
return false;
break;
}
temp = n % 10;
}
}
This is the code I have so far, but I'm definitely messing up. I'm not even using recurrsion.
c++ recursion
I need to make a simple function in c++ that will say if an entered integer has its digits ascending from left to right. Ex, 123 is ascending. We just started learning recurssion, which is what I'm supposed to use, but I'm confused. So far what I was thinking is that you store the last digit as a temp, then compare that to the next digit, but how would you manage to do that?
bool ascending(int n) {
int temp = n % 10;
while (n / 10 > 0) {
n = n / 10;
if (temp > n % 10) {
return false;
break;
}
temp = n % 10;
}
}
This is the code I have so far, but I'm definitely messing up. I'm not even using recurrsion.
c++ recursion
c++ recursion
edited Nov 14 '18 at 5:16


Swordfish
9,44811436
9,44811436
asked Nov 14 '18 at 5:00


Akash ThomasAkash Thomas
32
32
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
1
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05
add a comment |
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
1
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
1
1
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05
add a comment |
1 Answer
1
active
oldest
votes
Here is one way you can go about it.
On every iteration, you check that last 2 digits are in order. And when the number is a single digit, return true
bool ascending(int n) {
int last_digit = n % 10;
int remainder = n / 10;
if (remainder == 0)
{
return true;
}
int second_last_digit = remainder % 10;
if (last_digit < second_last_digit)
{
return false;
}
else
{
return ascending(remainder); // Recusrive call
}
}
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293438%2fhow-do-i-make-an-ascending-function-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Here is one way you can go about it.
On every iteration, you check that last 2 digits are in order. And when the number is a single digit, return true
bool ascending(int n) {
int last_digit = n % 10;
int remainder = n / 10;
if (remainder == 0)
{
return true;
}
int second_last_digit = remainder % 10;
if (last_digit < second_last_digit)
{
return false;
}
else
{
return ascending(remainder); // Recusrive call
}
}
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
add a comment |
Here is one way you can go about it.
On every iteration, you check that last 2 digits are in order. And when the number is a single digit, return true
bool ascending(int n) {
int last_digit = n % 10;
int remainder = n / 10;
if (remainder == 0)
{
return true;
}
int second_last_digit = remainder % 10;
if (last_digit < second_last_digit)
{
return false;
}
else
{
return ascending(remainder); // Recusrive call
}
}
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
add a comment |
Here is one way you can go about it.
On every iteration, you check that last 2 digits are in order. And when the number is a single digit, return true
bool ascending(int n) {
int last_digit = n % 10;
int remainder = n / 10;
if (remainder == 0)
{
return true;
}
int second_last_digit = remainder % 10;
if (last_digit < second_last_digit)
{
return false;
}
else
{
return ascending(remainder); // Recusrive call
}
}
Here is one way you can go about it.
On every iteration, you check that last 2 digits are in order. And when the number is a single digit, return true
bool ascending(int n) {
int last_digit = n % 10;
int remainder = n / 10;
if (remainder == 0)
{
return true;
}
int second_last_digit = remainder % 10;
if (last_digit < second_last_digit)
{
return false;
}
else
{
return ascending(remainder); // Recusrive call
}
}
answered Nov 14 '18 at 5:22
BishalBishal
556216
556216
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
add a comment |
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
shouldn't the second return be true? Because doesn't that say if the last digit is less than the second to last, it's false? But wouldn't that make it descending and not ascending?
– Akash Thomas
Nov 14 '18 at 5:27
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
The example above works from the last digit, so we want the last digit to be greater than the second last digit for the whole number to be in ascending order
– Bishal
Nov 14 '18 at 5:29
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
Oh man, I read that completley wrong, you're right. Thank you!
– Akash Thomas
Nov 14 '18 at 5:33
short version:
return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
short version:
return !value || (value % 10 >= value / 10 % 10 ? is_ascending(value / 10) : false);
– Swordfish
Nov 14 '18 at 5:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293438%2fhow-do-i-make-an-ascending-function-in-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Zd1hxONhReCUfDKsBZhHn ioS,vpXkazG,cra8mZC eDcBM
Please share your code here then we can help you in easy way
– Ved Prakash
Nov 14 '18 at 5:01
1
Recursion is about specifying a problem in terms of a simpler problem. In your case, the simpler problem is probably going to be a number with one less digit. So think about how to specify the problem that way.
– paddy
Nov 14 '18 at 5:05