How to upload file from React App to Express API using axios?
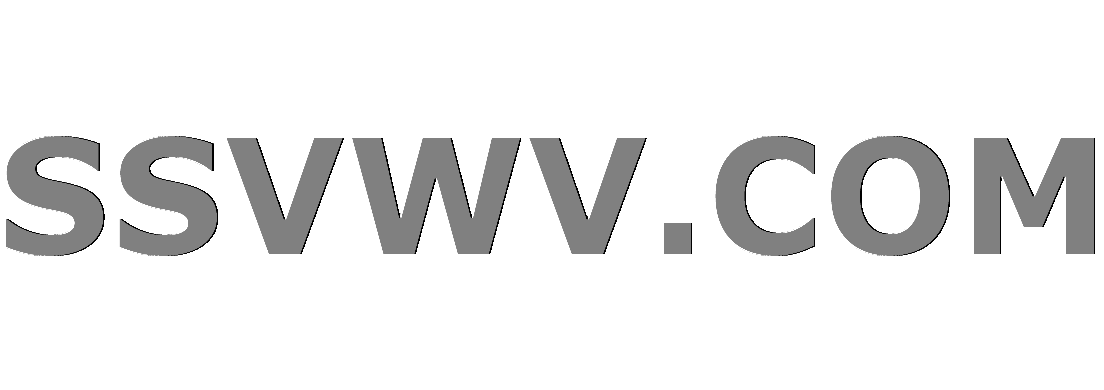
Multi tool use
I create react-app before, and made a form for post. And i want post can be upload image (thumbnail). All data i sent to API, which i created with Express. But if i console log the thumbnail, api got undefined result.
The 1st inside my post api, i create function to initial the store using multer:
const storage = multer.diskStorage({
destination: "./public/uploads/",
filename: function(req, file, cb){
cb(null,"IMAGE-" + Date.now() + path.extname(file.originalname))
}
})
const upload = multer({
storage: storage,
limits:{fileSize: 1000000},
}).single("thumbnail")
Then i created the route for edit post (example):
router.put('/post', (req, res) => {
if(!req.query.id){
return res.status(400).send('Missing URL parameter: id')
}
upload(req, res, (err) => {
console.log(req.file)
})
})
Then, here's my code from react-app:
import React, { Component } from 'react'
import Swal from 'sweetalert2'
import { Alert, Button, Card, CardBody, CardHeader, Col, Form, FormGroup, Label, Input, Row } from 'reactstrap'
import Editor from 'react-simplemde-editor'
import dateFormat from 'dateformat'
import 'simplemde/dist/simplemde.min.css'
import AuthService from '../../../auth'
import './EditPosts.scss'
export default class EditPosts extends Component {
constructor(props){
super(props)
let now = new Date()
this.state = {
postId: this.props.match.params.id,
title: '',
content: '',
description: '',
thumbnail: '',
author: '',
updatedAt: dateFormat(now, "mmmm dS, yyyy"),
imagePreviewUrl: '',
editError: ''
}
this.onTextboxChangeTitle = this.onTextboxChangeTitle.bind(this)
this.onTextboxChangeContent = this.onTextboxChangeContent.bind(this)
this.onTextboxChangeDescription = this.onTextboxChangeDescription.bind(this)
this.onUploadboxChange = this.onUploadboxChange.bind(this)
this.onEdit = this.onEdit.bind(this)
this.Auth = new AuthService()
}
onTextboxChangeTitle(event){
this.setState({
title: event.target.value
})
}
onTextboxChangeContent(value) {
this.setState({
content: value
})
}
onTextboxChangeDescription(event){
this.setState({
description: event.target.value
})
}
onUploadboxChange(e){
e.preventDefault()
let reader = new FileReader()
let thumbnail = e.target.files[0]
reader.onloadend = () => {
this.setState({
thumbnail: thumbnail,
imagePreviewUrl: reader.result
});
}
reader.readAsDataURL(thumbnail)
}
componentDidMount(){
this.getPost()
}
getPost(){
this.Auth.post(this.state.postId)
.then((res) => {
this.setState({
title: res.title,
content: res.content,
description: res.description,
thumbnail: res.thumbnail,
author: res.author
})
})
}
onEdit(e){
e.preventDefault()
const {
postId,
title,
content,
description,
thumbnail,
author,
updatedAt
} = this.state
const formData = new FormData()
formData.append('thumbnail', thumbnail)
this.Auth.edit(postId, title, content, description, formData, author, updatedAt)
.then(res => {
if(res.status >= 200 && res.status < 300){
Swal({
position: 'top-end',
type: 'success',
title: 'Your post has been update!',
showConfirmButton: false,
timer: 1500
}).then(res =>{
// window.location.href = '/posts'
})
}
})
}
render(){
let { imagePreviewUrl, editError, title, content, description, thumbnail } = this.state
let $imagePreview = thumbnail
if (imagePreviewUrl) {
$imagePreview = (<img src={imagePreviewUrl} alt="" />)
} else {
$imagePreview = (<div className="previewText">Please select an Image for Post Cover</div>);
}
return(
<div className="animated fadeIn">
<Card>
<CardHeader>Edit post</CardHeader>
<CardBody>
<Form onSubmit={this.onEdit}>
{
(editError) ? (
<Alert color="danger">{editError}</Alert>
): null
}
<Row>
<Col md={7}>
<FormGroup>
<Label for="title">Edit Title</Label>
<Input type="text" autoComplete="title" placeholder="Post Title" value={ (title) ? title : '' } onChange={this.onTextboxChangeTitle} />
</FormGroup>
<FormGroup>
<Label for="description">Description</Label>
<Input type="textarea" autoComplete="description" placeholder="Description" value={ (description) ? description : '' } onChange={this.onTextboxChangeDescription} />
</FormGroup>
<FormGroup>
<Label for="content">Content</Label>
<Editor
autoComplete="content"
value={ (content) ? content : '' }
onChange={this.onTextboxChangeContent}
options={
{ spellChecker: false }
}
/>
</FormGroup>
</Col>
<Col md={5}>
<FormGroup>
<Label for="thumbnail">Image Cover</Label>
<Input type="file" autoComplete="thumbnail" onChange={this.onUploadboxChange} />
</FormGroup>
<div className="imgPreview">
{$imagePreview}
</div>
</Col>
</Row>
<Row>
<Col className="text-right">
<Button color="primary" className="px-4" type="submit" value="Submit">Save</Button>
</Col>
</Row>
</Form>
</CardBody>
</Card>
</div>
)
}
}
Here's function to connect to api:
edit(id, title, content, description, thumbnail, author, updatedAt){
return axios.put('http://localhost:3000/post?id='+id, {
title,
content,
description,
thumbnail,
author,
updatedAt
})
.then(this._checkStatus)
.then(res => {
return res
})
.catch(err => {
throw err;
})
}
i always got undefined when upload the image
Did i missed something in process upload the image? If someone know, please help. Thanks.
reactjs api express create-react-app multer
add a comment |
I create react-app before, and made a form for post. And i want post can be upload image (thumbnail). All data i sent to API, which i created with Express. But if i console log the thumbnail, api got undefined result.
The 1st inside my post api, i create function to initial the store using multer:
const storage = multer.diskStorage({
destination: "./public/uploads/",
filename: function(req, file, cb){
cb(null,"IMAGE-" + Date.now() + path.extname(file.originalname))
}
})
const upload = multer({
storage: storage,
limits:{fileSize: 1000000},
}).single("thumbnail")
Then i created the route for edit post (example):
router.put('/post', (req, res) => {
if(!req.query.id){
return res.status(400).send('Missing URL parameter: id')
}
upload(req, res, (err) => {
console.log(req.file)
})
})
Then, here's my code from react-app:
import React, { Component } from 'react'
import Swal from 'sweetalert2'
import { Alert, Button, Card, CardBody, CardHeader, Col, Form, FormGroup, Label, Input, Row } from 'reactstrap'
import Editor from 'react-simplemde-editor'
import dateFormat from 'dateformat'
import 'simplemde/dist/simplemde.min.css'
import AuthService from '../../../auth'
import './EditPosts.scss'
export default class EditPosts extends Component {
constructor(props){
super(props)
let now = new Date()
this.state = {
postId: this.props.match.params.id,
title: '',
content: '',
description: '',
thumbnail: '',
author: '',
updatedAt: dateFormat(now, "mmmm dS, yyyy"),
imagePreviewUrl: '',
editError: ''
}
this.onTextboxChangeTitle = this.onTextboxChangeTitle.bind(this)
this.onTextboxChangeContent = this.onTextboxChangeContent.bind(this)
this.onTextboxChangeDescription = this.onTextboxChangeDescription.bind(this)
this.onUploadboxChange = this.onUploadboxChange.bind(this)
this.onEdit = this.onEdit.bind(this)
this.Auth = new AuthService()
}
onTextboxChangeTitle(event){
this.setState({
title: event.target.value
})
}
onTextboxChangeContent(value) {
this.setState({
content: value
})
}
onTextboxChangeDescription(event){
this.setState({
description: event.target.value
})
}
onUploadboxChange(e){
e.preventDefault()
let reader = new FileReader()
let thumbnail = e.target.files[0]
reader.onloadend = () => {
this.setState({
thumbnail: thumbnail,
imagePreviewUrl: reader.result
});
}
reader.readAsDataURL(thumbnail)
}
componentDidMount(){
this.getPost()
}
getPost(){
this.Auth.post(this.state.postId)
.then((res) => {
this.setState({
title: res.title,
content: res.content,
description: res.description,
thumbnail: res.thumbnail,
author: res.author
})
})
}
onEdit(e){
e.preventDefault()
const {
postId,
title,
content,
description,
thumbnail,
author,
updatedAt
} = this.state
const formData = new FormData()
formData.append('thumbnail', thumbnail)
this.Auth.edit(postId, title, content, description, formData, author, updatedAt)
.then(res => {
if(res.status >= 200 && res.status < 300){
Swal({
position: 'top-end',
type: 'success',
title: 'Your post has been update!',
showConfirmButton: false,
timer: 1500
}).then(res =>{
// window.location.href = '/posts'
})
}
})
}
render(){
let { imagePreviewUrl, editError, title, content, description, thumbnail } = this.state
let $imagePreview = thumbnail
if (imagePreviewUrl) {
$imagePreview = (<img src={imagePreviewUrl} alt="" />)
} else {
$imagePreview = (<div className="previewText">Please select an Image for Post Cover</div>);
}
return(
<div className="animated fadeIn">
<Card>
<CardHeader>Edit post</CardHeader>
<CardBody>
<Form onSubmit={this.onEdit}>
{
(editError) ? (
<Alert color="danger">{editError}</Alert>
): null
}
<Row>
<Col md={7}>
<FormGroup>
<Label for="title">Edit Title</Label>
<Input type="text" autoComplete="title" placeholder="Post Title" value={ (title) ? title : '' } onChange={this.onTextboxChangeTitle} />
</FormGroup>
<FormGroup>
<Label for="description">Description</Label>
<Input type="textarea" autoComplete="description" placeholder="Description" value={ (description) ? description : '' } onChange={this.onTextboxChangeDescription} />
</FormGroup>
<FormGroup>
<Label for="content">Content</Label>
<Editor
autoComplete="content"
value={ (content) ? content : '' }
onChange={this.onTextboxChangeContent}
options={
{ spellChecker: false }
}
/>
</FormGroup>
</Col>
<Col md={5}>
<FormGroup>
<Label for="thumbnail">Image Cover</Label>
<Input type="file" autoComplete="thumbnail" onChange={this.onUploadboxChange} />
</FormGroup>
<div className="imgPreview">
{$imagePreview}
</div>
</Col>
</Row>
<Row>
<Col className="text-right">
<Button color="primary" className="px-4" type="submit" value="Submit">Save</Button>
</Col>
</Row>
</Form>
</CardBody>
</Card>
</div>
)
}
}
Here's function to connect to api:
edit(id, title, content, description, thumbnail, author, updatedAt){
return axios.put('http://localhost:3000/post?id='+id, {
title,
content,
description,
thumbnail,
author,
updatedAt
})
.then(this._checkStatus)
.then(res => {
return res
})
.catch(err => {
throw err;
})
}
i always got undefined when upload the image
Did i missed something in process upload the image? If someone know, please help. Thanks.
reactjs api express create-react-app multer
add a comment |
I create react-app before, and made a form for post. And i want post can be upload image (thumbnail). All data i sent to API, which i created with Express. But if i console log the thumbnail, api got undefined result.
The 1st inside my post api, i create function to initial the store using multer:
const storage = multer.diskStorage({
destination: "./public/uploads/",
filename: function(req, file, cb){
cb(null,"IMAGE-" + Date.now() + path.extname(file.originalname))
}
})
const upload = multer({
storage: storage,
limits:{fileSize: 1000000},
}).single("thumbnail")
Then i created the route for edit post (example):
router.put('/post', (req, res) => {
if(!req.query.id){
return res.status(400).send('Missing URL parameter: id')
}
upload(req, res, (err) => {
console.log(req.file)
})
})
Then, here's my code from react-app:
import React, { Component } from 'react'
import Swal from 'sweetalert2'
import { Alert, Button, Card, CardBody, CardHeader, Col, Form, FormGroup, Label, Input, Row } from 'reactstrap'
import Editor from 'react-simplemde-editor'
import dateFormat from 'dateformat'
import 'simplemde/dist/simplemde.min.css'
import AuthService from '../../../auth'
import './EditPosts.scss'
export default class EditPosts extends Component {
constructor(props){
super(props)
let now = new Date()
this.state = {
postId: this.props.match.params.id,
title: '',
content: '',
description: '',
thumbnail: '',
author: '',
updatedAt: dateFormat(now, "mmmm dS, yyyy"),
imagePreviewUrl: '',
editError: ''
}
this.onTextboxChangeTitle = this.onTextboxChangeTitle.bind(this)
this.onTextboxChangeContent = this.onTextboxChangeContent.bind(this)
this.onTextboxChangeDescription = this.onTextboxChangeDescription.bind(this)
this.onUploadboxChange = this.onUploadboxChange.bind(this)
this.onEdit = this.onEdit.bind(this)
this.Auth = new AuthService()
}
onTextboxChangeTitle(event){
this.setState({
title: event.target.value
})
}
onTextboxChangeContent(value) {
this.setState({
content: value
})
}
onTextboxChangeDescription(event){
this.setState({
description: event.target.value
})
}
onUploadboxChange(e){
e.preventDefault()
let reader = new FileReader()
let thumbnail = e.target.files[0]
reader.onloadend = () => {
this.setState({
thumbnail: thumbnail,
imagePreviewUrl: reader.result
});
}
reader.readAsDataURL(thumbnail)
}
componentDidMount(){
this.getPost()
}
getPost(){
this.Auth.post(this.state.postId)
.then((res) => {
this.setState({
title: res.title,
content: res.content,
description: res.description,
thumbnail: res.thumbnail,
author: res.author
})
})
}
onEdit(e){
e.preventDefault()
const {
postId,
title,
content,
description,
thumbnail,
author,
updatedAt
} = this.state
const formData = new FormData()
formData.append('thumbnail', thumbnail)
this.Auth.edit(postId, title, content, description, formData, author, updatedAt)
.then(res => {
if(res.status >= 200 && res.status < 300){
Swal({
position: 'top-end',
type: 'success',
title: 'Your post has been update!',
showConfirmButton: false,
timer: 1500
}).then(res =>{
// window.location.href = '/posts'
})
}
})
}
render(){
let { imagePreviewUrl, editError, title, content, description, thumbnail } = this.state
let $imagePreview = thumbnail
if (imagePreviewUrl) {
$imagePreview = (<img src={imagePreviewUrl} alt="" />)
} else {
$imagePreview = (<div className="previewText">Please select an Image for Post Cover</div>);
}
return(
<div className="animated fadeIn">
<Card>
<CardHeader>Edit post</CardHeader>
<CardBody>
<Form onSubmit={this.onEdit}>
{
(editError) ? (
<Alert color="danger">{editError}</Alert>
): null
}
<Row>
<Col md={7}>
<FormGroup>
<Label for="title">Edit Title</Label>
<Input type="text" autoComplete="title" placeholder="Post Title" value={ (title) ? title : '' } onChange={this.onTextboxChangeTitle} />
</FormGroup>
<FormGroup>
<Label for="description">Description</Label>
<Input type="textarea" autoComplete="description" placeholder="Description" value={ (description) ? description : '' } onChange={this.onTextboxChangeDescription} />
</FormGroup>
<FormGroup>
<Label for="content">Content</Label>
<Editor
autoComplete="content"
value={ (content) ? content : '' }
onChange={this.onTextboxChangeContent}
options={
{ spellChecker: false }
}
/>
</FormGroup>
</Col>
<Col md={5}>
<FormGroup>
<Label for="thumbnail">Image Cover</Label>
<Input type="file" autoComplete="thumbnail" onChange={this.onUploadboxChange} />
</FormGroup>
<div className="imgPreview">
{$imagePreview}
</div>
</Col>
</Row>
<Row>
<Col className="text-right">
<Button color="primary" className="px-4" type="submit" value="Submit">Save</Button>
</Col>
</Row>
</Form>
</CardBody>
</Card>
</div>
)
}
}
Here's function to connect to api:
edit(id, title, content, description, thumbnail, author, updatedAt){
return axios.put('http://localhost:3000/post?id='+id, {
title,
content,
description,
thumbnail,
author,
updatedAt
})
.then(this._checkStatus)
.then(res => {
return res
})
.catch(err => {
throw err;
})
}
i always got undefined when upload the image
Did i missed something in process upload the image? If someone know, please help. Thanks.
reactjs api express create-react-app multer
I create react-app before, and made a form for post. And i want post can be upload image (thumbnail). All data i sent to API, which i created with Express. But if i console log the thumbnail, api got undefined result.
The 1st inside my post api, i create function to initial the store using multer:
const storage = multer.diskStorage({
destination: "./public/uploads/",
filename: function(req, file, cb){
cb(null,"IMAGE-" + Date.now() + path.extname(file.originalname))
}
})
const upload = multer({
storage: storage,
limits:{fileSize: 1000000},
}).single("thumbnail")
Then i created the route for edit post (example):
router.put('/post', (req, res) => {
if(!req.query.id){
return res.status(400).send('Missing URL parameter: id')
}
upload(req, res, (err) => {
console.log(req.file)
})
})
Then, here's my code from react-app:
import React, { Component } from 'react'
import Swal from 'sweetalert2'
import { Alert, Button, Card, CardBody, CardHeader, Col, Form, FormGroup, Label, Input, Row } from 'reactstrap'
import Editor from 'react-simplemde-editor'
import dateFormat from 'dateformat'
import 'simplemde/dist/simplemde.min.css'
import AuthService from '../../../auth'
import './EditPosts.scss'
export default class EditPosts extends Component {
constructor(props){
super(props)
let now = new Date()
this.state = {
postId: this.props.match.params.id,
title: '',
content: '',
description: '',
thumbnail: '',
author: '',
updatedAt: dateFormat(now, "mmmm dS, yyyy"),
imagePreviewUrl: '',
editError: ''
}
this.onTextboxChangeTitle = this.onTextboxChangeTitle.bind(this)
this.onTextboxChangeContent = this.onTextboxChangeContent.bind(this)
this.onTextboxChangeDescription = this.onTextboxChangeDescription.bind(this)
this.onUploadboxChange = this.onUploadboxChange.bind(this)
this.onEdit = this.onEdit.bind(this)
this.Auth = new AuthService()
}
onTextboxChangeTitle(event){
this.setState({
title: event.target.value
})
}
onTextboxChangeContent(value) {
this.setState({
content: value
})
}
onTextboxChangeDescription(event){
this.setState({
description: event.target.value
})
}
onUploadboxChange(e){
e.preventDefault()
let reader = new FileReader()
let thumbnail = e.target.files[0]
reader.onloadend = () => {
this.setState({
thumbnail: thumbnail,
imagePreviewUrl: reader.result
});
}
reader.readAsDataURL(thumbnail)
}
componentDidMount(){
this.getPost()
}
getPost(){
this.Auth.post(this.state.postId)
.then((res) => {
this.setState({
title: res.title,
content: res.content,
description: res.description,
thumbnail: res.thumbnail,
author: res.author
})
})
}
onEdit(e){
e.preventDefault()
const {
postId,
title,
content,
description,
thumbnail,
author,
updatedAt
} = this.state
const formData = new FormData()
formData.append('thumbnail', thumbnail)
this.Auth.edit(postId, title, content, description, formData, author, updatedAt)
.then(res => {
if(res.status >= 200 && res.status < 300){
Swal({
position: 'top-end',
type: 'success',
title: 'Your post has been update!',
showConfirmButton: false,
timer: 1500
}).then(res =>{
// window.location.href = '/posts'
})
}
})
}
render(){
let { imagePreviewUrl, editError, title, content, description, thumbnail } = this.state
let $imagePreview = thumbnail
if (imagePreviewUrl) {
$imagePreview = (<img src={imagePreviewUrl} alt="" />)
} else {
$imagePreview = (<div className="previewText">Please select an Image for Post Cover</div>);
}
return(
<div className="animated fadeIn">
<Card>
<CardHeader>Edit post</CardHeader>
<CardBody>
<Form onSubmit={this.onEdit}>
{
(editError) ? (
<Alert color="danger">{editError}</Alert>
): null
}
<Row>
<Col md={7}>
<FormGroup>
<Label for="title">Edit Title</Label>
<Input type="text" autoComplete="title" placeholder="Post Title" value={ (title) ? title : '' } onChange={this.onTextboxChangeTitle} />
</FormGroup>
<FormGroup>
<Label for="description">Description</Label>
<Input type="textarea" autoComplete="description" placeholder="Description" value={ (description) ? description : '' } onChange={this.onTextboxChangeDescription} />
</FormGroup>
<FormGroup>
<Label for="content">Content</Label>
<Editor
autoComplete="content"
value={ (content) ? content : '' }
onChange={this.onTextboxChangeContent}
options={
{ spellChecker: false }
}
/>
</FormGroup>
</Col>
<Col md={5}>
<FormGroup>
<Label for="thumbnail">Image Cover</Label>
<Input type="file" autoComplete="thumbnail" onChange={this.onUploadboxChange} />
</FormGroup>
<div className="imgPreview">
{$imagePreview}
</div>
</Col>
</Row>
<Row>
<Col className="text-right">
<Button color="primary" className="px-4" type="submit" value="Submit">Save</Button>
</Col>
</Row>
</Form>
</CardBody>
</Card>
</div>
)
}
}
Here's function to connect to api:
edit(id, title, content, description, thumbnail, author, updatedAt){
return axios.put('http://localhost:3000/post?id='+id, {
title,
content,
description,
thumbnail,
author,
updatedAt
})
.then(this._checkStatus)
.then(res => {
return res
})
.catch(err => {
throw err;
})
}
i always got undefined when upload the image
Did i missed something in process upload the image? If someone know, please help. Thanks.
reactjs api express create-react-app multer
reactjs api express create-react-app multer
asked Nov 13 '18 at 16:06
RezaReza
317
317
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284993%2fhow-to-upload-file-from-react-app-to-express-api-using-axios%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284993%2fhow-to-upload-file-from-react-app-to-express-api-using-axios%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pDAS1fL,WLU K ZAYH 50