Python class variable assignment using dictionary comprehension
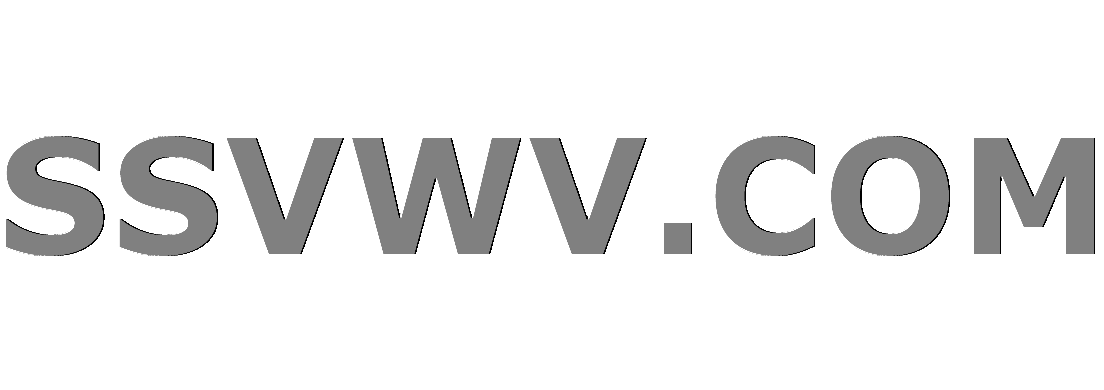
Multi tool use
During a class definition, a class variable defined as a dictionary is used in the construction of a second dictionary class variable, a subset pared down from the first, like this:
class C(object):
ALL_ITEMS = dict(a='A', b='B', c='C', d='D', e='E')
SUBSET_X = {k: v for k, v in ALL_ITEMS.items() if k in ('a', 'b', 'd')} # (this works)
SUBSET_Y = {k: ALL_ITEMS[k] for k in ('a', 'b', 'd')} # (this fails)
Pretty simple stuff, but the net effect of executing this code is quite surprising to me. My first approach was the code on line 4, but I had to resort to the solution on line 3 instead. There is something subtle about dictionary comprehension scoping rules that I'm clearly failing to grasp.
Specifically, the error raised in the failing case is:
File "goofy.py", line 4, in <dictcomp>
SUBSET_Y = {k: ALL_ITEMS.get(k) for k in ('a', 'b', 'd')}
NameError: name 'ALL_ITEMS' is not defined
The nature of this error is baffling to me for a few different reasons:
- The assignment to
SUBSET_Y
is a well-formed dictionary comprehension, and references a symbol which should be in-scope and accessible. - In the succeeding case (the assignment to
SUBSET_X
), which is also a dictionary comprehension, the symbolALL_ITEMS
is perfectly well-defined and accessible. Thus, the fact that the raised exception is aNameError
in the failing case seems manifestly wrong. (Or misleading, at best.) - Why would the scoping rules differ for
items()
vs.__getitem__
orget()
? (The same exception occurs replacingALL_ITEMS[k]
withALL_ITEMS.get(k)
in the failure case.)
(Even as a Python developer for over a decade, I've never run into this failure before, which either means I've been lucky or have lived a sheltered existence :^)
The same failure occurs in various 3.6.x CPython versions as well as 2.7.x versions.
EDIT: No, this is not a duplicate of a previous question. That pertained to list comprehensions, and even if one were to project the same explanation to dictionary comprehensions, it doesn't explain the difference between the two cases I cited. And also, it is not a Python 3-only phenomenon.
python class dictionary scoping
add a comment |
During a class definition, a class variable defined as a dictionary is used in the construction of a second dictionary class variable, a subset pared down from the first, like this:
class C(object):
ALL_ITEMS = dict(a='A', b='B', c='C', d='D', e='E')
SUBSET_X = {k: v for k, v in ALL_ITEMS.items() if k in ('a', 'b', 'd')} # (this works)
SUBSET_Y = {k: ALL_ITEMS[k] for k in ('a', 'b', 'd')} # (this fails)
Pretty simple stuff, but the net effect of executing this code is quite surprising to me. My first approach was the code on line 4, but I had to resort to the solution on line 3 instead. There is something subtle about dictionary comprehension scoping rules that I'm clearly failing to grasp.
Specifically, the error raised in the failing case is:
File "goofy.py", line 4, in <dictcomp>
SUBSET_Y = {k: ALL_ITEMS.get(k) for k in ('a', 'b', 'd')}
NameError: name 'ALL_ITEMS' is not defined
The nature of this error is baffling to me for a few different reasons:
- The assignment to
SUBSET_Y
is a well-formed dictionary comprehension, and references a symbol which should be in-scope and accessible. - In the succeeding case (the assignment to
SUBSET_X
), which is also a dictionary comprehension, the symbolALL_ITEMS
is perfectly well-defined and accessible. Thus, the fact that the raised exception is aNameError
in the failing case seems manifestly wrong. (Or misleading, at best.) - Why would the scoping rules differ for
items()
vs.__getitem__
orget()
? (The same exception occurs replacingALL_ITEMS[k]
withALL_ITEMS.get(k)
in the failure case.)
(Even as a Python developer for over a decade, I've never run into this failure before, which either means I've been lucky or have lived a sheltered existence :^)
The same failure occurs in various 3.6.x CPython versions as well as 2.7.x versions.
EDIT: No, this is not a duplicate of a previous question. That pertained to list comprehensions, and even if one were to project the same explanation to dictionary comprehensions, it doesn't explain the difference between the two cases I cited. And also, it is not a Python 3-only phenomenon.
python class dictionary scoping
3
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57
add a comment |
During a class definition, a class variable defined as a dictionary is used in the construction of a second dictionary class variable, a subset pared down from the first, like this:
class C(object):
ALL_ITEMS = dict(a='A', b='B', c='C', d='D', e='E')
SUBSET_X = {k: v for k, v in ALL_ITEMS.items() if k in ('a', 'b', 'd')} # (this works)
SUBSET_Y = {k: ALL_ITEMS[k] for k in ('a', 'b', 'd')} # (this fails)
Pretty simple stuff, but the net effect of executing this code is quite surprising to me. My first approach was the code on line 4, but I had to resort to the solution on line 3 instead. There is something subtle about dictionary comprehension scoping rules that I'm clearly failing to grasp.
Specifically, the error raised in the failing case is:
File "goofy.py", line 4, in <dictcomp>
SUBSET_Y = {k: ALL_ITEMS.get(k) for k in ('a', 'b', 'd')}
NameError: name 'ALL_ITEMS' is not defined
The nature of this error is baffling to me for a few different reasons:
- The assignment to
SUBSET_Y
is a well-formed dictionary comprehension, and references a symbol which should be in-scope and accessible. - In the succeeding case (the assignment to
SUBSET_X
), which is also a dictionary comprehension, the symbolALL_ITEMS
is perfectly well-defined and accessible. Thus, the fact that the raised exception is aNameError
in the failing case seems manifestly wrong. (Or misleading, at best.) - Why would the scoping rules differ for
items()
vs.__getitem__
orget()
? (The same exception occurs replacingALL_ITEMS[k]
withALL_ITEMS.get(k)
in the failure case.)
(Even as a Python developer for over a decade, I've never run into this failure before, which either means I've been lucky or have lived a sheltered existence :^)
The same failure occurs in various 3.6.x CPython versions as well as 2.7.x versions.
EDIT: No, this is not a duplicate of a previous question. That pertained to list comprehensions, and even if one were to project the same explanation to dictionary comprehensions, it doesn't explain the difference between the two cases I cited. And also, it is not a Python 3-only phenomenon.
python class dictionary scoping
During a class definition, a class variable defined as a dictionary is used in the construction of a second dictionary class variable, a subset pared down from the first, like this:
class C(object):
ALL_ITEMS = dict(a='A', b='B', c='C', d='D', e='E')
SUBSET_X = {k: v for k, v in ALL_ITEMS.items() if k in ('a', 'b', 'd')} # (this works)
SUBSET_Y = {k: ALL_ITEMS[k] for k in ('a', 'b', 'd')} # (this fails)
Pretty simple stuff, but the net effect of executing this code is quite surprising to me. My first approach was the code on line 4, but I had to resort to the solution on line 3 instead. There is something subtle about dictionary comprehension scoping rules that I'm clearly failing to grasp.
Specifically, the error raised in the failing case is:
File "goofy.py", line 4, in <dictcomp>
SUBSET_Y = {k: ALL_ITEMS.get(k) for k in ('a', 'b', 'd')}
NameError: name 'ALL_ITEMS' is not defined
The nature of this error is baffling to me for a few different reasons:
- The assignment to
SUBSET_Y
is a well-formed dictionary comprehension, and references a symbol which should be in-scope and accessible. - In the succeeding case (the assignment to
SUBSET_X
), which is also a dictionary comprehension, the symbolALL_ITEMS
is perfectly well-defined and accessible. Thus, the fact that the raised exception is aNameError
in the failing case seems manifestly wrong. (Or misleading, at best.) - Why would the scoping rules differ for
items()
vs.__getitem__
orget()
? (The same exception occurs replacingALL_ITEMS[k]
withALL_ITEMS.get(k)
in the failure case.)
(Even as a Python developer for over a decade, I've never run into this failure before, which either means I've been lucky or have lived a sheltered existence :^)
The same failure occurs in various 3.6.x CPython versions as well as 2.7.x versions.
EDIT: No, this is not a duplicate of a previous question. That pertained to list comprehensions, and even if one were to project the same explanation to dictionary comprehensions, it doesn't explain the difference between the two cases I cited. And also, it is not a Python 3-only phenomenon.
python class dictionary scoping
python class dictionary scoping
edited Nov 12 '18 at 22:06
trenchant
asked Nov 12 '18 at 21:54
trenchanttrenchant
82
82
3
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57
add a comment |
3
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57
3
3
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57
add a comment |
1 Answer
1
active
oldest
votes
There is one minor detail that explains why the first-version works but the second version fails. The reason the second version fails is the same reason that is given in this question, namely, all comprehension constructs (in Python 3, in Python 2, list-comprehensions were implemented differently) create a function scope where all of the local name-bindings occur. However, names in a class scope are not accessible to functions defined inside the class scope. This is why you have to use either self.MY_CLASS_VAR
or MyClass.MY_CLASS_VAR
to access a class variable from a method.
The reason your first case does happen to work is subtle. According to the language reference
The comprehension consists of a single expression followed by at least
one for clause and zero or more for or if clauses. In this case, the
elements of the new container are those that would be produced by
considering each of the for or if clauses a block, nesting from left
to right, and evaluating the expression to produce an element each
time the innermost block is reached.
However, aside from the iterable expression in the leftmost for
clause, the comprehension is executed in a separate implicitly nested
scope. This ensures that names assigned to in the target list don’t
“leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated
directly in the enclosing scope and then passed as an argument to the
implictly nested scope.
So, in the first case, ALL_ITEMS.items()
is in the left-most for-clause, so it is evaluated directly in the enclosing scope, in this case, the class scope, so it happily finds the ALL_ITEMS
name.
So a few things, 1) of course, a fully-qualified name (e.g.,MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!
– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270659%2fpython-class-variable-assignment-using-dictionary-comprehension%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is one minor detail that explains why the first-version works but the second version fails. The reason the second version fails is the same reason that is given in this question, namely, all comprehension constructs (in Python 3, in Python 2, list-comprehensions were implemented differently) create a function scope where all of the local name-bindings occur. However, names in a class scope are not accessible to functions defined inside the class scope. This is why you have to use either self.MY_CLASS_VAR
or MyClass.MY_CLASS_VAR
to access a class variable from a method.
The reason your first case does happen to work is subtle. According to the language reference
The comprehension consists of a single expression followed by at least
one for clause and zero or more for or if clauses. In this case, the
elements of the new container are those that would be produced by
considering each of the for or if clauses a block, nesting from left
to right, and evaluating the expression to produce an element each
time the innermost block is reached.
However, aside from the iterable expression in the leftmost for
clause, the comprehension is executed in a separate implicitly nested
scope. This ensures that names assigned to in the target list don’t
“leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated
directly in the enclosing scope and then passed as an argument to the
implictly nested scope.
So, in the first case, ALL_ITEMS.items()
is in the left-most for-clause, so it is evaluated directly in the enclosing scope, in this case, the class scope, so it happily finds the ALL_ITEMS
name.
So a few things, 1) of course, a fully-qualified name (e.g.,MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!
– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
add a comment |
There is one minor detail that explains why the first-version works but the second version fails. The reason the second version fails is the same reason that is given in this question, namely, all comprehension constructs (in Python 3, in Python 2, list-comprehensions were implemented differently) create a function scope where all of the local name-bindings occur. However, names in a class scope are not accessible to functions defined inside the class scope. This is why you have to use either self.MY_CLASS_VAR
or MyClass.MY_CLASS_VAR
to access a class variable from a method.
The reason your first case does happen to work is subtle. According to the language reference
The comprehension consists of a single expression followed by at least
one for clause and zero or more for or if clauses. In this case, the
elements of the new container are those that would be produced by
considering each of the for or if clauses a block, nesting from left
to right, and evaluating the expression to produce an element each
time the innermost block is reached.
However, aside from the iterable expression in the leftmost for
clause, the comprehension is executed in a separate implicitly nested
scope. This ensures that names assigned to in the target list don’t
“leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated
directly in the enclosing scope and then passed as an argument to the
implictly nested scope.
So, in the first case, ALL_ITEMS.items()
is in the left-most for-clause, so it is evaluated directly in the enclosing scope, in this case, the class scope, so it happily finds the ALL_ITEMS
name.
So a few things, 1) of course, a fully-qualified name (e.g.,MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!
– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
add a comment |
There is one minor detail that explains why the first-version works but the second version fails. The reason the second version fails is the same reason that is given in this question, namely, all comprehension constructs (in Python 3, in Python 2, list-comprehensions were implemented differently) create a function scope where all of the local name-bindings occur. However, names in a class scope are not accessible to functions defined inside the class scope. This is why you have to use either self.MY_CLASS_VAR
or MyClass.MY_CLASS_VAR
to access a class variable from a method.
The reason your first case does happen to work is subtle. According to the language reference
The comprehension consists of a single expression followed by at least
one for clause and zero or more for or if clauses. In this case, the
elements of the new container are those that would be produced by
considering each of the for or if clauses a block, nesting from left
to right, and evaluating the expression to produce an element each
time the innermost block is reached.
However, aside from the iterable expression in the leftmost for
clause, the comprehension is executed in a separate implicitly nested
scope. This ensures that names assigned to in the target list don’t
“leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated
directly in the enclosing scope and then passed as an argument to the
implictly nested scope.
So, in the first case, ALL_ITEMS.items()
is in the left-most for-clause, so it is evaluated directly in the enclosing scope, in this case, the class scope, so it happily finds the ALL_ITEMS
name.
There is one minor detail that explains why the first-version works but the second version fails. The reason the second version fails is the same reason that is given in this question, namely, all comprehension constructs (in Python 3, in Python 2, list-comprehensions were implemented differently) create a function scope where all of the local name-bindings occur. However, names in a class scope are not accessible to functions defined inside the class scope. This is why you have to use either self.MY_CLASS_VAR
or MyClass.MY_CLASS_VAR
to access a class variable from a method.
The reason your first case does happen to work is subtle. According to the language reference
The comprehension consists of a single expression followed by at least
one for clause and zero or more for or if clauses. In this case, the
elements of the new container are those that would be produced by
considering each of the for or if clauses a block, nesting from left
to right, and evaluating the expression to produce an element each
time the innermost block is reached.
However, aside from the iterable expression in the leftmost for
clause, the comprehension is executed in a separate implicitly nested
scope. This ensures that names assigned to in the target list don’t
“leak” into the enclosing scope.
The iterable expression in the leftmost for clause is evaluated
directly in the enclosing scope and then passed as an argument to the
implictly nested scope.
So, in the first case, ALL_ITEMS.items()
is in the left-most for-clause, so it is evaluated directly in the enclosing scope, in this case, the class scope, so it happily finds the ALL_ITEMS
name.
answered Nov 12 '18 at 22:24


juanpa.arrivillagajuanpa.arrivillaga
37.4k33572
37.4k33572
So a few things, 1) of course, a fully-qualified name (e.g.,MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!
– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
add a comment |
So a few things, 1) of course, a fully-qualified name (e.g.,MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!
– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
So a few things, 1) of course, a fully-qualified name (e.g.,
MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!– trenchant
Nov 12 '18 at 23:04
So a few things, 1) of course, a fully-qualified name (e.g.,
MyClass.MY_CLASS_VAR
) is not accessible in expressions assigning class variables inside the class definition, which I doubt you intended to suggest. Also, 2) the same workaround applies equally to Python 2 as in Python 3, so this doesn't appear to be a Python 3-ism as has been alluded to. And finally, 3) yes, your explanation holds water vis-a-vis the spec, but man, is this ever a subtle trip-wire for the unsuspecting!– trenchant
Nov 12 '18 at 23:04
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
@trenchant 1) yes, MyClass won't be available during class definition time because it doesn't exist, and the class scope only exists during the definition. 2) for list-comprehensions, this would apply only in Python 3, because in Python 2 they had an implementation that didn't create a class scope but instead had a "leaky" scope. Dict and set comprehension never suffered from this, and in Python 3, list comprehension were re-implemented to work like set and dict comprehension-constructs already did 3) yeah it's subtle. I just avoid comprehension-constructs inside class scopes.
– juanpa.arrivillaga
Nov 12 '18 at 23:08
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53270659%2fpython-class-variable-assignment-using-dictionary-comprehension%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3,hgXkqfqyf7bUXDMzRs1Aac7ulrGrLSyZ5DOtIdFl8 8TGtNA6Bb5IFZl4,jc,ZPIG hrvk
3
I don't see ALL_KEYS anywhere in your code. Is the error referencing ALL_ITEMS?
– pydude
Nov 12 '18 at 21:57